magicui
UI Library for Design Engineers. Animated components and effects you can copy and paste into your apps. Free. Open Source.
Top Related Projects
A set of beautifully-designed, accessible components and a code distribution platform. Works with your favorite frameworks. Open Source. Open Code.
Radix Primitives is an open-source UI component library for building high-quality, accessible design systems and web apps. Maintained by @workos.
A utility-first CSS framework for rapid UI development.
Chakra UI is a component system for building SaaS products with speed ⚡️
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
daisyUI components built with React 🌼
Quick Overview
MagicUI is a collection of beautifully designed, accessible, and customizable UI components for React applications. It aims to provide developers with a set of ready-to-use components that are both visually appealing and functionally robust, saving time and effort in building modern web interfaces.
Pros
- Extensive collection of pre-built, customizable components
- Emphasis on accessibility and modern design principles
- Well-documented with interactive examples
- Built with TypeScript for improved type safety and developer experience
Cons
- Relatively new project, may have fewer community contributions compared to more established UI libraries
- Limited theming options compared to some other UI frameworks
- Potential learning curve for developers new to React or component-based UI development
- May require additional setup for complex state management in larger applications
Code Examples
- Using a Button component:
import { Button } from '@magicui/react';
function MyComponent() {
return (
<Button variant="primary" onClick={() => console.log('Clicked!')}>
Click me
</Button>
);
}
- Implementing a Modal:
import { Modal, Button } from '@magicui/react';
import { useState } from 'react';
function ModalExample() {
const [isOpen, setIsOpen] = useState(false);
return (
<>
<Button onClick={() => setIsOpen(true)}>Open Modal</Button>
<Modal isOpen={isOpen} onClose={() => setIsOpen(false)}>
<Modal.Header>Modal Title</Modal.Header>
<Modal.Body>This is the modal content.</Modal.Body>
<Modal.Footer>
<Button onClick={() => setIsOpen(false)}>Close</Button>
</Modal.Footer>
</Modal>
</>
);
}
- Using a Form component with validation:
import { Form, Input, Button } from '@magicui/react';
function LoginForm() {
const handleSubmit = (values) => {
console.log('Form submitted:', values);
};
return (
<Form onSubmit={handleSubmit}>
<Input
name="email"
type="email"
label="Email"
required
validation={{ email: true }}
/>
<Input
name="password"
type="password"
label="Password"
required
validation={{ minLength: 8 }}
/>
<Button type="submit">Log In</Button>
</Form>
);
}
Getting Started
To start using MagicUI in your React project, follow these steps:
-
Install the package:
npm install @magicui/react
-
Import and use components in your React application:
import { Button, Card, Input } from '@magicui/react'; function App() { return ( <Card> <h1>Welcome to MagicUI</h1> <Input placeholder="Enter your name" /> <Button>Submit</Button> </Card> ); }
-
(Optional) If you're using TypeScript, the types are included and should work out of the box.
Competitor Comparisons
A set of beautifully-designed, accessible components and a code distribution platform. Works with your favorite frameworks. Open Source. Open Code.
Pros of shadcn/ui
- More customizable and flexible, allowing developers to copy and paste components
- Larger community and more frequent updates
- Better documentation and examples
Cons of shadcn/ui
- Steeper learning curve due to its modular nature
- Requires more setup and configuration
Code Comparison
MagicUI:
import { Button } from '@magicuidesign/magicui'
function App() {
return <Button>Click me</Button>
}
shadcn/ui:
import { Button } from "@/components/ui/button"
function App() {
return <Button>Click me</Button>
}
Summary
MagicUI offers a more straightforward, out-of-the-box solution with pre-built components, making it easier for beginners to get started quickly. It's ideal for projects that don't require extensive customization.
shadcn/ui, on the other hand, provides a more flexible and customizable approach. It allows developers to copy and paste components into their projects, giving them full control over the code. This makes it better suited for larger, more complex projects that require specific styling and functionality.
Both libraries are built on top of Tailwind CSS and offer a range of UI components. The choice between them depends on the project requirements, developer experience, and desired level of customization.
Radix Primitives is an open-source UI component library for building high-quality, accessible design systems and web apps. Maintained by @workos.
Pros of Primitives
- More comprehensive set of UI components
- Highly customizable and accessible out-of-the-box
- Larger community and ecosystem support
Cons of Primitives
- Steeper learning curve due to its flexibility
- Requires more setup and configuration
Code Comparison
MagicUI:
import { Button } from '@magicuidesign/magicui'
<Button variant="primary">Click me</Button>
Primitives:
import * as Button from '@radix-ui/react-button'
<Button.Root>
<Button.Text>Click me</Button.Text>
</Button.Root>
Key Differences
- MagicUI offers a more opinionated and ready-to-use design system
- Primitives provides unstyled, accessible components for custom designs
- MagicUI has a simpler API, while Primitives offers more granular control
Use Cases
MagicUI
- Rapid prototyping and development
- Projects requiring a consistent, pre-designed look
Primitives
- Custom design systems
- Applications needing fine-grained control over accessibility and styling
Community and Support
- Primitives has a larger community and more frequent updates
- MagicUI is newer but growing, with a focus on simplicity and ease of use
A utility-first CSS framework for rapid UI development.
Pros of Tailwind CSS
- Larger community and ecosystem, with more resources and third-party tools
- More comprehensive documentation and extensive examples
- Highly customizable with a robust configuration system
Cons of Tailwind CSS
- Steeper learning curve due to its utility-first approach
- Can lead to longer class strings in HTML, potentially reducing readability
- Requires additional build steps for optimal performance
Code Comparison
Tailwind CSS:
<button class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
Button
</button>
MagicUI:
<button class="btn btn-primary">
Button
</button>
MagicUI offers a more concise class structure, while Tailwind CSS provides more granular control over styles directly in the HTML. Tailwind CSS's approach allows for greater flexibility but can result in longer class strings. MagicUI's pre-defined components may lead to faster development for common UI elements but might be less customizable out of the box.
Both libraries aim to streamline UI development, with Tailwind CSS focusing on utility-first composition and MagicUI emphasizing pre-built components. The choice between them depends on project requirements, team preferences, and the desired balance between customization and development speed.
Chakra UI is a component system for building SaaS products with speed ⚡️
Pros of Chakra UI
- More mature and widely adopted, with a larger community and ecosystem
- Extensive documentation and examples, making it easier for developers to get started
- Built-in support for dark mode and color mode switching
Cons of Chakra UI
- Larger bundle size, which may impact initial load times for applications
- Steeper learning curve due to its extensive API and component library
- Less flexibility in customizing the underlying design system
Code Comparison
Chakra UI:
import { Box, Button, Text } from '@chakra-ui/react'
function MyComponent() {
return (
<Box p={4}>
<Text fontSize="xl">Hello, Chakra UI!</Text>
<Button colorScheme="blue">Click me</Button>
</Box>
)
}
MagicUI:
import { Box, Button, Text } from '@magicui/react'
function MyComponent() {
return (
<Box p="4">
<Text size="xl">Hello, MagicUI!</Text>
<Button variant="primary">Click me</Button>
</Box>
)
}
Both libraries offer similar component-based approaches, but Chakra UI uses a more prop-based styling system, while MagicUI leans towards predefined variants. Chakra UI's extensive customization options are evident in the colorScheme
prop, whereas MagicUI focuses on simplicity with predefined variants.
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
Pros of Material-UI
- Extensive component library with a wide range of pre-built UI elements
- Strong community support and regular updates
- Comprehensive documentation and examples
Cons of Material-UI
- Larger bundle size due to its extensive feature set
- Steeper learning curve for customization and theming
- More opinionated design system, which may limit flexibility
Code Comparison
Material-UI:
import { Button, TextField } from '@mui/material';
<Button variant="contained" color="primary">
Click me
</Button>
<TextField label="Enter text" variant="outlined" />
MagicUI:
import { Button, Input } from '@magicuidesign/magicui';
<Button variant="primary">Click me</Button>
<Input placeholder="Enter text" />
Key Differences
- Material-UI offers a more comprehensive set of components and features
- MagicUI focuses on simplicity and ease of use
- Material-UI has a steeper learning curve but provides more customization options
- MagicUI aims for a lighter bundle size and faster implementation
Use Cases
- Material-UI: Large-scale applications requiring a wide range of UI components
- MagicUI: Smaller projects or prototypes needing quick implementation
Community and Support
- Material-UI: Large, active community with extensive third-party resources
- MagicUI: Smaller community, but growing with a focus on simplicity
daisyUI components built with React 🌼
Pros of react-daisyui
- More comprehensive component library with a wider range of pre-built UI elements
- Better documentation and examples for each component
- Larger community and more frequent updates
Cons of react-daisyui
- Heavier bundle size due to the extensive component library
- Less flexibility in customization compared to MagicUI's utility-first approach
- Steeper learning curve for developers new to the DaisyUI ecosystem
Code Comparison
MagicUI:
<Button variant="primary" size="lg">
Click me
</Button>
react-daisyui:
<Button color="primary" size="lg">
Click me
</Button>
Both libraries offer similar syntax for basic components, but react-daisyui provides more pre-built variants and options out of the box. MagicUI focuses on utility classes for customization, while react-daisyui offers more opinionated components with built-in styles.
MagicUI is better suited for projects requiring high customization and a lightweight approach, while react-daisyui excels in rapid prototyping and projects that benefit from a rich set of pre-designed components.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
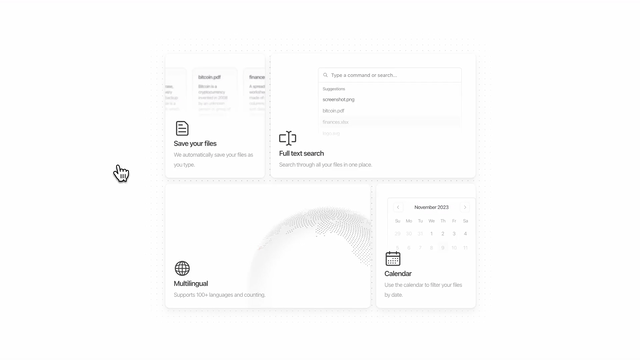
Magic UI
UI Library for Design Engineers
Documentation
Visit https://magicui.design/docs to view the documentation.
Contributing
Visit our contributing guide to learn how to contribute. It only takes ~5 minutes to add your own!
Let's talk
Authors
Deploy
Stats
License
Licensed under the MIT license.
Top Related Projects
A set of beautifully-designed, accessible components and a code distribution platform. Works with your favorite frameworks. Open Source. Open Code.
Radix Primitives is an open-source UI component library for building high-quality, accessible design systems and web apps. Maintained by @workos.
A utility-first CSS framework for rapid UI development.
Chakra UI is a component system for building SaaS products with speed ⚡️
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
daisyUI components built with React 🌼
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot