Top Related Projects
Detectron2 is a platform for object detection, segmentation and other visual recognition tasks.
Mask R-CNN for object detection and instance segmentation on Keras and TensorFlow
OpenMMLab Detection Toolbox and Benchmark
FAIR's research platform for object detection research, implementing popular algorithms like Mask R-CNN and RetinaNet.
Models and examples built with TensorFlow
PyTorch3D is FAIR's library of reusable components for deep learning with 3D data
Quick Overview
Mesh R-CNN is a deep learning framework developed by Facebook Research for 3D object reconstruction from 2D images. It extends Mask R-CNN to predict 3D meshes of objects in addition to 2D instance segmentation, combining 2D and 3D computer vision techniques.
Pros
- Integrates 2D instance segmentation with 3D mesh prediction
- Achieves state-of-the-art results in 3D object reconstruction
- Built on top of the popular Detectron2 framework
- Provides pre-trained models for easy use
Cons
- Requires significant computational resources for training and inference
- Limited to object classes present in the training dataset
- May struggle with complex or occluded objects
- Requires 3D ground truth data for training, which can be difficult to obtain
Code Examples
- Loading a pre-trained Mesh R-CNN model:
from detectron2.config import get_cfg
from meshrcnn import add_meshrcnn_config
from meshrcnn.predictor import MeshRCNNPredictor
cfg = get_cfg()
add_meshrcnn_config(cfg)
cfg.merge_from_file("meshrcnn_R50_FPN.yaml")
cfg.MODEL.WEIGHTS = "meshrcnn_R50.pth"
predictor = MeshRCNNPredictor(cfg)
- Performing inference on an image:
import cv2
image = cv2.imread("input_image.jpg")
outputs = predictor(image)
meshes = outputs["instances"].pred_meshes
- Visualizing the predicted 3D mesh:
from meshrcnn.utils import visualize_prediction
visualize_prediction(image, outputs)
Getting Started
To get started with Mesh R-CNN:
-
Clone the repository:
git clone https://github.com/facebookresearch/meshrcnn.git cd meshrcnn
-
Install dependencies:
pip install -r requirements.txt
-
Download pre-trained models:
wget https://dl.fbaipublicfiles.com/meshrcnn/pix3d/meshrcnn_R50_FPN.pth
-
Run inference on an image:
from meshrcnn.predictor import MeshRCNNPredictor predictor = MeshRCNNPredictor.from_pretrained("meshrcnn_R50_FPN.pth") outputs = predictor("path/to/image.jpg")
Competitor Comparisons
Detectron2 is a platform for object detection, segmentation and other visual recognition tasks.
Pros of Detectron2
- More comprehensive and versatile, supporting a wider range of computer vision tasks
- Better documentation and community support
- Regularly updated with new features and improvements
Cons of Detectron2
- Steeper learning curve due to its broader scope
- May be overkill for projects focused solely on 3D mesh reconstruction
Code Comparison
Detectron2:
from detectron2.engine import DefaultPredictor
from detectron2.config import get_cfg
cfg = get_cfg()
cfg.merge_from_file("path/to/config.yaml")
predictor = DefaultPredictor(cfg)
outputs = predictor(image)
MeshRCNN:
from meshrcnn.modeling.roi_heads.mesh_head import MeshRCNNHead
from meshrcnn.modeling.roi_heads.roi_heads import ROIHeads
mesh_head = MeshRCNNHead(cfg)
roi_heads = ROIHeads(cfg, mesh_head)
outputs = roi_heads(features, proposals)
Summary
Detectron2 is a more general-purpose computer vision library, while MeshRCNN focuses specifically on 3D mesh reconstruction. Detectron2 offers broader functionality and better support, but MeshRCNN may be more suitable for projects solely focused on 3D reconstruction tasks. The code examples demonstrate the different approaches to model initialization and prediction in each library.
Mask R-CNN for object detection and instance segmentation on Keras and TensorFlow
Pros of Mask_RCNN
- More widely adopted and community-supported
- Easier to set up and use for beginners
- Extensive documentation and tutorials available
Cons of Mask_RCNN
- Less advanced in 3D reconstruction capabilities
- May not perform as well on complex 3D scenes
- Limited to 2D instance segmentation tasks
Code Comparison
Mask_RCNN:
import mrcnn.model as modellib
model = modellib.MaskRCNN(mode="inference", config=config, model_dir=MODEL_DIR)
model.load_weights(COCO_MODEL_PATH, by_name=True)
results = model.detect([image], verbose=1)
MeshRCNN:
from meshrcnn.modeling.roi_heads.mesh_head import MeshRCNNHead
mesh_head = MeshRCNNHead(cfg)
mesh_outputs = mesh_head(features, instances)
meshes = mesh_outputs["meshes"]
The code snippets highlight the difference in focus between the two projects. Mask_RCNN is primarily designed for 2D instance segmentation, while MeshRCNN extends the functionality to 3D mesh reconstruction. MeshRCNN builds upon Mask_RCNN's architecture but adds specialized components for 3D tasks.
OpenMMLab Detection Toolbox and Benchmark
Pros of mmdetection
- Broader scope: Supports a wide range of object detection algorithms and tasks
- More active development: Frequent updates and contributions from the community
- Extensive documentation and tutorials for easier adoption
Cons of mmdetection
- Steeper learning curve due to its comprehensive nature
- May be overkill for projects focused solely on 3D mesh reconstruction
Code Comparison
mmdetection:
from mmdet.apis import init_detector, inference_detector
config_file = 'configs/faster_rcnn/faster_rcnn_r50_fpn_1x_coco.py'
checkpoint_file = 'checkpoints/faster_rcnn_r50_fpn_1x_coco_20200130-047c8118.pth'
model = init_detector(config_file, checkpoint_file, device='cuda:0')
result = inference_detector(model, 'test.jpg')
meshrcnn:
from detectron2.config import get_cfg
from meshrcnn import add_meshrcnn_config
cfg = get_cfg()
add_meshrcnn_config(cfg)
cfg.merge_from_file("configs/meshrcnn_R50_FPN.yaml")
predictor = DefaultPredictor(cfg)
outputs = predictor(image)
FAIR's research platform for object detection research, implementing popular algorithms like Mask R-CNN and RetinaNet.
Pros of Detectron
- More comprehensive object detection framework with multiple models
- Better documentation and community support
- Wider range of applications beyond 3D reconstruction
Cons of Detectron
- Lacks specific focus on 3D mesh reconstruction
- May require more setup and configuration for specialized tasks
- Potentially higher computational requirements for general use
Code Comparison
MeshRCNN:
cfg = get_cfg()
cfg.merge_from_file("configs/pix3d/meshrcnn_R50_FPN.yaml")
predictor = DefaultPredictor(cfg)
outputs = predictor(image)
Detectron:
cfg = get_cfg()
cfg.merge_from_file("configs/COCO-InstanceSegmentation/mask_rcnn_R_50_FPN_3x.yaml")
predictor = DefaultPredictor(cfg)
outputs = predictor(image)
Key Differences
- MeshRCNN focuses on 3D mesh reconstruction from single images
- Detectron offers a broader range of object detection and instance segmentation models
- MeshRCNN provides specialized tools for 3D shape analysis
- Detectron has more extensive pre-trained models and datasets
Use Cases
MeshRCNN is ideal for:
- 3D object reconstruction from 2D images
- Applications requiring detailed 3D shape analysis
Detectron is better suited for:
- General object detection and instance segmentation tasks
- Projects requiring a variety of detection models and architectures
Models and examples built with TensorFlow
Pros of models
- Broader scope with implementations of various ML models and architectures
- More extensive documentation and tutorials for beginners
- Larger community and more frequent updates
Cons of models
- Can be overwhelming due to its large size and diverse content
- May require more setup and configuration for specific tasks
- Potentially slower execution for certain models compared to specialized implementations
Code comparison
meshrcnn:
cfg = get_cfg()
cfg.merge_from_file(model_zoo.get_config_file("COCO-InstanceSegmentation/mask_rcnn_R_50_FPN_3x.yaml"))
cfg.MODEL.ROI_HEADS.SCORE_THRESH_TEST = 0.5
cfg.MODEL.WEIGHTS = model_zoo.get_checkpoint_url("COCO-InstanceSegmentation/mask_rcnn_R_50_FPN_3x.yaml")
models:
model = tf.keras.applications.MobileNetV2(input_shape=(224, 224, 3),
include_top=False,
weights='imagenet')
model.trainable = False
model = tf.keras.Sequential([
model,
tf.keras.layers.GlobalAveragePooling2D()
])
PyTorch3D is FAIR's library of reusable components for deep learning with 3D data
Pros of PyTorch3D
- More comprehensive 3D deep learning library with a wider range of functionalities
- Actively maintained with regular updates and improvements
- Better documentation and examples for easier adoption
Cons of PyTorch3D
- Steeper learning curve due to its broader scope
- May have higher computational requirements for some operations
Code Comparison
MeshRCNN (mesh reconstruction):
from meshrcnn.structures import Meshes
from meshrcnn.utils import mesh_ops
vertices, faces = mesh_ops.subdivide(vertices, faces)
mesh = Meshes(verts=[vertices], faces=[faces])
PyTorch3D (similar functionality):
from pytorch3d.structures import Meshes
from pytorch3d.ops import subdivide_meshes
mesh = Meshes(verts=[vertices], faces=[faces])
subdivided_mesh = subdivide_meshes(mesh, number_of_subdivisions=1)
Both libraries provide mesh manipulation capabilities, but PyTorch3D offers a more extensive set of operations and is designed for broader 3D deep learning tasks. MeshRCNN is more focused on mesh reconstruction from images, while PyTorch3D can be used for various 3D computer vision and graphics tasks.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Mesh R-CNN
Code for the paper
Mesh R-CNN
Georgia Gkioxari, Jitendra Malik, Justin Johnson
ICCV 2019
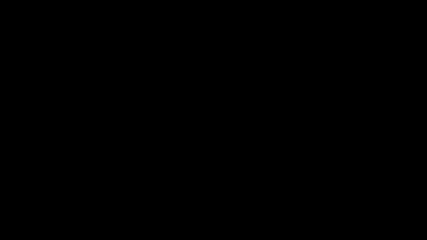
(thanks to Alberto Tono!)
Installation Requirements
The implementation of Mesh R-CNN is based on Detectron2 and PyTorch3D. You will first need to install those in order to be able to run Mesh R-CNN.
To install
git clone https://github.com/facebookresearch/meshrcnn.git
cd meshrcnn && pip install -e .
Demo
Run Mesh R-CNN on an input image
python demo/demo.py \
--config-file configs/pix3d/meshrcnn_R50_FPN.yaml \
--input /path/to/image \
--output output_demo \
--onlyhighest MODEL.WEIGHTS meshrcnn://meshrcnn_R50.pth
See demo.py for more details.
Running Experiments
Pix3D
See INSTRUCTIONS_PIX3D.md for more instructions.
ShapeNet
See INSTRUCTIONS_SHAPENET.md for more instructions.
License
The Mesh R-CNN codebase is released under BSD-3-Clause License
Top Related Projects
Detectron2 is a platform for object detection, segmentation and other visual recognition tasks.
Mask R-CNN for object detection and instance segmentation on Keras and TensorFlow
OpenMMLab Detection Toolbox and Benchmark
FAIR's research platform for object detection research, implementing popular algorithms like Mask R-CNN and RetinaNet.
Models and examples built with TensorFlow
PyTorch3D is FAIR's library of reusable components for deep learning with 3D data
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot