playwright-go
Playwright for Go a browser automation library to control Chromium, Firefox and WebKit with a single API.
Top Related Projects
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Fast, easy and reliable testing for anything that runs in a browser.
JavaScript API for Chrome and Firefox
A browser automation framework and ecosystem.
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Quick Overview
The playwright-community/playwright-go
repository provides a Go language binding for the Playwright browser automation library. Playwright is a powerful tool for automating web browsers, allowing developers to write end-to-end tests, scrape websites, and perform other browser-related tasks.
Pros
- Cross-Browser Compatibility: Playwright supports multiple browsers, including Chromium, Firefox, and WebKit, allowing for comprehensive testing and automation across different browser environments.
- Robust API: The Go binding for Playwright offers a comprehensive and well-documented API, making it easy for Go developers to integrate Playwright into their projects.
- Reliable and Stable: Playwright is known for its reliability and stability, providing a solid foundation for browser automation tasks.
- Active Community: The Playwright project has an active community of contributors and users, ensuring ongoing development, support, and improvements.
Cons
- Dependency on Playwright Core: The Go binding for Playwright is dependent on the underlying Playwright core library, which is primarily developed in TypeScript. This may introduce some complexity for Go developers who are not familiar with the Playwright ecosystem.
- Limited Adoption: Compared to other browser automation libraries in the Go ecosystem, the Playwright-Go binding may have a smaller user base and less widespread adoption.
- Learning Curve: While the Playwright API is well-documented, there may be a learning curve for Go developers who are new to the Playwright ecosystem.
- Performance Overhead: Depending on the specific use case, the overhead of running a full-fledged browser for automation tasks may be a concern for some projects.
Code Examples
Here are a few code examples demonstrating the usage of the playwright-community/playwright-go
library:
- Launching a Browser and Navigating to a Website:
package main
import (
"fmt"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
panic(err)
}
defer pw.Stop()
browser, err := pw.Chromium.Launch()
if err != nil {
panic(err)
}
defer browser.Close()
page, err := browser.NewPage()
if err != nil {
panic(err)
}
if _, err := page.Goto("https://www.example.com"); err != nil {
panic(err)
}
fmt.Println(page.Title())
}
- Taking a Screenshot of a Page:
package main
import (
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
panic(err)
}
defer pw.Stop()
browser, err := pw.Chromium.Launch()
if err != nil {
panic(err)
}
defer browser.Close()
page, err := browser.NewPage()
if err != nil {
panic(err)
}
if _, err := page.Goto("https://www.example.com"); err != nil {
panic(err)
}
if err := page.Screenshot(playwright.PageScreenshotOptions{
Path: playwright.String("example.png"),
}); err != nil {
panic(err)
}
}
- Interacting with Form Elements:
package main
import (
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
panic(err)
}
defer pw.Stop()
browser, err := pw.Chromium.Launch()
if err != nil {
panic(err)
}
defer browser.Close()
page, err := browser.NewPage()
if err != nil {
panic(err)
}
if _, err := page.Goto("https://www.example.com/form"); err != nil {
panic(err)
}
if err := page.Fill("input[name='name']", "John
Competitor Comparisons
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Pros of Playwright
- Playwright is developed and maintained by Microsoft, a large and reputable tech company, which can provide more resources and support for the project.
- Playwright has a wider range of browser support, including Chromium, Firefox, and WebKit, making it more versatile for cross-browser testing.
- Playwright has a more extensive set of features and APIs, such as support for mobile emulation and PDF generation.
Cons of Playwright
- Playwright is a newer project compared to playwright-community/playwright-go, which may have a smaller community and fewer third-party integrations.
- Playwright's Go implementation (playwright-go) is maintained by the community, while the official Playwright project is primarily focused on the JavaScript/TypeScript version.
Code Comparison
Playwright (JavaScript):
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://www.example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
playwright-go (Go):
package main
import (
"fmt"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
fmt.Println(err)
}
defer pw.Stop()
browser, err := pw.Chromium.Launch()
if err != nil {
fmt.Println(err)
}
defer browser.Close()
page, err := browser.NewPage()
if err != nil {
fmt.Println(err)
}
if _, err := page.Goto("https://www.example.com"); err != nil {
fmt.Println(err)
}
if _, err := page.Screenshot(playwright.PageScreenshotOptions{
Path: playwright.String("example.png"),
}); err != nil {
fmt.Println(err)
}
}
Fast, easy and reliable testing for anything that runs in a browser.
Pros of Cypress
- Cypress has a larger community and more active development compared to Playwright-Go.
- Cypress provides a more user-friendly and intuitive API, making it easier for developers to get started.
- Cypress has a robust set of built-in commands and utilities, reducing the need for custom implementations.
Cons of Cypress
- Cypress is primarily focused on browser-based testing, while Playwright-Go supports a wider range of browsers and platforms, including mobile.
- Cypress has a more limited set of features compared to Playwright-Go, which offers more advanced capabilities such as network interception and cross-browser testing.
Code Comparison
Cypress:
cy.visit('https://example.com')
cy.get('h1').should('have.text', 'Welcome to Example.com')
cy.get('button').click()
cy.url().should('include', '/dashboard')
cy.get('#user-profile').should('be.visible')
Playwright-Go:
browser, _ := playwright.Run()
defer browser.Close()
page, _ := browser.NewPage()
page.Goto("https://example.com")
assert.Equal(t, "Welcome to Example.com", page.Locator("h1").TextContent())
page.Click("button")
assert.True(t, strings.Contains(page.URL(), "/dashboard"))
assert.True(t, page.Locator("#user-profile").IsVisible())
JavaScript API for Chrome and Firefox
Pros of Puppeteer
- Puppeteer has a larger community and more active development, with more contributors and a wider range of plugins and tools available.
- Puppeteer has better documentation and more comprehensive examples, making it easier for new users to get started.
- Puppeteer has a more mature and stable API, with a longer track record of use in production environments.
Cons of Puppeteer
- Puppeteer is a Node.js-based library, which may not be the best fit for projects that don't use JavaScript or TypeScript.
- Puppeteer can be more resource-intensive than Playwright, as it runs a full instance of Chromium for each browser instance.
Code Comparison
Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://www.example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
Playwright:
package main
import (
"fmt"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
fmt.Println(err)
}
browser, err := pw.Chromium.Launch()
if err != nil {
fmt.Println(err)
}
page, err := browser.NewPage()
if err != nil {
fmt.Println(err)
}
if _, err := page.Goto("https://www.example.com"); err != nil {
fmt.Println(err)
}
if _, err := page.Screenshot(playwright.PageScreenshotOptions{
Path: playwright.String("example.png"),
}); err != nil {
fmt.Println(err)
}
if err := browser.Close(); err != nil {
fmt.Println(err)
}
if err := pw.Stop(); err != nil {
fmt.Println(err)
}
}
A browser automation framework and ecosystem.
Pros of Selenium
- Selenium has a larger community and more resources available, making it easier to find solutions to common problems.
- Selenium supports a wider range of browsers and platforms, including legacy browsers.
- Selenium has a more mature and stable API, with a longer history of development and support.
Cons of Selenium
- Selenium can be more complex to set up and configure, especially for beginners.
- Selenium's performance can be slower compared to newer tools like Playwright, especially for modern web applications.
- Selenium's API can be more verbose and less intuitive compared to Playwright.
Code Comparison
Selenium:
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
WebElement element = driver.findElement(By.id("my-element"));
element.click();
driver.quit();
Playwright:
browser, _ := playwright.Run()
page, _ := browser.NewPage()
page.Goto("https://www.example.com")
page.Click("#my-element")
defer browser.Close()
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Pros of Appium
- Appium supports a wide range of mobile platforms, including iOS, Android, and Windows Phone, making it a versatile choice for cross-platform mobile testing.
- Appium has a large and active community, with extensive documentation and a wealth of resources available for developers.
- Appium integrates with a variety of testing frameworks, such as Selenium, TestNG, and Cucumber, allowing for seamless integration with existing testing workflows.
Cons of Appium
- Appium can be more complex to set up and configure compared to Playwright-Go, especially for beginners.
- Appium's performance may be slower than Playwright-Go, particularly for large-scale testing scenarios.
- Appium's debugging and error reporting capabilities may not be as advanced as those of Playwright-Go.
Code Comparison
Playwright-Go:
package main
import (
"fmt"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
panic(err)
}
defer pw.Stop()
browser, err := pw.Chromium.Launch()
if err != nil {
panic(err)
}
defer browser.Close()
page, err := browser.NewPage()
if err != nil {
panic(err)
}
if _, err := page.Goto("https://www.example.com"); err != nil {
panic(err)
}
fmt.Println(page.Title())
}
Appium:
import io.appium.java_client.android.AndroidDriver;
import io.appium.java_client.remote.MobileCapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
import java.net.URL;
public class AppiumTest {
public static void main(String[] args) {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(MobileCapabilityType.PLATFORM_NAME, "Android");
capabilities.setCapability(MobileCapabilityType.DEVICE_NAME, "emulator-5554");
capabilities.setCapability(MobileCapabilityType.APP, "/path/to/your/app.apk");
AndroidDriver driver = new AndroidDriver(new URL("http://localhost:4723/wd/hub"), capabilities);
// Perform your tests here
driver.quit();
}
}
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
ð Playwright for 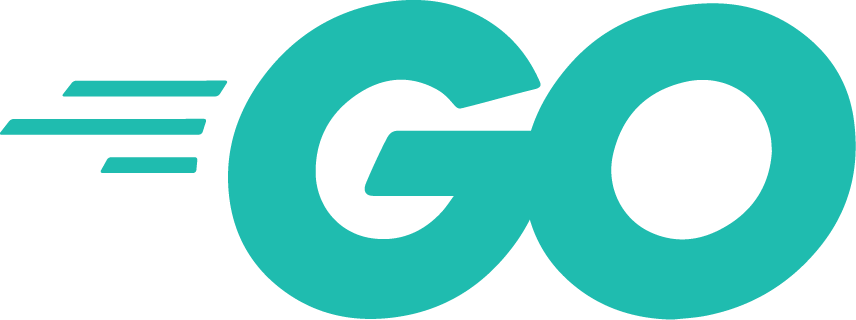
Looking for maintainers and see here. Thanks!
API reference | Example recipes
Playwright is a Go library to automate Chromium, Firefox and WebKit with a single API. Playwright is built to enable cross-browser web automation that is ever-green, capable, reliable and fast.
Linux | macOS | Windows | |
---|---|---|---|
Chromium 133.0.6943.16 | â | â | â |
WebKit 18.2 | â | â | â |
Firefox 134.0 | â | â | â |
Headless execution is supported for all the browsers on all platforms.
Installation
go get -u github.com/playwright-community/playwright-go
Install the browsers and OS dependencies:
go run github.com/playwright-community/playwright-go/cmd/playwright@latest install --with-deps
# Or
go install github.com/playwright-community/playwright-go/cmd/playwright@latest
playwright install --with-deps
Alternatively you can do it inside your program via which downloads the driver and browsers:
err := playwright.Install()
Capabilities
Playwright is built to automate the broad and growing set of web browser capabilities used by Single Page Apps and Progressive Web Apps.
- Scenarios that span multiple page, domains and iframes
- Auto-wait for elements to be ready before executing actions (like click, fill)
- Intercept network activity for stubbing and mocking network requests
- Emulate mobile devices, geolocation, permissions
- Support for web components via shadow-piercing selectors
- Native input events for mouse and keyboard
- Upload and download files
Example
The following example crawls the current top voted items from Hacker News.
package main
import (
"fmt"
"log"
"github.com/playwright-community/playwright-go"
)
func main() {
pw, err := playwright.Run()
if err != nil {
log.Fatalf("could not start playwright: %v", err)
}
browser, err := pw.Chromium.Launch()
if err != nil {
log.Fatalf("could not launch browser: %v", err)
}
page, err := browser.NewPage()
if err != nil {
log.Fatalf("could not create page: %v", err)
}
if _, err = page.Goto("https://news.ycombinator.com"); err != nil {
log.Fatalf("could not goto: %v", err)
}
entries, err := page.Locator(".athing").All()
if err != nil {
log.Fatalf("could not get entries: %v", err)
}
for i, entry := range entries {
title, err := entry.Locator("td.title > span > a").TextContent()
if err != nil {
log.Fatalf("could not get text content: %v", err)
}
fmt.Printf("%d: %s\n", i+1, title)
}
if err = browser.Close(); err != nil {
log.Fatalf("could not close browser: %v", err)
}
if err = pw.Stop(); err != nil {
log.Fatalf("could not stop Playwright: %v", err)
}
}
Docker
Refer to the Dockerfile.example to build your own docker image.
More examples
- Refer to helper_test.go for End-To-End testing
- Downloading files
- End-To-End testing a website
- Executing JavaScript in the browser
- Emulate mobile and geolocation
- Parallel scraping using a WaitGroup
- Rendering a PDF of a website
- Scraping HackerNews
- Take a screenshot
- Record a video
- Monitor network activity
How does it work?
Playwright is a Node.js library which uses:
- Chrome DevTools Protocol to communicate with Chromium
- Patched Firefox to communicate with Firefox
- Patched WebKit to communicate with WebKit
These patches are based on the original sources of the browsers and don't modify the browser behaviour so the browsers are basically the same (see here) as you see them in the wild. The support for different programming languages is based on exposing a RPC server in the Node.js land which can be used to allow other languages to use Playwright without implementing all the custom logic:
The bridge between Node.js and the other languages is basically a Node.js runtime combined with Playwright which gets shipped for each of these languages (around 50MB) and then communicates over stdio to send the relevant commands. This will also download the pre-compiled browsers.
Is Playwright for Go ready?
We are ready for your feedback, but we are still covering Playwright Go with the tests.
Resources
Top Related Projects
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Fast, easy and reliable testing for anything that runs in a browser.
JavaScript API for Chrome and Firefox
A browser automation framework and ecosystem.
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot