node-oidc-provider
OpenID Certified™ OAuth 2.0 Authorization Server implementation for Node.js
Top Related Projects
Open Source Identity and Access Management For Modern Applications and Services
The only web-scale, fully customizable OpenID Certified™ OpenID Connect and OAuth2 Provider in the world. Become an OpenID Connect and OAuth2 Provider over night. Written in Go, cloud native, headless, API-first. Available as a service on Ory Network and for self-hosters. Relied upon by OpenAI and others for web-scale security.
Simple, unobtrusive authentication for Node.js.
Open source alternative to Auth0 / Firebase Auth / AWS Cognito
The Single Sign-On Multi-Factor portal for web apps
Quick Overview
panva/node-oidc-provider is a certified OpenID Connect Provider (OP) implementation for Node.js. It offers a flexible and feature-rich solution for implementing OpenID Connect authentication and authorization in Node.js applications. The library is fully compliant with OpenID Connect specifications and supports various OAuth 2.0 flows.
Pros
- Fully certified and compliant with OpenID Connect specifications
- Highly customizable and extensible
- Supports various OAuth 2.0 flows and grant types
- Active development and community support
Cons
- Steep learning curve for beginners
- Requires a good understanding of OpenID Connect and OAuth 2.0 concepts
- Configuration can be complex for advanced use cases
- Limited built-in UI components for login and consent screens
Code Examples
- Basic Provider Setup:
const { Provider } = require('oidc-provider');
const configuration = {
clients: [{
client_id: 'foo',
client_secret: 'bar',
redirect_uris: ['https://example.com/cb'],
}],
};
const oidc = new Provider('https://your-op.example.com', configuration);
// Use with your web framework
app.use('/oidc', oidc.callback());
- Custom Claims:
const oidc = new Provider('https://your-op.example.com', {
async findAccount(ctx, id) {
return {
accountId: id,
async claims(use, scope) {
return { sub: id, email: 'foo@bar.com' };
},
};
},
});
- Custom Grant Type:
const oidc = new Provider('https://your-op.example.com', {
features: {
customGrants: {
enabled: true,
},
},
});
oidc.registerGrantType('custom_grant', async function customGrantTypeHandler(ctx, next) {
// Custom grant type logic here
ctx.oidc.grant = { ... };
await next();
});
Getting Started
-
Install the package:
npm install oidc-provider
-
Create a basic provider:
const { Provider } = require('oidc-provider'); const configuration = { clients: [{ client_id: 'client', client_secret: 'secret', redirect_uris: ['https://client.example.com/cb'], }], }; const oidc = new Provider('https://your-op.example.com', configuration);
-
Use with your web framework (e.g., Express):
const express = require('express'); const app = express(); app.use('/oidc', oidc.callback()); app.listen(3000);
Competitor Comparisons
Open Source Identity and Access Management For Modern Applications and Services
Pros of Keycloak
- Comprehensive identity and access management solution with a wide range of features
- Supports multiple protocols (OpenID Connect, SAML, OAuth 2.0) out of the box
- Provides a user-friendly admin console for easy management and configuration
Cons of Keycloak
- Heavier resource footprint and more complex setup compared to node-oidc-provider
- Steeper learning curve due to its extensive feature set
- Less flexibility for customization in certain scenarios
Code Comparison
Keycloak (Java):
@Override
protected void configureHttpSecurity(HttpSecurity http) throws Exception {
super.configureHttpSecurity(http);
http.authorizeRequests()
.antMatchers("/protected-resource").authenticated()
.anyRequest().permitAll();
}
node-oidc-provider (JavaScript):
const oidc = new Provider('https://your-op.example.com', {
clients: [
{ client_id: 'foo', client_secret: 'bar', redirect_uris: ['https://client.example.com/cb'] }
],
features: {
devInteractions: { enabled: false }
}
});
The code snippets demonstrate the configuration of security settings in Keycloak and the initialization of node-oidc-provider. Keycloak uses Java annotations and HttpSecurity configuration, while node-oidc-provider uses a more concise JavaScript setup.
The only web-scale, fully customizable OpenID Certified™ OpenID Connect and OAuth2 Provider in the world. Become an OpenID Connect and OAuth2 Provider over night. Written in Go, cloud native, headless, API-first. Available as a service on Ory Network and for self-hosters. Relied upon by OpenAI and others for web-scale security.
Pros of Hydra
- Written in Go, offering better performance and lower resource usage
- Supports multiple databases (PostgreSQL, MySQL, CockroachDB)
- Provides a more comprehensive OAuth2 and OpenID Connect implementation
Cons of Hydra
- Steeper learning curve due to its extensive feature set
- Less flexibility for customization compared to node-oidc-provider
- Requires separate components for user management and consent flows
Code Comparison
node-oidc-provider:
const provider = new Provider('https://my-oidc-provider.com', {
clients: [{ client_id: 'foo', client_secret: 'bar', redirect_uris: ['https://client.example.com/cb'] }],
// ... other configuration options
});
Hydra:
import "github.com/ory/hydra/driver"
d := driver.NewDefaultDriver(
driver.WithDSN("postgres://user:password@host:port/database"),
// ... other configuration options
)
Both projects offer robust OpenID Connect and OAuth 2.0 implementations, but they cater to different use cases. node-oidc-provider is more flexible and easier to customize, making it suitable for projects that require specific modifications. Hydra, on the other hand, provides a more comprehensive solution with better performance, making it ideal for large-scale deployments and enterprise environments.
Simple, unobtrusive authentication for Node.js.
Pros of Passport
- More versatile, supporting various authentication strategies beyond just OpenID Connect
- Larger community and ecosystem with numerous plugins and integrations
- Simpler to set up for basic authentication needs
Cons of Passport
- Less specialized for OpenID Connect, requiring additional configuration for full OIDC support
- May require more manual work to implement complex OIDC features
- Not as up-to-date with the latest OIDC specifications compared to node-oidc-provider
Code Comparison
Passport (basic setup):
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
passport.use(new LocalStrategy((username, password, done) => {
// Authentication logic here
}));
node-oidc-provider (basic setup):
const Provider = require('oidc-provider');
const configuration = {
// OIDC configuration options
};
const oidc = new Provider('http://localhost:3000', configuration);
Both libraries offer different approaches to authentication. Passport provides a more general-purpose solution with its strategy-based architecture, while node-oidc-provider focuses specifically on implementing a full-featured OpenID Connect Provider. The choice between them depends on the specific requirements of your project and the level of OIDC compliance needed.
Open source alternative to Auth0 / Firebase Auth / AWS Cognito
Pros of SuperTokens
- Offers a complete authentication solution with pre-built UI components
- Provides multi-tenancy support out of the box
- Includes features like passwordless login and social login integrations
Cons of SuperTokens
- Less flexible for custom OIDC implementations
- May have a steeper learning curve for developers familiar with raw OIDC
- Limited to specific programming languages and frameworks
Code Comparison
SuperTokens (Node.js SDK):
import supertokens from "supertokens-node";
import Session from "supertokens-node/recipe/session";
supertokens.init({
appInfo: {
apiDomain: "https://api.example.com",
appName: "MyApp",
websiteDomain: "https://example.com"
},
recipeList: [Session.init()]
});
node-oidc-provider:
const { Provider } = require('oidc-provider');
const configuration = {
clients: [{ client_id: 'foo', client_secret: 'bar', redirect_uris: ['http://example.com/cb'] }],
};
const oidc = new Provider('http://localhost:3000', configuration);
Both libraries offer authentication solutions, but SuperTokens provides a more comprehensive package with pre-built components, while node-oidc-provider offers greater flexibility for custom OIDC implementations. The choice between them depends on specific project requirements and developer expertise.
The Single Sign-On Multi-Factor portal for web apps
Pros of Authelia
- Comprehensive authentication solution with multi-factor authentication, single sign-on, and access control
- Supports various authentication backends (LDAP, file-based) and integrates with popular reverse proxies
- Offers a user-friendly web portal for authentication and account management
Cons of Authelia
- More complex setup and configuration compared to node-oidc-provider
- Limited focus on OpenID Connect (OIDC) functionality, as it's a broader authentication solution
- May have a steeper learning curve for developers primarily interested in OIDC implementation
Code Comparison
Authelia configuration (YAML):
jwt_secret: a_very_important_secret
default_redirection_url: https://public.example.com
server:
host: 0.0.0.0
port: 9091
node-oidc-provider configuration (JavaScript):
const oidc = new Provider('https://your-domain.com', {
clients: [
{ client_id: 'foo', client_secret: 'bar', redirect_uris: ['https://example.com/cb'] }
],
// ... other configuration options
});
While Authelia provides a more comprehensive authentication solution with additional features, node-oidc-provider offers a focused and flexible OIDC implementation. The choice between the two depends on specific project requirements and the desired level of OIDC functionality.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
oidc-provider
This module provides an OAuth 2.0 (RFC 6749) Authorization Server with support for OpenID Connect (OIDC) and many other additional features and standards.
Table of Contents
Implemented specs & features
The following specifications are implemented by oidc-provider (not exhaustive):
Note that not all features are enabled by default, check the configuration section on how to enable them.
RFC6749
- OAuth 2.0 &OIDC
Core 1.0- OIDC
Discovery 1.0
- Dynamic Client Registration
- OIDC
RP-Initiated Logout 1.0
- OIDC
Back-Channel Logout 1.0
RFC7009
- OAuth 2.0 Token RevocationRFC7636
- Proof Key for Code Exchange (PKCE
)RFC7662
- OAuth 2.0 Token IntrospectionRFC8252
- OAuth 2.0 for Native Apps BCP (AppAuth
)RFC8628
- OAuth 2.0 Device Authorization Grant (Device Flow
)RFC8705
- OAuth 2.0 Mutual TLS Client Authentication and Certificate Bound Access Tokens (MTLS
)RFC8707
- OAuth 2.0 Resource IndicatorsRFC9101
- OAuth 2.0 JWT-Secured Authorization Request (JAR
)RFC9126
- OAuth 2.0 Pushed Authorization Requests (PAR
)RFC9207
- OAuth 2.0 Authorization Server Issuer Identifier in Authorization ResponseRFC9449
- OAuth 2.0 Demonstration of Proof-of-Possession at the Application Layer (DPoP
)RFC9701
- JWT Response for OAuth Token Introspection- FAPI 1.0 Security Profile - Part 2: Advanced (
FAPI 1.0
) - FAPI 2.0 Security Profile (
FAPI 2.0
) - JWT Secured Authorization Response Mode for OAuth 2.0 (
JARM
) - OIDC Client Initiated Backchannel Authentication Flow (
CIBA
)
Supported Access Token formats:
The following draft specifications are implemented by oidc-provider:
- Financial-grade API: Client Initiated Backchannel Authentication Profile (
FAPI-CIBA
) - Implementer's Draft 01 - FAPI 2.0 Message Signing (
FAPI 2.0
) - Implementer's Draft 01 - OIDC Relying Party Metadata Choices 1.0 - draft 02
Updates to draft specification versions are released as MINOR library versions,
if you utilize these specification implementations consider using the tilde ~
operator in your
package.json since breaking changes may be introduced as part of these version updates. Alternatively
acknowledge the version and be notified of breaking changes as part of
your CI.
Certification
Filip Skokan has certified that oidc-provider
conforms to the following profiles of the OpenID Connect⢠protocol.
- Basic, Implicit, Hybrid, Config, Form Post, and 3rd Party-Init
- Back-Channel Logout and RP-Initiated Logout
- FAPI 1.0
- FAPI CIBA
- FAPI 2.0
Sponsor
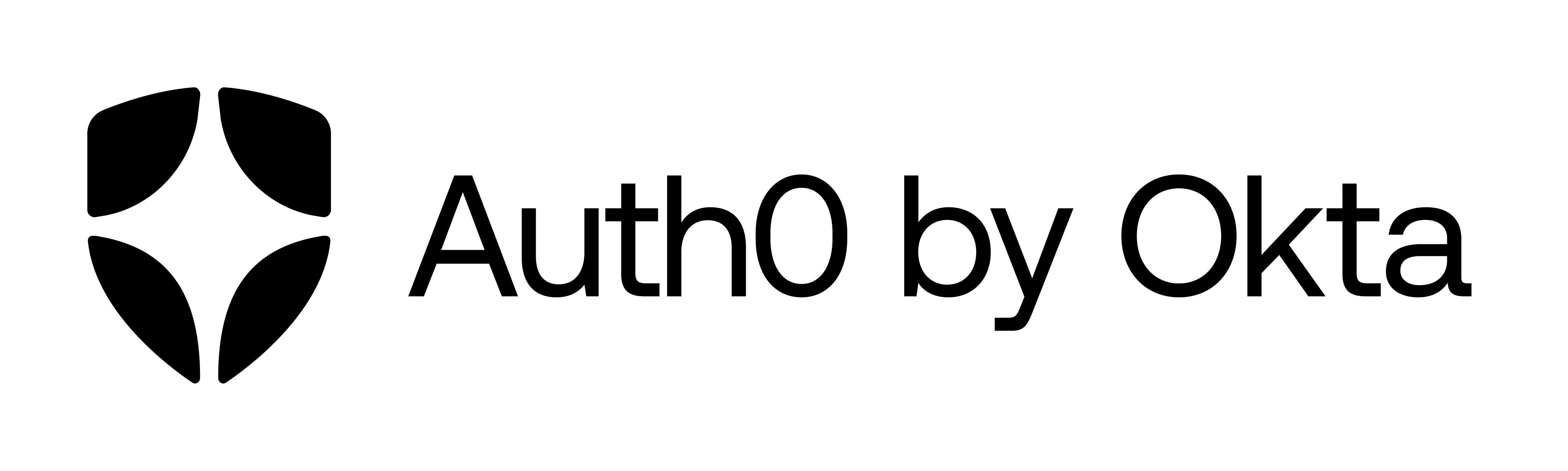
If you want to quickly add OpenID Connect authentication to Node.js apps, feel free to check out Auth0's Node.js SDK and free plan. Create an Auth0 account; it's free!
Support
If you or your company use this module, or you need help using/upgrading the module, please consider becoming a sponsor so I can continue maintaining it and adding new features carefree. The only way to guarantee you get feedback from the author & sole maintainer of this module is to support the package through GitHub Sponsors.
Documentation & Configuration
oidc-provider can be mounted to existing connect, express, fastify, hapi, or koa applications, see how. The authorization server allows to be extended and configured in various ways to fit a variety of uses. See the documentation and example folder.
import * as oidc from "oidc-provider";
const provider = new oidc.Provider("http://localhost:3000", {
// refer to the documentation for other available configuration
clients: [
{
client_id: "foo",
client_secret: "bar",
redirect_uris: ["http://lvh.me:8080/cb"],
// ... other client properties
},
],
});
const server = oidc.listen(3000, () => {
console.log(
"oidc-provider listening on port 3000, check http://localhost:3000/.well-known/openid-configuration",
);
});
External type definitions are available via DefinitelyTyped.
Community Guides
Collection of Community-maintained configuration use cases are in the Community Guides Discussions section
Events
oidc-provider instances are event emitters, using event handlers you can hook into the various actions and i.e. emit metrics that react to specific triggers. See the list of available emitted event names and their description.
Supported Versions
Version | Security Fixes ð | Other Bug Fixes ð | New Features â |
---|---|---|---|
v9.x | Security Policy | â | â |
v8.x | Security Policy | â | â |
Top Related Projects
Open Source Identity and Access Management For Modern Applications and Services
The only web-scale, fully customizable OpenID Certified™ OpenID Connect and OAuth2 Provider in the world. Become an OpenID Connect and OAuth2 Provider over night. Written in Go, cloud native, headless, API-first. Available as a service on Ory Network and for self-hosters. Relied upon by OpenAI and others for web-scale security.
Simple, unobtrusive authentication for Node.js.
Open source alternative to Auth0 / Firebase Auth / AWS Cognito
The Single Sign-On Multi-Factor portal for web apps
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot