Top Related Projects
Declarative API exposing platform native touch and gesture system to React Native.
Experimental implementation of high performance interactable views in React Native
The React Native Reanimated and Gesture Handler Toolbelt
SVG library for React Native, React Native Web, and plain React web projects.
Quick Overview
React Native Reanimated is a powerful animation library for React Native that provides a more flexible and performant way to create smooth, gesture-based animations. It allows developers to define complex animations using a declarative API and runs them on the native thread for optimal performance.
Pros
- High performance due to running animations on the native thread
- Declarative API for creating complex animations
- Seamless integration with React Native's Gesture Handler
- Extensive set of pre-built animations and transitions
Cons
- Steeper learning curve compared to React Native's built-in Animated API
- Requires additional setup and configuration
- Some features may not be fully compatible with older React Native versions
- Debugging can be more challenging due to native thread execution
Code Examples
- Basic opacity animation:
import Animated, { useAnimatedStyle, useSharedValue, withTiming } from 'react-native-reanimated';
function FadeInView() {
const opacity = useSharedValue(0);
const animatedStyle = useAnimatedStyle(() => {
return {
opacity: opacity.value,
};
});
React.useEffect(() => {
opacity.value = withTiming(1, { duration: 1000 });
}, []);
return <Animated.View style={[styles.box, animatedStyle]} />;
}
- Gesture-based animation:
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
import Animated, { useAnimatedStyle, useSharedValue } from 'react-native-reanimated';
function DraggableBox() {
const translateX = useSharedValue(0);
const translateY = useSharedValue(0);
const gesture = Gesture.Pan()
.onUpdate((event) => {
translateX.value = event.translationX;
translateY.value = event.translationY;
});
const animatedStyle = useAnimatedStyle(() => {
return {
transform: [
{ translateX: translateX.value },
{ translateY: translateY.value },
],
};
});
return (
<GestureDetector gesture={gesture}>
<Animated.View style={[styles.box, animatedStyle]} />
</GestureDetector>
);
}
- Interpolation example:
import Animated, { useAnimatedScrollHandler, useAnimatedStyle, useSharedValue, interpolate } from 'react-native-reanimated';
function AnimatedHeader() {
const scrollY = useSharedValue(0);
const scrollHandler = useAnimatedScrollHandler({
onScroll: (event) => {
scrollY.value = event.contentOffset.y;
},
});
const headerStyle = useAnimatedStyle(() => {
const height = interpolate(
scrollY.value,
[0, 200],
[200, 100],
Animated.Extrapolate.CLAMP
);
return {
height,
};
});
return (
<Animated.View style={[styles.header, headerStyle]}>
<Animated.ScrollView onScroll={scrollHandler} scrollEventThrottle={16}>
{/* Content */}
</Animated.ScrollView>
</Animated.View>
);
}
Getting Started
-
Install the library:
npm install react-native-reanimated
-
Add Reanimated's babel plugin to your
babel.config.js
:module.exports = { presets: ['module:metro-react-native-babel-preset'], plugins: ['react-native-reanimated/plugin'], };
-
For iOS, install pods:
cd ios && pod install
-
Rebuild your app and start using Reanimated in your components:
import Animated from 'react-native-reanimated';
Competitor Comparisons
Declarative API exposing platform native touch and gesture system to React Native.
Pros of react-native-gesture-handler
- Specialized for handling complex touch interactions and gestures
- Provides more precise control over touch events and their propagation
- Offers a wide range of pre-built gesture recognizers (e.g., pan, pinch, rotation)
Cons of react-native-gesture-handler
- Primarily focused on gesture handling, limiting its scope compared to Reanimated's broader animation capabilities
- May require additional setup and configuration for certain gesture types
- Learning curve can be steeper for developers new to gesture-based interactions
Code Comparison
react-native-gesture-handler:
import { PanGestureHandler, State } from 'react-native-gesture-handler';
<PanGestureHandler
onGestureEvent={this._onPanGestureEvent}
onHandlerStateChange={this._onPanHandlerStateChange}>
<Animated.View style={styles.box} />
</PanGestureHandler>
react-native-reanimated:
import Animated, { useAnimatedGestureHandler } from 'react-native-reanimated';
const gestureHandler = useAnimatedGestureHandler({
onStart: (_, ctx) => { ctx.startY = _.y; },
onActive: (event, ctx) => {
translateY.value = event.translationY + ctx.startY;
},
});
While both libraries can handle gestures, react-native-gesture-handler provides more specialized gesture recognition, while react-native-reanimated offers a broader range of animation capabilities with integrated gesture handling.
Experimental implementation of high performance interactable views in React Native
Pros of react-native-interactable
- Specialized for interactive animations and gestures
- Simpler API for common interaction patterns
- Better performance for specific use cases like draggable elements
Cons of react-native-interactable
- Less actively maintained compared to react-native-reanimated
- More limited in scope and flexibility
- Smaller community and ecosystem
Code Comparison
react-native-interactable:
<Interactable.View
snapPoints={[{x: 0}, {x: 200}]}
dragEnabled={true}
onSnap={this.onSnap}>
<View style={styles.box} />
</Interactable.View>
react-native-reanimated:
const animatedStyle = useAnimatedStyle(() => {
return {
transform: [{ translateX: withSpring(position.value * 200) }],
};
});
return <Animated.View style={[styles.box, animatedStyle]} />;
react-native-interactable provides a more declarative approach for common interactions, while react-native-reanimated offers greater flexibility and control over animations. react-native-reanimated has a steeper learning curve but allows for more complex and customized animations. react-native-interactable is easier to use for specific interaction patterns but is less versatile overall.
The React Native Reanimated and Gesture Handler Toolbelt
Pros of react-native-redash
- Provides a higher-level abstraction for common animation patterns
- Includes utility functions for vector operations and color manipulations
- Offers a more declarative API for complex animations
Cons of react-native-redash
- Less flexible for highly custom animations
- Depends on react-native-reanimated, adding an extra layer of complexity
- May have a steeper learning curve for developers new to React Native animations
Code Comparison
react-native-redash:
const animatedStyles = useAnimatedStyle(() => {
return {
transform: [{ translateY: withSpring(translation.value) }],
};
});
react-native-reanimated:
const animatedStyles = useAnimatedStyle(() => {
return {
transform: [{ translateY: withSpring(translation.value) }],
};
});
While the code snippets look similar, react-native-redash often provides additional utility functions and hooks that simplify complex animations. However, for basic animations like this example, the code may appear nearly identical.
react-native-redash builds upon react-native-reanimated, offering a more opinionated and higher-level API. It's particularly useful for developers who want to quickly implement common animation patterns without diving deep into the intricacies of react-native-reanimated. However, for those requiring maximum flexibility and control over animations, react-native-reanimated remains the more powerful option.
SVG library for React Native, React Native Web, and plain React web projects.
Pros of react-native-svg
- Specialized for SVG rendering, offering a comprehensive set of SVG elements and attributes
- Lightweight and focused, ideal for projects primarily needing SVG support
- Easier to use for simple SVG implementations without animation complexities
Cons of react-native-svg
- Limited animation capabilities compared to react-native-reanimated's powerful animation system
- Lacks the broader scope of UI interactions and gestures that react-native-reanimated provides
- May require additional libraries for complex animations or interactions
Code Comparison
react-native-svg:
import { Svg, Circle } from 'react-native-svg';
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
</Svg>
react-native-reanimated:
import Animated, { useAnimatedStyle, withSpring } from 'react-native-reanimated';
const animatedStyle = useAnimatedStyle(() => {
return { transform: [{ scale: withSpring(1.2) }] };
});
<Animated.View style={[styles.box, animatedStyle]} />
This comparison highlights the specialized nature of react-native-svg for SVG rendering, while react-native-reanimated offers more comprehensive animation and interaction capabilities for general UI elements.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
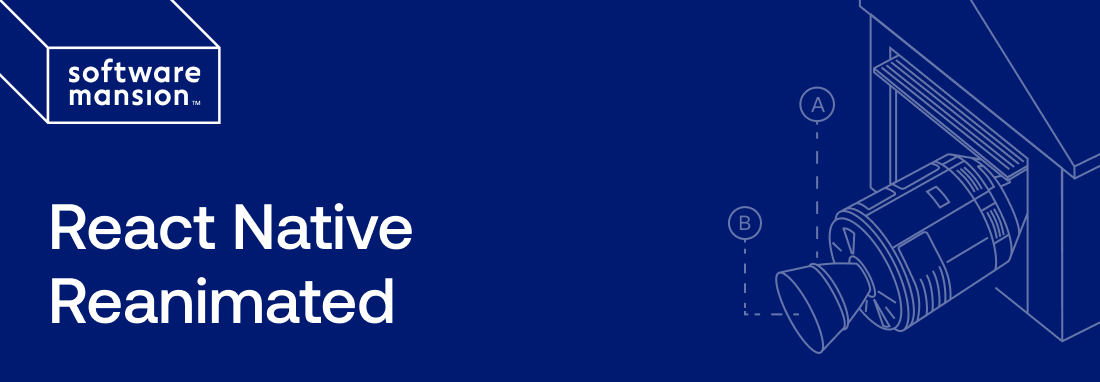
Create smooth animations with an excellent developer experience.
Reanimated 4 is here! Check out our documentation page for more information
Nightly CI state
Installation
Check out the installation section of our docs for the detailed installation instructions.
Compatibility
React Native Reanimated 4.x supports only the New React Native architecture and three latest React Native versions.
If your app still runs on the old architecture, please consider adopting the New Architecture or stay with latest 3.x release.
Documentation
Check out our dedicated documentation page for info about this library, API reference and more: https://docs.swmansion.com/react-native-reanimated/
Examples
The source code for the example (showcase) app is under the apps/common-app
directory.
If you want to play with the API but don't feel like trying it on a real app, you can run the example project. Check Example README for installation instructions.
License
Reanimated library is licensed under The MIT License.
Credits
This project has been built and is maintained thanks to the support from Shopify, Expo.io and Software Mansion
Community Discord
Join the Software Mansion Community Discord to chat about Reanimated or other Software Mansion libraries.
Reanimated is created by Software Mansion
Since 2012 Software Mansion is a software agency with experience in building web and mobile apps. We are Core React Native Contributors and experts in dealing with all kinds of React Native issues. We can help you build your next dream product â Hire us.
Top Related Projects
Declarative API exposing platform native touch and gesture system to React Native.
Experimental implementation of high performance interactable views in React Native
The React Native Reanimated and Gesture Handler Toolbelt
SVG library for React Native, React Native Web, and plain React web projects.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot