Top Related Projects
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
Parse Server for Node.js / Express
The flexible backend for all your projects π° Turn your DB into a headless CMS, admin panels, or apps with a custom UI, instant APIs, auth & more.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Quick Overview
Feathers is a lightweight web-framework for creating real-time applications and REST APIs using JavaScript or TypeScript. It's built on top of Express and provides a modular architecture with a powerful plugin system, making it easy to build scalable and maintainable applications.
Pros
- Flexible and modular architecture allowing easy customization and extension
- Built-in support for real-time functionality using WebSockets
- Supports multiple databases and ORMs out of the box
- Lightweight and performant, with minimal overhead
Cons
- Learning curve can be steep for developers new to its concepts
- Documentation can be overwhelming due to the number of plugins and options
- Smaller community compared to some other popular frameworks
- Less suitable for large, complex applications that require more opinionated structures
Code Examples
- Creating a basic Feathers application:
const feathers = require('@feathersjs/feathers');
const express = require('@feathersjs/express');
const app = express(feathers());
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.listen(3030);
- Defining a simple service:
class MessageService {
async find(params) {
return [{ id: 1, text: 'Hello, Feathers!' }];
}
}
app.use('messages', new MessageService());
- Using hooks for authentication:
const { authenticate } = require('@feathersjs/authentication').hooks;
app.service('messages').hooks({
before: {
all: [authenticate('jwt')]
}
});
Getting Started
To get started with Feathers, follow these steps:
- Install Feathers CLI:
npm install -g @feathersjs/cli
- Create a new Feathers project:
feathers generate app
- Start the development server:
npm start
This will create a new Feathers application with a basic structure and start the development server. You can then begin adding services, hooks, and other features to your application.
Competitor Comparisons
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
Pros of Nest
- Strong TypeScript support with decorators and dependency injection
- Modular architecture promoting scalability and maintainability
- Extensive ecosystem with built-in support for various technologies
Cons of Nest
- Steeper learning curve due to its opinionated structure
- Heavier framework with more boilerplate code
- Potentially slower startup time for smaller applications
Code Comparison
Nest:
@Controller('users')
export class UsersController {
@Get()
findAll(): string {
return 'This action returns all users';
}
}
Feathers:
app.use('users', {
async find() {
return 'This action returns all users';
}
});
Key Differences
- Nest uses decorators and classes for defining routes and services
- Feathers employs a more functional approach with hooks and services
- Nest provides a more structured, Angular-like development experience
- Feathers offers a lighter, more flexible setup suitable for rapid development
Use Cases
- Nest: Large-scale enterprise applications with complex architectures
- Feathers: Real-time applications and microservices with quick setup requirements
Both frameworks are powerful and well-maintained, with active communities. The choice between them depends on project requirements, team expertise, and development preferences.
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
Pros of Strapi
- User-friendly admin panel for content management
- Built-in authentication and user management system
- Extensive plugin ecosystem for easy customization
Cons of Strapi
- Less flexible for custom backend logic compared to Feathers
- Heavier resource usage due to its full-featured nature
- Steeper learning curve for developers new to headless CMS
Code Comparison
Strapi (Content Type definition):
module.exports = {
attributes: {
title: {
type: 'string',
required: true
},
content: {
type: 'richtext'
}
}
};
Feathers (Service definition):
const { Service } = require('feathers-nedb');
class PostsService extends Service {
constructor(options) {
super(options);
}
}
module.exports = function(app) {
app.use('/posts', new PostsService());
};
Strapi focuses on content modeling and management, while Feathers provides a more flexible approach for building custom APIs. Strapi's code revolves around defining content types and their attributes, whereas Feathers emphasizes service-oriented architecture for data operations. Strapi is ideal for content-heavy applications with admin requirements, while Feathers excels in building lightweight, customizable APIs for various use cases.
Parse Server for Node.js / Express
Pros of Parse Server
- More comprehensive out-of-the-box features, including user authentication, file storage, and push notifications
- Established ecosystem with a wide range of plugins and integrations
- Familiar API for developers transitioning from the original Parse platform
Cons of Parse Server
- Steeper learning curve due to its more complex architecture
- Less flexibility in customizing the core functionality
- Heavier resource usage, which may impact performance for smaller applications
Code Comparison
Parse Server:
const express = require('express');
const ParseServer = require('parse-server').ParseServer;
const api = new ParseServer({
databaseURI: 'mongodb://localhost:27017/dev',
appId: 'myAppId',
masterKey: 'myMasterKey',
serverURL: 'http://localhost:1337/parse'
});
const app = express();
app.use('/parse', api);
Feathers:
const feathers = require('@feathersjs/feathers');
const express = require('@feathersjs/express');
const app = express(feathers());
app.use('/messages', {
async find() {
return [{ id: 1, text: 'Hello' }];
}
});
app.listen(3030);
Both Parse Server and Feathers are powerful backend frameworks, but they cater to different needs. Parse Server offers a more complete solution with built-in features, while Feathers provides a lightweight and flexible approach for building real-time applications. The choice between them depends on the specific requirements of your project and your preferred development style.
The flexible backend for all your projects π° Turn your DB into a headless CMS, admin panels, or apps with a custom UI, instant APIs, auth & more.
Pros of Directus
- Provides a complete headless CMS solution with a user-friendly admin interface
- Offers automatic REST and GraphQL APIs for data management
- Includes built-in user authentication and role-based access control
Cons of Directus
- Less flexible for custom application development compared to Feathers
- Steeper learning curve for developers who need to extend core functionality
- More opinionated architecture, which may limit certain customization options
Code Comparison
Directus (API endpoint definition):
module.exports = {
id: 'custom',
handler: (router, { services, exceptions }) => {
router.get('/', (req, res) => {
res.send('Hello World!');
});
}
};
Feathers (Service creation):
const { Service } = require('@feathersjs/feathers');
class MyService extends Service {
async find(params) {
return { message: 'Hello World!' };
}
}
Both Directus and Feathers are powerful tools for building APIs and backend systems. Directus excels as a complete headless CMS solution with a user-friendly interface, while Feathers offers more flexibility for custom application development. The choice between them depends on project requirements and developer preferences.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Pros of AdonisJS
- More opinionated framework with a robust set of built-in features
- Strong TypeScript support out of the box
- Comprehensive documentation and active community
Cons of AdonisJS
- Steeper learning curve for beginners
- Less flexibility compared to Feathers' modular approach
- Heavier framework with more overhead
Code Comparison
AdonisJS route definition:
Route.get('/users', 'UsersController.index')
Route.post('/users', 'UsersController.store')
Feathers service setup:
app.use('/users', new UserService())
app.service('users').hooks({
before: { create: [validateUser] }
})
AdonisJS focuses on a more traditional MVC structure with controllers, while Feathers emphasizes services and hooks for a more functional approach. AdonisJS provides a more structured environment with built-in features like routing and ORM, whereas Feathers offers greater flexibility and a lighter footprint.
Both frameworks have their strengths, with AdonisJS excelling in full-featured applications and Feathers shining in real-time, service-oriented architectures. The choice between them depends on project requirements, team expertise, and desired development style.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
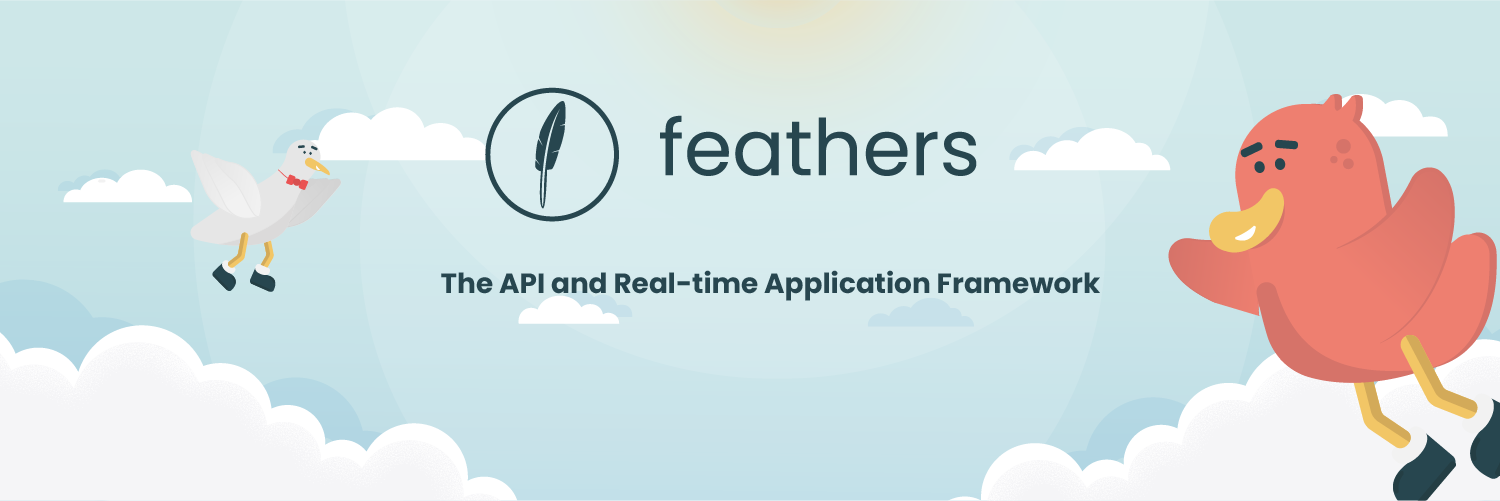
Feathers is a full-stack framework for creating web APIs and real-time applications with TypeScript or JavaScript.
Feathers can interact with any backend technology, supports many databases out of the box and works with any frontend like React, VueJS, Angular, React Native, Android or iOS.
Getting started
Get started with just three commands:
$ npm create feathers my-new-app
$ cd my-new-app
$ npm run dev
To learn more about Feathers visit the website at feathersjs.com or jump right into the Feathers guides.
Contributing
To start developing, clone this repository, then run:
cd feathers
npm install
To run all tests run
npm test
Individual tests can be run in the module you are working on:
cd packages/feathers
npm test
License
Copyright (c) 2024 Feathers contributors
Licensed under the MIT license.
Top Related Projects
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
Parse Server for Node.js / Express
The flexible backend for all your projects π° Turn your DB into a headless CMS, admin panels, or apps with a custom UI, instant APIs, auth & more.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot