Top Related Projects
Reactive Programming in Swift
Elegant HTTP Networking in Swift
The better way to deal with JSON data in Swift.
Promises for Swift & ObjC.
Network abstraction layer written in Swift.
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
Quick Overview
Cosmos is an iOS library that provides a beautiful and customizable star rating control. It allows developers to easily add star ratings to their iOS applications with smooth animations and a high degree of customization.
Pros
- Easy to integrate and use in iOS projects
- Highly customizable, allowing for various star shapes, colors, and sizes
- Smooth animations for a polished user experience
- Written in Swift, making it compatible with modern iOS development practices
Cons
- Limited to iOS platform, not available for other mobile or web platforms
- Requires iOS 8.0 or later, which may exclude older devices
- Relatively small community and less frequent updates compared to some larger libraries
Code Examples
- Basic usage:
let cosmosView = CosmosView()
view.addSubview(cosmosView)
This code creates a default star rating view and adds it to the current view.
- Customizing appearance:
cosmosView.settings.starSize = 30
cosmosView.settings.fillMode = .precise
cosmosView.settings.filledColor = .orange
cosmosView.settings.emptyBorderColor = .gray
This example demonstrates how to customize the size, fill mode, and colors of the stars.
- Setting up a rating change callback:
cosmosView.didTouchCosmos = { rating in
print("Rating changed to: \(rating)")
}
This code sets up a closure that will be called whenever the user changes the rating.
Getting Started
To use Cosmos in your iOS project:
-
Add Cosmos to your project using CocoaPods, Carthage, or Swift Package Manager.
-
For CocoaPods, add the following to your Podfile:
pod 'Cosmos', '~> 23.0'
-
Import Cosmos in your Swift file:
import Cosmos
-
Create and customize a CosmosView:
let cosmosView = CosmosView() cosmosView.rating = 3.5 cosmosView.settings.updateOnTouch = true view.addSubview(cosmosView)
-
Position the view using Auto Layout or frames as needed in your UI.
Competitor Comparisons
Reactive Programming in Swift
Pros of RxSwift
- Comprehensive reactive programming framework for Swift
- Large community and extensive documentation
- Supports complex asynchronous operations and data streams
Cons of RxSwift
- Steeper learning curve for developers new to reactive programming
- Can be overkill for simpler projects or UI components
- Larger codebase and potential performance overhead
Code Comparison
Cosmos (Star rating control):
let cosmosView = CosmosView()
cosmosView.rating = 3.5
cosmosView.settings.fillMode = .half
RxSwift (Observable sequence):
Observable.of(1, 2, 3)
.map { $0 * 2 }
.subscribe(onNext: { print($0) })
.disposed(by: disposeBag)
Summary
Cosmos is a lightweight star rating control for iOS/tvOS, while RxSwift is a comprehensive reactive programming framework. Cosmos is easier to implement for simple rating functionality, whereas RxSwift offers powerful tools for managing complex asynchronous operations and data flows. The choice between the two depends on the project's scope and requirements.
Elegant HTTP Networking in Swift
Pros of Alamofire
- More comprehensive networking library with advanced features
- Larger community and ecosystem, with frequent updates
- Supports a wide range of networking tasks beyond HTTP requests
Cons of Alamofire
- Larger codebase and dependency footprint
- Steeper learning curve for beginners
- May be overkill for simple networking tasks
Code Comparison
Cosmos (Star rating view):
let starRating = CosmosView()
starRating.rating = 3.7
starRating.settings.fillMode = .precise
view.addSubview(starRating)
Alamofire (HTTP request):
AF.request("https://api.example.com/data")
.responseDecodable(of: ResponseType.self) { response in
switch response.result {
case .success(let value):
print("Response: \(value)")
case .failure(let error):
print("Error: \(error)")
}
}
Summary
Cosmos is a lightweight star rating control for iOS and tvOS, while Alamofire is a comprehensive networking library for iOS, macOS, tvOS, and watchOS. Cosmos is focused on a specific UI component, making it simpler to use for its intended purpose. Alamofire, on the other hand, offers a wide range of networking capabilities but may be more complex for simple tasks. The choice between the two depends on the specific needs of your project.
The better way to deal with JSON data in Swift.
Pros of SwiftyJSON
- Focused specifically on JSON parsing and manipulation
- More comprehensive JSON handling features
- Widely adopted and well-maintained
Cons of SwiftyJSON
- Limited to JSON processing only
- Larger codebase and potentially higher overhead
- May require more setup for basic JSON operations
Code Comparison
SwiftyJSON:
let json = JSON(data: dataFromNetworking)
if let name = json["user"]["name"].string {
// Do something with name
}
Cosmos:
// No direct JSON parsing functionality
// Cosmos is primarily for UI star rating
Additional Notes
Cosmos is primarily a UI component for star ratings in iOS apps, while SwiftyJSON is a JSON parsing library. They serve different purposes and are not directly comparable in terms of functionality.
Cosmos offers a simple, customizable star rating view for iOS applications, which can be useful for user feedback and ratings display. SwiftyJSON, on the other hand, provides robust JSON handling capabilities, making it easier to work with JSON data in Swift projects.
When choosing between these libraries, consider your specific project needs. If you require JSON parsing and manipulation, SwiftyJSON is the clear choice. For implementing star ratings in your UI, Cosmos would be more appropriate.
Promises for Swift & ObjC.
Pros of PromiseKit
- Focuses on asynchronous programming and simplifies complex async operations
- Extensive documentation and community support
- Supports a wide range of iOS and macOS versions
Cons of PromiseKit
- Larger library size and potential performance overhead
- Steeper learning curve for developers new to promises
Code Comparison
PromiseKit:
firstly {
fetchUser()
}.then { user in
fetchAvatar(for: user)
}.done { avatar in
self.imageView.image = avatar
}.catch { error in
print("Error: \(error)")
}
Cosmos:
cosmosView.rating = 3.7
cosmosView.settings.fillMode = .precise
cosmosView.didTouchCosmos = { rating in
print("Rating: \(rating)")
}
Summary
PromiseKit is a powerful library for handling asynchronous operations in Swift, offering extensive features and community support. However, it may introduce complexity and overhead for simpler projects.
Cosmos, on the other hand, is a lightweight star rating control for iOS and tvOS. It's easy to use and integrate but has a more specific focus compared to PromiseKit's broad async programming capabilities.
The choice between these libraries depends on the project's requirements. PromiseKit is ideal for complex async tasks, while Cosmos is perfect for implementing star ratings in user interfaces.
Network abstraction layer written in Swift.
Pros of Moya
- More comprehensive networking abstraction, providing a complete API layer
- Stronger type safety with enum-based API definitions
- Better support for testing and stubbing network requests
Cons of Moya
- Steeper learning curve due to its more complex architecture
- Potentially overkill for simpler networking needs
- Requires more setup and configuration compared to Cosmos
Code Comparison
Cosmos (HTTP GET request):
Cosmos.get("https://api.example.com/data") { result in
switch result {
case .success(let response):
print(response.text)
case .failure(let error):
print("Error: \(error)")
}
}
Moya (HTTP GET request):
provider.request(.getData) { result in
switch result {
case .success(let response):
print(response.data)
case .failure(let error):
print("Error: \(error)")
}
}
Both libraries offer simple ways to make network requests, but Moya requires more upfront setup with its provider and target system. Cosmos provides a more straightforward API for quick networking tasks, while Moya offers a more structured approach for larger projects with complex networking needs.
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
Pros of ReactiveCocoa
- Comprehensive reactive programming framework for iOS and macOS
- Supports functional reactive programming paradigms
- Large community and extensive documentation
Cons of ReactiveCocoa
- Steeper learning curve for developers new to reactive programming
- Can introduce complexity in simpler applications
- Requires careful management to avoid memory leaks
Code Comparison
ReactiveCocoa:
let searchResults = searchText
.throttle(0.3, on: QueueScheduler.main)
.flatMap(.latest) { (query: String) -> SignalProducer<[SearchResult], NoError> in
return API.search(query)
}
Cosmos:
cosmosView.rating = 3.7
cosmosView.settings.fillMode = .half
cosmosView.didTouchCosmos = { rating in
print("Rating: \(rating)")
}
Summary
ReactiveCocoa is a powerful reactive programming framework, offering extensive functionality for complex iOS and macOS applications. It excels in handling asynchronous operations and data flows but may be overkill for simpler projects. Cosmos, on the other hand, is a focused star rating control library, providing an easy-to-use solution for implementing ratings in iOS apps. While ReactiveCocoa offers more flexibility and power, Cosmos is more straightforward for its specific use case.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Cosmos, a star rating control for iOS and tvOS

This is a UI control for iOS and tvOS written in Swift. It shows a star rating and takes rating input from the user. Cosmos is a subclass of a UIView that will allow your users to post those inescapable 1-star reviews!
- Shows star rating with an optional text label.
- Can be used as a rating input control (iOS only).
- Cosmos view can be customized in the Storyboard without writing code.
- Includes different star filling modes: full, half-filled and precise.
- Cosmos is accessible and works with voice-over.
- Supports right-to-left languages.
Picture of binary star system of Sirius A and Sirius B by NASA, ESA and G. Bacon (STScI). Source: spacetelescope.org.
Video tutorial
Thanks to Alex Nagy from rebeloper.com for creating this amazing video tutorial that shows how to use and customize Cosmos from code.
Setup
There are various ways you can add Cosmos to your Xcode project.
Add source (iOS 8+)
Simply add CosmosDistrib.swift file into your Xcode project.
Setup with Carthage (iOS 8+)
Alternatively, add github "evgenyneu/Cosmos" ~> 25.0
to your Cartfile and run carthage update
.
Setup with CocoaPods (iOS 8+)
If you are using CocoaPods add this text to your Podfile and run pod install
.
use_frameworks!
target 'Your target name'
pod 'Cosmos', '~> 25.0'
Setup with Swift Package Manager
- In Xcode 11+ select File > Packages > Add Package Dependency....
- Enter this project's URL: https://github.com/evgenyneu/Cosmos.git
Legacy Swift versions
Setup a previous version of the library if you use an older version of Swift.
Usage
- Drag
View
object from the Object Library into your storyboard.
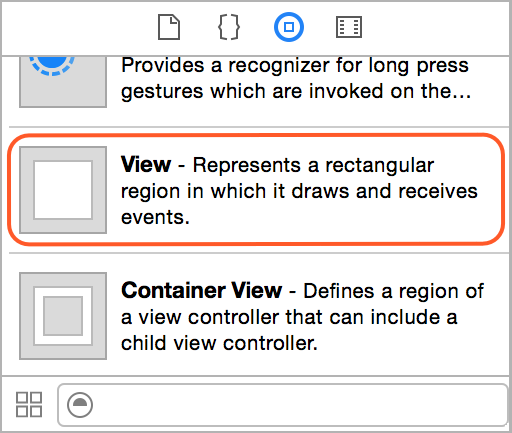
- Set the view's class to
CosmosView
in the Identity Inspector. Set its module property toCosmos
, unless you used the file setup method.
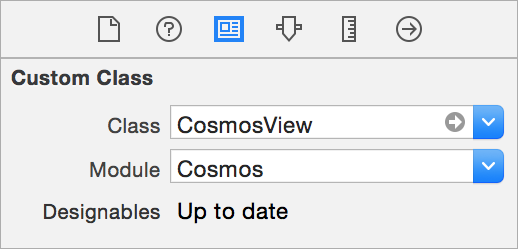
tvOS note: read the collowing setup instructions for tvOS if you see build errors at this stage.
- Customize the Cosmos view appearance in the Attributes Inspector. If storyboard does not show the stars click Refresh All Views from the Editor menu.

Positioning the Cosmos view
One can position the Cosmos view using Auto Layout constaints. The width and height of the view is determined automatically based on the size of its content - stars and text. Therefore, there is no need to set width/height constaints on the Cosmos view.
Using Cosmos in code
Add import Cosmos
to your source code, unless you used the file setup method.
You can style and control Cosmos view from your code by creating an outlet in your view controller. Alternatively, one can instantiate CosmosView
class and add it to the view manually without using Storyboard.
// Change the cosmos view rating
cosmosView.rating = 4
// Change the text
cosmosView.text = "(123)"
// Called when user finishes changing the rating by lifting the finger from the view.
// This may be a good place to save the rating in the database or send to the server.
cosmosView.didFinishTouchingCosmos = { rating in }
// A closure that is called when user changes the rating by touching the view.
// This can be used to update UI as the rating is being changed by moving a finger.
cosmosView.didTouchCosmos = { rating in }
Customization
One can customize Cosmos from code by changing its settings
. See the Cosmos configuration manual for the complete list of configuration options.
// Do not change rating when touched
// Use if you need just to show the stars without getting user's input
cosmosView.settings.updateOnTouch = false
// Show only fully filled stars
cosmosView.settings.fillMode = .full
// Other fill modes: .half, .precise
// Change the size of the stars
cosmosView.settings.starSize = 30
// Set the distance between stars
cosmosView.settings.starMargin = 5
// Set the color of a filled star
cosmosView.settings.filledColor = UIColor.orange
// Set the border color of an empty star
cosmosView.settings.emptyBorderColor = UIColor.orange
// Set the border color of a filled star
cosmosView.settings.filledBorderColor = UIColor.orange
Supplying images for the stars
By default, Cosmos draws the stars from an array of points. Alternatively, one can supply custom images for the stars, both in the Storyboard and from code.
Using star images from the Storyboard

Using star images from code
// Set image for the filled star
cosmosView.settings.filledImage = UIImage(named: "GoldStarFilled")
// Set image for the empty star
cosmosView.settings.emptyImage = UIImage(named: "GoldStarEmpty")
Note: you need to have the images for the filled and empty star in your project for this code to work.
Download star image files
Images for the golden star used in the demo app are available in here. Contributions for other star images are very welcome: add vector images to /graphics/Stars/
directory and submit a pull request.
Using Cosmos in a scroll/table view
Here is how to use Cosmos in a scroll view or a table view.
Using Cosmos with SwiftUI
Here is how to show a Cosmos view with SwiftUI.
Using Cosmos in a modal screen
iOS 13 introduced swiping gesture for closing modal screens, which prevents Cosmos from working properly. The following setting fixes this problem:
cosmosView.settings.disablePanGestures = true
Using Cosmos settings from Objective-C
This manual describes how to set/read Cosmos settings in Objective-C apps.
Demo app
This project includes a demo iOS app.
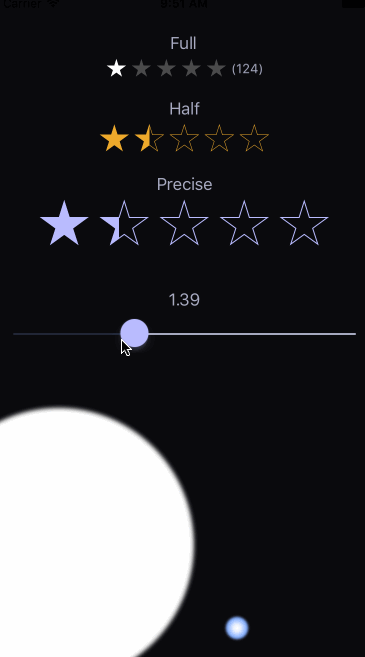
Using cosmos in a table view
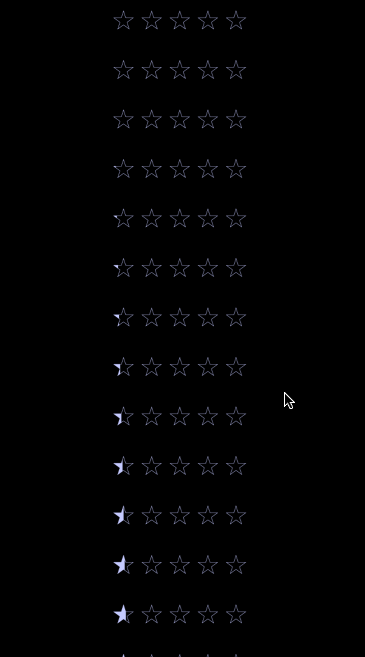
Alternative solutions
Here are some other star rating controls for iOS:
- danwilliams64/DJWStarRatingView
- dlinsin/DLStarRating
- dyang/DYRateView
- erndev/EDStarRating
- hugocampossousa/HCSStarRatingView
- shuhrat10/STRatingControl
- strekfus/FloatRatingView
- yanguango/ASStarRatingView
Thanks ð
We would like to thank the following people for their valuable contributions.
- jsahoo for adding ability to customize the Cosmos view from the interface builder with Carthage setup method.
- 0x7fffffff for changing
public
access-level modifiers toopen
. - ali-zahedi for updating to the latest version of Swift 3.0.
- augmentedworks for adding borders to filled stars.
- craiggrummitt for Xcode 8 beta 4 support.
- JimiSmith for Xcode 8 beta 6 support.
- nickhart for adding compatibility with Xcode 6.
- staticdreams for bringing tvOS support.
- wagnersouz4 for Swift 3.1 update.
- paoloq for reporting the CosmoView frame size issue when the view is used without Auto Layout.
- danshevluk for adding ability to reuse settings in multiple cosmos views.
- xrayman for reporting a table view reusability bug and improving the table view screen of the demo app.
- chlumik for updating to Swift 4.2.
- rebeloper for creating a video tutorial.
- yuravake for adding
passTouchesToSuperview
setting. - gcharita for adding Swift Package Manager support.
- benpackard for fixing Cosmos when used in a modal screen on iOS 13.
- dkk for the dark mode update.
License
Cosmos is released under the MIT License.
ðâï¸ððð
We are a way for the cosmos to know itself.
Carl Sagan, from 1980 "Cosmos: A Personal Voyage" TV series.
Top Related Projects
Reactive Programming in Swift
Elegant HTTP Networking in Swift
The better way to deal with JSON data in Swift.
Promises for Swift & ObjC.
Network abstraction layer written in Swift.
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot