Top Related Projects
🚦 Microservices Status Page. Monitors a distributed infrastructure and sends alerts (Slack, SMS, etc.).
Apprise - Push Notifications that work with just about every platform!
Quick and Easy server testing/validation
Prometheus Alertmanager
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.
Create agents that monitor and act on your behalf. Your agents are standing by!
Quick Overview
Shoutrrr is a notification library for Go, providing a unified way to send notifications across various services. It supports multiple notification services like Slack, Discord, Telegram, and email, allowing developers to easily integrate notifications into their Go applications with a consistent API.
Pros
- Supports a wide range of notification services
- Easy to use with a simple, unified API
- Highly configurable with support for custom templates
- Actively maintained and regularly updated
Cons
- Limited to Go applications
- Some services may require additional setup or API keys
- Documentation could be more comprehensive for advanced use cases
- May introduce additional dependencies to your project
Code Examples
Sending a simple notification:
import "github.com/containrrr/shoutrrr"
url := "slack://token@channel"
err := shoutrrr.Send(url, "Hello, World!")
if err != nil {
log.Fatal(err)
}
Using multiple services:
urls := []string{
"slack://token@channel",
"telegram://token@telegram",
"discord://token@channel",
}
err := shoutrrr.SendAll(urls, "Notification sent to multiple services")
if err != nil {
log.Fatal(err)
}
Using a custom template:
sender, err := shoutrrr.CreateSender("slack://token@channel")
if err != nil {
log.Fatal(err)
}
params := &shoutrrr.Params{
"title": "Custom Title",
"message": "Custom message body",
}
err = sender.Send("{{ .title }}\n{{ .message }}", params)
if err != nil {
log.Fatal(err)
}
Getting Started
-
Install Shoutrrr:
go get github.com/containrrr/shoutrrr
-
Import the library in your Go code:
import "github.com/containrrr/shoutrrr"
-
Create a notification URL for your desired service:
url := "slack://token@channel"
-
Send a notification:
err := shoutrrr.Send(url, "Your notification message") if err != nil { log.Fatal(err) }
Competitor Comparisons
🚦 Microservices Status Page. Monitors a distributed infrastructure and sends alerts (Slack, SMS, etc.).
Pros of Vigil
- Comprehensive monitoring solution with built-in status page generation
- Supports both push and pull monitoring methods
- Offers a user-friendly Web UI for configuration and monitoring
Cons of Vigil
- More complex setup and configuration compared to Shoutrrr
- Limited notification options compared to Shoutrrr's extensive integrations
- Requires more system resources due to its full-featured nature
Code Comparison
Vigil configuration (TOML):
[[probe]]
id = "my-website"
label = "My Website"
description = "My company website"
Shoutrrr notification (Go):
url := "slack://token@channel"
err := shoutrrr.Send(url, "Hello World!")
Summary
Vigil is a comprehensive monitoring solution with a status page generator, while Shoutrrr focuses solely on notifications. Vigil offers more features but requires more setup, whereas Shoutrrr is simpler to use but limited to notifications. Choose Vigil for a complete monitoring system or Shoutrrr for easy integration of notifications into existing projects.
Apprise - Push Notifications that work with just about every platform!
Pros of Apprise
- Supports a wider range of notification services (80+)
- Provides a command-line interface for easy integration
- Offers a web-based API for remote notifications
Cons of Apprise
- Written in Python, which may not be ideal for all environments
- Lacks built-in rate limiting features
- May have a steeper learning curve for complex configurations
Code Comparison
Apprise:
import apprise
apobj = apprise.Apprise()
apobj.add('mailto://user:pass@gmail.com')
apobj.notify('Title', 'Body of message')
Shoutrrr:
package main
import "github.com/containrrr/shoutrrr"
url := "smtp://username:password@host:port/?fromAddress=sender@example.com&toAddresses=recipient@example.com"
shoutrrr.Send(url, "Title", "Body of message")
Both libraries aim to simplify sending notifications across various services. Apprise offers more flexibility with its extensive service support and CLI, while Shoutrrr provides a more lightweight Go-based solution with built-in rate limiting. The choice between them may depend on your preferred programming language, required notification services, and specific use case requirements.
Quick and Easy server testing/validation
Pros of goss
- Focused on system and service testing, providing a YAML-based DSL for defining tests
- Supports multiple output formats (JSON, TAP, JUnit) for easy integration with CI/CD pipelines
- Lightweight and fast execution, suitable for both development and production environments
Cons of goss
- Limited to system and service testing, not designed for general-purpose notifications
- Requires installation on the target system, which may not be feasible in all scenarios
- Learning curve for writing YAML-based test definitions, especially for complex scenarios
Code Comparison
goss (YAML test definition):
file:
/etc/passwd:
exists: true
mode: "0644"
owner: root
group: root
shoutrrr (Go code for sending notifications):
url := "slack://token@channel"
err := shoutrrr.Send(url, "Hello World!")
if err != nil {
log.Fatal(err)
}
While goss focuses on system testing with YAML definitions, shoutrrr provides a simple API for sending notifications across various services. The projects serve different purposes, with goss being more specialized for system validation and shoutrrr offering a flexible notification solution.
Prometheus Alertmanager
Pros of Alertmanager
- Deeply integrated with Prometheus ecosystem
- Supports advanced alert routing and grouping
- Provides a web UI for managing alerts
Cons of Alertmanager
- Steeper learning curve for configuration
- Primarily designed for Prometheus, less flexible for other use cases
Code Comparison
Alertmanager configuration (YAML):
route:
receiver: 'team-X-mails'
receivers:
- name: 'team-X-mails'
email_configs:
- to: 'team-X+alerts@example.org'
Shoutrrr usage (Go):
url := "smtp://user:pass@host:port/?to=recipient@example.com"
shoutrrr.Send(url, "Alert message")
Key Differences
- Alertmanager is a full-featured alert management system, while Shoutrrr is a simpler notification library
- Shoutrrr supports a wider range of notification services out-of-the-box
- Alertmanager offers more advanced alert handling and deduplication features
- Shoutrrr is easier to integrate into existing applications as a library
Use Cases
- Alertmanager: Best for large-scale monitoring systems, especially those using Prometheus
- Shoutrrr: Ideal for adding notification capabilities to existing applications or smaller projects
Both tools have their strengths, and the choice depends on the specific requirements of your project and infrastructure.
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.
Pros of Grafana
- Comprehensive data visualization and analytics platform
- Extensive plugin ecosystem and integrations
- Robust alerting and notification system
Cons of Grafana
- Steeper learning curve due to its complexity
- Requires more resources to run and maintain
Code Comparison
Grafana (dashboard configuration):
{
"panels": [
{
"type": "graph",
"title": "CPU Usage",
"datasource": "Prometheus",
"targets": [
{ "expr": "node_cpu_usage_percent" }
]
}
]
}
Shoutrrr (sending a notification):
url := "telegram://token@telegram?channels=channel-1,channel-2"
err := shoutrrr.Send(url, "Hello, World!")
if err != nil {
log.Fatal(err)
}
Summary
Grafana is a powerful data visualization and monitoring platform, offering extensive features and integrations. It's ideal for complex monitoring setups but may be overkill for simple notification needs. Shoutrrr, on the other hand, is a lightweight notification library focused solely on sending alerts through various services. It's simpler to use and integrate but lacks the comprehensive monitoring and visualization capabilities of Grafana.
Create agents that monitor and act on your behalf. Your agents are standing by!
Pros of Huginn
- More comprehensive automation platform with a wide range of agents for various tasks
- Web-based interface for easy management and visualization of workflows
- Supports complex scenarios and multi-step automations
Cons of Huginn
- Steeper learning curve due to its complexity and extensive features
- Requires more system resources and setup compared to lightweight alternatives
- Less focused on notifications, as it's a broader automation tool
Code Comparison
Huginn (Ruby):
class Agents::WebhookAgent < Agent
def receive(incoming_events)
incoming_events.each do |event|
handle(event)
end
end
end
Shoutrrr (Go):
func main() {
url := "slack://token@channel"
err := shoutrrr.Send(url, "Hello World!")
if err != nil {
log.Fatal(err)
}
}
Summary
Huginn is a powerful, all-in-one automation platform with a web interface, suitable for complex workflows. Shoutrrr, on the other hand, is a lightweight library focused specifically on sending notifications across various services. Huginn offers more flexibility but requires more resources and setup, while Shoutrrr is simpler to use for basic notification needs.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
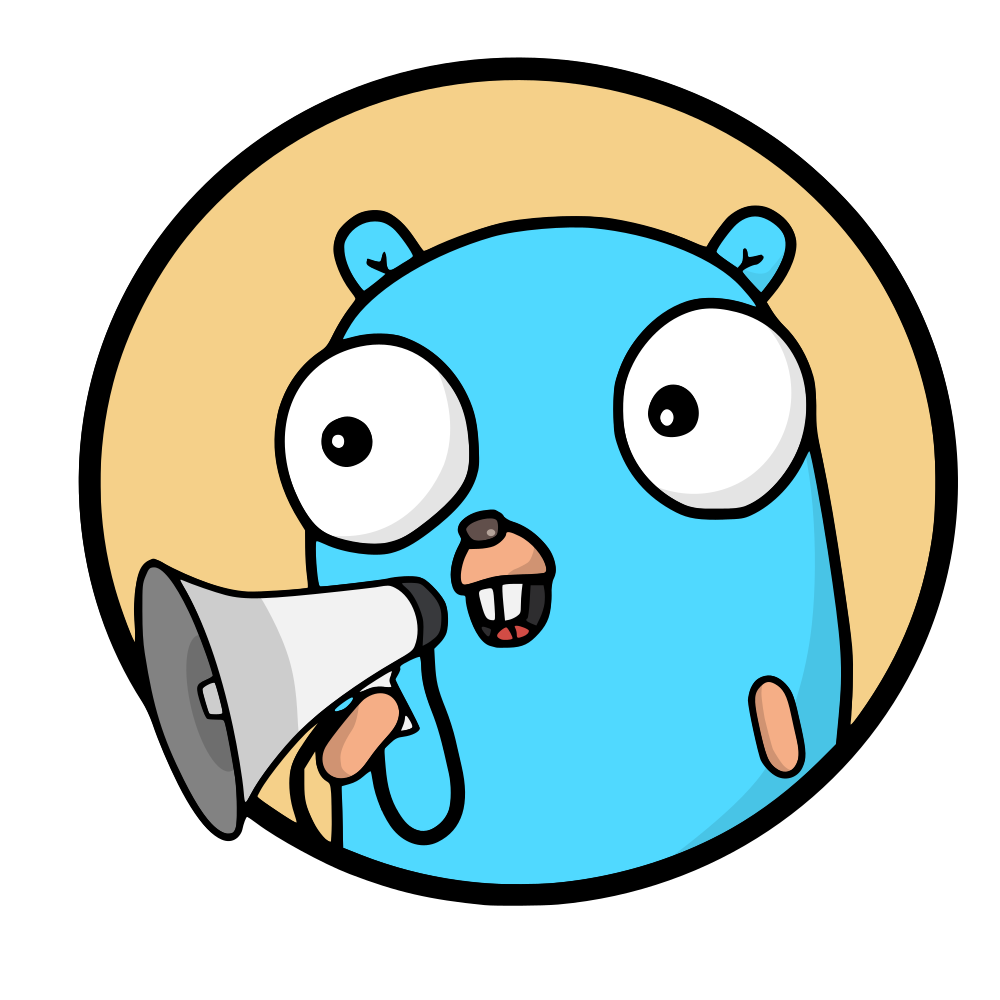
Shoutrrr
Notification library for gophers and their furry friends. Heavily inspired by caronc/apprise.
Installation
Using the snap
$ sudo snap install shoutrrr
Using the Go CLI
$ go install github.com/containrrr/shoutrrr/shoutrrr@latest
From Source
$ go build -o shoutrrr ./shoutrrr
Quick Start
As a package
Using shoutrrr is easy! There is currently two ways of using it as a package.
Using the direct send command
url := "slack://token-a/token-b/token-c"
err := shoutrrr.Send(url, "Hello world (or slack channel) !")
Using a sender
url := "slack://token-a/token-b/token-c"
sender, err := shoutrrr.CreateSender(url)
sender.Send("Hello world (or slack channel) !", map[string]string { /* ... */ })
Using a sender with multiple URLs
urls := []string {
"slack://token-a/token-b/token-c"
"discord://token@channel"
}
sender, err := shoutrrr.CreateSender(urls...)
sender.Send("Hello world (or slack channel) !", map[string]string { /* ... */ })
Through the CLI
Start by running the build.sh
script.
You may then run send notifications using the shoutrrr executable:
$ shoutrrr send [OPTIONS] <URL> <Message [...]>
From a GitHub Actions workflow
You can also use Shoutrrr from a GitHub Actions workflow.
See this example and the action on GitHub Marketplace:
name: Deploy
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Some other steps needed for deploying
run: ...
- name: Shoutrrr
uses: containrrr/shoutrrr-action@v1
with:
url: ${{ secrets.SHOUTRRR_URL }}
title: Deployed ${{ github.sha }}
message: See changes at ${{ github.event.compare }}.
Documentation
For additional details, visit the full documentation.
Contributors â¨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
Related Project(s)
- watchtower - process for automating Docker container base image updates that uses shoutrrr for notifications
- kured - kubernetes reboot daemon has adopted shoutrrr as their unified notification method starting with version 1.7.0.
- Green Orb - a versatile 'observe and report' buddy for your application logs
Top Related Projects
🚦 Microservices Status Page. Monitors a distributed infrastructure and sends alerts (Slack, SMS, etc.).
Apprise - Push Notifications that work with just about every platform!
Quick and Easy server testing/validation
Prometheus Alertmanager
The open and composable observability and data visualization platform. Visualize metrics, logs, and traces from multiple sources like Prometheus, Loki, Elasticsearch, InfluxDB, Postgres and many more.
Create agents that monitor and act on your behalf. Your agents are standing by!
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot