Top Related Projects
vue calendar fullCalendar. no jquery required. Schedule events management
Quick Overview
V-Calendar is a modern and versatile calendar plugin for Vue.js applications. It provides a highly customizable and feature-rich calendar component that can be easily integrated into Vue projects, offering support for date pickers, date ranges, and various calendar views.
Pros
- Highly customizable with a wide range of props and slots
- Supports multiple calendar views (day, week, month, year)
- Responsive design and mobile-friendly
- Excellent documentation and examples
Cons
- Learning curve for advanced customizations
- Limited built-in internationalization support
- Some users report performance issues with large datasets
- Dependency on Vue.js limits its use in other frameworks
Code Examples
- Basic calendar implementation:
<template>
<v-calendar />
</template>
<script>
import VCalendar from 'v-calendar';
export default {
components: {
VCalendar,
},
};
</script>
- Date picker with custom date format:
<template>
<v-date-picker v-model="date" :input-props="{ format: 'MM/dd/yyyy' }" />
</template>
<script>
import { DatePicker } from 'v-calendar';
export default {
components: {
VDatePicker: DatePicker,
},
data() {
return {
date: new Date(),
};
},
};
</script>
- Calendar with custom event rendering:
<template>
<v-calendar :attributes="attributes">
<template #day-content="{ day, attributes }">
<div v-for="attr in attributes" :key="attr.key" class="dot" :style="{ backgroundColor: attr.dot.color }"></div>
</template>
</v-calendar>
</template>
<script>
import VCalendar from 'v-calendar';
export default {
components: {
VCalendar,
},
data() {
return {
attributes: [
{
key: 'today',
dates: new Date(),
dot: { color: 'red' },
},
{
key: 'events',
dates: [new Date(2023, 4, 1), new Date(2023, 4, 15)],
dot: { color: 'blue' },
},
],
};
},
};
</script>
Getting Started
-
Install v-calendar:
npm install v-calendar@next
-
Import and use v-calendar in your Vue 3 app:
import { createApp } from 'vue'; import VCalendar from 'v-calendar'; import 'v-calendar/dist/style.css'; const app = createApp(App); app.use(VCalendar, {}); app.mount('#app');
-
Use v-calendar components in your Vue templates:
<template> <v-calendar /> <v-date-picker v-model="date" /> </template>
Competitor Comparisons
vue calendar fullCalendar. no jquery required. Schedule events management
Pros of vue-fullcalendar
- Lightweight and simple to use, with a focus on basic calendar functionality
- Easy integration with Vue.js projects
- Supports drag-and-drop event handling out of the box
Cons of vue-fullcalendar
- Less actively maintained compared to v-calendar
- Fewer customization options and advanced features
- Limited documentation and community support
Code Comparison
v-calendar:
<v-calendar
:attributes="attributes"
is-expanded
:columns="$screens({ default: 1, lg: 2 })"
/>
vue-fullcalendar:
<full-calendar
:events="events"
:config="config"
@event-selected="eventSelected"
/>
v-calendar offers more built-in props for customization, while vue-fullcalendar relies on a separate config object for advanced settings. v-calendar also provides responsive layout options, which are not directly available in vue-fullcalendar.
v-calendar generally offers more features and flexibility, making it suitable for complex calendar applications. vue-fullcalendar is a simpler option that may be sufficient for basic calendar needs in Vue.js projects. However, its limited maintenance and documentation may pose challenges for long-term use or advanced implementations.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
An elegant calendar and datepicker plugin for Vuejs.
npm i --save v-calendar
Documentation
For full documentation, visit vcalendar.io.
Attributes
Highlights | Dots |
---|---|
![]() | ![]() |
Bars | Popovers |
---|---|
![]() | ![]() |
Multi-Paned Calendars
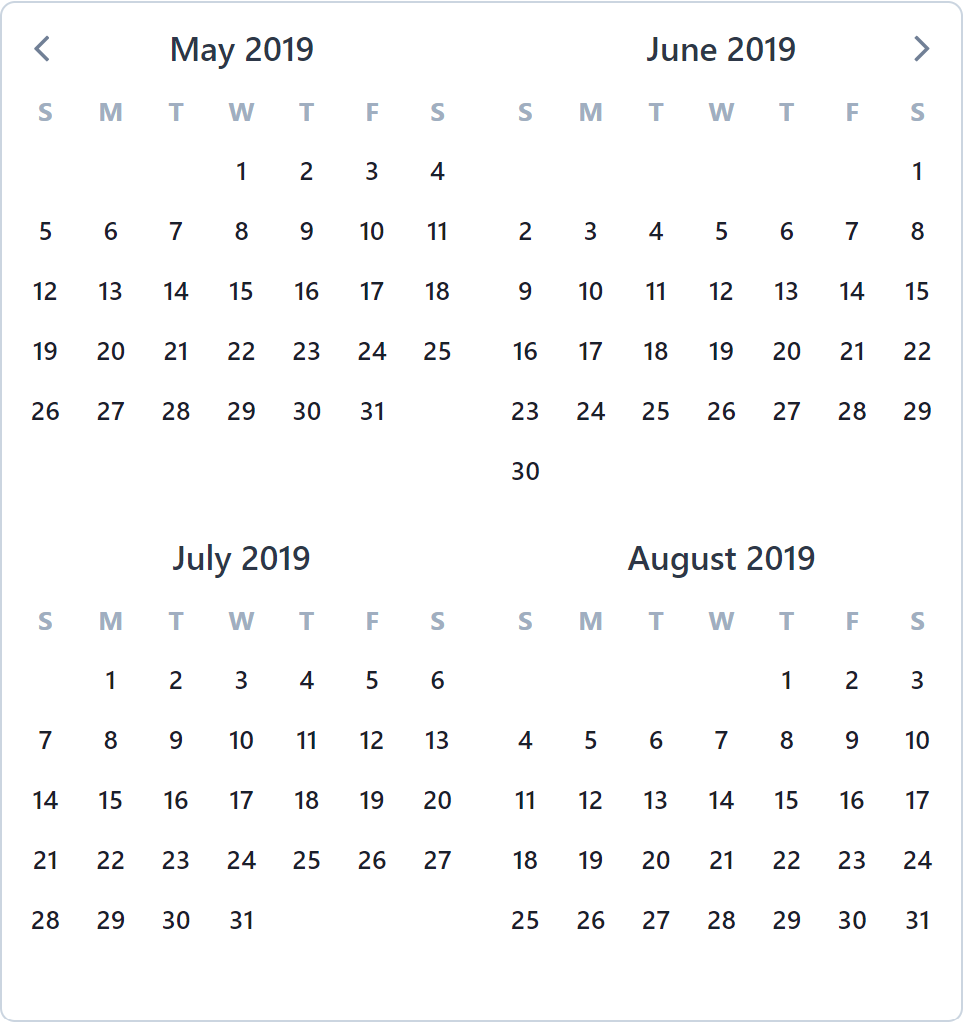
Theme Colors & Dark-Mode
![]() | ![]() |
![]() | ![]() |
Date Pickers
Single Date | Multiple Date | Date Range |
---|---|---|
![]() | ![]() | ![]() |
Custom Calendars w/ Scoped Slots
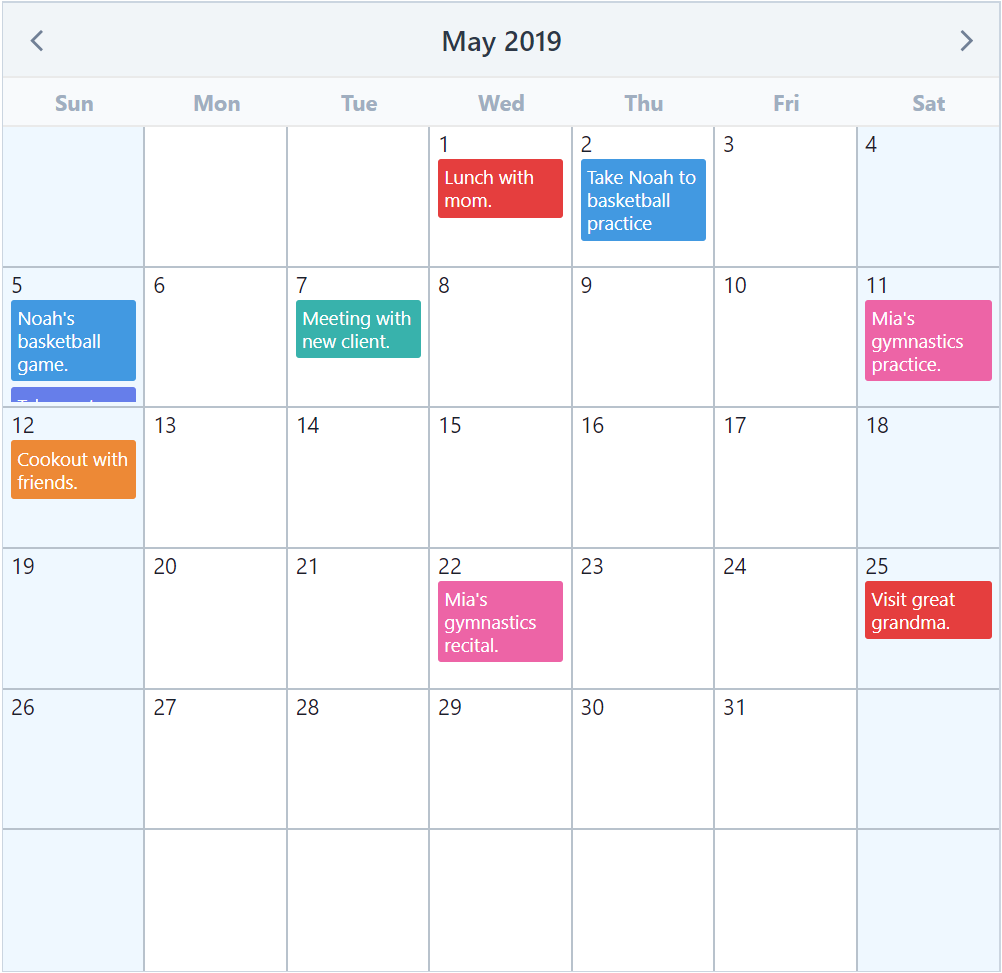
Top Related Projects
vue calendar fullCalendar. no jquery required. Schedule events management
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot