Top Related Projects
Asynchronous image downloader with cache support as a UIImageView category
A lightweight, pure-Swift library for downloading and caching images from the web.
AlamofireImage is an image component library for Alamofire
Promises for Swift & ObjC.
Reactive Programming in Swift
Network abstraction layer written in Swift.
Quick Overview
Nuke is a powerful image loading and caching system for iOS and macOS applications. It provides an efficient and easy-to-use solution for downloading, processing, and displaying images from various sources, including network URLs and local storage.
Pros
- High performance and memory efficiency
- Extensive customization options for image processing and caching
- Supports progressive image loading and animated GIFs
- Well-documented and actively maintained
Cons
- Limited to Apple platforms (iOS, macOS, tvOS, watchOS)
- Steeper learning curve compared to simpler image loading libraries
- May be overkill for small projects with basic image loading needs
Code Examples
Loading an image from a URL:
import Nuke
let imageView = UIImageView()
let url = URL(string: "https://example.com/image.jpg")!
Nuke.loadImage(with: url, into: imageView)
Applying image processing:
import Nuke
let processor = ImageProcessors.Resize(width: 320)
let request = ImageRequest(url: url, processors: [processor])
Nuke.loadImage(with: request, into: imageView)
Preheating images:
import Nuke
let urls = [URL(string: "https://example.com/image1.jpg")!,
URL(string: "https://example.com/image2.jpg")!]
Nuke.ImagePipeline.shared.prefetch(with: urls)
Getting Started
-
Add Nuke to your project using Swift Package Manager, CocoaPods, or Carthage.
-
Import Nuke in your Swift file:
import Nuke
- Load an image into an image view:
let imageView = UIImageView()
let url = URL(string: "https://example.com/image.jpg")!
Nuke.loadImage(with: url, into: imageView)
- Customize the image pipeline (optional):
let pipeline = ImagePipeline {
$0.dataLoader = DataLoader(configuration: {
$0.urlCache = URLCache(memoryCapacity: 100_000_000, diskCapacity: 1_000_000_000)
})
$0.imageCache = ImageCache.shared
}
ImagePipeline.shared = pipeline
For more advanced usage and configuration options, refer to the official documentation.
Competitor Comparisons
Asynchronous image downloader with cache support as a UIImageView category
Pros of SDWebImage
- Wider platform support (iOS, tvOS, macOS, watchOS)
- More comprehensive feature set, including animated image support
- Larger community and longer history, potentially leading to better stability
Cons of SDWebImage
- Larger codebase, which may lead to increased app size
- Potentially more complex API due to its extensive feature set
- Slower adoption of newer Swift features and patterns
Code Comparison
SDWebImage:
let imageView = UIImageView()
imageView.sd_setImage(with: URL(string: "https://example.com/image.jpg"))
Nuke:
let imageView = UIImageView()
Nuke.loadImage(with: URL(string: "https://example.com/image.jpg")!, into: imageView)
Both libraries offer simple one-line methods for loading images into views. SDWebImage uses a category on UIImageView, while Nuke uses a static method. The syntax is slightly different, but the functionality is similar.
SDWebImage provides a more extensive set of features and wider platform support, making it suitable for complex projects with diverse requirements. Nuke, on the other hand, offers a more focused and lightweight solution, which may be preferable for simpler projects or those prioritizing a smaller codebase and faster adoption of modern Swift practices.
A lightweight, pure-Swift library for downloading and caching images from the web.
Pros of Kingfisher
- More extensive feature set, including image processing and filters
- Better support for GIF animations and WebP format
- More active community and frequent updates
Cons of Kingfisher
- Slightly larger library size, which may impact app size
- Can be more complex to set up and use for simple image loading tasks
Code Comparison
Kingfisher:
let url = URL(string: "https://example.com/image.jpg")
imageView.kf.setImage(with: url)
Nuke:
let url = URL(string: "https://example.com/image.jpg")
Nuke.loadImage(with: url, into: imageView)
Both Kingfisher and Nuke are popular image loading libraries for iOS, offering efficient caching and asynchronous image downloading. Kingfisher provides a more comprehensive set of features, including image processing and filters, making it suitable for complex image handling requirements. However, this comes at the cost of a slightly larger library size.
Nuke, on the other hand, focuses on simplicity and performance, making it an excellent choice for projects that prioritize speed and minimal overhead. Its API is straightforward and easy to use, but it may lack some advanced features found in Kingfisher.
Ultimately, the choice between Kingfisher and Nuke depends on the specific needs of your project, balancing feature richness with performance considerations.
AlamofireImage is an image component library for Alamofire
Pros of AlamofireImage
- Integrated with Alamofire, providing seamless networking and image loading
- Supports image filters and transformations out of the box
- Extensive caching options, including memory and disk caching
Cons of AlamofireImage
- Larger dependency footprint due to Alamofire integration
- Less flexible for non-Alamofire projects
- May have a steeper learning curve for developers unfamiliar with Alamofire
Code Comparison
AlamofireImage:
AF.request("https://example.com/image.jpg").responseImage { response in
if case .success(let image) = response.result {
imageView.image = image
}
}
Nuke:
Nuke.loadImage(with: URL(string: "https://example.com/image.jpg")!, into: imageView)
Summary
AlamofireImage is a powerful image loading library tightly integrated with Alamofire, offering robust features for networking and image processing. It's ideal for projects already using Alamofire. Nuke, on the other hand, is a lightweight and flexible image loading solution that can be easily integrated into any project, regardless of the networking stack. Nuke's simplicity and performance make it a great choice for projects that prioritize efficiency and ease of use.
Promises for Swift & ObjC.
Pros of PromiseKit
- Broader scope: PromiseKit is a general-purpose asynchronous programming framework, useful for various tasks beyond image loading
- Extensive ecosystem: Offers extensions for many popular libraries and frameworks
- Powerful chaining and composition: Allows for complex asynchronous operations to be expressed clearly and concisely
Cons of PromiseKit
- Learning curve: Requires understanding of promises and asynchronous programming concepts
- Overhead: May introduce additional complexity for simpler tasks compared to Nuke's focused approach
- Performance: Potentially slower for image loading specific tasks due to its general-purpose nature
Code Comparison
Nuke (Image loading):
Nuke.loadImage(with: url, into: imageView)
PromiseKit (Generic asynchronous operation):
firstly {
URLSession.shared.dataTask(.promise, with: url)
}.done { data in
// Process data
}.catch { error in
// Handle error
}
While Nuke provides a simple, one-line solution for image loading, PromiseKit offers a more verbose but flexible approach for handling various asynchronous operations. PromiseKit's syntax allows for clear chaining of multiple asynchronous tasks, error handling, and transformation of results, making it suitable for complex workflows beyond just image loading.
Reactive Programming in Swift
Pros of RxSwift
- Comprehensive reactive programming framework for Swift
- Supports complex asynchronous operations and event streams
- Large ecosystem with many extensions and integrations
Cons of RxSwift
- Steeper learning curve for developers new to reactive programming
- Can lead to increased code complexity for simple tasks
- Larger library size and potential performance overhead
Code Comparison
RxSwift:
Observable.from([1, 2, 3, 4, 5])
.filter { $0 % 2 == 0 }
.map { $0 * 2 }
.subscribe(onNext: { print($0) })
Nuke:
let imageView = UIImageView()
Nuke.loadImage(with: URL(string: "https://example.com/image.jpg")!, into: imageView)
Summary
RxSwift is a powerful reactive programming framework, offering extensive capabilities for handling asynchronous operations and event streams. It has a large ecosystem but comes with a steeper learning curve and potential complexity. Nuke, on the other hand, is a focused image loading system, providing a simpler API for image loading tasks. While RxSwift is more versatile for general reactive programming, Nuke excels in its specific domain of efficient image loading and caching.
Network abstraction layer written in Swift.
Pros of Moya
- Provides a higher level of abstraction for network requests, making API integration simpler
- Offers built-in support for testing and stubbing network calls
- Includes plugins for common tasks like authentication and logging
Cons of Moya
- May be overkill for simple networking tasks
- Requires additional setup and configuration compared to more lightweight solutions
- Learning curve can be steeper for developers new to reactive programming concepts
Code Comparison
Moya example:
let provider = MoyaProvider<MyService>()
provider.request(.userProfile) { result in
switch result {
case let .success(response):
let data = response.data
// Handle the response
case let .failure(error):
// Handle the error
}
}
Nuke example:
Nuke.loadImage(with: URL(string: "https://example.com/image.jpg")!, into: imageView)
While Moya is focused on general networking and API requests, Nuke is specifically designed for efficient image loading and caching. Moya provides a more comprehensive networking solution, while Nuke excels in its specialized domain of image handling.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
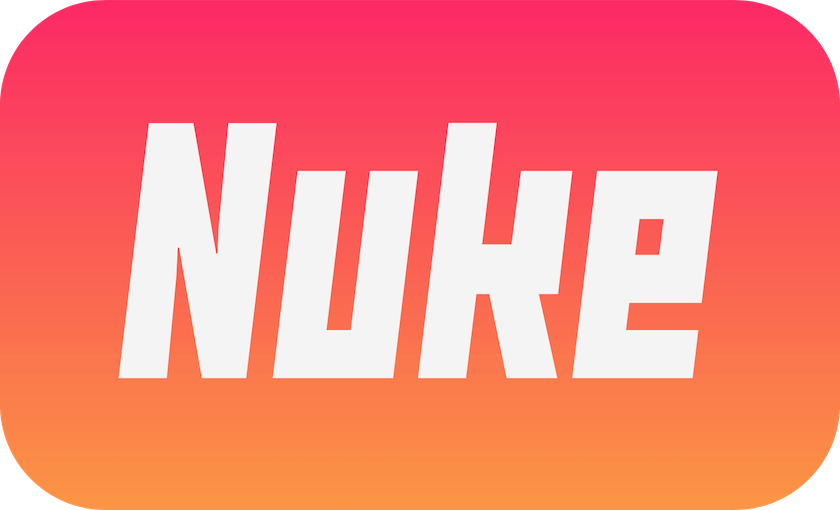
Image Loading System
Serving Images Since 2015
Load images from different sources and display them in your app using simple and flexible APIs. Take advantage of the powerful image processing capabilities and a robust caching system.
The framework is lean and compiles in under 2 seconds¹. It has an automated test suite 2x the codebase size, ensuring excellent reliability. Nuke is optimized for performance, and its advanced architecture enables virtually unlimited possibilities for customization.
Memory and Disk Cache · Image Processing & Decompression · Request Coalescing & Priority · Prefetching · Resumable Downloads · Progressive JPEG · HEIF, WebP, SVG, GIF · SwiftUI · Async/Await
Sponsors
Lapse: friends not followers.
Nuke is also supported by:
![]() Proxyman |
Backspace Travel |
Installation
Nuke supports Swift Package Manager, which is the recommended option. If that doesn't work for you, you can use binary frameworks attached to the releases.
The package ships with four modules that you can install depending on your needs:
Module | Description |
---|---|
Nuke | The lean core framework with ImagePipeline , ImageRequest , and more |
NukeUI | The UI components: LazyImage (SwiftUI) and ImageView (UIKit, AppKit) |
NukeExtensions | The extensions for UIImageView (UIKit, AppKit) |
NukeVideo | The components for decoding and playing short videos |
Documentation
Nuke is easy to learn and use, thanks to its extensive documentation and a modern API.
You can load images using ImagePipeline
from the lean core Nuke module:
func loadImage() async throws {
let imageTask = ImagePipeline.shared.imageTask(with: url)
for await progress in imageTask.progress {
// Update progress
}
imageView.image = try await imageTask.image
}
Or you can use the built-in UI components from the NukeUI module:
struct ContentView: View {
var body: some View {
LazyImage(url: URL(string: "https://example.com/image.jpeg"))
}
}
The Getting Started guide is the best place to start learning about these and many other APIs provided by the framework. Check out Nuke Demo for more usage examples.
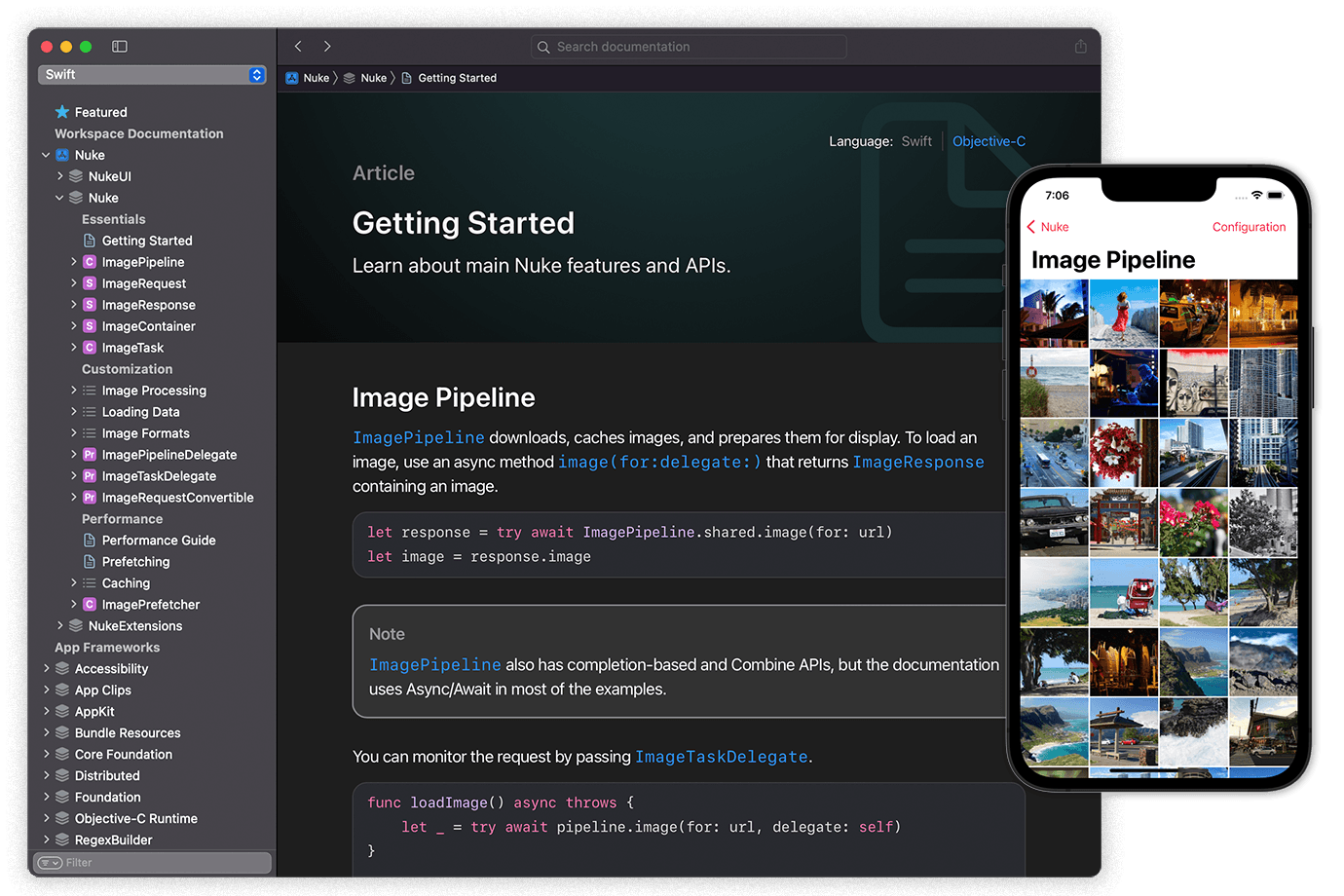
Extensions
The image pipeline is easy to customize and extend. Check out the following first-class extensions and packages built by the community.
Name | Description |
---|---|
Alamofire Plugin | Replace networking layer with Alamofire |
NukeWebP | Community. WebP support, built by Maxim Kolesnik |
WebP Plugin | Community. WebP support, built by Ryo Kosuge |
AVIF Plugin | Community. AVIF support, built by Denis |
RxNuke | RxSwift extensions for Nuke with examples |
Looking for a way to log your network requests, including image requests? Check out Pulse.
Minimum Requirements
Upgrading from the previous version? Use a Migration Guide.
Nuke | Date | Swift | Xcode | Platforms |
---|---|---|---|---|
Nuke 12.0 | Mar 4, 2023 | Swift 5.7 | Xcode 15.0 | iOS 13.0, watchOS 6.0, macOS 10.15, tvOS 13.0 |
Nuke 11.0 | Jul 20, 2022 | Swift 5.6 | Xcode 13.3 | iOS 13.0, watchOS 6.0, macOS 10.15, tvOS 13.0 |
Nuke 10.0 | Jun 1, 2021 | Swift 5.3 | Xcode 12.0 | iOS 11.0, watchOS 4.0, macOS 10.13, tvOS 11.0 |
Starting with version 12.3, Nuke also ships with visionOS support (in beta)
License
Nuke is available under the MIT license. See the LICENSE file for more info.
¹ Measured on MacBook Pro 14" 2021 (10-core M1 Pro)
Top Related Projects
Asynchronous image downloader with cache support as a UIImageView category
A lightweight, pure-Swift library for downloading and caching images from the web.
AlamofireImage is an image component library for Alamofire
Promises for Swift & ObjC.
Reactive Programming in Swift
Network abstraction layer written in Swift.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot