Top Related Projects
GPL version of Javascript Gantt Chart
Gantt Gantt Gantt Timeline Schedule Calendar [ javascript gantt, js gantt, projects gantt, timeline, scheduler, gantt timeline, reservation timeline, react gantt, angular gantt, vue gantt, svelte gantt, booking manager ]
🍞📅A JavaScript calendar that has everything you need.
A datepicker for twitter bootstrap (@twbs)
Quick Overview
jQueryGantt is a jQuery plugin that allows developers to create interactive Gantt charts for project management and scheduling. It provides a feature-rich, customizable interface for visualizing tasks, dependencies, and timelines in a user-friendly manner.
Pros
- Highly customizable with numerous configuration options
- Supports task dependencies and critical path calculation
- Includes resource management and workload visualization
- Offers export functionality to various formats (PNG, PDF, XML)
Cons
- Relies on jQuery, which may not be ideal for modern web applications
- Limited documentation and examples for advanced use cases
- May require additional styling efforts for seamless integration with modern UI frameworks
- Performance can degrade with large datasets
Code Examples
- Basic Gantt chart initialization:
$("#gantt").gantt({
source: [
{
name: "Task 1",
desc: "Description",
values: [{
from: "/Date(1320192000000)/",
to: "/Date(1322401600000)/",
label: "Task 1",
customClass: "ganttRed"
}]
}
]
});
- Adding dependencies between tasks:
$("#gantt").gantt({
source: [
{
name: "Task 1",
desc: "Description",
values: [{
from: "/Date(1320192000000)/",
to: "/Date(1322401600000)/",
label: "Task 1"
}]
},
{
name: "Task 2",
desc: "Depends on Task 1",
depends: "Task 1",
values: [{
from: "/Date(1322401600000)/",
to: "/Date(1323802800000)/",
label: "Task 2"
}]
}
]
});
- Enabling resource management:
$("#gantt").gantt({
source: [
{
name: "Task 1",
desc: "Description",
values: [{
from: "/Date(1320192000000)/",
to: "/Date(1322401600000)/",
label: "Task 1"
}]
}
],
resources: [
{ id: 1, name: "Resource 1" },
{ id: 2, name: "Resource 2" }
],
resourceAssignments: [
{ taskId: "Task 1", resourceId: 1, value: 100 }
]
});
Getting Started
- Include jQuery and jQueryGantt files in your HTML:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="path/to/jquery.gantt.min.js"></script>
<link rel="stylesheet" href="path/to/jquery.gantt.min.css">
- Create a container element in your HTML:
<div id="gantt"></div>
- Initialize the Gantt chart with JavaScript:
$(document).ready(function() {
$("#gantt").gantt({
source: [
{
name: "Task 1",
desc: "Description",
values: [{
from: "/Date(1320192000000)/",
to: "/Date(1322401600000)/",
label: "Task 1"
}]
}
]
});
});
Competitor Comparisons
GPL version of Javascript Gantt Chart
Pros of DHTMLX Gantt
- More feature-rich and customizable
- Better documentation and support
- Regular updates and active development
Cons of DHTMLX Gantt
- Commercial license required for most use cases
- Steeper learning curve due to more complex API
Code Comparison
jQueryGantt:
var g = new JSGantt.GanttChart(document.getElementById('GanttChartDIV'), 'week');
g.setShowRes(1);
g.setShowDur(1);
g.setShowComp(1);
g.Draw();
DHTMLX Gantt:
gantt.init("gantt_here");
gantt.parse({
data: [
{id: 1, text: "Task #1", start_date: "2023-05-01", duration: 3, progress: 0.6},
{id: 2, text: "Task #2", start_date: "2023-05-03", duration: 3, progress: 0.4}
]
});
Both libraries offer Gantt chart functionality, but DHTMLX Gantt provides a more comprehensive solution with advanced features and better documentation. However, it comes at the cost of a commercial license and a steeper learning curve. jQueryGantt is simpler to use and free, but may lack some advanced features and ongoing support.
Gantt Gantt Gantt Timeline Schedule Calendar [ javascript gantt, js gantt, projects gantt, timeline, scheduler, gantt timeline, reservation timeline, react gantt, angular gantt, vue gantt, svelte gantt, booking manager ]
Pros of gantt-schedule-timeline-calendar
- More modern and feature-rich, with support for various view modes (timeline, schedule, calendar)
- Better performance and scalability for large datasets
- Active development and regular updates
Cons of gantt-schedule-timeline-calendar
- Steeper learning curve due to more complex API and configuration options
- Requires more setup and customization to achieve desired functionality
- Larger file size and potentially higher resource usage
Code Comparison
jQueryGantt:
var g = new JSGantt.GanttChart(document.getElementById('GanttChartDIV'), 'week');
g.AddTaskItem(new JSGantt.TaskItem(1, 'Task 1', '2023-05-01', '2023-05-07', 'ff0000', '', 0, 'John', 0, 1, 0, 1));
g.Draw();
gantt-schedule-timeline-calendar:
const gstc = GSTC({
element: document.getElementById('gstc'),
config: {
list: { rows: [{ id: '1', label: 'Task 1' }] },
chart: { items: [{ id: '1', rowId: '1', time: { start: '2023-05-01', end: '2023-05-07' } }] }
}
});
Both libraries offer Gantt chart functionality, but gantt-schedule-timeline-calendar provides more advanced features and flexibility at the cost of increased complexity. jQueryGantt is simpler to set up and use, making it suitable for basic Gantt chart needs, while gantt-schedule-timeline-calendar is better suited for complex project management applications requiring advanced scheduling and visualization capabilities.
🍞📅A JavaScript calendar that has everything you need.
Pros of tui.calendar
- More comprehensive calendar functionality, including day, week, and month views
- Actively maintained with regular updates and bug fixes
- Extensive documentation and examples
Cons of tui.calendar
- Steeper learning curve due to more complex API
- Larger file size and potentially higher resource usage
Code Comparison
tui.calendar:
import Calendar from '@toast-ui/calendar';
const calendar = new Calendar('#calendar', {
defaultView: 'week',
template: {
time: function(schedule) {
return moment(schedule.start.getTime()).format('HH:mm');
}
}
});
jQueryGantt:
$("#gantt").gantt({
source: [
{name: "Task 1", desc: "Description", values: [{from: "/Date(1320192000000)/", to: "/Date(1322401600000)/", label: "Label", customClass: "ganttRed"}]}
],
scale: "weeks",
minScale: "days",
maxScale: "months"
});
Summary
tui.calendar offers a more feature-rich calendar solution with active maintenance and comprehensive documentation. However, it may be more complex to implement and have a larger footprint. jQueryGantt is simpler and more focused on Gantt chart functionality, making it easier to use for specific project management tasks but potentially limiting for broader calendar applications.
A datepicker for twitter bootstrap (@twbs)
Pros of bootstrap-datepicker
- More focused and specialized for date picking functionality
- Extensive documentation and examples available
- Wider community adoption and regular updates
Cons of bootstrap-datepicker
- Limited to date selection, lacks project management features
- May require additional plugins for more complex date-related tasks
Code Comparison
bootstrap-datepicker:
$('#datepicker').datepicker({
format: 'mm/dd/yyyy',
startDate: '-3d',
autoclose: true
});
jQueryGantt:
$(".gantt").gantt({
source: tasks,
navigate: 'scroll',
scale: 'weeks',
maxScale: 'months',
minScale: 'days'
});
Summary
While bootstrap-datepicker excels in providing a robust date selection tool, jQueryGantt offers a more comprehensive solution for project management and timeline visualization. bootstrap-datepicker is ideal for applications requiring simple date input, whereas jQueryGantt is better suited for complex project scheduling and Gantt chart creation. The code examples demonstrate the different focus areas, with bootstrap-datepicker configuring date selection options and jQueryGantt setting up a full Gantt chart with various parameters.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
jQueryGantt
jQuery Gantt editor has been written by Roberto Bicchierai and Silvia Chelazzi
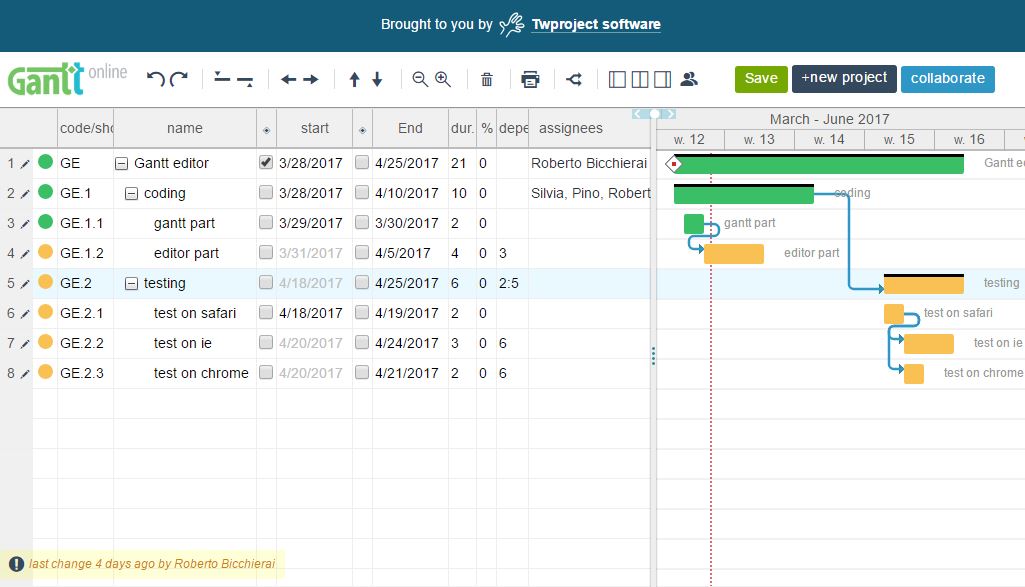
These are some key features:
- jQuery based 3.2
- MIT licensed: you can reuse everywhere https://opensource.org/licenses/MIT
- JSON import-export
- internationalizable
- manage task status â> project workflow
- manage dependencies
- manage assignments (resources, roles efforts)
- server synchronization ready
- full undo-redo support
- cross browser (at least for recent versions)
- keyboard editing support
- SVG visual editor
- print friendly
- collapsible branches
- critical path
- milestones, progress etc.
- zoom
Try the online working demo here: https://gantt.twproject.com
Read here about latest release: https://roberto.twproject.com/2017/04/05/new-gantt-editor-release-the-best-ever/
Read the genesis of this component here: http://roberto.twproject.com/2012/06/14/the-javascript-gantt-odyssey/
Documentation is here: http://roberto.twproject.com/2012/08/24/jquery-gantt-editor/
jQuery Gantt editor is part of Twproject 6 project
Top Related Projects
GPL version of Javascript Gantt Chart
Gantt Gantt Gantt Timeline Schedule Calendar [ javascript gantt, js gantt, projects gantt, timeline, scheduler, gantt timeline, reservation timeline, react gantt, angular gantt, vue gantt, svelte gantt, booking manager ]
🍞📅A JavaScript calendar that has everything you need.
A datepicker for twitter bootstrap (@twbs)
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot