Top Related Projects
Transforming styles with JS plugins
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
👩🎤 CSS-in-JS library designed for high performance style composition
A utility-first CSS framework for rapid UI development.
Sass makes CSS fun!
JSS is an authoring tool for CSS which uses JavaScript as a host language.
Quick Overview
CSS Modules is a methodology for writing modular and reusable CSS in modern web applications. It allows developers to write CSS that is scoped locally by default, avoiding global namespace conflicts and making styles more maintainable. CSS Modules works by automatically generating unique class names, ensuring that styles don't leak or clash between components.
Pros
- Eliminates global scope issues and naming conflicts in CSS
- Improves code maintainability and reusability
- Enables better collaboration in large projects
- Provides a simple and intuitive API for working with styles in JavaScript
Cons
- Requires a build step, which may increase complexity in project setup
- Can be challenging to integrate with existing CSS methodologies or legacy codebases
- May have a learning curve for developers used to traditional CSS approaches
- Limited browser support without proper tooling
Code Examples
- Basic usage of CSS Modules:
/* Button.module.css */
.button {
background-color: blue;
color: white;
padding: 10px 20px;
}
// Button.js
import React from 'react';
import styles from './Button.module.css';
const Button = ({ children }) => (
<button className={styles.button}>{children}</button>
);
export default Button;
- Composing multiple classes:
/* styles.module.css */
.base {
font-size: 16px;
}
.primary {
composes: base;
color: blue;
}
.secondary {
composes: base;
color: green;
}
- Using global styles within CSS Modules:
/* Header.module.css */
:global(.header) {
background-color: #f0f0f0;
}
.title {
font-size: 24px;
}
Getting Started
To use CSS Modules in a React project:
-
Install necessary dependencies:
npm install css-loader style-loader
-
Configure your webpack.config.js:
module.exports = { module: { rules: [ { test: /\.css$/, use: [ 'style-loader', { loader: 'css-loader', options: { modules: true, }, }, ], }, ], }, };
-
Create a CSS file with a .module.css extension and import it in your component:
import styles from './MyComponent.module.css';
-
Use the imported styles in your component:
<div className={styles.myClass}>Hello, CSS Modules!</div>
Competitor Comparisons
Transforming styles with JS plugins
Pros of PostCSS
- More flexible and extensible with a plugin ecosystem
- Supports a wider range of CSS transformations and optimizations
- Can be used alongside other CSS preprocessors
Cons of PostCSS
- Steeper learning curve due to its plugin-based architecture
- Requires more configuration and setup compared to CSS Modules
Code Comparison
CSS Modules:
.button {
color: red;
}
.button:hover {
color: blue;
}
PostCSS (with plugins):
@custom-media --mobile (width <= 640px);
.button {
color: red;
@media (--mobile) {
font-size: 14px;
}
&:hover {
color: blue;
}
}
PostCSS offers more advanced features like custom media queries and nesting, while CSS Modules focuses on scoping and modularization of styles. PostCSS's flexibility allows for a wider range of transformations, but CSS Modules provides a simpler, more focused approach to solving CSS conflicts in component-based architectures.
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
Pros of styled-components
- Allows for dynamic styling based on props and theme
- Eliminates the need for separate CSS files, keeping styles and components together
- Provides automatic vendor prefixing and unique class names
Cons of styled-components
- Larger bundle size due to runtime CSS generation
- Steeper learning curve for developers unfamiliar with CSS-in-JS
- Potential performance issues with complex styling logic
Code Comparison
styled-components:
const Button = styled.button`
background-color: ${props => props.primary ? 'blue' : 'white'};
color: ${props => props.primary ? 'white' : 'blue'};
padding: 10px 20px;
`;
CSS Modules:
.button {
padding: 10px 20px;
}
.primary {
background-color: blue;
color: white;
}
Summary
styled-components offers a more dynamic and integrated approach to styling React components, while CSS Modules provides a more traditional CSS experience with local scoping. The choice between the two depends on project requirements, team preferences, and performance considerations.
👩🎤 CSS-in-JS library designed for high performance style composition
Pros of emotion
- Runtime CSS-in-JS with dynamic styling capabilities
- Built-in theming support and server-side rendering
- Smaller bundle size and better performance compared to other CSS-in-JS libraries
Cons of emotion
- Steeper learning curve for developers used to traditional CSS
- Potential runtime performance overhead for complex styles
- Lack of static analysis and type checking for styles
Code Comparison
css-modules:
.button {
background-color: blue;
color: white;
}
import styles from './Button.css';
const Button = () => <button className={styles.button}>Click me</button>;
emotion:
import { css } from '@emotion/react';
const buttonStyle = css`
background-color: blue;
color: white;
`;
const Button = () => <button css={buttonStyle}>Click me</button>;
CSS Modules focuses on locally scoped CSS classes, while emotion allows for dynamic, JavaScript-powered styling. CSS Modules provides better separation of concerns, but emotion offers more flexibility and runtime styling capabilities. The choice between the two depends on project requirements and team preferences.
A utility-first CSS framework for rapid UI development.
Pros of Tailwind CSS
- Rapid development with utility-first approach
- Highly customizable with a comprehensive configuration system
- Smaller file sizes due to purging unused styles in production
Cons of Tailwind CSS
- Steeper learning curve for developers new to utility-first CSS
- Can lead to cluttered HTML with many class names
- Requires additional tooling for optimal performance
Code Comparison
CSS Modules:
.button {
background-color: blue;
color: white;
padding: 0.5rem 1rem;
}
Tailwind CSS:
<button class="bg-blue-500 text-white py-2 px-4">
Click me
</button>
CSS Modules uses traditional CSS classes, while Tailwind CSS employs utility classes directly in HTML. CSS Modules offers better separation of concerns, whereas Tailwind CSS provides faster development and more flexibility at the cost of potentially cluttered markup.
Both approaches have their merits, and the choice between them often depends on project requirements, team preferences, and development workflow. CSS Modules excels in maintaining clean HTML and modular styles, while Tailwind CSS shines in rapid prototyping and consistent design implementation across large projects.
Sass makes CSS fun!
Pros of Sass
- Offers more advanced features like mixins, functions, and control directives
- Provides a more robust and mature ecosystem with extensive documentation
- Allows for nested selectors, making code organization easier
Cons of Sass
- Requires compilation, which can add complexity to the build process
- Has a steeper learning curve due to its additional syntax and features
- May lead to overly complex stylesheets if not used carefully
Code Comparison
Sass:
$primary-color: #3498db;
@mixin button-styles {
padding: 10px 15px;
border-radius: 5px;
}
.button {
@include button-styles;
background-color: $primary-color;
}
CSS Modules:
.button {
padding: 10px 15px;
border-radius: 5px;
background-color: #3498db;
}
CSS Modules focuses on scoping CSS classes to specific components, while Sass provides a more feature-rich preprocessor for writing CSS. Sass offers variables, mixins, and nesting, which can lead to more maintainable stylesheets. However, CSS Modules excels in preventing class name conflicts and promoting modular CSS architecture without introducing new syntax.
JSS is an authoring tool for CSS which uses JavaScript as a host language.
Pros of JSS
- Runtime styling allows for dynamic, theme-based styling
- Better performance for large-scale applications due to optimized style injection
- Supports plugins for extended functionality and customization
Cons of JSS
- Steeper learning curve compared to traditional CSS
- Requires additional setup and configuration
- May lead to larger bundle sizes in some cases
Code Comparison
CSS Modules:
.button {
background-color: blue;
color: white;
}
JSS:
const styles = {
button: {
backgroundColor: 'blue',
color: 'white',
},
};
CSS Modules uses traditional CSS syntax with local scoping, while JSS defines styles as JavaScript objects. JSS allows for more dynamic styling capabilities, but CSS Modules maintains a familiar CSS-like structure.
Both approaches aim to solve the problem of CSS scoping and modularity, but they do so in different ways. CSS Modules focuses on locally scoped CSS classes, while JSS takes a JavaScript-first approach to styling.
CSS Modules is generally easier to adopt for teams familiar with traditional CSS, while JSS offers more flexibility and programmatic control over styles. The choice between the two often depends on project requirements, team preferences, and the overall architecture of the application.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
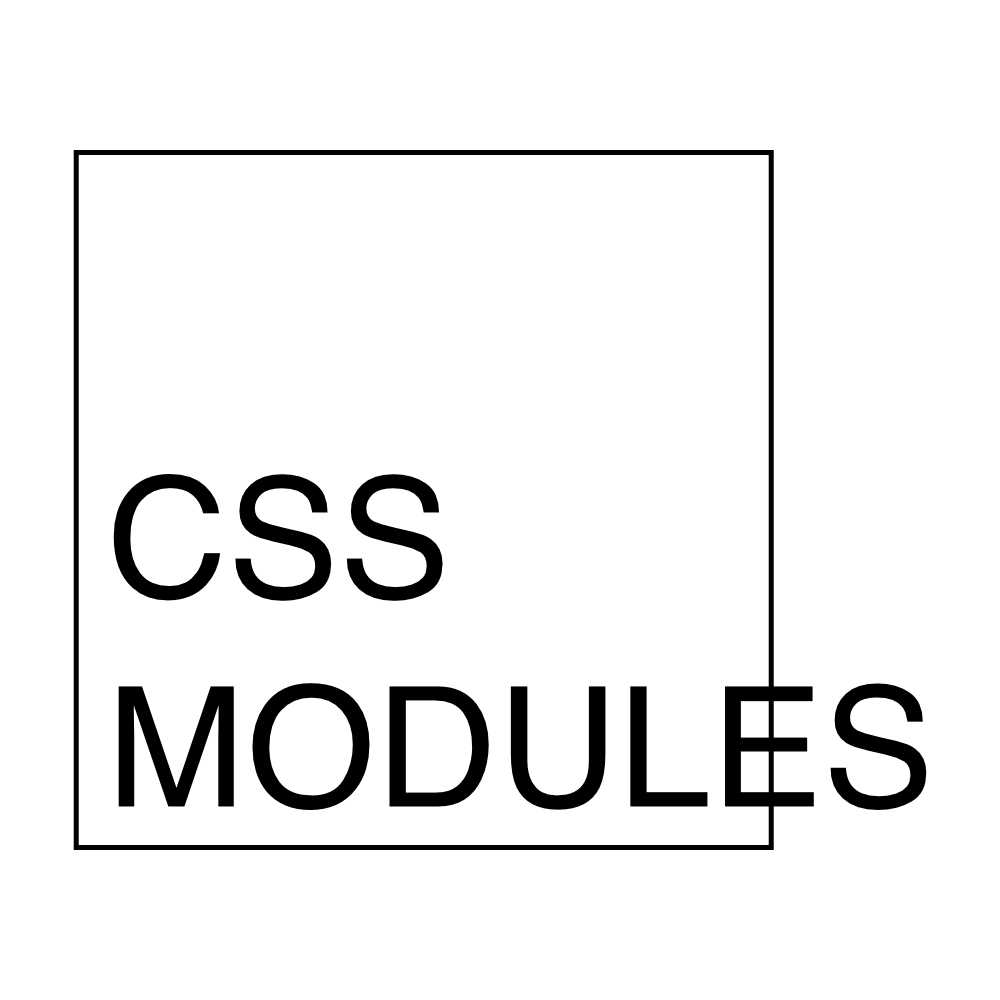
CSS Modules
A CSS Module is a CSS file where all class names and animation names are scoped locally by default. All URLs (url(...)
) and @imports
are in module request format (./xxx
and ../xxx
means relative, xxx
and xxx/yyy
means in modules folder, i.e. in node_modules
).
CSS Modules compile to a low-level interchange format called ICSS (or Interoperable CSS) but are written like normal CSS files:
/* style.css */
.className {
color: green;
}
When importing a CSS Module from a JavaScript Module, it exports an object with all mappings from local names to global names.
import styles from './style.css';
element.innerHTML = '<div class="' + styles.className + '">';
Table of Contents
Why CSS Modules?
- Local Scope Prevents Clashes: CSS Modules use local scope to avoid style conflicts across different project parts, allowing component-scoped styling.
- Clear Style Dependencies: Importing styles into their respective components clarifies which styles impact which areas, enhancing code readability and maintenance.
- Solves Global Scope Problems: CSS Modules prevent the common issue of styles in one file affecting the entire project by localizing styles to specific components.
- Boosts Reusability and Modularity: CSS Modules allow the same class names in different modules, promoting modular, reusable styling.
Top Related Projects
Transforming styles with JS plugins
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
👩🎤 CSS-in-JS library designed for high performance style composition
A utility-first CSS framework for rapid UI development.
Sass makes CSS fun!
JSS is an authoring tool for CSS which uses JavaScript as a host language.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot