Top Related Projects
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
React components for efficiently rendering large lists and tabular data
The most powerful virtual list component for React
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
A tiny but mighty 3kb list virtualization library, with zero dependencies 💪 Supports variable heights/widths, sticky items, scrolling to index, and more!
Quick Overview
React Window is a lightweight, efficient windowing library for React applications. It enables rendering large lists and tabular data with improved performance by only rendering visible items. This library is particularly useful for handling large datasets in web applications.
Pros
- Significantly improves performance for large lists and grids
- Supports both fixed-size and variable-size lists and grids
- Lightweight and has minimal dependencies
- Easy to integrate with existing React applications
Cons
- Limited built-in features compared to some other windowing libraries
- May require additional configuration for complex use cases
- Learning curve for developers new to windowing concepts
- Some edge cases might require custom implementations
Code Examples
- Basic fixed-size list:
import { FixedSizeList } from 'react-window';
const Row = ({ index, style }) => (
<div style={style}>Row {index}</div>
);
const Example = () => (
<FixedSizeList
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{Row}
</FixedSizeList>
);
- Variable-size grid:
import { VariableSizeGrid } from 'react-window';
const Cell = ({ columnIndex, rowIndex, style }) => (
<div style={style}>
Item {rowIndex},{columnIndex}
</div>
);
const Example = () => (
<VariableSizeGrid
columnCount={1000}
columnWidth={index => (index % 3 === 0 ? 100 : 50)}
height={150}
rowCount={1000}
rowHeight={index => (index % 2 === 0 ? 50 : 30)}
width={300}
>
{Cell}
</VariableSizeGrid>
);
- Using with dynamic content:
import { FixedSizeList } from 'react-window';
import AutoSizer from 'react-virtualized-auto-sizer';
const Row = ({ index, style, data }) => (
<div style={style}>{data[index]}</div>
);
const Example = ({ items }) => (
<AutoSizer>
{({ height, width }) => (
<FixedSizeList
height={height}
itemCount={items.length}
itemSize={35}
width={width}
itemData={items}
>
{Row}
</FixedSizeList>
)}
</AutoSizer>
);
Getting Started
-
Install the package:
npm install react-window
-
Import and use in your React component:
import { FixedSizeList } from 'react-window'; const MyList = () => ( <FixedSizeList height={400} width={300} itemSize={50} itemCount={1000} > {({ index, style }) => ( <div style={style}>Row {index}</div> )} </FixedSizeList> );
-
Customize as needed for your specific use case, considering different list types (FixedSizeList, VariableSizeList) or grid components (FixedSizeGrid, VariableSizeGrid) based on your requirements.
Competitor Comparisons
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
Pros of Virtual
- Framework-agnostic, supporting React, Vue, Solid, and more
- More flexible API with advanced features like dynamic item sizes and sticky items
- Active development and community support
Cons of Virtual
- Steeper learning curve due to more complex API
- Potentially larger bundle size due to additional features
Code Comparison
React Window:
import { FixedSizeList } from 'react-window';
const Example = () => (
<FixedSizeList
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{({ index, style }) => <div style={style}>Row {index}</div>}
</FixedSizeList>
);
Virtual:
import { useVirtualizer } from '@tanstack/react-virtual';
const Example = () => {
const virtualizer = useVirtualizer({
count: 1000,
getScrollElement: () => parentRef.current,
estimateSize: () => 35,
});
return (
<div ref={parentRef} style={{ height: '150px', overflow: 'auto' }}>
<div style={{ height: `${virtualizer.getTotalSize()}px` }}>
{virtualizer.getVirtualItems().map((virtualItem) => (
<div key={virtualItem.key} style={{
position: 'absolute',
top: 0,
left: 0,
width: '100%',
height: `${virtualItem.size}px`,
transform: `translateY(${virtualItem.start}px)`,
}}>
Row {virtualItem.index}
</div>
))}
</div>
</div>
);
};
React components for efficiently rendering large lists and tabular data
Pros of react-virtualized
- More comprehensive feature set, including support for various layouts (Grid, List, Table, etc.)
- Offers additional utilities like CellMeasurer for dynamic content sizing
- Mature project with extensive documentation and community support
Cons of react-virtualized
- Larger bundle size due to its comprehensive nature
- More complex API, which can lead to a steeper learning curve
- Higher memory usage, especially for large datasets
Code Comparison
react-virtualized:
import { List } from 'react-virtualized';
<List
width={300}
height={300}
rowCount={1000}
rowHeight={20}
rowRenderer={({ index, key, style }) => (
<div key={key} style={style}>Row {index}</div>
)}
/>
react-window:
import { FixedSizeList } from 'react-window';
<FixedSizeList
height={300}
width={300}
itemCount={1000}
itemSize={20}
>
{({ index, style }) => <div style={style}>Row {index}</div>}
</FixedSizeList>
Both libraries serve similar purposes, but react-window is a more lightweight and focused alternative to react-virtualized. It offers improved performance and a simpler API, making it easier to use for basic virtualization needs. However, react-virtualized remains a powerful choice for complex use cases requiring advanced features and layouts.
The most powerful virtual list component for React
Pros of react-virtuoso
- More flexible and feature-rich, supporting dynamic heights and complex layouts
- Built-in support for grouping, sticky headers, and footer
- Easier to use with less boilerplate code required
Cons of react-virtuoso
- Slightly larger bundle size due to additional features
- May have a steeper learning curve for simpler use cases
- Less established in the React ecosystem compared to react-window
Code Comparison
react-window:
import { FixedSizeList } from 'react-window';
<FixedSizeList
height={400}
itemCount={1000}
itemSize={35}
width={300}
>
{({ index, style }) => <div style={style}>Row {index}</div>}
</FixedSizeList>
react-virtuoso:
import { Virtuoso } from 'react-virtuoso';
<Virtuoso
style={{ height: '400px', width: '300px' }}
totalCount={1000}
itemContent={index => <div>Row {index}</div>}
/>
Both libraries aim to efficiently render large lists in React applications, but react-virtuoso offers more built-in features and flexibility at the cost of a slightly larger bundle size. react-window is more lightweight and may be preferable for simpler use cases, while react-virtuoso shines in complex scenarios with dynamic content and layouts.
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
Pros of Virtual
- Framework-agnostic, supporting React, Vue, Solid, and more
- More flexible API with advanced features like dynamic item sizes and sticky items
- Active development and community support
Cons of Virtual
- Steeper learning curve due to more complex API
- Potentially larger bundle size due to additional features
Code Comparison
React Window:
import { FixedSizeList } from 'react-window';
const Example = () => (
<FixedSizeList
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{({ index, style }) => <div style={style}>Row {index}</div>}
</FixedSizeList>
);
Virtual:
import { useVirtualizer } from '@tanstack/react-virtual';
const Example = () => {
const virtualizer = useVirtualizer({
count: 1000,
getScrollElement: () => parentRef.current,
estimateSize: () => 35,
});
return (
<div ref={parentRef} style={{ height: '150px', overflow: 'auto' }}>
<div style={{ height: `${virtualizer.getTotalSize()}px` }}>
{virtualizer.getVirtualItems().map((virtualItem) => (
<div key={virtualItem.key} style={{
position: 'absolute',
top: 0,
left: 0,
width: '100%',
height: `${virtualItem.size}px`,
transform: `translateY(${virtualItem.start}px)`,
}}>
Row {virtualItem.index}
</div>
))}
</div>
</div>
);
};
A tiny but mighty 3kb list virtualization library, with zero dependencies 💪 Supports variable heights/widths, sticky items, scrolling to index, and more!
Pros of react-tiny-virtual-list
- Smaller bundle size, making it more lightweight for simpler use cases
- Easier to set up and use for basic virtualization needs
- Supports both fixed and variable height items out of the box
Cons of react-tiny-virtual-list
- Less actively maintained compared to react-window
- Fewer features and customization options
- Limited support for horizontal lists and grid layouts
Code Comparison
react-tiny-virtual-list:
import VirtualList from 'react-tiny-virtual-list';
<VirtualList
width={300}
height={600}
itemCount={10000}
itemSize={50}
renderItem={({ index, style }) => (
<div style={style}>Item {index}</div>
)}
/>
react-window:
import { FixedSizeList } from 'react-window';
<FixedSizeList
height={600}
width={300}
itemCount={10000}
itemSize={50}
>
{({ index, style }) => (
<div style={style}>Item {index}</div>
)}
</FixedSizeList>
Both libraries offer similar basic functionality for rendering large lists efficiently. react-tiny-virtual-list provides a simpler API, while react-window offers more advanced features and better performance for complex use cases. The choice between them depends on the specific requirements of your project and the level of customization needed.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
react-window
React components for efficiently rendering large lists and tabular data
If you like this project, ð become a sponsor or â buy me a coffee
React window works by only rendering part of a large data set (just enough to fill the viewport). This helps address some common performance bottlenecks:
- It reduces the amount of work (and time) required to render the initial view and to process updates.
- It reduces the memory footprint by avoiding over-allocation of DOM nodes.
Sponsors
The following wonderful companies have sponsored react-window:
Learn more about becoming a sponsor!
Install
# Yarn
yarn add react-window
# NPM
npm install --save react-window
Usage
Learn more at react-window.now.sh:
Related libraries
react-virtualized-auto-sizer
: HOC that grows to fit all of the available space and passes the width and height values to its child.react-window-infinite-loader
: Helps break large data sets down into chunks that can be just-in-time loaded as they are scrolled into view. It can also be used to create infinite loading lists (e.g. Facebook or Twitter).react-vtree
: Lightweight and flexible solution to render large tree structures (e.g., file system).
Frequently asked questions
How is react-window
different from react-virtualized
?
I wrote react-virtualized
several years ago. At the time, I was new to both React and the concept of windowing. Because of this, I made a few API decisions that I later came to regret. One of these was adding too many non-essential features and components. Once you add something to an open source project, removing it is pretty painful for users.
react-window
is a complete rewrite of react-virtualized
. I didn't try to solve as many problems or support as many use cases. Instead I focused on making the package smaller1 and faster. I also put a lot of thought into making the API (and documentation) as beginner-friendly as possible (with the caveat that windowing is still kind of an advanced use case).
If react-window
provides the functionality your project needs, I would strongly recommend using it instead of react-virtualized
. However if you need features that only react-virtualized
provides, you have two options:
- Use
react-virtualized
. (It's still widely used by a lot of successful projects!) - Create a component that decorates one of the
react-window
primitives and adds the functionality you need. You may even want to release this component to NPM (as its own, standalone package)! ð
1 - Adding a react-virtualized
list to a CRA project increases the (gzipped) build size by ~33.5 KB. Adding a react-window
list to a CRA project increases the (gzipped) build size by <2 KB.
Can a list or a grid fill 100% the width or height of a page?
Yes. I recommend using the react-virtualized-auto-sizer
package:
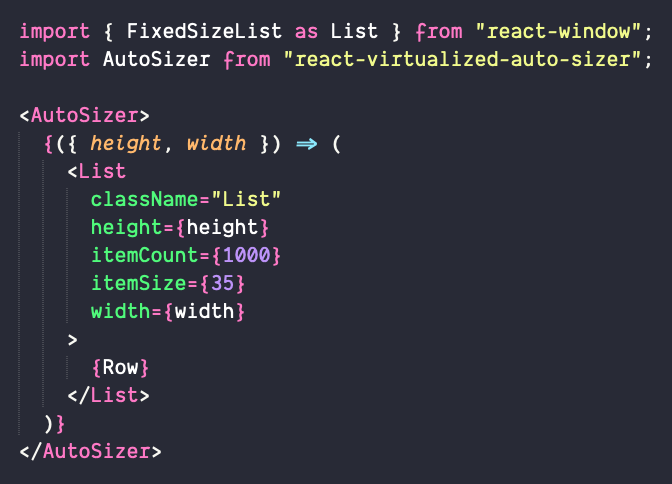
Here's a Code Sandbox demo.
Why is my list blank when I scroll?
If your list looks something like this...
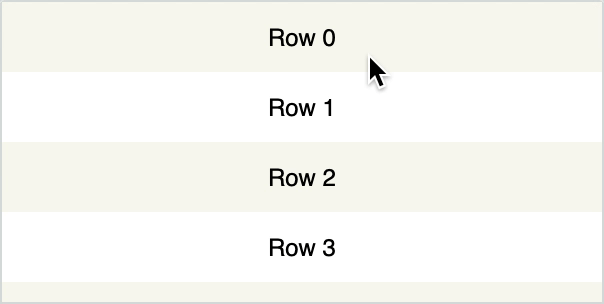
...then you probably forgot to use the style
parameter! Libraries like react-window work by absolutely positioning the list items (via an inline style), so don't forget to attach it to the DOM element you render!
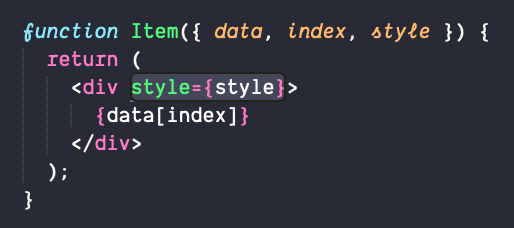
Can I lazy load data for my list?
Yes. I recommend using the react-window-infinite-loader
package:
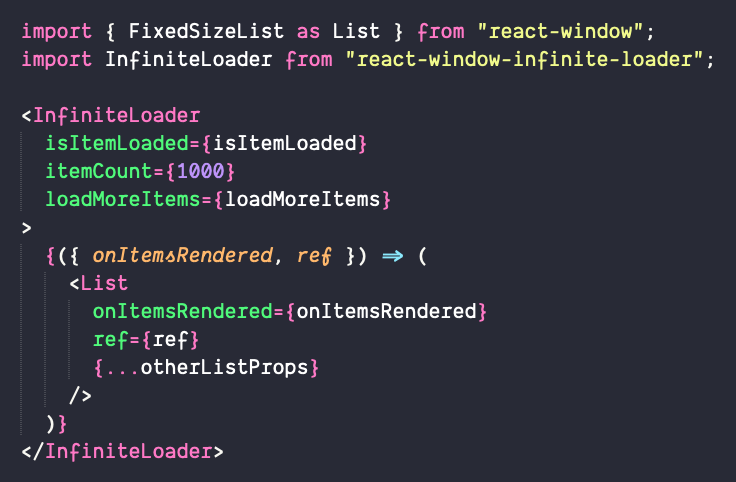
Here's a Code Sandbox demo.
Can I attach custom properties or event handlers?
Yes, using the outerElementType
prop.
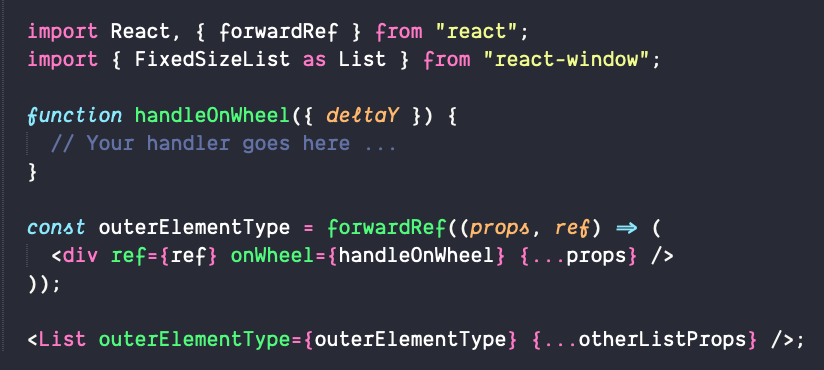
Here's a Code Sandbox demo.
Can I add padding to the top and bottom of a list?
Yes, although it requires a bit of inline styling.
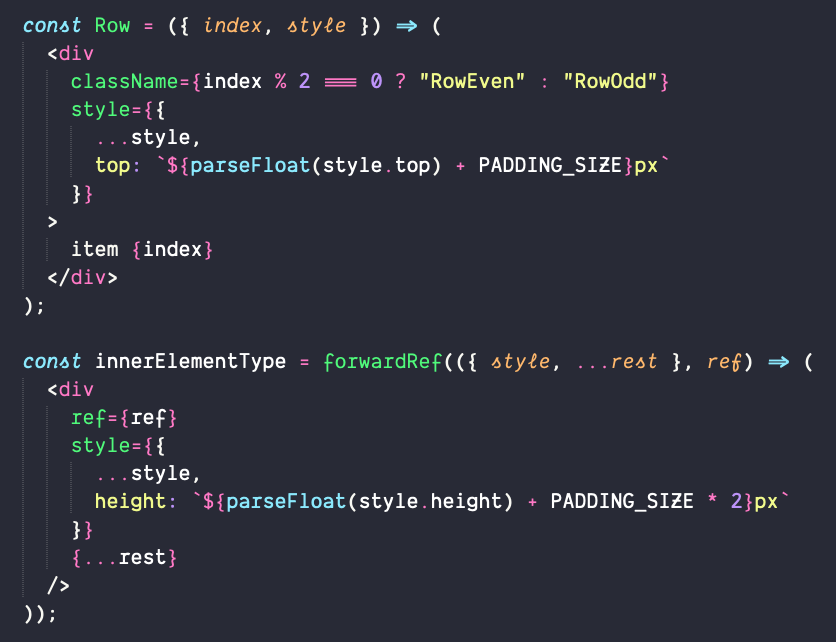
Here's a Code Sandbox demo.
Can I add gutter or padding between items?
Yes, although it requires a bit of inline styling.
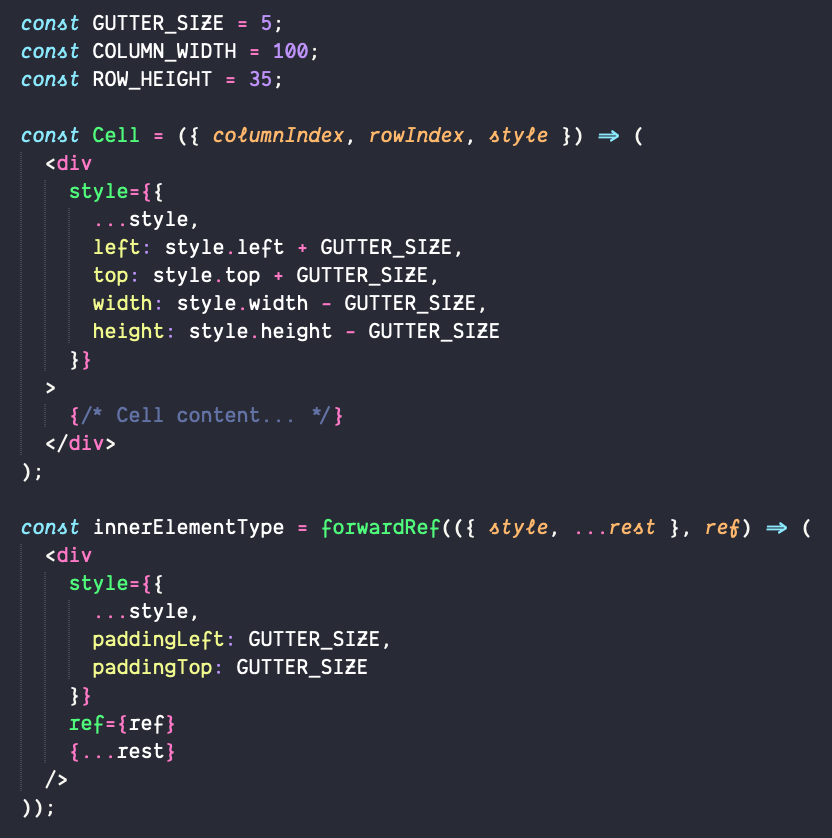
Here's a Code Sandbox demo.
Does this library support "sticky" items?
Yes, although it requires a small amount of user code. Here's a Code Sandbox demo.
License
MIT © bvaughn
Top Related Projects
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
React components for efficiently rendering large lists and tabular data
The most powerful virtual list component for React
🤖 Headless UI for Virtualizing Large Element Lists in JS/TS, React, Solid, Vue and Svelte
A tiny but mighty 3kb list virtualization library, with zero dependencies 💪 Supports variable heights/widths, sticky items, scrolling to index, and more!
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot