Top Related Projects
A lightweight, extendable front-end developer tool for mobile web page.
微信调试,各种WebView样式调试、手机浏览器的页面真机调试。便捷的远程调试手机页面、抓包工具,支持:HTTP/HTTPS,无需USB连接设备。
Chrome DevTools packaged as an app via Electron
JavaScript Performance Monitor
Official Sentry SDKs for JavaScript
Quick Overview
Eruda is a mobile web developer tool that can be used to inspect and debug web pages on mobile devices. It provides a set of developer tools similar to the browser's built-in developer tools, allowing developers to inspect the DOM, view network requests, and more, all within the mobile browser.
Pros
- Comprehensive Developer Tools: Eruda provides a wide range of developer tools, including a DOM inspector, a network monitor, a console, and more, making it a powerful tool for debugging mobile web applications.
- Cross-Platform Compatibility: Eruda works across various mobile browsers, including iOS Safari, Android Chrome, and others, making it a versatile tool for mobile web development.
- Easy Integration: Eruda can be easily integrated into any web page with a single line of code, making it a convenient tool for developers.
- Open-Source: Eruda is an open-source project, allowing developers to contribute to its development and customize it to their needs.
Cons
- Limited Functionality: While Eruda provides a comprehensive set of tools, it may not have the same level of functionality as the browser's built-in developer tools, which can be more powerful and feature-rich.
- Performance Impact: Depending on the complexity of the web page, Eruda may have a noticeable impact on the page's performance, especially on older or less powerful mobile devices.
- Requires Manual Activation: Eruda is not automatically enabled on the web page, and developers need to manually activate it, which can be an extra step in the development process.
- Potential Security Concerns: As Eruda provides access to sensitive information about the web page, it's important to ensure that it's only used in a secure development environment and not exposed to end-users.
Code Examples
Here are a few examples of how to use Eruda in your web application:
- Initializing Eruda:
eruda.init();
This code initializes the Eruda developer tools on the current web page.
- Customizing Eruda:
eruda.init({
tool: ['console', 'elements', 'network', 'resources', 'sources', 'info']
});
This code initializes Eruda with a custom set of tools, allowing you to select which tools you want to use.
- Integrating Eruda with Other Libraries:
eruda.add(eruda.get('console')).add(eruda.get('network'));
This code adds the Console and Network tools to the Eruda interface, allowing you to use them alongside other tools or libraries.
- Controlling Eruda Programmatically:
eruda.show('console');
eruda.hide('network');
eruda.toggle('elements');
These commands allow you to show, hide, and toggle the visibility of specific Eruda tools programmatically.
Getting Started
To get started with Eruda, follow these steps:
- Include the Eruda script in your HTML file:
<script src="https://cdn.jsdelivr.net/npm/eruda"></script>
- Initialize Eruda in your JavaScript code:
eruda.init();
- (Optional) Customize the Eruda tools:
eruda.init({
tool: ['console', 'elements', 'network', 'resources', 'sources', 'info']
});
- (Optional) Integrate Eruda with other libraries:
eruda.add(eruda.get('console')).add(eruda.get('network'));
- (Optional) Control Eruda programmatically:
eruda.show('console');
eruda.hide('network');
eruda.toggle('elements');
That's it! With these steps, you can easily integrate Eruda into your mobile web application and start debugging your code on the go.
Competitor Comparisons
A lightweight, extendable front-end developer tool for mobile web page.
Pros of vConsole
- More lightweight and focused on mobile debugging
- Better integration with WeChat mini-programs
- Simpler UI, potentially easier for beginners
Cons of vConsole
- Less feature-rich compared to Eruda
- Limited customization options
- Slower development and update cycle
Code Comparison
Eruda initialization:
eruda.init();
eruda.add(eruda.DOM);
eruda.add(eruda.ORIENTATION);
vConsole initialization:
var vConsole = new VConsole();
vConsole.setOption('maxLogNumber', 1000);
Both Eruda and vConsole are popular console tools for mobile web debugging. Eruda offers a more comprehensive set of features, including a DOM viewer, resource inspector, and orientation helper. It's highly customizable and frequently updated.
vConsole, on the other hand, is more lightweight and focuses on essential debugging features. It's particularly well-suited for WeChat mini-programs and provides a simpler interface that may be more approachable for beginners.
Eruda's code initialization allows for easy addition of plugins, while vConsole's setup is more straightforward but offers fewer customization options out of the box.
Ultimately, the choice between Eruda and vConsole depends on the specific needs of the project, with Eruda being more suitable for complex debugging scenarios and vConsole for simpler, mobile-focused debugging tasks.
微信调试,各种WebView样式调试、手机浏览器的页面真机调试。便捷的远程调试手机页面、抓包工具,支持:HTTP/HTTPS,无需USB连接设备。
Pros of spy-debugger
- Focuses on remote debugging for mobile devices, offering a more specialized solution
- Provides weinre functionality out of the box, simplifying the setup process
- Supports HTTPS and HTTP2 debugging, which is crucial for modern web applications
Cons of spy-debugger
- Less comprehensive feature set compared to Eruda's all-in-one console solution
- May require more setup and configuration for basic debugging tasks
- Limited to remote debugging scenarios, while Eruda can be used for both local and remote debugging
Code Comparison
spy-debugger:
const spyDebugger = require('spy-debugger');
spyDebugger({
port: 9888
});
Eruda:
(function () {
var script = document.createElement('script');
script.src = "//cdn.jsdelivr.net/npm/eruda";
document.body.appendChild(script);
script.onload = function () {
eruda.init();
}
})();
Both tools serve different purposes in the debugging ecosystem. spy-debugger is tailored for remote mobile debugging, offering specialized features like weinre integration and HTTPS support. Eruda, on the other hand, provides a more comprehensive in-browser console with a wider range of debugging tools. The choice between the two depends on the specific debugging needs and the target environment of the project.
Chrome DevTools packaged as an app via Electron
Pros of chrome-devtools-app
- Provides a standalone Chrome DevTools application
- Offers a more familiar interface for developers used to Chrome DevTools
- Supports remote debugging capabilities
Cons of chrome-devtools-app
- Larger file size and more resource-intensive
- Less suitable for mobile debugging scenarios
- Requires more setup and configuration
Code Comparison
Eruda:
eruda.init();
eruda.show();
chrome-devtools-app:
const CDP = require('chrome-remote-interface');
CDP((client) => {
// Use DevTools Protocol here
});
Key Differences
- Eruda is a lightweight, in-page console for mobile web debugging
- chrome-devtools-app is a standalone application that replicates Chrome DevTools
- Eruda is easier to integrate into web projects with minimal setup
- chrome-devtools-app offers more advanced features and a closer experience to browser-based DevTools
Use Cases
- Eruda: Quick debugging of mobile web applications, especially when access to desktop tools is limited
- chrome-devtools-app: Detailed debugging and profiling of web applications, particularly useful for remote debugging scenarios
Community and Maintenance
- Eruda: Active development, regular updates, and a growing community
- chrome-devtools-app: Less frequent updates, but still maintained with a stable user base
Both tools serve different purposes and cater to different debugging scenarios, making them complementary rather than direct competitors in many cases.
JavaScript Performance Monitor
Pros of stats.js
- Lightweight and focused solely on performance monitoring
- Easy to integrate with minimal setup
- Provides real-time FPS, MS, and MB graphs
Cons of stats.js
- Limited to performance metrics only
- Lacks advanced debugging features
- No built-in console or network monitoring
Code Comparison
stats.js:
var stats = new Stats();
stats.showPanel(0); // 0: fps, 1: ms, 2: mb, 3+: custom
document.body.appendChild(stats.dom);
function animate() {
stats.begin();
// monitored code goes here
stats.end();
requestAnimationFrame(animate);
}
Eruda:
eruda.init();
eruda.show();
// Additional configuration
eruda.add(new eruda.Plugin('fps'));
eruda.get('fps').show();
stats.js is a lightweight performance monitoring tool focused on providing real-time FPS, MS, and MB graphs. It's easy to integrate but limited in scope. Eruda, on the other hand, is a comprehensive mobile web developer tool that includes a console, network monitor, and various debugging features.
While stats.js excels in simplicity and performance monitoring, Eruda offers a broader range of debugging tools suitable for more complex development scenarios. The code comparison shows that stats.js requires manual integration into the animation loop, whereas Eruda can be initialized with a single line and offers plugin support for extended functionality.
Official Sentry SDKs for JavaScript
Pros of Sentry
- Comprehensive error tracking and monitoring solution for JavaScript applications
- Integrates with various frameworks and platforms, offering broader compatibility
- Provides detailed error reports, including stack traces and user context
Cons of Sentry
- More complex setup and configuration compared to Eruda
- Requires a Sentry account and backend infrastructure
- May have a steeper learning curve for beginners
Code Comparison
Eruda initialization:
eruda.init();
Sentry initialization:
Sentry.init({
dsn: "https://examplePublicKey@o0.ingest.sentry.io/0",
integrations: [new BrowserTracing()],
tracesSampleRate: 1.0,
});
Key Differences
- Eruda focuses on in-browser debugging and console emulation, while Sentry specializes in error tracking and monitoring
- Eruda is self-contained and doesn't require external services, whereas Sentry relies on its cloud-based infrastructure
- Sentry offers more advanced features for production environments, such as release tracking and performance monitoring
Use Cases
- Eruda: Ideal for mobile web development, quick debugging, and inspecting DOM elements on devices without developer tools
- Sentry: Better suited for large-scale applications, production error monitoring, and teams requiring detailed error analytics
Both tools serve different purposes in the JavaScript ecosystem, with Eruda excelling in on-device debugging and Sentry providing robust error tracking and monitoring capabilities.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Eruda
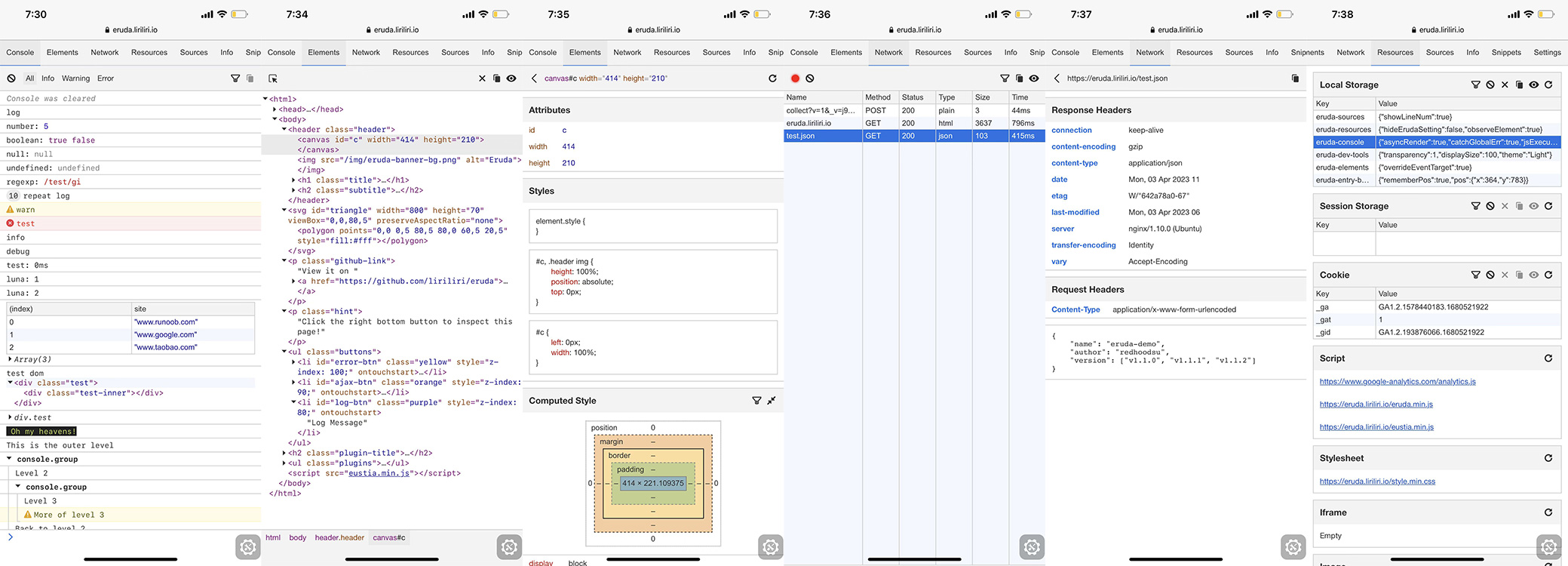
Demo
Browse it on your phone: eruda.liriliri.io
Install
You can get it on npm.
npm install eruda --save-dev
Add this script to your page.
<script src="node_modules/eruda/eruda.js"></script>
<script>eruda.init();</script>
It's also available on jsDelivr and cdnjs.
<script src="https://cdn.jsdelivr.net/npm/eruda"></script>
<script>eruda.init();</script>
For more detailed usage instructions, please read the documentation at eruda.liriliri.io!
Related Projects
- eruda-android: Simple webview with eruda loaded automatically.
- chii: Remote debugging tool.
- chobitsu: Chrome devtools protocol JavaScript implementation.
- licia: Utility library used by eruda.
- luna: UI components used by eruda.
- vivy: Icon image generation.
Third Party
- eruda-pixel: UI pixel restoration tool.
- eruda-webpack-plugin: Eruda webpack plugin.
- eruda-vue-devtools: Eruda Vue-devtools plugin.
Backers
Contribution
Read Contributing Guide for development setup instructions.
Top Related Projects
A lightweight, extendable front-end developer tool for mobile web page.
微信调试,各种WebView样式调试、手机浏览器的页面真机调试。便捷的远程调试手机页面、抓包工具,支持:HTTP/HTTPS,无需USB连接设备。
Chrome DevTools packaged as an app via Electron
JavaScript Performance Monitor
Official Sentry SDKs for JavaScript
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot