react-native-svg
SVG library for React Native, React Native Web, and plain React web projects.
Top Related Projects
Lottie wrapper for React Native.
A <LinearGradient /> component for react-native
React Native Cross-Platform WebView
React Native Mapview component for iOS + Android
A Camera component for React Native. Also supports barcode scanning!
UI Components Library for React Native
Quick Overview
React Native SVG is a powerful library that brings SVG support to React Native applications. It allows developers to easily render and manipulate SVG graphics within their mobile apps, providing a seamless way to work with vector graphics across different platforms.
Pros
- Comprehensive SVG support, including most SVG elements and attributes
- High performance and optimized rendering
- Seamless integration with React Native's layout system
- Active development and community support
Cons
- Learning curve for developers unfamiliar with SVG
- Some advanced SVG features may not be fully supported
- Potential performance issues with complex SVG animations
- Occasional compatibility issues with certain React Native versions
Code Examples
- Basic SVG rendering:
import Svg, { Circle, Rect } from 'react-native-svg';
function BasicShapes() {
return (
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
<Rect x="15" y="15" width="70" height="70" stroke="red" strokeWidth="2" fill="yellow" />
</Svg>
);
}
- Animated SVG:
import Svg, { Circle } from 'react-native-svg';
import { Animated, Easing } from 'react-native';
function AnimatedCircle() {
const animatedValue = new Animated.Value(0);
Animated.loop(
Animated.timing(animatedValue, {
toValue: 1,
duration: 2000,
easing: Easing.linear,
useNativeDriver: true,
})
).start();
return (
<Svg height="100" width="100">
<AnimatedCircle
cx="50"
cy="50"
r="45"
stroke="blue"
strokeWidth="2.5"
fill="green"
opacity={animatedValue}
/>
</Svg>
);
}
- SVG with gradients:
import Svg, { Defs, LinearGradient, Stop, Rect } from 'react-native-svg';
function GradientExample() {
return (
<Svg height="150" width="300">
<Defs>
<LinearGradient id="grad" x1="0" y1="0" x2="1" y2="0">
<Stop offset="0" stopColor="rgb(255,0,0)" stopOpacity="0.5" />
<Stop offset="1" stopColor="rgb(0,0,255)" stopOpacity="1" />
</LinearGradient>
</Defs>
<Rect x="10" y="10" width="280" height="130" fill="url(#grad)" stroke="black" strokeWidth="1" />
</Svg>
);
}
Getting Started
-
Install the library:
npm install react-native-svg
-
For iOS, run:
cd ios && pod install
-
Import and use SVG components in your React Native app:
import Svg, { Circle, Rect } from 'react-native-svg'; function MyComponent() { return ( <Svg height="100" width="100"> <Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" /> <Rect x="15" y="15" width="70" height="70" stroke="red" strokeWidth="2" fill="yellow" /> </Svg> ); }
Competitor Comparisons
Lottie wrapper for React Native.
Pros of Lottie React Native
- Supports complex animations with keyframes and effects
- Smaller file sizes for intricate animations
- Easier to create and edit animations using design tools like Adobe After Effects
Cons of Lottie React Native
- Limited to pre-designed animations; less flexibility for runtime modifications
- May have performance issues with very complex animations on low-end devices
- Steeper learning curve for designers to create custom animations
Code Comparison
React Native SVG:
import Svg, { Circle, Rect } from 'react-native-svg';
function SvgExample() {
return (
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
<Rect x="15" y="15" width="70" height="70" stroke="red" strokeWidth="2" fill="yellow" />
</Svg>
);
}
Lottie React Native:
import LottieView from 'lottie-react-native';
function LottieExample() {
return (
<LottieView
source={require('./animation.json')}
autoPlay
loop
/>
);
}
The React Native SVG example shows direct creation of vector graphics, while the Lottie example demonstrates loading a pre-designed animation file. React Native SVG offers more programmatic control, whereas Lottie provides a simpler API for complex, pre-built animations.
A <LinearGradient /> component for react-native
Pros of react-native-linear-gradient
- Simpler API for creating linear gradients
- Lightweight and focused on a single task
- Easier to implement for basic gradient needs
Cons of react-native-linear-gradient
- Limited to linear gradients only
- Less flexibility in terms of customization
- Fewer options for complex designs compared to SVG
Code Comparison
react-native-linear-gradient:
import LinearGradient from 'react-native-linear-gradient';
<LinearGradient
colors={['#4c669f', '#3b5998', '#192f6a']}
style={styles.linearGradient}
>
<Text style={styles.buttonText}>Sign in with Facebook</Text>
</LinearGradient>
react-native-svg:
import Svg, { Defs, LinearGradient, Stop, Rect } from 'react-native-svg';
<Svg height="150" width="300">
<Defs>
<LinearGradient id="grad" x1="0" y1="0" x2="1" y2="0">
<Stop offset="0" stopColor="#4c669f" stopOpacity="1" />
<Stop offset="1" stopColor="#192f6a" stopOpacity="1" />
</LinearGradient>
</Defs>
<Rect x="0" y="0" width="300" height="150" fill="url(#grad)" />
</Svg>
react-native-linear-gradient is more straightforward for simple linear gradients, while react-native-svg offers greater flexibility and control over gradient creation, as well as support for other SVG elements and more complex designs.
React Native Cross-Platform WebView
Pros of react-native-webview
- Versatile: Can render any web content, including complex HTML, CSS, and JavaScript
- Familiar: Developers with web experience can easily create content
- Feature-rich: Supports file uploads, geolocation, and other web APIs
Cons of react-native-webview
- Performance: Generally slower than native components, especially for animations
- Memory usage: Can be resource-intensive, particularly with complex web content
- Limited native integration: Interaction with native components can be challenging
Code comparison
react-native-webview:
import { WebView } from 'react-native-webview';
<WebView
source={{ uri: 'https://example.com' }}
style={{ flex: 1 }}
/>
react-native-svg:
import Svg, { Circle } from 'react-native-svg';
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
</Svg>
react-native-webview is ideal for rendering web content and leveraging existing web skills, while react-native-svg excels at creating and manipulating vector graphics with better performance and native feel. Choose based on your specific needs and performance requirements.
React Native Mapview component for iOS + Android
Pros of react-native-maps
- Specialized for map-related functionalities, offering a wide range of map-specific features
- Integrates seamlessly with popular map providers like Google Maps and Apple Maps
- Provides built-in support for markers, polygons, and other map overlays
Cons of react-native-maps
- Limited to map-specific use cases, not suitable for general SVG rendering
- May have a larger bundle size due to map-specific dependencies
- Potentially more complex setup process, especially when configuring map provider APIs
Code Comparison
react-native-maps:
import MapView, { Marker } from 'react-native-maps';
<MapView
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
>
<Marker coordinate={{ latitude: 37.78825, longitude: -122.4324 }} />
</MapView>
react-native-svg:
import Svg, { Circle } from 'react-native-svg';
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
</Svg>
This comparison highlights the specialized nature of react-native-maps for map-related tasks, while react-native-svg offers more flexibility for general SVG rendering. The code examples demonstrate the different approaches and use cases for each library.
A Camera component for React Native. Also supports barcode scanning!
Pros of react-native-camera
- Specialized for camera functionality, offering a wide range of camera-related features
- Supports both photo and video capture, as well as barcode scanning
- Provides access to device-specific camera features and settings
Cons of react-native-camera
- Limited to camera-related functionality, not suitable for general-purpose SVG rendering
- May have a larger impact on app size due to its comprehensive camera features
- Requires additional setup and permissions for camera access
Code Comparison
react-native-camera:
import { RNCamera } from 'react-native-camera';
<RNCamera
style={styles.preview}
type={RNCamera.Constants.Type.back}
flashMode={RNCamera.Constants.FlashMode.on}
androidCameraPermissionOptions={{
title: 'Permission to use camera',
message: 'We need your permission to use your camera',
buttonPositive: 'Ok',
buttonNegative: 'Cancel',
}}
/>
react-native-svg:
import Svg, { Circle, Rect } from 'react-native-svg';
<Svg height="100" width="100">
<Rect x="0" y="0" width="100" height="100" fill="black" />
<Circle cx="50" cy="50" r="30" fill="yellow" />
</Svg>
UI Components Library for React Native
Pros of react-native-ui-lib
- Comprehensive UI component library with a wide range of pre-built components
- Customizable theming system for consistent design across the app
- Built-in support for RTL languages and accessibility features
Cons of react-native-ui-lib
- Larger package size due to the extensive component library
- Steeper learning curve for developers new to the library's conventions
- May require more frequent updates to stay compatible with React Native versions
Code Comparison
react-native-ui-lib:
import {View, Text, Button} from 'react-native-ui-lib';
const MyComponent = () => (
<View>
<Text>Hello, World!</Text>
<Button label="Click me" onPress={() => {}} />
</View>
);
react-native-svg:
import Svg, {Circle, Rect} from 'react-native-svg';
const MySvgComponent = () => (
<Svg height="100" width="100">
<Circle cx="50" cy="50" r="45" stroke="blue" strokeWidth="2.5" fill="green" />
<Rect x="15" y="15" width="70" height="70" stroke="red" strokeWidth="2" fill="yellow" />
</Svg>
);
While react-native-ui-lib provides a comprehensive set of UI components, react-native-svg focuses specifically on SVG rendering capabilities. The choice between the two depends on the project's specific needs for either general UI components or SVG manipulation.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
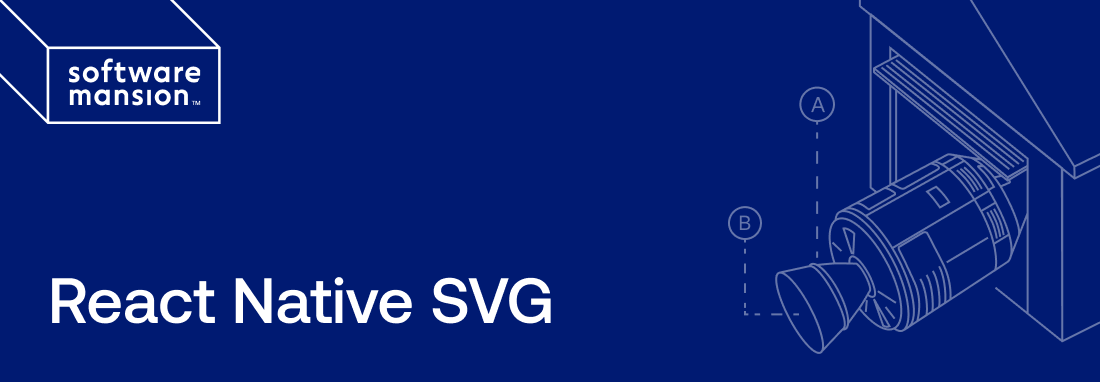
react-native-svg
provides SVG support to React Native on iOS, Android, macOS, Windows, and a compatibility layer for the web.
Features
- Supports most SVG elements and properties (Rect, Circle, Line, Polyline, Polygon, G ...).
- Easy to convert SVG code to react-native-svg.
Installation
With expo
â The Expo client app comes with the native code installed!
Install the JavaScript with:
npx expo install react-native-svg
ð See the Expo docs for more info or jump ahead to Usage.
With react-native-cli
-
Install library
from npm
npm install react-native-svg
from yarn
yarn add react-native-svg
-
Link native code
cd ios && pod install
Supported react-native versions
react-native-svg | react-native |
---|---|
3.2.0 | 0.29 |
4.2.0 | 0.32 |
4.3.0 | 0.33 |
4.4.0 | 0.38 |
4.5.0 | 0.40 |
5.1.8 | 0.44 |
5.2.0 | 0.45 |
5.3.0 | 0.46 |
5.4.1 | 0.47 |
5.5.1 | >=0.50 |
>=6 | >=0.50 |
>=7 | >=0.57.4 |
>=8 | >=0.57.4 |
>=9 | >=0.57.4 |
>=12.3.0 | >=0.64.0 |
>=15.0.0 | >=0.70.0 |
>=15.8.0 | >=0.73.0 |
Support for Fabric
Fabric is React Native's new rendering system. As of version 13.0.0
of this project, Fabric is supported only for react-native 0.69.0+. Support for earlier versions is not possible due to breaking changes in configuration.
react-native-svg | react-native |
---|---|
>=13.0.0 | 0.69.0+ |
>=13.6.0 | 0.70.0+ |
>=13.10.0 | 0.72.0+ |
Troubleshooting
Unexpected behavior
If you have unexpected behavior, please create a clean project with the latest versions of react-native and react-native-svg
react-native init CleanProject
cd CleanProject/
yarn add react-native-svg
cd ios && pod install && cd ..
Make a reproduction of the problem in App.js
react-native run-ios
react-native run-android
Adding Windows support
npx react-native-windows-init --overwrite
react-native run-windows
Opening issues
Verify that it is still an issue with the latest version as specified in the previous step. If so, open a new issue, include the entire App.js
file, specify what platforms you've tested, and the results of running this command:
react-native info
If you suspect that you've found a spec conformance bug, then you can test using your component in a react-native-web project by forking this codesandbox, to see how different browsers render the same content: https://codesandbox.io/s/pypn6mn3y7 If any evergreen browser with significant userbase or other svg user agent renders some svg content better, or supports more of the svg and related specs, please open an issue asap.
Usage
To check how to use the library, see USAGE.md
Known issues:
- Unable to apply focus point of RadialGradient on Android.
React Native SVG is maintained by Software Mansion
Since 2012 Software Mansion is a software agency with experience in building web and mobile apps. We are Core React Native Contributors and experts in dealing with all kinds of React Native issues. We can help you build your next dream product â Hire us.
Top Related Projects
Lottie wrapper for React Native.
A <LinearGradient /> component for react-native
React Native Cross-Platform WebView
React Native Mapview component for iOS + Android
A Camera component for React Native. Also supports barcode scanning!
UI Components Library for React Native
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot