Top Related Projects
An enhanced, animated, customizable Modal for React Native.
A react native modals library. Swipeable. Highly customizable. Support multi modals & Support custom animation. For IOS & Android.
Standard set of easy to use animations and declarative transitions for React Native
React Native Cross-Platform WebView
Quick Overview
React Native Modalbox is a customizable modal component for React Native applications. It provides a flexible and easy-to-use solution for creating modals with various animations and styles, enhancing the user interface of mobile apps.
Pros
- Highly customizable with numerous props for styling and behavior
- Supports multiple animation types for opening and closing
- Compatible with both iOS and Android platforms
- Lightweight and easy to integrate into existing projects
Cons
- Limited documentation and examples for advanced use cases
- Some reported issues with performance on older devices
- Lack of recent updates and maintenance (as of the last check)
- May require additional customization for complex modal layouts
Code Examples
- Basic Modal Usage:
import Modal from 'react-native-modalbox';
<Modal isOpen={this.state.isOpen} onClosed={() => this.setState({isOpen: false})}>
<Text>Basic Modal</Text>
</Modal>
- Customized Modal with Animation:
<Modal
style={styles.modal}
position={"center"}
backdrop={true}
entry={"slide"}
swipeToClose={false}
backdropPressToClose={true}
onClosed={() => this.setState({isOpen: false})}
>
<Text>Customized Modal</Text>
</Modal>
- Modal with Custom Styles:
<Modal
style={[styles.modal, styles.modal3]}
position={"center"}
backdrop={false}
coverScreen={true}
>
<Text style={styles.text}>Modal with custom styles</Text>
</Modal>
Getting Started
-
Install the package:
npm install react-native-modalbox
-
Import the Modal component in your React Native file:
import Modal from 'react-native-modalbox';
-
Use the Modal component in your render method:
render() { return ( <View> <Modal isOpen={this.state.isModalOpen} onClosed={() => this.setState({isModalOpen: false})}> <Text>Your modal content here</Text> </Modal> </View> ); }
-
Open the modal by setting the
isOpen
prop totrue
:this.setState({isModalOpen: true});
Competitor Comparisons
An enhanced, animated, customizable Modal for React Native.
Pros of react-native-modal
- More actively maintained with frequent updates
- Extensive customization options and props
- Better TypeScript support
Cons of react-native-modal
- Slightly more complex API
- Larger package size
Code Comparison
react-native-modal:
import Modal from "react-native-modal";
<Modal isVisible={isModalVisible} onBackdropPress={toggleModal}>
<View style={styles.modalContent}>
<Text>Hello!</Text>
</View>
</Modal>
react-native-modalbox:
import Modal from 'react-native-modalbox';
<Modal isOpen={isModalVisible} onClosed={toggleModal}>
<View style={styles.modalContent}>
<Text>Hello!</Text>
</View>
</Modal>
Both libraries offer similar basic functionality, but react-native-modal provides more advanced features and customization options. It has better community support and documentation, making it easier to implement complex modal behaviors. However, react-native-modalbox is simpler to use for basic modal needs and has a smaller footprint.
The choice between the two depends on the project requirements. For more advanced modal functionality and ongoing support, react-native-modal is recommended. For simpler use cases or projects with size constraints, react-native-modalbox might be sufficient.
A react native modals library. Swipeable. Highly customizable. Support multi modals & Support custom animation. For IOS & Android.
Pros of react-native-modals
- More comprehensive set of modal types (e.g., bottom modal, slide animation)
- Better TypeScript support and type definitions
- More active development and maintenance
Cons of react-native-modals
- Slightly more complex API due to additional features
- Larger package size due to more components and animations
Code Comparison
react-native-modals:
import Modal from 'react-native-modals';
<Modal
visible={this.state.visible}
onTouchOutside={() => this.setState({ visible: false })}
>
<ModalContent>
<Text>Hello World!</Text>
</ModalContent>
</Modal>
react-native-modalbox:
import Modal from 'react-native-modalbox';
<Modal isOpen={this.state.isOpen} onClosed={() => this.setState({isOpen: false})}>
<Text>Hello World!</Text>
</Modal>
Both libraries offer similar basic functionality, but react-native-modals provides more customization options and a wider range of modal types. react-native-modalbox has a simpler API and smaller package size, which may be preferable for projects with basic modal requirements. The choice between the two depends on the specific needs of your project, such as the variety of modal styles required and the importance of TypeScript support.
Standard set of easy to use animations and declarative transitions for React Native
Pros of react-native-animatable
- More versatile, offering a wide range of animations for various UI elements
- Supports custom animations and easing functions
- Larger community and more frequent updates
Cons of react-native-animatable
- Steeper learning curve due to more complex API
- May require more code for simple animations
- Not specifically designed for modal components
Code Comparison
react-native-animatable:
import * as Animatable from 'react-native-animatable';
<Animatable.View animation="fadeIn" duration={1000}>
<Text>Animated content</Text>
</Animatable.View>
react-native-modalbox:
import Modal from 'react-native-modalbox';
<Modal isOpen={this.state.isOpen} onClosed={() => this.setState({isOpen: false})}>
<Text>Modal content</Text>
</Modal>
react-native-animatable provides a more general-purpose animation solution, allowing developers to animate various components with different effects. It offers greater flexibility but may require more setup for specific use cases like modals.
react-native-modalbox, on the other hand, is specifically designed for creating modal components. It provides a simpler API for implementing modals but lacks the extensive animation capabilities of react-native-animatable.
Choose react-native-animatable for diverse animation needs across your app, or opt for react-native-modalbox if you primarily need a straightforward modal solution with basic animations.
React Native Cross-Platform WebView
Pros of react-native-webview
- Specifically designed for rendering web content within React Native apps
- Offers more advanced web-related features like JavaScript injection and navigation control
- Actively maintained with regular updates and a large community
Cons of react-native-webview
- Limited to web content display, not suitable for creating custom modal interfaces
- May have performance overhead when used for simple content display
- Requires additional setup and configuration for basic modal-like functionality
Code Comparison
react-native-webview:
import { WebView } from 'react-native-webview';
<WebView
source={{ uri: 'https://example.com' }}
onLoad={() => console.log('Loaded')}
/>
react-native-modalbox:
import Modal from 'react-native-modalbox';
<Modal isOpen={this.state.isOpen} onClosed={() => this.setState({isOpen: false})}>
<Text>Modal content</Text>
</Modal>
react-native-webview is focused on rendering web content within React Native apps, offering advanced web-related features. It's ideal for displaying web pages or HTML content but may be overkill for simple modal interfaces. react-native-modalbox, on the other hand, is specifically designed for creating customizable modal dialogs with native performance. It's more suitable for creating app-specific modal interfaces but lacks web content rendering capabilities.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
react-native-modalbox
A react native
Wanna implement IAP in your beautiful modal? ð
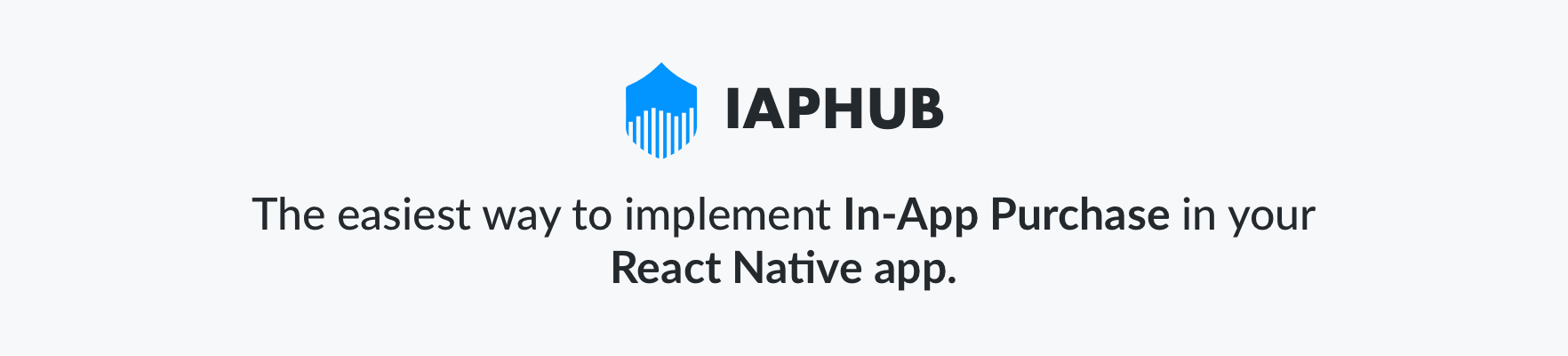
Preview
Install
npm install react-native-modalbox@latest --save
Example
Check index.js in the Example folder.
Version note
react-native | react-native-modalbox |
---|---|
<= 0.57 | <= 1.6.0 |
>= 0.58 | >= 1.6.1 |
Properties
Prop | Default | Type | Description |
---|---|---|---|
isOpen | false | bool | Open/close the modal, optional, you can use the open/close methods instead |
isDisabled | false | bool | Disable any action on the modal (open, close, swipe) |
backdropPressToClose | true | bool | Close the the modal by pressing on the backdrop |
swipeToClose | true | bool | Set to true to enable the swipe down to close feature |
swipeThreshold | 50 | number | The threshold to reach in pixels to close the modal |
swipeArea | - | number | The height in pixels of the swipeable area, window height by default |
position | center | string | Control the modal position using top or center or bottom |
entry | bottom | string | Control the modal entry position top or bottom |
backdrop | true | bool | Display a backdrop behind the modal |
backdropOpacity | 0.5 | number | Opacity of the backdrop |
backdropColor | black | string | backgroundColor of the backdrop |
backdropContent | null | ReactElement | Add an element in the backdrop (a close button for example) |
animationDuration | 400 | number | Duration of the animation |
easing | Easing.elastic(0.8) | function | Easing function applied to opening modal animation |
backButtonClose | false | bool | (Android only) Close modal when receiving back button event |
startOpen | false | bool | Allow modal to appear open without animation upon first mount |
coverScreen | false | bool | Will use RN Modal component to cover the entire screen wherever the modal is mounted in the component hierarchy |
keyboardTopOffset | ios:22, android:0 | number | This property prevent the modal to cover the ios status bar when the modal is scrolling up because the keyboard is opening |
useNativeDriver | true | bool | Enables the hardware acceleration to animate the modal. Please note that enabling this can cause some flashes in a weird way when animating |
Events
Prop | Params | Description |
---|---|---|
onClosed | - | When the modal is close and the animation is done |
onOpened | - | When the modal is open and the animation is done |
onClosingState | state bool | When the state of the swipe to close feature has changed (usefull to change the content of the modal, display a message for example) |
Methods
These methods are optional, you can use the isOpen property instead
Prop | Params | Description |
---|---|---|
open | - | Open the modal |
close | - | Close the modal |
Top Related Projects
An enhanced, animated, customizable Modal for React Native.
A react native modals library. Swipeable. Highly customizable. Support multi modals & Support custom animation. For IOS & Android.
Standard set of easy to use animations and declarative transitions for React Native
React Native Cross-Platform WebView
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot