Top Related Projects
🐰 Rax is a progressive framework for building universal application. https://rax.js.org
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Quasar Framework - Build high-performance VueJS user interfaces in record time
A framework for building native applications using React
Quick Overview
uni-app is a cross-platform framework for developing mobile and web applications using Vue.js. It allows developers to write once and deploy to multiple platforms, including iOS, Android, H5, and various mini-program ecosystems like WeChat and Alipay.
Pros
- Cross-platform development with a single codebase
- Large ecosystem with extensive plugin support
- Seamless integration with Vue.js and its ecosystem
- High performance with native rendering capabilities
Cons
- Learning curve for developers new to Vue.js or cross-platform development
- Limited customization options for platform-specific features
- Dependency on the uni-app ecosystem for certain functionalities
- Potential performance overhead compared to native development
Code Examples
- Creating a basic page:
<template>
<view class="content">
<text>Hello uni-app!</text>
</view>
</template>
<script>
export default {
data() {
return {}
},
methods: {}
}
</script>
<style>
.content {
display: flex;
justify-content: center;
align-items: center;
}
</style>
- Making an API request:
uni.request({
url: 'https://api.example.com/data',
method: 'GET',
success: (res) => {
console.log(res.data);
},
fail: (err) => {
console.error(err);
}
});
- Navigation between pages:
// Navigate to a new page
uni.navigateTo({
url: '/pages/detail/detail?id=1'
});
// Go back to the previous page
uni.navigateBack();
Getting Started
-
Install Vue CLI and the uni-app plugin:
npm install -g @vue/cli vue add @dcloudio/vue-cli-plugin-uni
-
Create a new uni-app project:
vue create -p dcloudio/uni-preset-vue my-project
-
Run the project:
cd my-project npm run dev:h5
This will start a development server for the H5 version of your app. You can use different commands to run on other platforms, such as npm run dev:mp-weixin
for WeChat mini-program.
Competitor Comparisons
🐰 Rax is a progressive framework for building universal application. https://rax.js.org
Pros of Rax
- Lightweight and performant, optimized for mobile development
- Supports multiple rendering targets (Web, Weex, Mini-programs)
- Backed by Alibaba, with strong community support and ecosystem
Cons of Rax
- Steeper learning curve compared to Uni-app
- Less comprehensive documentation and tutorials
- Smaller plugin ecosystem than Uni-app
Code Comparison
Rax component:
import { createElement, render } from 'rax';
import View from 'rax-view';
function App() {
return <View>Hello, Rax!</View>;
}
render(<App />);
Uni-app component:
<template>
<view>Hello, Uni-app!</view>
</template>
<script>
export default {
name: 'App'
}
</script>
Key Differences
- Rax uses a React-like syntax, while Uni-app uses Vue-style components
- Rax focuses on performance and flexibility, Uni-app emphasizes ease of use and cross-platform compatibility
- Rax has a more modular approach, allowing developers to choose specific packages, while Uni-app provides a more integrated solution
Use Cases
- Rax: Ideal for complex, high-performance mobile applications, especially within the Alibaba ecosystem
- Uni-app: Better suited for rapid development of cross-platform apps with a gentler learning curve
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
Pros of Taro
- Supports more frameworks (React, Vue, Nerv) compared to uni-app's Vue-only approach
- Better TypeScript support and type checking
- More active community and frequent updates
Cons of Taro
- Steeper learning curve, especially for developers new to React
- Less comprehensive documentation compared to uni-app
- May have compatibility issues with some native APIs
Code Comparison
Taro (React):
import { Component } from 'react'
import { View, Text } from '@tarojs/components'
export default class Index extends Component {
render() {
return (
<View className='index'>
<Text>Hello, World!</Text>
</View>
)
}
}
uni-app (Vue):
<template>
<view class="content">
<text>Hello, World!</text>
</view>
</template>
<script>
export default {
name: 'App'
}
</script>
Both frameworks aim to create cross-platform applications, but Taro offers more flexibility in terms of framework choice and has better TypeScript integration. uni-app, on the other hand, provides a more straightforward development experience with its Vue-based approach and comprehensive documentation. The choice between the two depends on the developer's familiarity with React or Vue, and the specific requirements of the project.
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Pros of Ionic Framework
- Larger community and ecosystem, with more third-party plugins and resources
- Better documentation and learning resources
- More mature and established framework with a longer track record
Cons of Ionic Framework
- Steeper learning curve, especially for developers new to Angular or React
- Larger bundle sizes, which can impact app performance
- Less native-like look and feel compared to Uni-app's closer adherence to platform-specific UI
Code Comparison
Ionic Framework (Angular):
import { Component } from '@angular/core';
@Component({
selector: 'app-home',
template: '<ion-content><h1>Hello Ionic!</h1></ion-content>'
})
export class HomePage {}
Uni-app:
<template>
<view>
<text>Hello Uni-app!</text>
</view>
</template>
<script>
export default {
// Component logic here
}
</script>
Both frameworks aim to simplify cross-platform app development, but they take different approaches. Ionic Framework offers a more web-centric development experience with a focus on Angular or React, while Uni-app provides a Vue-like syntax and aims for a more native feel. The choice between them depends on the developer's background, project requirements, and target platforms.
Quasar Framework - Build high-performance VueJS user interfaces in record time
Pros of Quasar
- More comprehensive ecosystem with built-in components and tools
- Better documentation and community support
- Supports both Vue 2 and Vue 3
Cons of Quasar
- Steeper learning curve due to its extensive feature set
- Larger bundle size, which may impact performance on low-end devices
Code Comparison
uni-app:
<template>
<view class="content">
<text>{{ title }}</text>
</view>
</template>
<script>
export default {
data() {
return {
title: 'Hello'
}
}
}
</script>
Quasar:
<template>
<q-page class="flex flex-center">
<q-btn color="primary" label="Hello" />
</q-page>
</template>
<script>
export default {
name: 'PageIndex'
}
</script>
Key Differences
- uni-app uses a custom
<view>
component, while Quasar uses standard HTML elements with its own components like<q-page>
and<q-btn>
- Quasar provides built-in styling and theming options, evident in the
color="primary"
attribute - uni-app's syntax is closer to standard Vue, making it easier for Vue developers to adopt
Both frameworks aim to simplify cross-platform development, but Quasar offers a more feature-rich environment at the cost of complexity, while uni-app focuses on ease of use and familiarity for Vue developers.
A framework for building native applications using React
Pros of React Native
- Larger community and ecosystem, with more third-party libraries and resources
- Better performance for complex applications due to native rendering
- Stronger corporate backing from Facebook, ensuring long-term support
Cons of React Native
- Steeper learning curve, especially for developers new to React
- More complex setup and configuration process
- Less consistent cross-platform UI, requiring platform-specific adjustments
Code Comparison
React Native:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => (
<View style={styles.container}>
<Text>Hello, React Native!</Text>
</View>
);
uni-app:
<template>
<view class="container">
<text>Hello, uni-app!</text>
</view>
</template>
<style>
.container { /* styles */ }
</style>
Key Differences
- React Native uses JSX syntax, while uni-app uses Vue-like template syntax
- React Native requires separate styling with StyleSheet, uni-app uses Vue-style CSS
- uni-app offers a more unified development experience across platforms
- React Native provides more granular control over native components
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
uni-app
ç®ä½ä¸æ | English
uni-app
æ¯ä¸ä¸ªä½¿ç¨ Vue.js
å¼åå°ç¨åºãH5ãAppçç»ä¸åç«¯æ¡æ¶ãå®ç½å°åï¼https://uniapp.dcloud.io
å¼åè
ä½¿ç¨ Vue
è¯æ³ç¼å代ç ï¼uni-app
æ¡æ¶å°å
¶ç¼è¯å° å°ç¨åºï¼å¾®ä¿¡/æ¯ä»å®/ç¾åº¦/åèè·³å¨/QQ/å¿«æ/éé/å°çº¢ä¹¦ï¼ãAppï¼iOS/Androidï¼ãH5çå¤ä¸ªå¹³å°ï¼ä¿è¯å
¶æ£ç¡®è¿è¡å¹¶è¾¾å°ä¼ç§ä½éªã
uni-appçç¹ç¹
- å¼åè 忡便´å¤ï¼HBuilderè£ æºé800ä¸å°ï¼å¼åè ç¤¾åºææ´»ç¾ä¸ï¼70å¤ä¸ªQQ微信群æ¿è½½10ä¸äººãæ¡ä¾ä¼å¤ï¼uniç»è®¡ææ´»è¶ 10亿ï¼è¯¦è§ï¼
- æ§è½æ´é«ï¼è§è¯æµï¼
- æ´ä¸°å¯çå¨è¾¹çæï¼æä»¶å¸åºæ°å款æä»¶
- æä¾æ¯å°ç¨åºåçå¼åæ´å¥½çå¼åä½éªãæ´é«çå·¥ç¨åæç
- 跨端æ¹å¹³åº¦æ´å®åï¼ä¸å端ç¹è²åæ¥æ´çµæ´»ï¼å¯çæ£å®ç°ä¸å¥ä»£ç å¤ç«¯è¦çï¼æ éå端å¤å¤´ç»´æ¤å级
- æå¨è®¤å¯ï¼é¿éå°ç¨åºå®æ¹å·¥å ·å ç½®uni-appï¼è¯¦è§ï¼ãè ¾è®¯è¯¾å 宿¹èªå¶uni-appå¹è®è§é¢ï¼è¯¦è§ï¼
æ«ç ä½éª
å¼å䏿¬¡ï¼ç¼è¯å°14个平å°ã便¬¡æ«æ14个äºç»´ç ï¼äº²èªä½éªæå ¨é¢çè·¨å¹³å°ææï¼
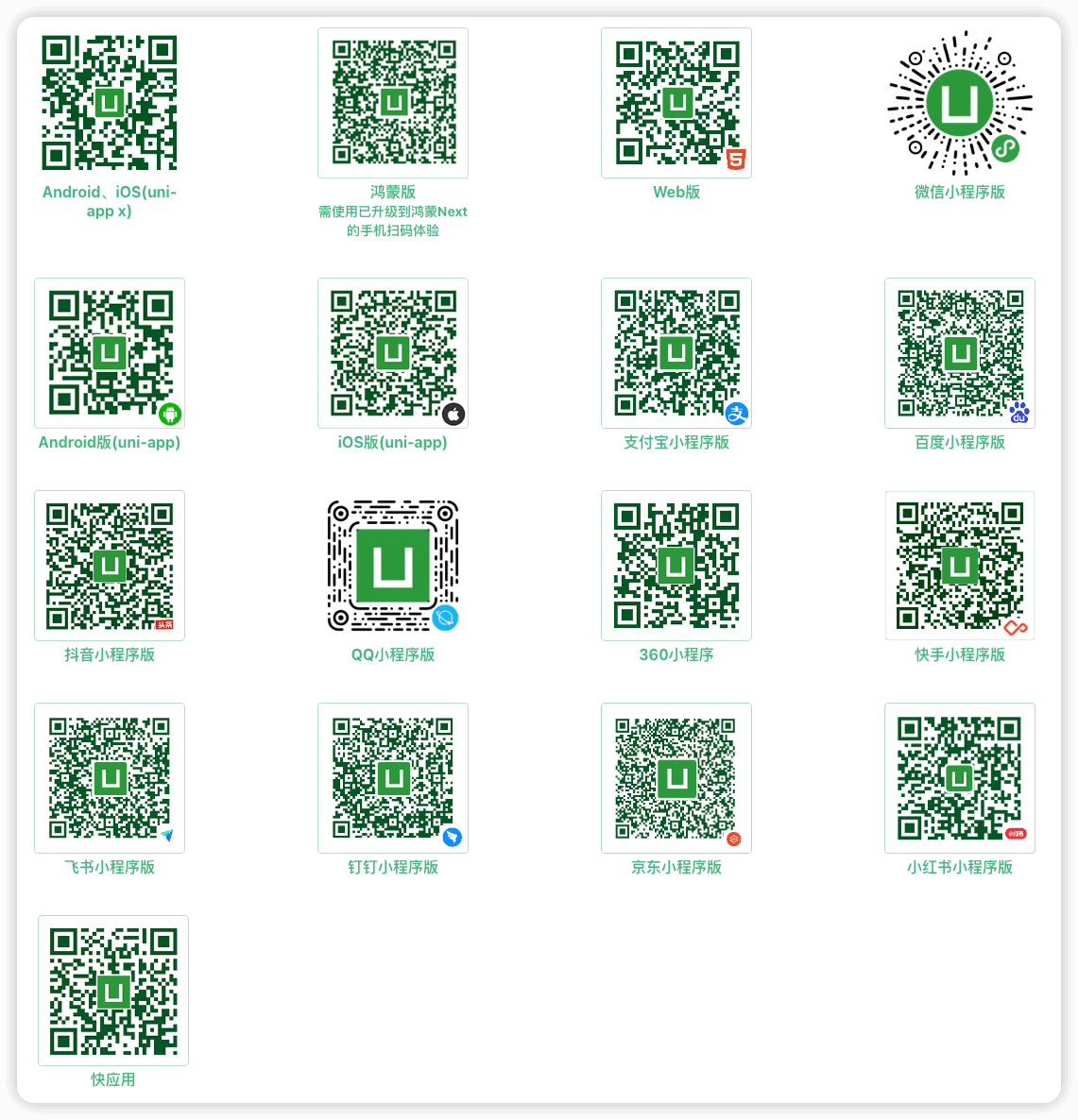
æ³¨ï¼ æäºå¹³å°ä¸è½æäº¤ç®ådemoï¼è¡¥å äºä¸äºå ¶ä»åè½ã
å¿«éå¼å§
uni-app
æ¯æéè¿vue-cli
å½ä»¤è¡ãHBuilderX
å¯è§åçé¢ä¸¤ç§æ¹å¼å¿«éå建项ç®ï¼
- vue-cliå½ä»¤è¡æ¹å¼ï¼ä¸éIDEï¼éå对nodeçæçå¼åè ï¼æ©å±é 读ï¼å¨vscodeä¸å¼åuni-appãå¨ WebStorm ä¸å¼å uni-app
- HBuilderXå¯è§åçé¢ï¼ä¸ç¨IDEï¼å ç½®ç¸å ³ç¯å¢ï¼å¼ç®±å³ç¨ï¼å¼åæçæ´é«ã
é¡¹ç®æ¡ä¾
æ¡ä¾å±ç¤ºï¼uniapp.dcloud.io/case
æ¬¢è¿æäº¤ä½ çåºç¨ï¼uni-appæ¡ä¾å¾é
éæ±å¢
uni-app
è®¡åæ¯æçåè½ç¹ï¼ä¼å¨éæ±å¢ä¸è¿è¡å±ç¤ºï¼å¾éå¼åè
çæç¥¨æè§ï¼åå¾æç¥¨ã
æ´æ°æ¥å¿
uni-app
ä¸ç´ä¿æé«é¢çæ´æ°è¿ä»£ï¼è¯¦è§æ£å¼çæ´æ°æ¥å¿ãAlphaçæ´æ°æ¥å¿ã
论å
ç±äºDCloud
æ70å¤ä¸ªQQã微信群ï¼å®æ¹å·²æ æ³ç»´æ¤æ´å¤äº¤æµç¾¤ã请å¼åè
å°å®æ¹è®ºå交æµï¼https://ask.dcloud.net.cn/explore/ ã论åæä¾äºæ¯issuesæ´ä¸ä¸çå·¥å
·æå¡ã
æä»¶å¸åº
uni-app
æä¸°å¯çæä»¶çæï¼ä¼å¤å¼åè
æäº¤äºæ°å款ç»ä»¶ãsdkãé¡¹ç®æ¨¡æ¿ï¼è¯¦è§ï¼https://ext.dcloud.net.cn/
é¤äºä¼å¤ä¸æ¹uiåºï¼å®æ¹è¿æä¾äºuni-uiï¼å¨æ§è½åè·¨ç«¯å ¼å®¹æ¹é¢ææ´å¼ºçä¼å¿ã详è§ï¼https://ext.dcloud.net.cn/plugin?id=55
ç°æé¡¹ç®å¦ä½è¿ç§»å°uni-appä½ç³»
- 微信å°ç¨åºè½¬æ¢uni-appæåå转æ¢å¨ï¼https://ask.dcloud.net.cn/article/35786
- vue h5项ç®è½¬æ¢uni-appæåï¼https://ask.dcloud.net.cn/article/36174
- mpvue 项ç®ï¼ç»ä»¶ï¼è¿ç§»æåã示ä¾åèµæºæ±æ»ï¼ https://ask.dcloud.net.cn/article/34945
- wepy转uni-app转æ¢å¨ï¼https://github.com/zhangdaren/wepy-to-uniapp
常è§çé®
-
é®ï¼ä¸å端æä¸åçéæ±ãä¸åçç¹è²ï¼ç»å½æ¯ä»ä¹ä¸ä¸æ ·ï¼å¦ä½ç»ä¸ï¼
-
çï¼å·®å¼é¨åä½¿ç¨æ¡ä»¶ç¼è¯ãuni-appæä¾äºçµæ´»å¼ºå¤§çæ¡ä»¶ç¼è¯ãå¯ä»¥å®ç¾å¤çå¤ç¨é¨ååå·®å¼é¨åãçæ£ä¸å¥å·¥ç¨æºç ãå½ä¸å¡å级æ¶ï¼ä¸åéè¦å¤ç«¯ç»´æ¤ã妿å¤ç«¯ç»´æ¤ï¼ç»å¸¸ä¼å 为æäºç«¯çæµéä¸å¤§ï¼å°±ä¸ç´æå»¶æ æ³è®©é£äºç¨æ·äº«åå°ææ°æå¡ãå¦å¤ç»å½æ¯ä»å¨å®¢æ·ç«¯é¨åï¼å·²ç»è¢«uni-appç»ä¸æä¸æ ·çapiäºã
-
é®ï¼å¤ç«¯æ¯ä¸æ¯ä¸ç§å¦¥åï¼æ¯å¦ä¼é ææ§è½ä¸éï¼
-
çï¼good questionãå¤ç«¯ä¸ä¸å½±åæ§è½ï¼ç¡®å®å¾é¾ï¼ä½uni-appåå°äºãå¨h5端ï¼å®çæ§è½ãå ä½ç§¯ä¸ç´æ¥ä½¿ç¨vue.jså¼åä¸è´ï¼ å¨å°ç¨åºç«¯ï¼å®çæ§è½æ¯å¤§å¤æ°å¼åæ¡æ¶æ´å¥½ï¼uni-appåºå±èªå¨å¤ççsetdataå·®é忥æºå¶ï¼æ¯å¼åè æå¨åsetdataæ´å¥½ï¼å°±å使ç¨vue.jsæ´æ°ç颿¯æå¨åjsä¿®æ¹domæ´é«æä¸æ ·ï¼ å¨Appï¼uni-appæ¯æwebview渲æååçæ¸²æå弿ï¼å¯ç¨åçæ¸²ææ¶ï¼cssåæ³åéï¼ä½æ§è½æ¯å¾æ¥è¿åçå¼åçææçï¼å¨å½åçææºç¯å¢ä¸ï¼å䏿¥æ´»ä»¥ä¸çåºç¨å¨App使ç¨uni-appä¹ä¸ä¼éå°ä»»ä½ååãå½ç¶ä¹å¯ä»¥å¨å·²ç»å好çåçAppä¸å°é¨å页颿¹ä¸ºuni-appå®ç°; æ¤å¤ï¼æä»¬ä¼æå¾å¤è·¨ç«¯å¤çæ¾å¨ç¼è¯æå®æçï¼è¿æ ·ä¼åå°å¯¹è¿è¡æçæçå½±åã
-
é®ï¼ä¸åå¤ç«¯ï¼æ¯ä¸æ¯ä¸éè¦uni-appï¼
-
çï¼ä¸æ¯ã大éå¼åè ç¨uni-appåªåä¸ä¸ªç«¯ï¼è¯¦è§æ¡ä¾ã对äºå¼åè èè¨ï¼ä¸ä¸ªä¼ç§å·¥å ·å¨æï¼åä»ä¹é½ä¸æã
-
é®ï¼uni-app以åä¼ä¸ä¼åæ´å¼æºåè®®ï¼è½¬åæ¶è´¹ï¼
-
çï¼å®æ¹æ¿è¯ºæ°¸è¿ä¸ä¼åæ´å¼æºåè®®ãæ è®ºHBuilderXãuni-appãAppï¼é¢åä¸å½äººæ°¸ä¹ å è´¹ã
æ´å¤èµæ
- è¯æµï¼Appè·¨å¹³å°æ¡æ¶VSåçå¼åæ·±åº¦è¯æµä¹2023ç
- è¯æµï¼è·¨ç«¯å¼åæ¡æ¶æ·±åº¦æ¨ªè¯ä¹2020ç
- è¯æµï¼æ·±å ¥æµè¯ä¸å¨ï¼ä¸»æµå¤ç«¯æ¡æ¶å¤§æ¯æ¦ä¹2019ç
- uni-app xå¨App端åflutterçæ¯è¾
è´¡ç®æå
å¦æä½ æ³åä¸è´¡ç®ï¼è¯·å é è¯»è´¡ç®æåã
Top Related Projects
🐰 Rax is a progressive framework for building universal application. https://rax.js.org
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Quasar Framework - Build high-performance VueJS user interfaces in record time
A framework for building native applications using React
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot