mand-mobile
💰 A mobile UI toolkit, based on Vue.js 2, designed for financial scenarios.
Top Related Projects
A lightweight, customizable Vue UI library for mobile web apps.
Essential UI blocks for building mobile web apps.
🏄 A rich interaction, lightweight, high performance UI library based on Weex.
:dog: 一套组件化、可复用、易扩展的微信小程序 UI 组件库
一款基于 Taro 框架开发的多端 UI 组件库
Mobile UI elements for Vue.js
Quick Overview
Mand Mobile is a mobile UI toolkit for Vue.js, developed by DiDi. It provides a set of high-quality components and demos for building mobile web apps with a consistent look and feel. The library is designed to be lightweight, customizable, and easy to use.
Pros
- Comprehensive set of mobile-optimized UI components
- Customizable themes and styles
- Well-documented with live demos and API references
- Actively maintained by DiDi, a major tech company
Cons
- Primarily focused on Chinese market, which may limit its global adoption
- Limited community support compared to more popular UI frameworks
- Some components may not follow Western design conventions
- Documentation is primarily in Chinese, which can be a barrier for non-Chinese speakers
Code Examples
- Basic Button Component:
<template>
<md-button>Click me</md-button>
</template>
<script>
import { Button } from 'mand-mobile'
export default {
components: {
[Button.name]: Button
}
}
</script>
- Form Input with Validation:
<template>
<md-field>
<md-input-item
title="Username"
placeholder="Please enter username"
v-model="username"
:error="usernameError"
/>
</md-field>
</template>
<script>
import { Field, InputItem } from 'mand-mobile'
export default {
components: {
[Field.name]: Field,
[InputItem.name]: InputItem
},
data() {
return {
username: '',
usernameError: ''
}
}
}
</script>
- Dialog Component:
<template>
<md-button @click="showDialog">Show Dialog</md-button>
</template>
<script>
import { Button, Dialog } from 'mand-mobile'
export default {
components: {
[Button.name]: Button
},
methods: {
showDialog() {
Dialog.alert({
title: 'Title',
content: 'This is the dialog content',
confirmText: 'OK'
})
}
}
}
</script>
Getting Started
- Install Mand Mobile:
npm install mand-mobile --save
- Import and use components in your Vue.js project:
import Vue from 'vue'
import { Button, Dialog } from 'mand-mobile'
import 'mand-mobile/lib/mand-mobile.css'
Vue.component(Button.name, Button)
Vue.use(Dialog)
- Use components in your Vue template:
<template>
<div>
<md-button @click="showDialog">Show Dialog</md-button>
</div>
</template>
<script>
export default {
methods: {
showDialog() {
this.$dialog.alert({
content: 'Hello, Mand Mobile!'
})
}
}
}
</script>
Competitor Comparisons
A lightweight, customizable Vue UI library for mobile web apps.
Pros of Vant
- Larger community and more frequent updates (30k+ stars, weekly releases)
- More comprehensive component library (80+ components)
- Better documentation with live demos and playground
Cons of Vant
- Primarily focused on Vue.js, less flexible for other frameworks
- Steeper learning curve due to more complex API and options
Code Comparison
Vant:
<template>
<van-button type="primary" @click="showToast">Click me</van-button>
</template>
<script>
import { Toast } from 'vant';
export default {
methods: {
showToast() {
Toast('Hello Vant');
}
}
}
</script>
Mand Mobile:
<template>
<md-button type="primary" @click="showToast">Click me</md-button>
</template>
<script>
import { Toast } from 'mand-mobile'
export default {
methods: {
showToast() {
Toast.info('Hello Mand Mobile')
}
}
}
</script>
Both libraries offer similar functionality, but Vant provides more customization options and a wider range of components. Mand Mobile has a simpler API and may be easier for beginners to pick up. The choice between the two depends on project requirements and team expertise.
Essential UI blocks for building mobile web apps.
Pros of Ant Design Mobile
- Larger community and more frequent updates
- More comprehensive documentation and examples
- Better integration with React ecosystem
Cons of Ant Design Mobile
- Heavier bundle size
- Less customizable design system
- Steeper learning curve for beginners
Code Comparison
Ant Design Mobile:
import { Button } from 'antd-mobile'
const App = () => (
<Button color='primary' fill='solid'>
Submit
</Button>
)
Mand Mobile:
<template>
<md-button type="primary">Submit</md-button>
</template>
<script>
import { Button } from 'mand-mobile'
export default {
components: { [Button.name]: Button }
}
</script>
Ant Design Mobile uses a more React-centric approach, while Mand Mobile is designed for Vue.js applications. Ant Design Mobile offers more customization options directly in the component props, whereas Mand Mobile keeps its API simpler.
Both libraries provide similar functionality, but Ant Design Mobile has a more extensive component library and better documentation. Mand Mobile, on the other hand, offers a lighter-weight solution with a focus on performance and ease of use for Vue.js developers.
🏄 A rich interaction, lightweight, high performance UI library based on Weex.
Pros of Weex UI
- Larger community and more contributors, potentially leading to better support and faster development
- Part of the Apache Software Foundation, which may provide more stability and long-term support
- Wider range of components and more comprehensive documentation
Cons of Weex UI
- Steeper learning curve due to its integration with the Weex framework
- Less frequent updates compared to Mand Mobile
- May require more setup and configuration for non-Weex projects
Code Comparison
Mand Mobile component usage:
import { Button } from 'mand-mobile'
<Button type="primary">Primary Button</Button>
Weex UI component usage:
import { WxcButton } from 'weex-ui'
<wxc-button text="Primary Button" type="primary"></wxc-button>
Both libraries offer similar component-based structures, but Weex UI components are typically prefixed with "wxc-" and use attributes for configuration, while Mand Mobile follows a more React-like approach with props.
Weex UI is better suited for projects already using the Weex framework, while Mand Mobile may be more accessible for general mobile web development. The choice between the two depends on the specific project requirements, existing tech stack, and developer familiarity with each library's ecosystem.
:dog: 一套组件化、可复用、易扩展的微信小程序 UI 组件库
Pros of wux-weapp
- More comprehensive component library with a wider range of UI elements
- Better documentation and examples for each component
- Active community support and regular updates
Cons of wux-weapp
- Specifically designed for WeChat Mini Programs, limiting its use in other platforms
- Steeper learning curve due to the extensive component library
- Larger file size and potential performance impact on smaller applications
Code Comparison
wux-weapp:
<wux-button size="small" type="positive">Button</wux-button>
<wux-input label="Username" />
<wux-calendar />
mand-mobile:
<md-button type="primary">Button</md-button>
<md-field title="Username">
<md-input-item />
</md-field>
<md-date-picker />
Both libraries offer similar components, but wux-weapp tends to have more self-contained and straightforward implementations. mand-mobile often requires nested components for complex UI elements.
wux-weapp is tailored for WeChat Mini Programs, providing a rich set of components specific to that platform. It offers extensive customization options and detailed documentation, making it ideal for complex WeChat applications.
mand-mobile, developed by DiDi, is a more versatile mobile UI toolkit that can be used across various mobile web platforms. It has a cleaner, more minimalist design approach and may be better suited for simpler applications or those requiring cross-platform compatibility.
一款基于 Taro 框架开发的多端 UI 组件库
Pros of Taro UI
- Cross-platform compatibility: Supports multiple frameworks (React, Vue, Nerv)
- Extensive component library: Offers a wide range of UI components
- Active community and frequent updates
Cons of Taro UI
- Steeper learning curve due to Taro framework integration
- Limited customization options compared to Mand Mobile
Code Comparison
Taro UI (Button component):
import { AtButton } from 'taro-ui'
const MyComponent = () => {
return <AtButton type='primary'>I'm a button</AtButton>
}
Mand Mobile (Button component):
import { Button } from 'mand-mobile'
const MyComponent = () => {
return <Button type="primary">I'm a button</Button>
}
Key Differences
- Taro UI is built on top of the Taro framework, while Mand Mobile is a standalone UI library
- Mand Mobile focuses primarily on Vue.js, whereas Taro UI supports multiple frameworks
- Taro UI has a larger component library, but Mand Mobile offers more detailed customization options
Use Cases
- Choose Taro UI for cross-platform projects or when using the Taro framework
- Opt for Mand Mobile when working exclusively with Vue.js or requiring fine-grained component customization
Both libraries provide comprehensive mobile UI solutions, but their ideal use cases differ based on project requirements and framework preferences.
Mobile UI elements for Vue.js
Pros of mint-ui
- More mature and established project with a larger community
- Wider range of components and features
- Better documentation and examples
Cons of mint-ui
- Less frequent updates and maintenance
- Heavier bundle size due to more components
- Limited customization options compared to mand-mobile
Code Comparison
mand-mobile:
<template>
<md-button type="primary" @click="handleClick">Click me</md-button>
</template>
<script>
import { Button } from 'mand-mobile'
export default {
components: { 'md-button': Button },
methods: {
handleClick() {
console.log('Button clicked')
}
}
}
</script>
mint-ui:
<template>
<mt-button type="primary" @click="handleClick">Click me</mt-button>
</template>
<script>
import { Button } from 'mint-ui'
export default {
components: { 'mt-button': Button },
methods: {
handleClick() {
console.log('Button clicked')
}
}
}
</script>
Both libraries offer similar component usage, with slight differences in naming conventions and import statements. mand-mobile uses the md-
prefix for components, while mint-ui uses mt-
. The overall structure and implementation are quite similar, making it easy for developers to switch between the two if needed.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
mand-mobile
A mobile UI toolkit, based on Vue.js 2, designed for financial scenarios
English | 䏿
Links
Preview
You can scan the following QR code to access the examples:
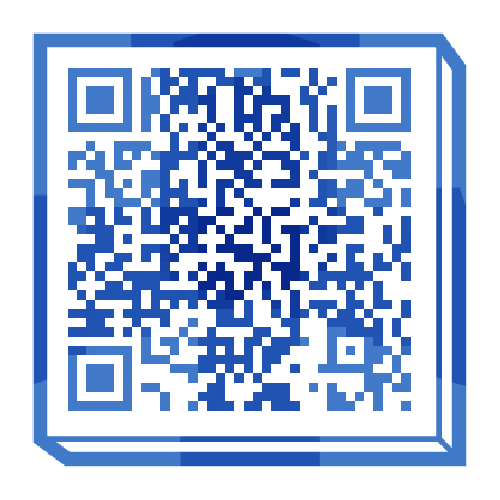
Install & Usage
Template for new project
Vue CLI 2
New project can be initialized and integrated with mand-mobile by vue-cli-2 with mand-mobile-template.
vue init mand-mobile/mand-mobile-template my-project
Vue CLI 3
New project can be initialized and integrated with mand-mobile by vue-cli with vue-cli-plugin-mand.
vue create my-project
cd my-project
npm install --save-dev vue-cli-plugin-mand
vue invoke mand
Manually
npm install mand-mobile --save
Import
- Use babel-plugin-import or ts-import-plugin (Recommended)
import { Button } from 'mand-mobile'
- Manually import
import Button from 'mand-mobile/lib/button'
- Totally import
import Vue from 'vue'
import mandMobile from 'mand-mobile'
import 'mand-mobile/lib/mand-mobile.css'
Vue.use(mandMobile)
Usage
Select the components you need to build your webapp. Find more details in Quick Start.
Development
git clone git@github.com:didi/mand-mobile.git
cd mand-mobile
npm install
npm run dev
Open your browser and visit http://127.0.0.1:4000. Find more details in Development Guide.
Contributing 
Welcome to contribute by creating issues or sending pull requests. See Contributing Guide for guidelines.
Community
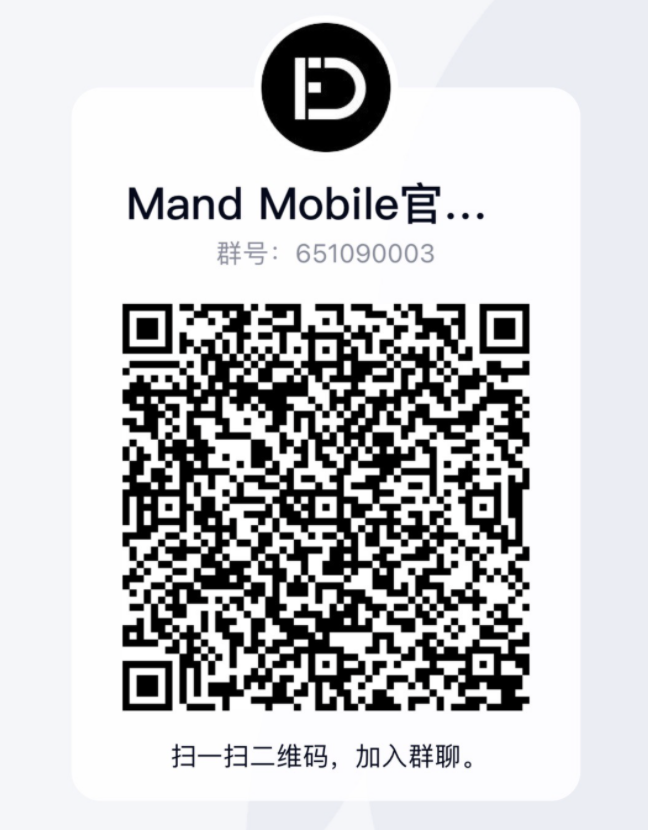
License
Mand Mobile is licensed under the Apache License 2.0. See the LICENSE file.
Useful Links
-
Hummer is a set of high-performance and highly available cross-terminal development framework, a set of code can support the development of Android and iOS applications at the same time. Now supports Vue/TypeScript/JavaScript, for front-end developers, there is always one suitable for you.
-
DoraemonKit /'dÉ:ra:'emÉn/: A full-featured App (iOS & Android) development assistant. You deserve it.
-
Chameleon /kÉËmiËlɪÉn/: Unify all platforms(Web/Weex/Mini program) with MVVM. Focus on Write Once Run AnyWhere.
Top Related Projects
A lightweight, customizable Vue UI library for mobile web apps.
Essential UI blocks for building mobile web apps.
🏄 A rich interaction, lightweight, high performance UI library based on Weex.
:dog: 一套组件化、可复用、易扩展的微信小程序 UI 组件库
一款基于 Taro 框架开发的多端 UI 组件库
Mobile UI elements for Vue.js
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot