Top Related Projects
Point Cloud Library (PCL)
Open Source Computer Vision Library
Cross-platform, customizable ML solutions for live and streaming media.
Intel® RealSense™ SDK
A GPU-accelerated library containing highly optimized building blocks and an execution engine for data processing to accelerate deep learning training and inference applications.
Quick Overview
Open3D is an open-source library that supports rapid development of software dealing with 3D data. It provides an extensive set of capabilities for 3D data processing, including I/O, visualization, and algorithms for various 3D geometry operations. Open3D is designed to be efficient, easy to use, and well-integrated with popular numerical libraries like NumPy.
Pros
- Comprehensive set of 3D data processing tools and algorithms
- Efficient implementation with C++ core and Python bindings
- Excellent documentation and examples
- Active community and regular updates
Cons
- Steep learning curve for beginners in 3D processing
- Limited support for some specialized 3D formats
- Can be resource-intensive for large datasets
- Some advanced features may require additional dependencies
Code Examples
Loading and visualizing a point cloud:
import open3d as o3d
# Read point cloud
pcd = o3d.io.read_point_cloud("example.pcd")
# Visualize the point cloud
o3d.visualization.draw_geometries([pcd])
Performing ICP (Iterative Closest Point) registration:
import open3d as o3d
import numpy as np
def draw_registration_result(source, target, transformation):
source_temp = source.clone()
target_temp = target.clone()
source_temp.paint_uniform_color([1, 0.706, 0])
target_temp.paint_uniform_color([0, 0.651, 0.929])
source_temp.transform(transformation)
o3d.visualization.draw_geometries([source_temp, target_temp])
source = o3d.io.read_point_cloud("source.pcd")
target = o3d.io.read_point_cloud("target.pcd")
threshold = 0.02
trans_init = np.asarray([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]])
reg_p2p = o3d.pipelines.registration.registration_icp(
source, target, threshold, trans_init,
o3d.pipelines.registration.TransformationEstimationPointToPoint())
print(reg_p2p)
draw_registration_result(source, target, reg_p2p.transformation)
Creating and manipulating a triangle mesh:
import open3d as o3d
import numpy as np
# Create a triangle mesh
vertices = np.array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]])
triangles = np.array([[0, 1, 2], [0, 1, 3], [0, 2, 3], [1, 2, 3]])
mesh = o3d.geometry.TriangleMesh()
mesh.vertices = o3d.utility.Vector3dVector(vertices)
mesh.triangles = o3d.utility.Vector3iVector(triangles)
# Compute normals and visualize
mesh.compute_vertex_normals()
o3d.visualization.draw_geometries([mesh])
Getting Started
-
Install Open3D:
pip install open3d
-
Import the library in your Python script:
import open3d as o3d
-
Start using Open3D functions, e.g., to read and visualize a point cloud:
pcd = o3d.io.read_point_cloud("example.pcd") o3d.visualization.draw_geometries([pcd])
For more detailed instructions and examples, refer to the official Open3D documentation at http://www.open3d.org/docs/release/.
Competitor Comparisons
Point Cloud Library (PCL)
Pros of PCL
- More mature and established library with a larger community
- Extensive documentation and tutorials available
- Wider range of algorithms and features for point cloud processing
Cons of PCL
- Steeper learning curve due to its complexity
- Slower compilation times and larger binary sizes
- Less focus on modern C++ practices and GPU acceleration
Code Comparison
PCL:
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
#include <pcl/filters/voxel_grid.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::VoxelGrid<pcl::PointXYZ> voxel_grid;
voxel_grid.setInputCloud(cloud);
voxel_grid.setLeafSize(0.01f, 0.01f, 0.01f);
voxel_grid.filter(*cloud);
Open3D:
import open3d as o3d
pcd = o3d.io.read_point_cloud("input.ply")
downpcd = pcd.voxel_down_sample(voxel_size=0.01)
o3d.visualization.draw_geometries([downpcd])
The code comparison demonstrates that Open3D offers a more concise and user-friendly API, especially for Python users, while PCL provides a more detailed C++ interface with finer control over operations.
Open Source Computer Vision Library
Pros of OpenCV
- Broader scope: Covers a wide range of computer vision tasks, including 2D image processing
- Larger community and ecosystem: More resources, tutorials, and third-party integrations
- Multi-language support: Bindings for Python, C++, Java, and more
Cons of OpenCV
- Less specialized for 3D processing: Fewer tools for point cloud manipulation and 3D reconstruction
- Steeper learning curve: Due to its extensive feature set, it can be more challenging for beginners
- Heavier resource usage: Generally requires more system resources compared to Open3D
Code Comparison
Open3D (Point cloud visualization):
import open3d as o3d
pcd = o3d.io.read_point_cloud("example.ply")
o3d.visualization.draw_geometries([pcd])
OpenCV (Image display):
import cv2
img = cv2.imread("example.jpg")
cv2.imshow("Image", img)
cv2.waitKey(0)
Both libraries offer powerful tools for their respective domains. Open3D excels in 3D processing and visualization, while OpenCV provides a comprehensive suite for general computer vision tasks. The choice between them depends on the specific requirements of your project.
Cross-platform, customizable ML solutions for live and streaming media.
Pros of MediaPipe
- Specialized for real-time machine learning applications on mobile and edge devices
- Extensive support for various ML tasks like face detection, pose estimation, and hand tracking
- Cross-platform compatibility (iOS, Android, web)
Cons of MediaPipe
- Less focused on 3D data processing and visualization
- May have a steeper learning curve for non-ML-specific tasks
- Limited support for point cloud operations compared to Open3D
Code Comparison
MediaPipe (Python):
import mediapipe as mp
mp_hands = mp.solutions.hands
hands = mp_hands.Hands()
results = hands.process(image)
if results.multi_hand_landmarks:
# Process hand landmarks
Open3D (Python):
import open3d as o3d
pcd = o3d.io.read_point_cloud("example.pcd")
downpcd = pcd.voxel_down_sample(voxel_size=0.05)
o3d.visualization.draw_geometries([downpcd])
MediaPipe focuses on ML-based perception tasks, while Open3D specializes in 3D data processing and visualization. MediaPipe is better suited for real-time applications on mobile devices, whereas Open3D excels in point cloud operations and 3D geometry manipulation.
Intel® RealSense™ SDK
Pros of librealsense
- Specialized for Intel RealSense devices, offering optimized performance and features
- Extensive documentation and examples for Intel RealSense hardware
- Active development and support from Intel
Cons of librealsense
- Limited to Intel RealSense devices, lacking support for other 3D sensors
- Narrower scope compared to Open3D's broader 3D data processing capabilities
- Less focus on high-level 3D processing algorithms and visualization tools
Code Comparison
librealsense example:
rs2::pipeline pipe;
pipe.start();
rs2::frameset frames = pipe.wait_for_frames();
rs2::depth_frame depth = frames.get_depth_frame();
Open3D example:
import open3d as o3d
pcd = o3d.io.read_point_cloud("example.pcd")
o3d.visualization.draw_geometries([pcd])
While librealsense focuses on device-specific data acquisition, Open3D provides a more general-purpose toolkit for 3D data processing and visualization across various sources and formats.
A GPU-accelerated library containing highly optimized building blocks and an execution engine for data processing to accelerate deep learning training and inference applications.
Pros of DALI
- Optimized for GPU acceleration, providing faster data processing for deep learning workflows
- Specialized in image, video, and audio processing pipelines
- Seamless integration with NVIDIA GPU ecosystem and deep learning frameworks
Cons of DALI
- Limited to NVIDIA GPUs, reducing portability across different hardware
- Narrower focus on data loading and preprocessing, less versatile for general 3D tasks
- Steeper learning curve for users not familiar with NVIDIA's ecosystem
Code Comparison
DALI (image processing pipeline):
import nvidia.dali as dali
@dali.pipeline_def
def image_pipeline():
images = dali.fn.readers.file(file_root="path/to/images")
images = dali.fn.decoders.image(images, device="mixed")
images = dali.fn.resize(images, resize_x=224, resize_y=224)
return images
Open3D (3D point cloud processing):
import open3d as o3d
pcd = o3d.io.read_point_cloud("path/to/pointcloud.ply")
downpcd = pcd.voxel_down_sample(voxel_size=0.05)
o3d.visualization.draw_geometries([downpcd])
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Open3D: A Modern Library for 3D Data Processing
Homepage | Docs | Quick Start | Compile | Python | C++ | Open3D-ML | Viewer | Contribute | Demo | Forum
Open3D is an open-source library that supports rapid development of software that deals with 3D data. The Open3D frontend exposes a set of carefully selected data structures and algorithms in both C++ and Python. The backend is highly optimized and is set up for parallelization. We welcome contributions from the open-source community.
Core features of Open3D include:
- 3D data structures
- 3D data processing algorithms
- Scene reconstruction
- Surface alignment
- 3D visualization
- Physically based rendering (PBR)
- 3D machine learning support with PyTorch and TensorFlow
- GPU acceleration for core 3D operations
- Available in C++ and Python
Here's a brief overview of the different components of Open3D and how they fit together to enable full end to end pipelines:
For more, please visit the Open3D documentation.
Python quick start
Pre-built pip packages support Ubuntu 20.04+, macOS 10.15+ and Windows 10+ (64-bit) with Python 3.8-3.11.
# Install
pip install open3d # or
pip install open3d-cpu # Smaller CPU only wheel on x86_64 Linux (v0.17+)
# Verify installation
python -c "import open3d as o3d; print(o3d.__version__)"
# Python API
python -c "import open3d as o3d; \
mesh = o3d.geometry.TriangleMesh.create_sphere(); \
mesh.compute_vertex_normals(); \
o3d.visualization.draw(mesh, raw_mode=True)"
# Open3D CLI
open3d example visualization/draw
To get the latest features in Open3D, install the development pip package. To compile Open3D from source, refer to compiling from source.
C++ quick start
Checkout the following links to get started with Open3D C++ API
- Download Open3D binary package: Release or latest development version
- Compiling Open3D from source
- Open3D C++ API
To use Open3D in your C++ project, checkout the following examples
Open3D-Viewer app
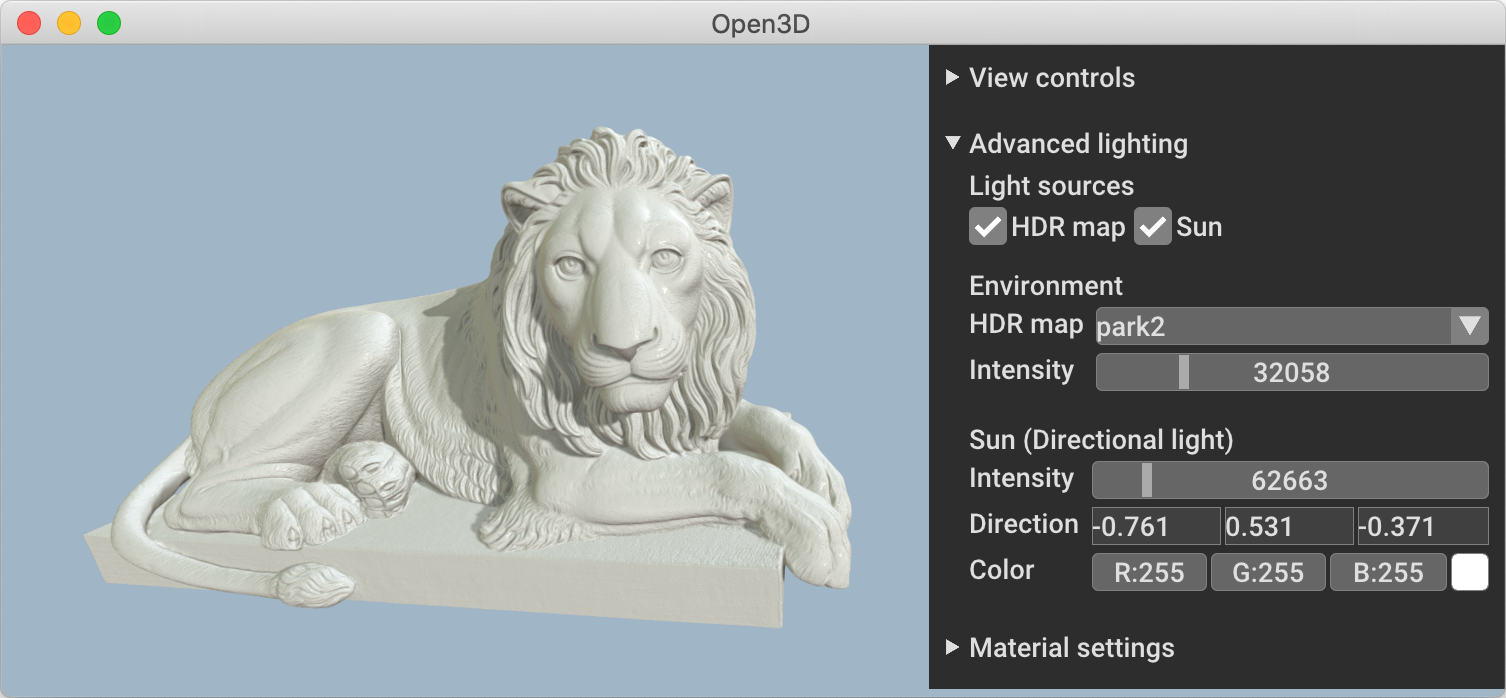
Open3D-Viewer is a standalone 3D viewer app available on Debian (Ubuntu), macOS and Windows. Download Open3D Viewer from the release page.
Open3D-ML
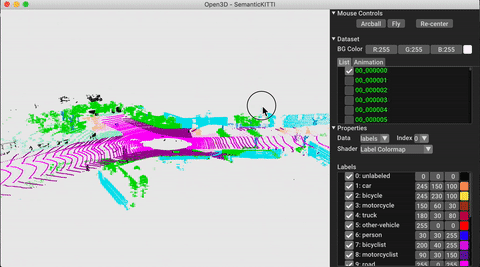
Open3D-ML is an extension of Open3D for 3D machine learning tasks. It builds on top of the Open3D core library and extends it with machine learning tools for 3D data processing. To try it out, install Open3D with PyTorch or TensorFlow and check out Open3D-ML.
Communication channels
- GitHub Issue: bug reports, feature requests, etc.
- Forum: discussion on the usage of Open3D.
- Discord Chat: online chats, discussions, and collaboration with other users and developers.
Citation
Please cite our work if you use Open3D.
@article{Zhou2018,
author = {Qian-Yi Zhou and Jaesik Park and Vladlen Koltun},
title = {{Open3D}: {A} Modern Library for {3D} Data Processing},
journal = {arXiv:1801.09847},
year = {2018},
}
Top Related Projects
Point Cloud Library (PCL)
Open Source Computer Vision Library
Cross-platform, customizable ML solutions for live and streaming media.
Intel® RealSense™ SDK
A GPU-accelerated library containing highly optimized building blocks and an execution engine for data processing to accelerate deep learning training and inference applications.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot