react-native-modals
A react native modals library. Swipeable. Highly customizable. Support multi modals & Support custom animation. For IOS & Android.
Top Related Projects
An enhanced, animated, customizable Modal for React Native.
A <Modal/> component for react-native
Standard set of easy to use animations and declarative transitions for React Native
Quick Overview
react-native-modals is a customizable and flexible modal component library for React Native applications. It provides a range of modal types and animations, allowing developers to create engaging and interactive user interfaces with ease.
Pros
- Highly customizable with various modal types and animation options
- Easy to integrate and use in React Native projects
- Supports both iOS and Android platforms
- Actively maintained with regular updates and bug fixes
Cons
- May have a slight learning curve for developers new to React Native
- Some advanced customizations might require deeper understanding of the library
- Limited documentation for certain edge cases or complex scenarios
- Potential performance impact with heavy use of animations on older devices
Code Examples
- Basic Modal Usage:
import Modal from 'react-native-modals';
<Modal
visible={this.state.visible}
onTouchOutside={() => this.setState({ visible: false })}
>
<ModalContent>
<Text>Hello World!</Text>
</ModalContent>
</Modal>
- Slide Animation Modal:
import Modal, { SlideAnimation, ModalContent } from 'react-native-modals';
<Modal
visible={this.state.visible}
modalAnimation={new SlideAnimation({
slideFrom: 'bottom',
})}
>
<ModalContent>
<Text>Sliding Modal Content</Text>
</ModalContent>
</Modal>
- Custom Footer Modal:
import Modal, { ModalFooter, ModalButton, ModalContent } from 'react-native-modals';
<Modal
visible={this.state.visible}
footer={
<ModalFooter>
<ModalButton
text="CANCEL"
onPress={() => this.setState({ visible: false })}
/>
<ModalButton
text="OK"
onPress={() => this.setState({ visible: false })}
/>
</ModalFooter>
}
>
<ModalContent>
<Text>Modal with Custom Footer</Text>
</ModalContent>
</Modal>
Getting Started
-
Install the package:
npm install react-native-modals
-
Import and use in your React Native component:
import Modal, { ModalContent } from 'react-native-modals'; function MyComponent() { const [visible, setVisible] = useState(false); return ( <> <Button title="Show Modal" onPress={() => setVisible(true)} /> <Modal visible={visible} onTouchOutside={() => setVisible(false)} > <ModalContent> <Text>Your modal content here</Text> </ModalContent> </Modal> </> ); }
-
Customize as needed using the various props and components provided by the library.
Competitor Comparisons
An enhanced, animated, customizable Modal for React Native.
Pros of react-native-modal
- More actively maintained with frequent updates
- Larger community and better documentation
- Supports custom animations and transitions out of the box
Cons of react-native-modal
- Slightly more complex API for basic use cases
- May require additional configuration for certain advanced features
Code Comparison
react-native-modal:
<Modal isVisible={isModalVisible} onBackdropPress={toggleModal}>
<View style={styles.modalContent}>
<Text>Hello!</Text>
<Button title="Hide modal" onPress={toggleModal} />
</View>
</Modal>
react-native-modals:
<Modal.BottomModal
visible={isModalVisible}
onTouchOutside={toggleModal}
height={0.5}
width={1}
onSwipeOut={toggleModal}
>
<ModalContent>
<Text>Hello!</Text>
<Button title="Hide modal" onPress={toggleModal} />
</ModalContent>
</Modal.BottomModal>
Both libraries offer similar functionality, but react-native-modal provides a more straightforward API for basic modal implementations. react-native-modals offers more specific modal types (e.g., BottomModal) out of the box, which can be beneficial for certain use cases.
react-native-modal is generally recommended for most projects due to its active maintenance and larger community support. However, react-native-modals may be preferred if you need specific modal types or a slightly different API structure.
A <Modal/> component for react-native
Pros of react-native-modalbox
- Simpler API with fewer configuration options, making it easier to use for basic modal needs
- Lightweight and focused solely on modal functionality
- Supports swipe-to-close gesture out of the box
Cons of react-native-modalbox
- Less customizable compared to react-native-modals
- Fewer animation options and less control over modal behavior
- Not actively maintained (last update was in 2019)
Code Comparison
react-native-modalbox:
<Modal style={styles.modal} position={"center"} isOpen={this.state.isOpen}>
<Text>Basic modal</Text>
</Modal>
react-native-modals:
<Modal
visible={this.state.visible}
onTouchOutside={() => this.setState({ visible: false })}
>
<ModalContent>
<Text>Basic modal</Text>
</ModalContent>
</Modal>
Both libraries offer a straightforward way to create modals, but react-native-modals provides more granular control over modal behavior and appearance. react-native-modalbox has a simpler API, which can be beneficial for basic use cases. However, for more complex modal requirements, react-native-modals offers greater flexibility and customization options.
Standard set of easy to use animations and declarative transitions for React Native
Pros of react-native-animatable
- More comprehensive animation library with a wide range of pre-defined animations
- Supports chaining and sequencing of animations for complex effects
- Lightweight and easy to integrate into existing React Native projects
Cons of react-native-animatable
- Focused solely on animations, lacks modal-specific features
- May require additional setup for creating custom modal-like components
- Less specialized for modal use cases compared to react-native-modals
Code Comparison
react-native-animatable:
import * as Animatable from 'react-native-animatable';
<Animatable.View animation="fadeIn" duration={1000}>
<Text>Animated content</Text>
</Animatable.View>
react-native-modals:
import Modal from 'react-native-modals';
<Modal visible={isVisible} onTouchOutside={() => setIsVisible(false)}>
<ModalContent>
<Text>Modal content</Text>
</ModalContent>
</Modal>
react-native-animatable offers a more versatile animation system suitable for various UI elements, while react-native-modals provides a specialized solution for creating and managing modal dialogs in React Native applications. The choice between the two depends on the specific requirements of your project, with react-native-animatable being better for general animations and react-native-modals excelling in modal-specific functionality.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
React Native Modals
React Native Modals Library for iOS & Android.
How to thank me ?
Just click on âï¸ button ð
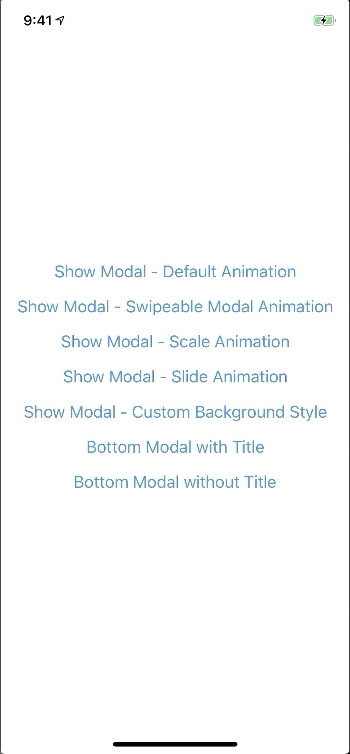
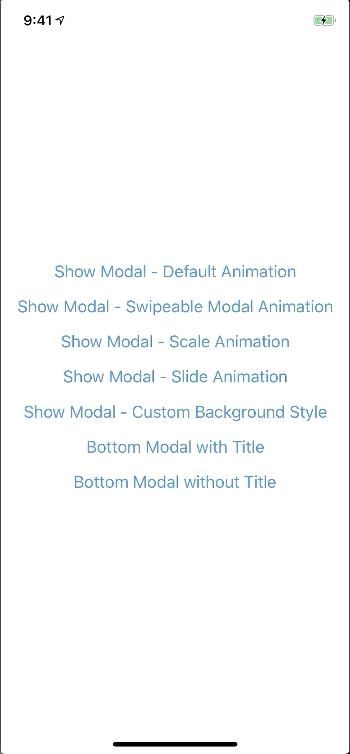
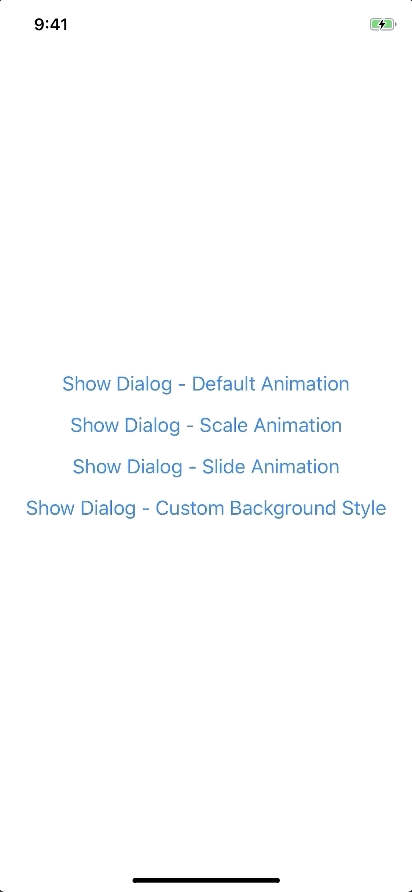
BREAKING CHANGE
A lot of backward incompatible changes in v0.22.0
. Please, Read the Docs before upgrading to v0.22.0
Installation
npm install --save react-native-modals
# OR
yarn add react-native-modals
Exposed Modules
- Modal
- ButtomModal
- ModalPortal
- Backdrop
- ModalButton
- ModalContent
- ModalTitle
- ModalFooter
- Animation
- FadeAnimation
- ScaleAnimation
- SlideAnimation
- DragEvent,
- SwipeDirection,
- ModalProps
- ModalFooterProps
- ModalButtonProps
- ModalTitleProps
- ModalContentProps
- BackdropProps
Examples
Setup - this is essential step
The Component can not be used until ModalPortal
is mounted.
You should register in your app root. For example:
import { ModalPortal } from 'react-native-modals';
import { Provider } from 'react-redux';
const Root = () => {
return (
<Provider store={store}>
<App />
<ModalPortal />
</Provider>
);
}
Basic Usage
import { Modal, ModalContent } from 'react-native-modals';
import { Button } from 'react-native'
<View style={styles.container}>
<Button
title="Show Modal"
onPress={() => {
this.setState({ visible: true });
}}
/>
<Modal
visible={this.state.visible}
onTouchOutside={() => {
this.setState({ visible: false });
}}
>
<ModalContent>
{...}
</ModalContent>
</Modal>
</View>
Usage - Imperative APIs
show
import { ModalPortal } from 'react-native-modals';
const id = ModalPortal.show((
<View>
{...}
</View>
));
update
ModalPortal.update(id, {
children: (
<View>
<Text>Updated</Text>
</View>
),
});
dismiss
ModalPortal.dismiss(id);
dismissAll
ModalPortal.dismissAll(id);
Usage - Animation
import { Modal, SlideAnimation, ModalContent } from 'react-native-modals';
<View style={styles.container}>
<Modal
visible={this.state.visible}
modalAnimation={new SlideAnimation({
slideFrom: 'bottom',
})}
>
<ModalContent>
{...}
</ModalContent>
</Modal>
</View>
Usage - Swipe
import { Modal, ModalContent } from 'react-native-modals';
import { Button } from 'react-native'
<View style={styles.container}>
<Modal
visible={this.state.visible}
swipeDirection={['up', 'down']} // can be string or an array
swipeThreshold={200} // default 100
onSwipeOut={(event) => {
this.setState({ visible: false });
}}
>
<ModalContent>
{...}
</ModalContent>
</Modal>
</View>
Usage - Modal Title
import { Modal, ModalTitle, ModalContent } from 'react-native-modals';
<View style={styles.container}>
<Modal
visible={this.state.visible}
modalTitle={<ModalTitle title="Modal Title" />}
>
<ModalContent>
{...}
</ModalContent>
</Modal>
</View>
Usage - Modal Action
import { Modal, ModalFooter, ModalButton, ModalContent } from 'react-native-modals';
<View style={styles.container}>
<Modal
visible={this.state.visible}
footer={
<ModalFooter>
<ModalButton
text="CANCEL"
onPress={() => {}}
/>
<ModalButton
text="OK"
onPress={() => {}}
/>
</ModalFooter>
}
>
<ModalContent>
{...}
</ModalContent>
</Modal>
</View>
Props
Modal
Prop | Type | Default | Note |
---|---|---|---|
visible | boolean | false | |
rounded | boolean | true | |
useNativeDriver | boolean | true | |
children | any | ||
modalTitle? | React Element | You can pass a modalTitle component or pass a View for customizing titlebar | |
width? | Number | Your device width | The Width of modal, you can use fixed width or use percentage. For example 0.5 it means 50% |
height? | Number | 300 | The Height of modal, you can use fixed height or use percentage. For example 0.5 it means 50% |
modalAnimation? | FadeAnimation | animation for modal | |
modalStyle? | any | ||
containerStyle? | any | null | For example: { zIndex: 10, elevation: 10 } |
animationDuration? | Number | 200 | |
overlayPointerEvents? | String | Available option: auto , none | |
overlayBackgroundColor? | String | #000 | |
overlayOpacity? | Number | 0.5 | |
hasOverlay? | Boolean | true | |
onShow? | Function | You can pass shown function as a callback function, will call the function when modal shown | |
onDismiss? | Function | You can pass onDismiss function as a callback function, will call the function when modal dismissed | |
onTouchOutside? | Function | () => {} | |
onHardwareBackPress? | Function | () => true | Handle hardware button presses |
onMove? | Function | () => {} | |
onSwiping? | Function | () => {} | |
onSwipeRelease? | Function | () => {} | |
onSwipingOut? | Function | () => {} | |
onSwipeOut? | Function | ||
swipeDirection? | string or Array<string> | [] | Available option: up , down , left , right |
swipeThreshold? | number | 100 | |
footer? | React Element | null | for example: <View><Button text="DISMISS" align="center" onPress={() => {}}/></View> |
ModalTitle
Prop | Type | Default | Note |
---|---|---|---|
title | String | ||
style? | any | null | |
textStyle? | any | null | |
align? | String | center | Available option: left , center , right |
hasTitleBar? | Bool | true |
ModalContent
Prop | Type | Default | Note |
---|---|---|---|
children | any | ||
style? | any | null |
ModalFooter
Prop | Type | Default | Note |
---|---|---|---|
children | ModalButton | ||
bordered? | Boolean | true | |
style? | any | null |
ModalButton
Prop | Type | Default | Note |
---|---|---|---|
text | String | ||
onPress | Function | ||
align? | String | center | Available option: left , center , right |
style? | any | null | |
textStyle? | any | null | |
activeOpacity? | Number | 0.6 | |
disabled? | Boolean | false | |
bordered? | Boolean | false |
Backdrop
Prop | Type | Default | Note |
---|---|---|---|
visible | Boolean | ||
opacity | Number | 0.5 | |
onPress? | Function | ||
backgroundColor? | string | #000 | |
animationDuration? | Number | 200 | |
pointerEvents? | String | null | Available option: auto , none |
useNativeDriver? | Boolean | true |
Animation
Params for (*)Animation
FadeAnimation
Preview:

Example:
new FadeAnimation({
initialValue: 0, // optional
animationDuration: 150, // optional
useNativeDriver: true, // optional
})
Param | Type | Default | Note |
---|---|---|---|
initialValue | Number | 0 | |
animationDuration? | Number | 150 | |
useNativeDriver? | Boolean | true |
ScaleAnimation
Preview:

Example:
new ScaleAnimation({
initialValue: 0, // optional
useNativeDriver: true, // optional
})
Param | Type | Default | Note |
---|---|---|---|
initialValue | Number | 0 | |
useNativeDriver | Boolean | true |
SlideAnimation
Preview:
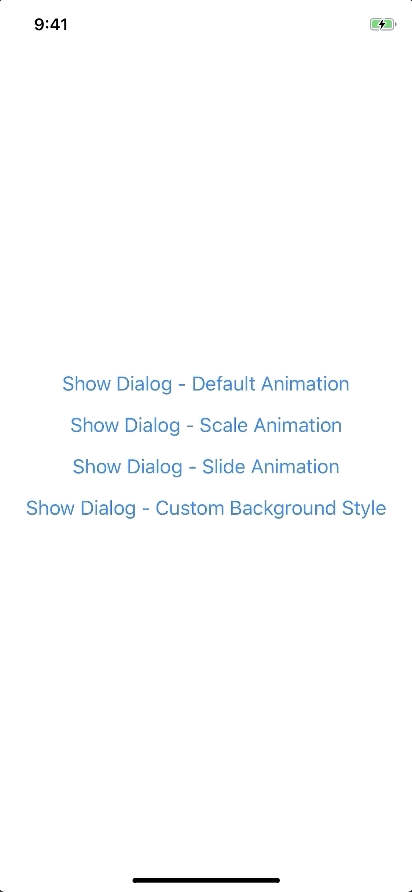
Example:
new SlideAnimation({
initialValue: 0, // optional
slideFrom: 'bottom', // optional
useNativeDriver: true, // optional
})
Param | Type | Default | Note |
---|---|---|---|
initialValue | Number | 0 | |
slideFrom | String | bottom | Available option: top , bottom , left , right |
useNativeDriver | Boolean | true |
Create your custom animation
Example:
import { Animated } from 'react-native';
import { Animation } from 'react-native-modals';
class CustomAnimation extends Animation {
in(onFinished) {
Animated.spring(this.animate, {
toValue: 1,
useNativeDriver: this.useNativeDriver,
}).start(onFinished);
}
out(onFinished) {
Animated.spring(this.animate, {
toValue: 0,
useNativeDriver: this.useNativeDriver,
}).start(onFinished);
}
getAnimations() {
return {
transform: [{
translateY: this.animate.interpolate({
inputRange: [0, 1],
outputRange: [800, 1],
}),
}],
};
}
}
Development
yarn
yarn run build
yarn test
Top Related Projects
An enhanced, animated, customizable Modal for React Native.
A <Modal/> component for react-native
Standard set of easy to use animations and declarative transitions for React Native
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot