react-native-gesture-handler
Declarative API exposing platform native touch and gesture system to React Native.
Top Related Projects
React Native's Animated library reimplemented
Experimental implementation of high performance interactable views in React Native
A complete native navigation solution for React Native
React Native Mapview component for iOS + Android
Material Design for React Native (Android & iOS)
Quick Overview
React Native Gesture Handler is a declarative API for handling complex gestures and touch interactions in React Native applications. It provides a more powerful and customizable alternative to the built-in touch system, allowing developers to create smooth, native-like gesture experiences across both iOS and Android platforms.
Pros
- Improved performance compared to React Native's built-in gesture system
- Supports a wide range of gestures, including pan, pinch, rotation, and more
- Provides a unified API for both iOS and Android platforms
- Allows for complex gesture compositions and interactions
Cons
- Steeper learning curve compared to React Native's built-in touch system
- May require additional setup and configuration
- Some advanced features might not be fully supported on all devices or OS versions
- Documentation can be overwhelming for beginners
Code Examples
- Basic tap gesture:
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
const tap = Gesture.Tap()
.onStart(() => {
console.log('Tap gesture started');
})
.onEnd(() => {
console.log('Tap gesture ended');
});
return (
<GestureDetector gesture={tap}>
<View style={styles.box} />
</GestureDetector>
);
- Pan gesture with animation:
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
import Animated, { useSharedValue, useAnimatedStyle } from 'react-native-reanimated';
const translateX = useSharedValue(0);
const translateY = useSharedValue(0);
const pan = Gesture.Pan()
.onUpdate((event) => {
translateX.value = event.translationX;
translateY.value = event.translationY;
});
const animatedStyle = useAnimatedStyle(() => ({
transform: [
{ translateX: translateX.value },
{ translateY: translateY.value },
],
}));
return (
<GestureDetector gesture={pan}>
<Animated.View style={[styles.box, animatedStyle]} />
</GestureDetector>
);
- Pinch gesture:
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
const pinch = Gesture.Pinch()
.onUpdate((event) => {
console.log('Scale:', event.scale);
});
return (
<GestureDetector gesture={pinch}>
<View style={styles.container} />
</GestureDetector>
);
Getting Started
-
Install the library:
npm install react-native-gesture-handler
-
For iOS, run:
cd ios && pod install
-
Wrap your app's root component with
GestureHandlerRootView
:
import { GestureHandlerRootView } from 'react-native-gesture-handler';
export default function App() {
return (
<GestureHandlerRootView style={{ flex: 1 }}>
{/* Your app content */}
</GestureHandlerRootView>
);
}
- Start using gestures in your components as shown in the code examples above.
Competitor Comparisons
React Native's Animated library reimplemented
Pros of react-native-reanimated
- More powerful and flexible animation system
- Better performance for complex animations
- Supports declarative animation definitions
Cons of react-native-reanimated
- Steeper learning curve
- Requires additional setup and configuration
- May be overkill for simple animations
Code Comparison
react-native-reanimated:
import Animated, { useAnimatedStyle, useSharedValue, withSpring } from 'react-native-reanimated';
const animatedStyles = useAnimatedStyle(() => {
return {
transform: [{ translateX: withSpring(offset.value) }],
};
});
react-native-gesture-handler:
import { PanGestureHandler } from 'react-native-gesture-handler';
<PanGestureHandler
onGestureEvent={onGestureEvent}
onHandlerStateChange={onHandlerStateChange}
>
<Animated.View style={animatedStyles} />
</PanGestureHandler>
react-native-reanimated focuses on creating complex animations with a more declarative approach, while react-native-gesture-handler specializes in handling gestures and touch events. react-native-reanimated offers more advanced animation capabilities but requires a deeper understanding of its concepts. react-native-gesture-handler is simpler to use for basic gesture handling but may be less flexible for complex animations. Both libraries can be used together to create rich, interactive user interfaces in React Native applications.
Experimental implementation of high performance interactable views in React Native
Pros of react-native-interactable
- Provides high-level, ready-to-use interactive components
- Offers smoother animations and physics-based interactions
- Easier to implement complex gestures with less code
Cons of react-native-interactable
- Less actively maintained compared to react-native-gesture-handler
- Limited customization options for advanced use cases
- Smaller community and fewer resources available
Code Comparison
react-native-interactable:
<Interactable.View
snapPoints={[{x: 0}, {x: 200}]}
dragEnabled={true}
onSnap={this.onSnap}>
<View style={styles.box} />
</Interactable.View>
react-native-gesture-handler:
<PanGestureHandler
onGestureEvent={this.onPanGestureEvent}
onHandlerStateChange={this.onPanHandlerStateChange}>
<Animated.View style={[styles.box, {transform: [{translateX: this.translateX}]}]} />
</PanGestureHandler>
react-native-interactable provides a more declarative API with built-in snap points and physics, while react-native-gesture-handler offers lower-level control over gestures and animations. The latter requires more setup but provides greater flexibility for custom interactions.
A complete native navigation solution for React Native
Pros of React Native Navigation
- Provides a complete navigation solution with native performance
- Offers extensive customization options for navigation UI
- Supports complex navigation patterns like tabs, drawers, and modals
Cons of React Native Navigation
- Steeper learning curve due to its comprehensive nature
- May require more setup and configuration compared to simpler solutions
- Can be overkill for projects with basic navigation needs
Code Comparison
React Native Navigation:
Navigation.setRoot({
root: {
stack: {
children: [
{ component: { name: 'Home' } }
]
}
}
});
React Native Gesture Handler:
import { PanGestureHandler } from 'react-native-gesture-handler';
<PanGestureHandler
onGestureEvent={this.onPanGestureEvent}
onHandlerStateChange={this.onHandlerStateChange}>
<Animated.View style={animatedStyles} />
</PanGestureHandler>
While React Native Navigation focuses on providing a comprehensive navigation solution, React Native Gesture Handler specializes in handling touch and gesture interactions. The code examples demonstrate their different purposes: React Native Navigation sets up a navigation structure, while React Native Gesture Handler implements gesture recognition for UI elements.
React Native Mapview component for iOS + Android
Pros of react-native-maps
- Specialized for map functionality, offering a wide range of map-related features
- Integrates well with popular map providers like Google Maps and Apple Maps
- Extensive documentation and community support for map-specific use cases
Cons of react-native-maps
- Limited to map-related gestures and interactions
- May require additional setup and API keys for map providers
- Potentially larger bundle size due to map-specific dependencies
Code Comparison
react-native-maps:
import MapView, { Marker } from 'react-native-maps';
<MapView
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
>
<Marker coordinate={{ latitude: 37.78825, longitude: -122.4324 }} />
</MapView>
react-native-gesture-handler:
import { PanGestureHandler } from 'react-native-gesture-handler';
<PanGestureHandler
onGestureEvent={event => {
console.log(event.nativeEvent);
}}
>
<View style={{ width: 100, height: 100, backgroundColor: 'blue' }} />
</PanGestureHandler>
While react-native-maps focuses on map-specific functionality, react-native-gesture-handler provides a more general-purpose gesture handling system for React Native applications. The choice between the two depends on the specific requirements of your project, with react-native-maps being ideal for map-centric apps and react-native-gesture-handler offering more flexibility for custom gesture interactions across various UI elements.
Material Design for React Native (Android & iOS)
Pros of React Native Paper
- Comprehensive UI component library specifically designed for React Native
- Follows Material Design guidelines, ensuring a consistent and modern look
- Offers a wide range of pre-built components, reducing development time
Cons of React Native Paper
- Limited to Material Design aesthetics, which may not suit all app designs
- Potentially larger bundle size due to the extensive component library
- Less focused on gesture handling compared to React Native Gesture Handler
Code Comparison
React Native Paper (Button component):
import { Button } from 'react-native-paper';
<Button mode="contained" onPress={() => console.log('Pressed')}>
Press me
</Button>
React Native Gesture Handler (Gesture handling):
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
const tap = Gesture.Tap().onStart(() => {
console.log('Tap gesture detected');
});
<GestureDetector gesture={tap}>
<View style={styles.box} />
</GestureDetector>
While React Native Paper focuses on providing ready-to-use UI components, React Native Gesture Handler specializes in advanced gesture recognition and handling. The choice between the two depends on whether you need a comprehensive UI library or more control over gesture interactions in your React Native application.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
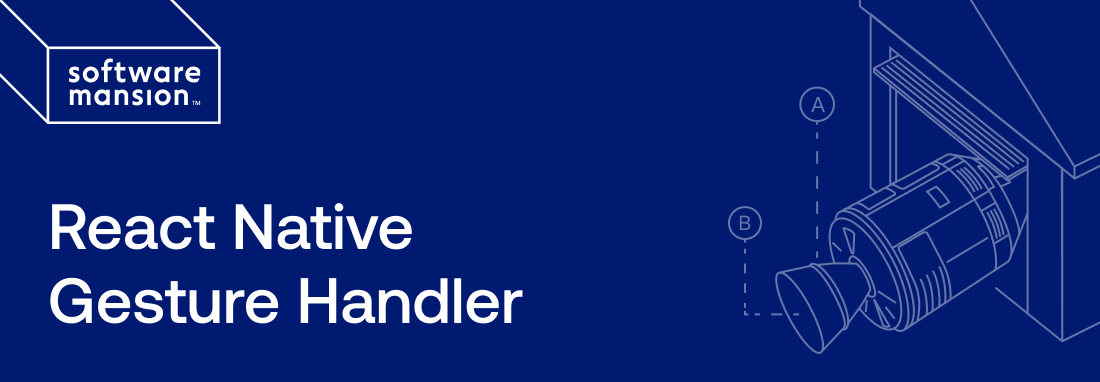
Declarative API exposing platform native touch and gesture system to React Native.
React Native Gesture Handler provides native-driven gesture management APIs for building best possible touch-based experiences in React Native.
With this library gestures are no longer controlled by the JS responder system, but instead are recognized and tracked in the UI thread. It makes touch interactions and gesture tracking not only smooth, but also dependable and deterministic.
Installation
Check getting started section of our docs for the detailed installation instructions.
Fabric
To learn how to use react-native-gesture-handler
with Fabric architecture, head over to Fabric README. Instructions on how to run Fabric Example within this repo can be found in the FabricExample README.
Documentation
Check out our dedicated documentation page for info about this library, API reference and more: https://docs.swmansion.com/react-native-gesture-handler/docs/
Examples
If you want to play with the API but don't feel like trying it on a real app, you can run the example project. Clone the repo, go to the example
folder and run:
yarn install
Run yarn start
to start the metro bundler
Run yarn android
or yarn ios
(depending on which platform you want to run the example app on).
You will need to have an Android or iOS device or emulator connected.
React Native Support
version | react-native version |
---|---|
2.18.0+ | 0.73.0+ |
2.16.0+ | 0.68.0+ |
2.14.0+ | 0.67.0+ |
2.10.0+ | 0.64.0+ |
2.0.0+ | 0.63.0+ |
1.4.0+ | 0.60.0+ |
1.1.0+ | 0.57.2+ |
<1.1.0 | 0.50.0+ |
It may be possible to use newer versions of react-native-gesture-handler on React Native with version <= 0.59 by reverse Jetifying. Read more on that here https://github.com/mikehardy/jetifier#to-reverse-jetify--convert-node_modules-dependencies-to-support-libraries
License
Gesture handler library is licensed under The MIT License.
Credits
This project has been build and is maintained thanks to the support from Shopify, Expo.io and Software Mansion
Community Discord
Join the Software Mansion Community Discord to chat about Gesture Handler or other Software Mansion libraries.
Gesture Handler is created by Software Mansion
Since 2012 Software Mansion is a software agency with experience in building web and mobile apps. We are Core React Native Contributors and experts in dealing with all kinds of React Native issues. We can help you build your next dream product â Hire us.
Top Related Projects
React Native's Animated library reimplemented
Experimental implementation of high performance interactable views in React Native
A complete native navigation solution for React Native
React Native Mapview component for iOS + Android
Material Design for React Native (Android & iOS)
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot