Top Related Projects
A cross-platform framework using Vue.js
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
A framework for building native applications using React
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
Quick Overview
Chameleon is a universal cross-platform framework that allows developers to build high-performance, battery-efficient, and feature-rich applications for multiple platforms, including web, iOS, and Android, using a single codebase.
Pros
- Cross-Platform Compatibility: Chameleon enables developers to create applications that can run seamlessly across different platforms, reducing the need for separate codebases and development efforts.
- Performance Optimization: The framework is designed to deliver high-performance applications, with a focus on battery efficiency and smooth user experiences.
- Unified Development Experience: Chameleon provides a unified development experience, allowing developers to use a single codebase and familiar tools, such as Vue.js, to build applications for various platforms.
- Extensive Ecosystem: The Chameleon ecosystem includes a wide range of built-in components, plugins, and tools, making it easier for developers to build feature-rich applications.
Cons
- Learning Curve: Developers who are new to Chameleon may need to invest time in learning the framework's unique concepts and development workflow, which can be a barrier to entry.
- Ecosystem Maturity: While Chameleon has a growing ecosystem, it may not have the same level of community support and third-party integrations as some more established cross-platform frameworks.
- Platform-Specific Customization: Developers may still need to write platform-specific code to handle unique requirements or take advantage of platform-specific features, which can add complexity to the development process.
- Documentation Quality: The quality and comprehensiveness of the project's documentation may vary, which can make it challenging for new users to get started or troubleshoot issues.
Code Examples
// Example 1: Creating a simple Chameleon component
<template>
<view>
<text>Hello, Chameleon!</text>
</view>
</template>
<script>
export default {
name: 'HelloWorld'
}
</script>
This code creates a simple Chameleon component that displays the text "Hello, Chameleon!".
// Example 2: Using Chameleon's built-in components
<template>
<view>
<button @click="handleClick">Click me</button>
<text>{{ message }}</text>
</view>
</template>
<script>
export default {
data() {
return {
message: ''
}
},
methods: {
handleClick() {
this.message = 'Button clicked!'
}
}
}
</script>
This example demonstrates the use of Chameleon's built-in button
and text
components, as well as how to handle user interactions and update the component's data.
// Example 3: Integrating Chameleon with Vue.js
import Vue from 'vue'
import Chameleon from 'chameleon-runtime'
Vue.use(Chameleon)
new Vue({
el: '#app',
template: '<HelloWorld />'
components: {
HelloWorld
}
})
This code shows how to integrate Chameleon with a Vue.js application, allowing developers to use Chameleon components within their Vue.js projects.
Getting Started
To get started with Chameleon, follow these steps:
-
Install the Chameleon CLI:
npm install -g chameleon-tool
-
Create a new Chameleon project:
cml create my-app
-
Navigate to the project directory and start the development server:
cd my-app cml dev
-
Open your browser and navigate to
http://localhost:8080
to see your Chameleon application running. -
Start building your application by modifying the code in the
src/
directory. Chameleon will automatically reload the page when you save changes to your files.
For more detailed information on Chameleon's features, configuration, and advanced usage, please refer to the Chameleon documentation.
Competitor Comparisons
A cross-platform framework using Vue.js
Pros of uni-app
- Larger community and more active development, with over 37k stars on GitHub
- Supports a wider range of platforms, including H5, iOS, Android, and various mini-programs
- More comprehensive documentation and learning resources available
Cons of uni-app
- Steeper learning curve due to its more complex architecture
- Potentially slower performance compared to Chameleon's lightweight approach
- Less flexibility in customizing the underlying framework
Code Comparison
uni-app:
<template>
<view class="content">
<text>{{ title }}</text>
</view>
</template>
<script>
export default {
data() {
return {
title: 'Hello'
}
}
}
</script>
Chameleon:
<template>
<view class="content">
<text>{{ title }}</text>
</view>
</template>
<script>
class Index {
data = {
title: 'Hello'
}
}
export default new Index();
</script>
Both frameworks use a similar Vue-like syntax for component structure, but uni-app follows Vue more closely, while Chameleon uses a class-based approach for the component logic.
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
Pros of Taro
- Larger community and more active development, with over 31k GitHub stars
- Supports more frameworks including React, Vue, and Nerv
- More comprehensive documentation and examples
Cons of Taro
- Steeper learning curve due to its broader scope
- May have more overhead for simpler projects
- Less focus on specific optimizations for certain platforms
Code Comparison
Taro component:
import { Component } from 'react'
import { View, Text } from '@tarojs/components'
export default class Index extends Component {
render () {
return (
<View className='index'>
<Text>Hello, World!</Text>
</View>
)
}
}
Chameleon component:
<template>
<view class="index">
<text>Hello, World!</text>
</view>
</template>
<script>
class Index {
created() {}
}
export default new Index();
</script>
Both frameworks aim to create cross-platform applications, but Taro offers more flexibility in terms of framework choice and has a larger community. Chameleon, on the other hand, provides a more focused approach with its own custom syntax, which may be easier to learn for developers new to cross-platform development. The code examples show that Taro uses a React-like syntax, while Chameleon employs a Vue-like template structure with custom scripting.
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Pros of Ionic Framework
- Larger community and ecosystem, with more resources and third-party plugins
- Supports multiple frameworks (Angular, React, Vue) for greater flexibility
- More mature and battle-tested in production environments
Cons of Ionic Framework
- Steeper learning curve, especially for developers new to web technologies
- Larger bundle sizes, which can impact performance on low-end devices
- More opinionated architecture, which may limit customization options
Code Comparison
Chameleon (Vue.js component):
<template>
<view class="container">
<text>{{ message }}</text>
</view>
</template>
<script>
export default {
data: () => ({ message: 'Hello, Chameleon!' })
}
</script>
Ionic Framework (React component):
import React from 'react';
import { IonContent, IonText } from '@ionic/react';
const HelloWorld: React.FC = () => (
<IonContent>
<IonText>Hello, Ionic!</IonText>
</IonContent>
);
export default HelloWorld;
Both frameworks aim to simplify cross-platform development, but Ionic Framework offers a more comprehensive solution with wider framework support. Chameleon focuses on a unified development experience across platforms, while Ionic leverages web technologies for hybrid app development. The choice between them depends on project requirements, team expertise, and desired customization level.
A framework for building native applications using React
Pros of React Native
- Larger community and ecosystem, with more third-party libraries and resources
- Better performance for complex applications due to native rendering
- Stronger corporate backing and long-term support from Facebook
Cons of React Native
- Steeper learning curve, especially for developers new to React
- More complex setup and configuration process
- Potentially larger app size due to bundled JavaScript runtime
Code Comparison
React Native:
import React from 'react';
import { View, Text } from 'react-native';
const App = () => (
<View>
<Text>Hello, React Native!</Text>
</View>
);
Chameleon:
<template>
<view>
<text>Hello, Chameleon!</text>
</view>
</template>
<script>
class App {}
export default new App();
</script>
React Native uses JSX syntax and React components, while Chameleon employs a Vue-like template structure. React Native's approach may be more familiar to React developers, whereas Chameleon's syntax might be easier for those with Vue experience.
Both frameworks aim to create cross-platform mobile applications, but React Native has a more established presence in the market. Chameleon, developed by DiDi, focuses on multi-end adaptation and may be more suitable for simpler applications or those targeting multiple platforms beyond just iOS and Android.
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
Pros of Flutter
- Larger community and ecosystem, with more resources and third-party packages
- Better performance for complex UI and animations
- More mature and stable, with backing from Google
Cons of Flutter
- Steeper learning curve, especially for developers new to Dart
- Larger app size compared to native applications
- Limited access to platform-specific features without plugins
Code Comparison
Flutter:
import 'package:flutter/material.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter App')),
body: Center(child: Text('Hello, World!')),
),
);
}
}
Chameleon:
<template>
<view class="container">
<text>Hello, World!</text>
</view>
</template>
<script>
export default {
// Component logic here
}
</script>
Flutter uses Dart and a widget-based approach, while Chameleon uses a Vue-like syntax with HTML-style templates. Flutter's code is more verbose but offers more control over the UI structure. Chameleon's syntax is simpler and may be more familiar to web developers.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Chameleon/kÉËmiËlɪÉn/ï¼ç®åCML
ï¼ä¸æå塿¢
é¾
ï¼ä¸æææåè²é¾
ï¼æå³çå°±ååè²é¾ä¸æ ·è½éåºä¸åç¯å¢ç跨端æ´ä½è§£å³æ¹æ¡ã
English Introduction | 䏿ä»ç»
ææ¡£
- å®è£
- å¿«é䏿
- API ææ¡£
- ç»ä»¶ææ¡£
- èµæºæ±æ» ð°awesome-cmlï¼ä¾èµåºãdemoã宿´åºç¨ç¤ºä¾ãå¦ä¹ èµæº
- åç»è§å
- äºåé䏿è§é¢æç¨
- éæ¡å车 chameleon 跨平å°å®è·µå享
- è°å¨ä½¿ç¨ï¼
ä»åºæ´æ°è¯´æ
æ¬ä»åºä» å å«ç¼è¯æ¶ä»£ç ï¼å ¨é¨å¼æºä»£ç åè§ï¼https://github.com/chameleon-team
master为稳å®çæ¬ï¼é¤äºç´§æ¥ bug ä¿®å¤ï¼æ¯ä»½ä»£ç æäº¤é½æå¾ä¸¥æ ¼çå叿µç¨è§èï¼ä¼å å¨åæ¯ç»å䏿®µæ¶é´ç°åº¦æï¼ç¡®è®¤ç¨³å®å¯ç¨åæä¼åå¹¶å° masterï¼ è¿è¡ä¸ç项ç®åæ¯ä»ç»
CML å³ å¤ç«¯
æ¯æå¹³å°ï¼webã微信å°ç¨åºãæ¯ä»å®å°ç¨åºãç¾åº¦å°ç¨åºãandroid(weex)ãios(weex)ãqq å°ç¨åºãåèè·³å¨å°ç¨åºãå¿«åºç¨ãæç»æ´æ°ä¸
ä¸ç«¯æè§å³å¤ç«¯æè§ââå¤ç«¯é«åº¦ä¸è´ï¼æ éå ³æ³¨åç«¯ææ¡£ã
åºäºå¤æåè®®ä¸å½±åå端差å¼åçµæ´»æ§
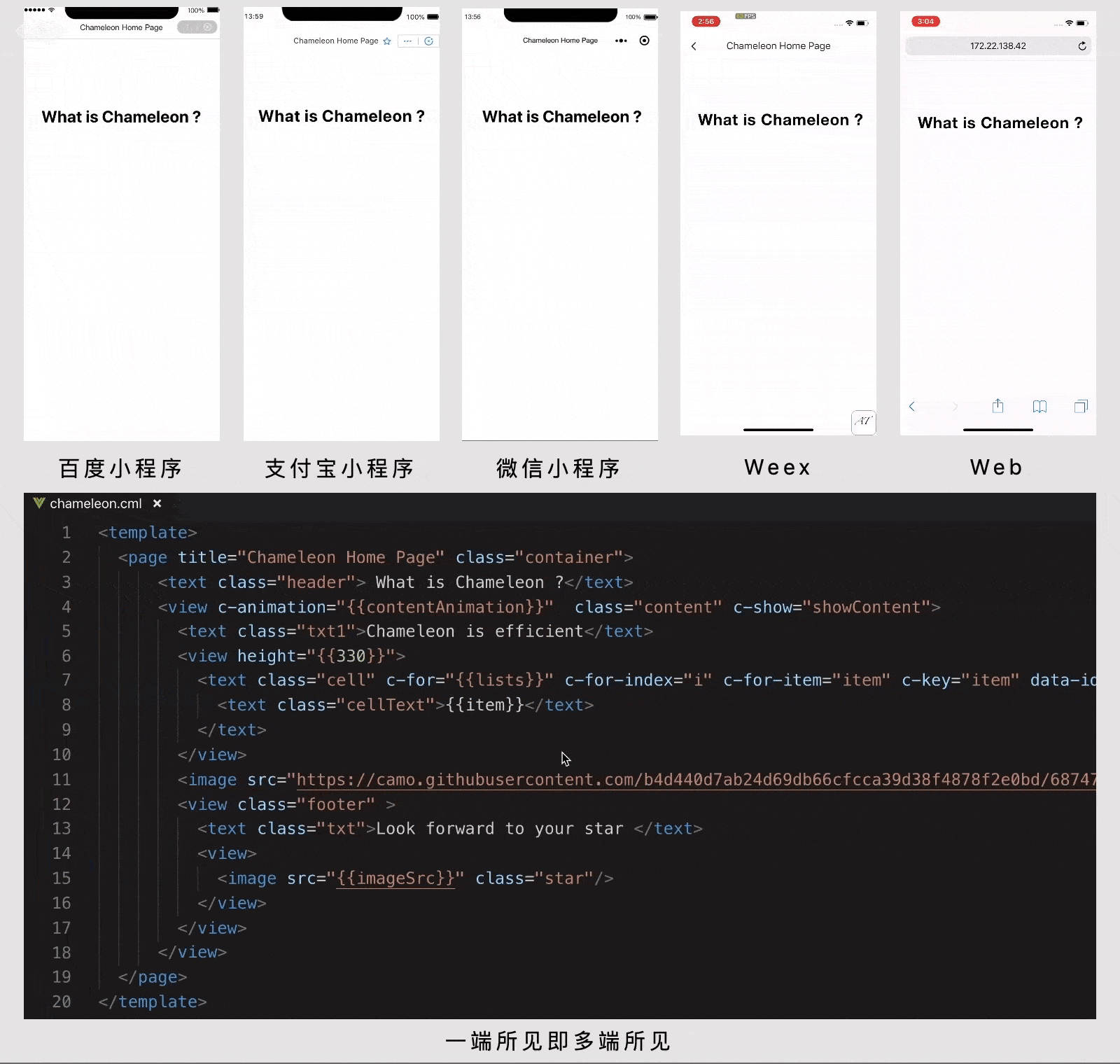
web | 微信å°ç¨åº | native-weex | ç¾åº¦å°ç¨åº | æ¯ä»å®å°ç¨åº |
---|---|---|---|---|
![]() | ![]() | ![]() | ![]() | ![]() |
èæ¯
ç ååå¦å¨ç«¯å æ¢è¿½æ± h5 ççµæ´»æ§ï¼ä¹è¦è¿½æ±æ§è½è¶è¿äºåçã é¢å¯¹å ¥å£æ©å¼ ï¼App 客æ·ç«¯ã微信å°ç¨åºãæ¯ä»å®å°ç¨åºãç¾åº¦å°ç¨åºãAndroid ååèçå¿«åºç¨ãå ¶ä»ç±»å°ç¨åºï¼åä¸åè½å¨åå¹³å°é½è¦éå¤å®ç°ï¼å¼ååç»´æ¤ææ¬æåå¢å ãè¿«åéè¦ç»´æ¤ä¸å¥ä»£ç å¯ä»¥æå»ºå¤å ¥å£çè§£å³æ¹æ¡ï¼æ»´æ»´è·¨ç«¯è§£å³æ¹æ¡ Chameleon ç»äºåå¸ãçæ£ä¸æ³¨äºè®©ä¸å¥ä»£ç è¿è¡å¤ç«¯ã
设计ç念
è½¯ä»¶æ¶æè®¾è®¡é颿åºç¡çæ¦å¿µâæåâåâåå¹¶âï¼æåçæä¹æ¯âåèæ²»ä¹âï¼å°å¤æé®é¢æåæåä¸é®é¢è§£å³ï¼æ¯å¦å端ä¸å¡ç³»ç»çâå¾®æå¡åâ设计ï¼âåå¹¶âçæä¹æ¯å°åæ ·çä¸å¡éæ±æ½è±¡æ¶æå°ä¸åï¼è¾¾æé«æçé«è´¨éçç®çï¼ä¾å¦å端ä¸å¡ç³»ç»ä¸çâä¸å°æå¡â设计ã
è Chameleon å±äºåè ï¼éè¿å®ä¹ç»ä¸çè¯è¨æ¡æ¶+ç»ä¸å¤æåè®®ï¼ä»å¤ç«¯ï¼å¯¹åºå¤ä¸ªç¬ç«æå¡ï¼ä¸å¡ä¸æ½ç¦»åºèªæä½ç³»ãè¿ç»æ§å¼ºãå¯ç»´æ¤å¼ºçâå端ä¸å°æå¡âã
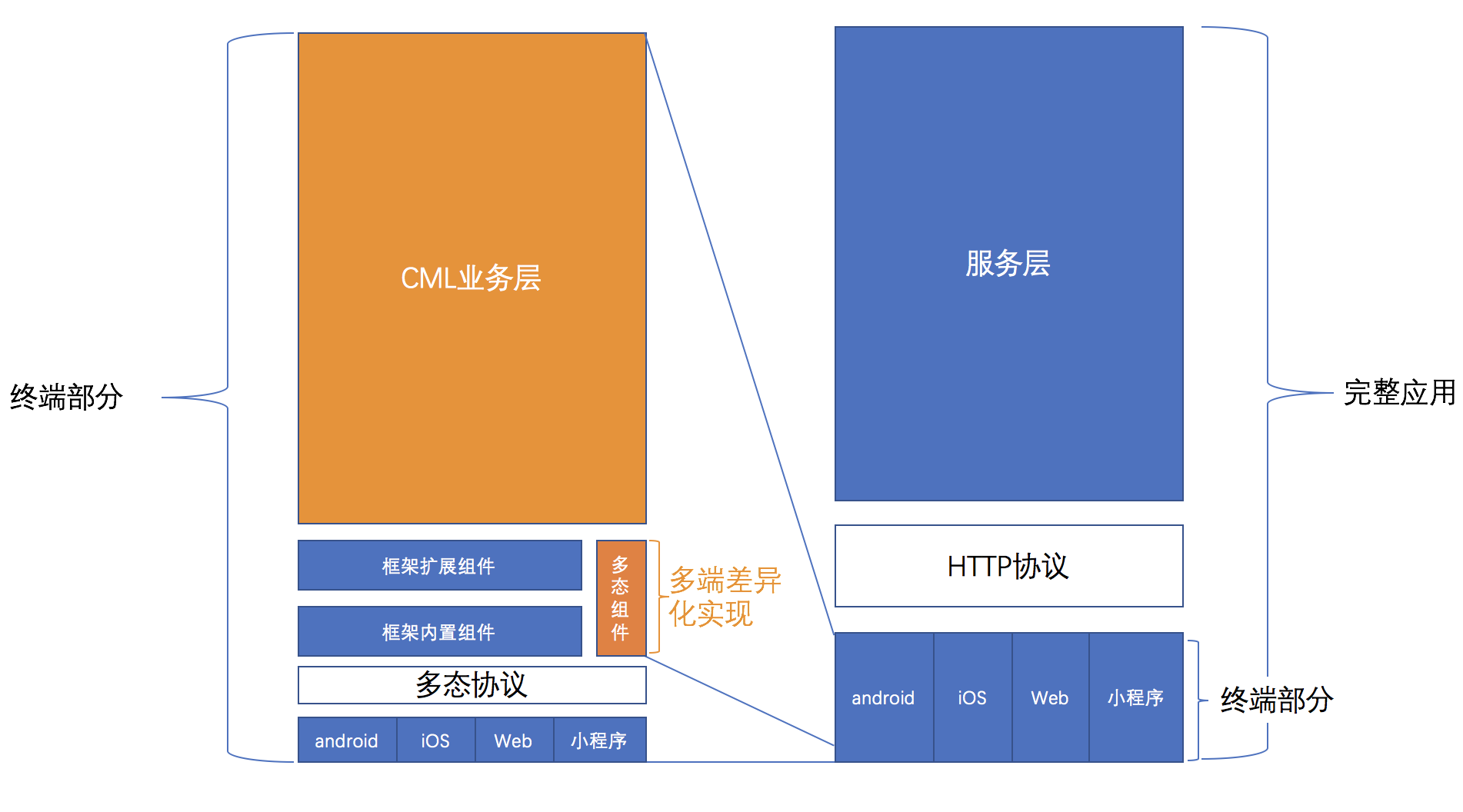
è·¨ç«¯ç®æ
è½ç¶ä¸åå端ç¯å¢ååä¸åï¼ä½ä¸åä¸ç¦»å ¶å®çæ¯ MVVM æ¶æææ³ï¼Chameleon ç®æ æ¯è®© MVVM 跨端ç¯å¢å¤§ç»ä¸ã
å¼åè¯è¨
代ç 示ä¾
<template>
<view>
<text>{{title}}</text><text>{{reversedTitle}}</text>
</view>
</template>
<script>
class Index {
data = {
title: "chameleon"
}
computed = {
reversedTitle: function () {
return this.title.split('').reverse().join('')
}
}
mounted() {}
destroyed() {}
}
export default new Index();
</script>
ä»äºè¿ç½é¡µç¼ç¨ç人ç¥éï¼ç½é¡µç¼ç¨éç¨çæ¯ HTML + CSS + JS è¿æ ·çç»åï¼åæ ·éçï¼chameleon ä¸éç¨çæ¯ CML + CMSS + JSã
JSè¯æ³ç¨äºå¤ç页é¢çé»è¾å±ï¼ä¸æ®éç½é¡µç¼ç¨ç¸æ¯ï¼æ¬é¡¹ç®ç®æ å®ä¹æ å MVVM æ¡æ¶ï¼æ¥æå®æ´ççå½å¨æï¼watchï¼computedï¼æ°æ®ååç»å®çä¼ç§çç¹æ§ï¼è½å¤å¿«éæé«å¼åé度ãéä½ç»´æ¤ææ¬ã
CMLï¼Chameleon Markup Languageï¼ç¨äºæè¿°é¡µé¢çç»æï¼æä»¬ç¥é HTML æ¯æä¸å¥æ åçè¯ä¹åæ ç¾ï¼ä¾å¦ææ¬æ¯<span>
æé®æ¯<button>
ãCML åæ ·å
·æä¸å¥æ åçæ ç¾ï¼æä»¬å°æ ç¾å®ä¹ä¸ºç»ä»¶
ï¼CML ä¸ºç¨æ·æä¾äºä¸ç³»åç»ä»¶ãåæ¶ CML ä¸è¿æ¯ææ¨¡æ¿è¯æ³ï¼ä¾å¦æ¡ä»¶æ¸²æãå表渲æï¼æ°æ®ç»å®ççãåæ¶ï¼CML æ¯æä½¿ç¨ç±» VUE è¯æ³ï¼è®©ä½ æ´å¿«å
¥æã
CMSS(Chameleon Style Sheets)ç¨äºæè¿° CML 页é¢ç»æçæ ·å¼è¯è¨ï¼å
¶å
·æå¤§é¨å CSS çç¹æ§ï¼å¹¶ä¸è¿å¯ä»¥æ¯æåç§ css çé¢å¤è¯è¨less stylus
ã
CML éç¨ä¸ Vue ä¸è´çç»ä»¶åæ¹æ¡ãåæä»¶ç»ç»æ¹å¼ãçå½å¨æï¼åæ¶æ°æ®ååºè½åå¯¹é½ Vueï¼æ°æ®ç®¡çè½åå¯¹é½ Vuexï¼é常æ¹ä¾¿å¼åè 䏿ãç»´æ¤ã
éè¿ä»¥ä¸å¯¹äºå¼åè¯è¨çä»ç»ï¼ç¸ä¿¡ä½ çå°åªè¦æ¯æè¿ç½é¡µç¼ç¨ç¥è¯ç人é½å¯ä»¥å¿«éç䏿chameleonçå¼åã
å¤ç«¯é«åº¦ä¸è´
æ·±å ¥å°ç¼ç¨è¯è¨ç»´åº¦ä¿éä¸è´æ§ï¼å æ¬æ¡æ¶ãçå½å¨æãå ç½®ç»ä»¶ãäºä»¶éä¿¡ãè·¯ç±ãçé¢å¸å±ãçé¢åä½ãç»ä»¶ä½ç¨åãç»ä»¶éä¿¡çé«åº¦ç»ä¸
丰å¯çç»ä»¶
å¨ç¨ CML å页颿¶ï¼chameleon æä¾äºä¸°å¯çç»ä»¶ä¾å¼åè
使ç¨ï¼å
ç½®çæbutton switch radio checkbox
çç»ä»¶ï¼æ©å±çæc-picker c-dialog c-loading
çç,è¦çäºå¼åå·¥ä½ä¸å¸¸ç¨çç»ä»¶ã
丰å¯ç API
ä¸ºäºæ¹ä¾¿å¼åè
ç髿å¼åï¼chameleon æä¾äºä¸°å¯ç API åº,åå¸ä¸º npm å
chameleon-api
ï¼éé¢å
æ¬äºç½ç»è¯·æ±ãæ°æ®åå¨ãå°çä½ç½®ãç³»ç»ä¿¡æ¯ãå¨ç»çæ¹æ³ã
èªç±å®å¶ API åç»ä»¶
åºäºå¼ºå¤§ç夿åè®®ï¼å¯èªç±æ©å±ä»»æ API åç»ä»¶ï¼ä¸å¼ºä¾èµæ¡æ¶çæ´æ°ãå端åå§é¡¹ç®ä¸å·²ç§¯ç´¯å¤§éç»ä»¶ï¼ä¹è½ç´æ¥å¼å ¥å°è·¨ç«¯é¡¹ç®ä¸ä½¿ç¨ã
åºäºå¼ºå¤§ç夿åè®®ï¼å åé离å端差å¼åå®ç°ï¼è½»æ¾ç»´æ¤ä¸å¥ä»£ç å®ç°è·¨å¤ç«¯
è§èæ ¡éª
代ç è§èæ ¡éªï¼å½åºç°ä¸ç¬¦åè§èè¦æ±çä»£ç æ¶ï¼ç¼è¾å¨ä¼å±ç¤ºæºè½æç¤ºï¼ä¸ç¨æ¨ä¸ªè°è¯å端代ç ï¼åæ¶å½ä»¤è¡å¯å¨çªå£ä¹ä¼æç¤ºä»£ç çé误ä½ç½®ã
æ¸è¿å¼è·¨ç«¯
æ¢æ³ä¸å¥ä»£ç è¿è¡å¤ç«¯ï¼åä¸ç¨å¤§åéæ§çéæé¡¹ç®ï¼ä¸ä» å¯ä»¥ç¨ cml å¼å页é¢ï¼ä¹å¯ä»¥å°å¤ç«¯éç¨ç»ä»¶ç¨ cml å¼åï¼ç´æ¥å¨åæé¡¹ç®éé¢è°ç¨ã
å è¿å端å¼åä½éª
Chameleon ä¸ä» ä» æ¯è·¨ç«¯è§£å³æ¹æ¡ãåºäºä¼ç§çå端æå å·¥å · Webpackï¼å¸æ¶äºä¸å å¤å¹´æ¥ç§¯ç´¯çææç¨çå·¥ç¨åè®¾è®¡ï¼æä¾äºå端åºç¡å¼åèææ¶å½ä»¤å·¥å ·ï¼å¸®å©ç«¯å¼åè ä»å¼åãèè°ãæµè¯ãä¸çº¿çå ¨æµç¨é«æç宿ä¸å¡å¼åã
èç³»æä»¬
Beatles Chameleon å¢é
è´è´£äººï¼Conan
å 鍿åï¼éå¿åãSgoddonã卿ºä¸çº¯ãJalonãJackãå¡å°ºååãchangeãObserverãKevinãguoqingSmileãMr.MYãJiMãlzcãååå¾ å®ãæ±æºæãäºãé¾ç£ãw55ãå°é¾ã䏿å°å½¬ãè£æ¯è¶
ç¹å«é¸£è°¢
(头å空) | (头å空) | ||
---|---|---|---|
zheyizhifengï¼å¿«åºç¨ï¼ | whuhenryleeï¼å¿«åºç¨ï¼ | brovenï¼é¿éå½±ä¸ï¼ | Jeanyï¼èæ TV) |
è´¡ç®è 们
å¿«åºç¨å®æ¹ç åå¢éãluyixinãz-mirrorãå¤å¤ç°ç«ï¼ç¾åº¦ï¼ kingsleydon
微信 & QQ 交æµç¾¤
微信
QQ
åè®®
Apache-2.0 license
Chameleon åºäº Apache-2.0 åè®®è¿è¡ååå使ç¨ï¼æ´å¤ä¿¡æ¯åè§åè®®æä»¶ã
åæ é¾æ¥
DoraemonKit /'dÉ:ra:'emÉn/ï¼ç®ç§°DoKitï¼ ä¸æ¬¾åè½é½å ¨ç客æ·ç«¯ï¼ iOS ãAndroidã微信å°ç¨åº ï¼ç å婿ï¼ä½ å¼å¾æ¥æã
Mand Mobile 䏿¬¾ä¼ç§çé¢åéèåºæ¯ç ç§»å¨ç«¯UIç»ä»¶åºã
Cube-UI åºäº Vue.js å®ç°çç²¾è´ç§»å¨ç«¯ç»ä»¶åºã
Top Related Projects
A cross-platform framework using Vue.js
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
A framework for building native applications using React
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot