Top Related Projects
Free and Open Source, Distributed, RESTful Search Engine
Deprecated: Use the official Elasticsearch client for Go at https://github.com/elastic/go-elasticsearch
The official Go client for Elasticsearch
Official Python client for Elasticsearch
This strongly-typed, client library enables working with Elasticsearch. It is the official client maintained and supported by Elastic.
Ruby integrations for Elasticsearch
Quick Overview
Elasticsearch-js is the official Node.js client for Elasticsearch. It provides a simple and intuitive API for interacting with Elasticsearch clusters, allowing developers to perform various operations such as indexing, searching, and managing documents and indices.
Pros
- Officially maintained by Elastic, ensuring compatibility and up-to-date features
- Supports both callback and promise-based programming styles
- Comprehensive documentation and examples
- Automatic node selection and load balancing for cluster connections
Cons
- Learning curve for complex queries and aggregations
- Performance overhead for large-scale operations compared to direct HTTP requests
- Limited support for older Elasticsearch versions
- Some advanced features require additional configuration
Code Examples
- Creating a client and indexing a document:
const { Client } = require('@elastic/elasticsearch')
const client = new Client({ node: 'http://localhost:9200' })
async function indexDocument() {
await client.index({
index: 'my-index',
document: {
title: 'Test document',
content: 'This is a test document'
}
})
}
- Performing a search query:
async function searchDocuments() {
const result = await client.search({
index: 'my-index',
query: {
match: { content: 'test' }
}
})
console.log(result.hits.hits)
}
- Updating a document:
async function updateDocument() {
await client.update({
index: 'my-index',
id: 'document_id',
doc: {
title: 'Updated title'
}
})
}
Getting Started
- Install the package:
npm install @elastic/elasticsearch
- Create a client and perform operations:
const { Client } = require('@elastic/elasticsearch')
const client = new Client({ node: 'http://localhost:9200' })
async function run() {
// Index a document
await client.index({
index: 'my-index',
document: {
title: 'Hello Elasticsearch',
content: 'This is my first document'
}
})
// Search for documents
const result = await client.search({
index: 'my-index',
query: {
match: { content: 'first' }
}
})
console.log(result.hits.hits)
}
run().catch(console.error)
Competitor Comparisons
Free and Open Source, Distributed, RESTful Search Engine
Pros of Elasticsearch
- Written in Java, offering high performance and scalability
- Provides a comprehensive set of features for full-text search and analytics
- Supports a wide range of plugins and integrations
Cons of Elasticsearch
- Steeper learning curve for developers not familiar with Java
- Requires more system resources compared to lightweight clients
- Configuration and setup can be more complex
Code Comparison
Elasticsearch (Java):
SearchResponse response = client.prepareSearch("index")
.setQuery(QueryBuilders.matchQuery("field", "value"))
.setSize(10)
.execute()
.actionGet();
elasticsearch-js (JavaScript):
const response = await client.search({
index: 'index',
query: { match: { field: 'value' } },
size: 10
});
Key Differences
- Elasticsearch is the core search engine, while elasticsearch-js is a client library for Node.js and browsers
- Elasticsearch handles indexing, searching, and data storage, whereas elasticsearch-js provides an API to interact with Elasticsearch
- Elasticsearch requires a separate server setup, while elasticsearch-js can be easily integrated into existing JavaScript projects
Use Cases
- Elasticsearch: Building large-scale search applications, log analysis, and complex data processing
- elasticsearch-js: Developing web applications that need to interact with Elasticsearch, creating admin interfaces, or building lightweight search functionality in JavaScript environments
Deprecated: Use the official Elasticsearch client for Go at https://github.com/elastic/go-elasticsearch
Pros of elastic
- Written in Go, offering better performance and concurrency support
- More comprehensive and feature-rich API, covering a wider range of Elasticsearch functionalities
- Actively maintained with frequent updates and improvements
Cons of elastic
- Steeper learning curve due to its extensive feature set
- Larger codebase and dependencies, potentially increasing project size
- May be overkill for simple Elasticsearch integrations
Code Comparison
elasticsearch-js:
const { Client } = require('@elastic/elasticsearch')
const client = new Client({ node: 'http://localhost:9200' })
const result = await client.search({
index: 'my-index',
body: { query: { match: { title: 'search' } } }
})
elastic:
import "github.com/olivere/elastic/v7"
client, _ := elastic.NewClient()
result, _ := client.Search().
Index("my-index").
Query(elastic.MatchQuery("title", "search")).
Do(context.Background())
Both libraries provide similar functionality for basic Elasticsearch operations. elasticsearch-js offers a more JavaScript-friendly API, while elastic provides a fluent interface typical of Go libraries. The elastic library requires more verbose setup but offers stronger typing and compile-time checks.
The official Go client for Elasticsearch
Pros of go-elasticsearch
- Better performance and lower memory footprint due to Go's efficiency
- Strong type safety and compile-time checks
- Concurrency support with goroutines and channels
Cons of go-elasticsearch
- Less mature ecosystem compared to JavaScript
- Steeper learning curve for developers not familiar with Go
- Fewer third-party libraries and integrations available
Code Comparison
elasticsearch-js:
const { Client } = require('@elastic/elasticsearch')
const client = new Client({ node: 'http://localhost:9200' })
const result = await client.search({
index: 'my-index',
body: { query: { match: { title: 'search' } } }
})
go-elasticsearch:
es, _ := elasticsearch.NewDefaultClient()
res, _ := es.Search(
es.Search.WithIndex("my-index"),
es.Search.WithBody(strings.NewReader(`{"query":{"match":{"title":"search"}}}`)),
)
Both libraries provide similar functionality for interacting with Elasticsearch, but their syntax and usage differ due to the underlying language characteristics. The go-elasticsearch client offers a more verbose but type-safe approach, while elasticsearch-js provides a more concise and flexible API typical of JavaScript libraries.
Official Python client for Elasticsearch
Pros of elasticsearch-py
- Native Python implementation, offering better integration with Python ecosystems
- Supports both synchronous and asynchronous clients
- More Pythonic API design, following Python conventions and best practices
Cons of elasticsearch-py
- Generally slower performance compared to the JavaScript client
- Less frequent updates and potentially slower adoption of new Elasticsearch features
- Smaller community and fewer third-party resources available
Code Comparison
elasticsearch-py:
from elasticsearch import Elasticsearch
es = Elasticsearch(["http://localhost:9200"])
result = es.search(index="my-index", body={"query": {"match_all": {}}})
elasticsearch-js:
const { Client } = require('@elastic/elasticsearch')
const client = new Client({ node: 'http://localhost:9200' })
const result = await client.search({
index: 'my-index',
body: { query: { match_all: {} } }
})
Both clients offer similar functionality, but elasticsearch-py follows Python conventions, while elasticsearch-js adheres to JavaScript patterns. The Python client uses a more object-oriented approach, whereas the JavaScript client leverages promises and async/await syntax for asynchronous operations.
This strongly-typed, client library enables working with Elasticsearch. It is the official client maintained and supported by Elastic.
Pros of elasticsearch-net
- Strongly-typed client for C# and .NET developers
- Supports both synchronous and asynchronous operations
- Comprehensive documentation and examples for .NET ecosystem
Cons of elasticsearch-net
- Limited to .NET environment, less versatile than JavaScript
- May have a steeper learning curve for developers not familiar with C#
- Potentially slower release cycle compared to the JavaScript client
Code Comparison
elasticsearch-net (C#):
var client = new ElasticClient();
var response = client.Search<Document>(s => s
.Query(q => q
.Match(m => m
.Field(f => f.Title)
.Query("elasticsearch")
)
)
);
elasticsearch-js (JavaScript):
const client = new Client({ node: 'http://localhost:9200' });
const response = await client.search({
index: 'my-index',
body: {
query: {
match: { title: 'elasticsearch' }
}
}
});
Both clients provide similar functionality, but elasticsearch-net offers a more strongly-typed approach with LINQ-like syntax, while elasticsearch-js uses a more flexible JSON-based query structure. The choice between them largely depends on the developer's preferred language and ecosystem.
Ruby integrations for Elasticsearch
Pros of elasticsearch-ruby
- Native Ruby implementation, providing better integration with Ruby ecosystem
- Supports Ruby-specific features and idioms
- Potentially easier to use for Ruby developers due to familiar syntax and conventions
Cons of elasticsearch-ruby
- Generally slower performance compared to the JavaScript client
- Smaller community and fewer resources available
- May have less frequent updates and feature additions
Code Comparison
elasticsearch-ruby:
client = Elasticsearch::Client.new
response = client.search(index: 'my_index', body: { query: { match: { title: 'search' } } })
puts response['hits']['hits']
elasticsearch-js:
const client = new Client({ node: 'http://localhost:9200' })
const response = await client.search({
index: 'my_index',
body: { query: { match: { title: 'search' } } }
})
console.log(response.hits.hits)
Both clients offer similar functionality for interacting with Elasticsearch, but the syntax and structure differ based on the language-specific conventions. The Ruby client uses a more object-oriented approach, while the JavaScript client leverages async/await for handling asynchronous operations.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
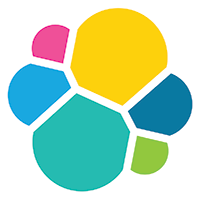
Elasticsearch Node.js client
Download the latest version of Elasticsearch or sign-up for a free trial of Elastic Cloud.
The official Node.js client for Elasticsearch.
Installation
Refer to the Installation section of the getting started documentation.
Connecting
Refer to the Connecting section of the getting started documentation.
Compatibility
The Elasticsearch client is compatible with currently maintained JS versions.
Language clients are forward compatible; meaning that clients support communicating with greater or equal minor versions of Elasticsearch without breaking. It does not mean that the client automatically supports new features of newer Elasticsearch versions; it is only possible after a release of a new client version. For example, a 8.12 client version won't automatically support the new features of the 8.13 version of Elasticsearch, the 8.13 client version is required for that. Elasticsearch language clients are only backwards compatible with default distributions and without guarantees made.
Elasticsearch Version | Elasticsearch-JS Branch |
---|---|
main | main |
9.x | 9.x |
8.x | 8.x |
7.x | 7.x |
Usage
- Creating an index
- Indexing a document
- Getting documents
- Searching documents
- Updating documents
- Deleting documents
- Deleting an index
Node.js support
NOTE: The minimum supported version of Node.js is v20
.
The client versioning follows the Elastic Stack versioning, this means that major, minor, and patch releases are done following a precise schedule that often does not coincide with the Node.js release times.
To avoid support insecure and unsupported versions of Node.js, the client will drop the support of EOL versions of Node.js between minor releases. Typically, as soon as a Node.js version goes into EOL, the client will continue to support that version for at least another minor release. If you are using the client with a version of Node.js that will be unsupported soon, you will see a warning in your logs (the client will start logging the warning with two minors in advance).
Unless you are always using a supported version of Node.js,
we recommend defining the client dependency in your
package.json
with the ~
instead of ^
. In this way, you will lock the
dependency on the minor release and not the major. (for example, ~7.10.0
instead
of ^7.10.0
).
Node.js Version | Node.js EOL date | End of support |
---|---|---|
8.x | December 2019 | 7.11 (early 2021) |
10.x | April 2021 | 7.12 (mid 2021) |
12.x | April 2022 | 8.2 (early 2022) |
14.x | April 2023 | 8.8 (early 2023) |
16.x | September 2023 | 8.11 (late 2023) |
18.x | April 2025 | 9.1 (mid 2025) |
Browser
[!WARNING] There is no official support for the browser environment. It exposes your Elasticsearch instance to everyone, which could lead to security issues. We recommend that you write a lightweight proxy that uses this client instead, you can see a proxy example here.
Documentation
- Introduction
- Usage
- Client configuration
- API reference
- Authentication
- Observability
- Creating a child client
- Client helpers
- Typescript support
- Testing
- Examples
Install multiple versions
If you are using multiple versions of Elasticsearch, you need to use multiple versions of the client. In the past, install multiple versions of the same package was not possible, but with npm v6.9
, you can do that via aliasing.
The command you must run to install different version of the client is:
npm install <alias>@npm:@elastic/elasticsearch@<version>
So for example if you need to install 7.x
and 6.x
, you will run:
npm install es6@npm:@elastic/elasticsearch@6
npm install es7@npm:@elastic/elasticsearch@7
And your package.json
will look like the following:
"dependencies": {
"es6": "npm:@elastic/elasticsearch@^6.7.0",
"es7": "npm:@elastic/elasticsearch@^7.0.0"
}
You will require the packages from your code by using the alias you have defined.
const { Client: Client6 } = require('es6')
const { Client: Client7 } = require('es7')
const client6 = new Client6({
cloud: { id: '<cloud-id>' },
auth: { apiKey: 'base64EncodedKey' }
})
const client7 = new Client7({
cloud: { id: '<cloud-id>' },
auth: { apiKey: 'base64EncodedKey' }
})
client6.info().then(console.log, console.log)
client7.info().then(console.log, console.log)
Finally, if you want to install the client for the next version of Elasticsearch (the one that lives in Elasticsearchâs main branch), you can use the following command:
npm install esmain@github:elastic/elasticsearch-js
License
This software is licensed under the Apache License 2.0.
Top Related Projects
Free and Open Source, Distributed, RESTful Search Engine
Deprecated: Use the official Elasticsearch client for Go at https://github.com/elastic/go-elasticsearch
The official Go client for Elasticsearch
Official Python client for Elasticsearch
This strongly-typed, client library enables working with Elasticsearch. It is the official client maintained and supported by Elastic.
Ruby integrations for Elasticsearch
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot