trading-vue-js
💹 Hackable charting lib for traders. You can draw literally ANYTHING on top of candlestick charts. [Not Maintained]
Top Related Projects
📊 Interactive JavaScript Charts built on SVG
Performant financial charts built with HTML5 canvas
Highcharts JS, the JavaScript charting framework
Open-source JavaScript charting library behind Plotly and Dash
Simple HTML5 Charts using the <canvas> tag
Bring data to life with SVG, Canvas and HTML. :bar_chart::chart_with_upwards_trend::tada:
Quick Overview
Trading-vue-js is a lightweight and fast charting library for building interactive financial charts. It's designed specifically for trading applications, offering a wide range of technical analysis tools and customizable components. The library is built with Vue.js, making it easy to integrate into Vue-based projects.
Pros
- High performance and lightweight, suitable for real-time data visualization
- Extensive set of built-in technical indicators and drawing tools
- Highly customizable with a flexible overlay system
- Responsive design, works well on both desktop and mobile devices
Cons
- Limited documentation and examples compared to more established charting libraries
- Steeper learning curve for developers not familiar with Vue.js
- Fewer pre-built chart types compared to some alternatives
- May require additional effort to integrate with non-Vue.js projects
Code Examples
- Creating a basic chart:
<template>
<trading-vue :data="chartData" :width="800" :height="400"></trading-vue>
</template>
<script>
import TradingVue from 'trading-vue-js'
export default {
components: { TradingVue },
data() {
return {
chartData: {
ohlcv: [/* Your OHLCV data here */],
}
}
}
}
</script>
- Adding a custom indicator:
import { Overlay } from 'trading-vue-js'
class MyIndicator extends Overlay {
draw(ctx) {
// Custom drawing logic here
}
}
// In your component
components: { TradingVue },
data() {
return {
chartConfig: {
overlays: [MyIndicator]
}
}
}
- Implementing a custom tool:
import { Tool } from 'trading-vue-js'
class MyTool extends Tool {
meta_info() {
return { name: 'MyTool', icon: toolIcon }
}
draw(ctx) {
// Custom drawing logic here
}
}
// In your component
components: { TradingVue },
data() {
return {
chartConfig: {
tools: [MyTool]
}
}
}
Getting Started
-
Install the library:
npm install trading-vue-js
-
Import and use in your Vue component:
import TradingVue from 'trading-vue-js' export default { components: { TradingVue }, data() { return { chartData: { ohlcv: [/* Your OHLCV data */], } } } }
-
Add the component to your template:
<trading-vue :data="chartData" :width="800" :height="400"></trading-vue>
-
Customize as needed by adding indicators, tools, and overlays.
Competitor Comparisons
📊 Interactive JavaScript Charts built on SVG
Pros of ApexCharts.js
- More comprehensive and versatile, supporting a wider range of chart types
- Better documentation and larger community support
- Easier integration with various frameworks and libraries
Cons of ApexCharts.js
- Less specialized for financial and trading charts
- Potentially heavier and slower for complex real-time trading data
- May require more configuration for advanced trading-specific features
Code Comparison
ApexCharts.js:
var options = {
chart: { type: 'candlestick' },
series: [{ data: seriesData }],
xaxis: { type: 'datetime' }
};
var chart = new ApexCharts(document.querySelector("#chart"), options);
chart.render();
trading-vue-js:
new TradingVue({
el: '#chart',
data: {
ohlcv: yourOHLCVData,
overlays: [/* Your overlay objects */],
indicators: [/* Your indicator objects */]
}
});
ApexCharts.js offers a more general-purpose charting solution with extensive customization options, while trading-vue-js provides a more specialized and streamlined approach for trading-specific charts. The code comparison shows that trading-vue-js has a more concise setup for trading data, while ApexCharts.js requires more configuration but offers greater flexibility for various chart types.
Performant financial charts built with HTML5 canvas
Pros of lightweight-charts
- Developed and maintained by TradingView, ensuring high-quality and professional-grade charting
- Lightweight and performant, optimized for rendering large datasets quickly
- Supports a wide range of chart types and technical indicators out-of-the-box
Cons of lightweight-charts
- Less customizable compared to trading-vue-js, which offers more flexibility in chart design
- Steeper learning curve for developers not familiar with TradingView's API structure
- Limited community-driven development and contributions
Code Comparison
lightweight-charts:
const chart = createChart(document.body, { width: 400, height: 300 });
const lineSeries = chart.addLineSeries();
lineSeries.setData([
{ time: '2019-04-11', value: 80.01 },
{ time: '2019-04-12', value: 96.63 },
]);
trading-vue-js:
<trading-vue :data="this.$data" :width="this.width" :height="this.height"
:color-back="colors.colorBack"
:color-grid="colors.colorGrid"
:color-text="colors.colorText">
</trading-vue>
Both libraries offer powerful charting capabilities, but lightweight-charts focuses on performance and simplicity, while trading-vue-js provides more customization options and a Vue.js-centric approach. The choice between them depends on specific project requirements and developer preferences.
Highcharts JS, the JavaScript charting framework
Pros of Highcharts
- More comprehensive and feature-rich charting library
- Extensive documentation and community support
- Supports a wider range of chart types and customization options
Cons of Highcharts
- Commercial license required for most use cases
- Steeper learning curve due to its extensive API
- Larger file size, which may impact page load times
Code Comparison
Highcharts:
Highcharts.chart('container', {
series: [{
data: [1, 2, 3, 4, 5]
}]
});
trading-vue-js:
new TradingVue({
el: '#chart',
data: {
ohlcv: [[1, 10, 12, 9, 11], [2, 11, 13, 10, 12]]
}
});
Summary
Highcharts is a more established and feature-rich charting library with extensive documentation and support. It offers a wide range of chart types and customization options but comes with a commercial license requirement and a steeper learning curve. trading-vue-js, on the other hand, is specifically designed for financial charting and is more lightweight, making it easier to integrate into Vue.js projects. However, it may have fewer features and less extensive documentation compared to Highcharts.
Open-source JavaScript charting library behind Plotly and Dash
Pros of Plotly.js
- More versatile, supporting a wide range of chart types beyond financial charts
- Larger community and more extensive documentation
- Built-in support for interactive features like zooming and panning
Cons of Plotly.js
- Steeper learning curve for financial-specific visualizations
- Larger file size, which may impact page load times
- Less specialized for trading and financial data representation
Code Comparison
Trading-Vue-js:
new TradingVue({
el: '#chart',
data: {
ohlcv: [[1525651200000, 8750, 8800, 8500, 8650, 1000]],
onchart: [{ name: 'EMA', type: 'EMA', data: [] }]
}
})
Plotly.js:
Plotly.newPlot('chart', [{
x: [1525651200000],
close: [8650],
high: [8800],
low: [8500],
open: [8750],
type: 'candlestick'
}])
Both libraries offer ways to create financial charts, but Trading-Vue-js is more specialized for trading data, while Plotly.js provides a more general-purpose charting solution. Trading-Vue-js uses a more compact data structure, while Plotly.js requires separate arrays for each OHLC component. Plotly.js offers more flexibility for various chart types, but may require more setup for specific trading visualizations.
Simple HTML5 Charts using the <canvas> tag
Pros of Chart.js
- More versatile and suitable for various chart types beyond trading
- Larger community and ecosystem, with extensive documentation and resources
- Easier to integrate into existing projects due to its popularity and wide adoption
Cons of Chart.js
- Less specialized for trading-specific visualizations and features
- May require more customization to achieve advanced trading chart functionality
- Potentially slower performance for real-time data updates in trading scenarios
Code Comparison
trading-vue-js:
import TradingVue from 'trading-vue-js'
export default {
components: { TradingVue },
data() {
return { chartData: /* ... */ }
}
}
Chart.js:
import { Chart } from 'chart.js'
const ctx = document.getElementById('myChart')
new Chart(ctx, {
type: 'line',
data: /* ... */,
options: /* ... */
})
Summary
Chart.js is a more general-purpose charting library with broader applications, while trading-vue-js is specifically designed for trading charts. Chart.js offers greater flexibility and community support, but may require more customization for trading-specific features. trading-vue-js provides out-of-the-box functionality for trading visualizations but has a narrower focus. The choice between the two depends on the specific requirements of the project and the level of trading-specific functionality needed.
Bring data to life with SVG, Canvas and HTML. :bar_chart::chart_with_upwards_trend::tada:
Pros of d3
- Highly flexible and powerful data visualization library
- Extensive ecosystem with numerous plugins and extensions
- Widely adopted and well-documented
Cons of d3
- Steeper learning curve for beginners
- Requires more low-level coding for custom visualizations
- Not specifically tailored for financial charting
Code Comparison
d3:
const svg = d3.select("body").append("svg")
.attr("width", 960)
.attr("height", 500);
svg.append("circle")
.attr("cx", 480)
.attr("cy", 250)
.attr("r", 40)
.style("fill", "blue");
trading-vue-js:
<template>
<trading-vue :data="chart_data" :width="this.width" :height="this.height">
</trading-vue>
</template>
<script>
export default {
data() {
return {
chart_data: { /* ... */ }
}
}
}
</script>
Summary
d3 is a versatile data visualization library with a large ecosystem, while trading-vue-js is specifically designed for financial charting. d3 offers more flexibility but requires more low-level coding, whereas trading-vue-js provides a simpler API for creating trading charts. The choice between the two depends on the specific requirements of the project and the developer's familiarity with each library.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME

TradingVue.js

TradingVue.js was a hackable charting lib for traders. You could draw literally ANYTHING on top of candlestick charts. [Not Maintained]
Why
If you create trading software - this lib is probably for you. If you like to make custom indicators and think out of the box - this lib is most likely for you. And if you miss usability of TradingView.com in other open-source libraries and can't stand it - you are definetly in the right place!
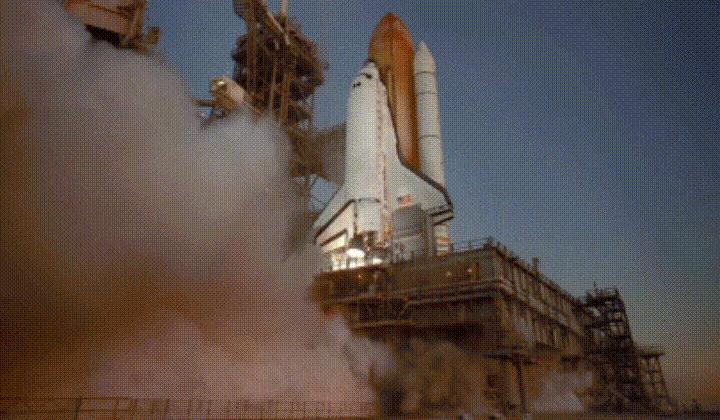
Features
- Scrolling & zooming as we all like
- Simple API for making new overlays
- Custom drawing tools
- Non-time based charts (e.g. Renko)
- One overlay === one .vue component
- Fully reactive
- Fully responsive
- Customizable colors and fonts
- Quite fast (works even with 3 mil candles)
- Scripts (make your own indicators)
Demo & Docs
Demo | Getting Started | API Book | Built-in Overlays | Examples | 101 Project | llll Gitter | FAQ | FREE Overlay Pack | Free XP Pack
To run the examples, download the repo & npm run test
Install
NPM
npm i trading-vue-js
In browser
<script src="trading-vue.min.js"></script>
How to use
Minimal working example:
<template>
<trading-vue :data="this.$data"></trading-vue>
</template>
<script>
import TradingVue from 'trading-vue-js'
export default {
name: 'app',
components: { TradingVue },
data() {
return {
ohlcv: [
[ 1551128400000, 33, 37.1, 14, 14, 196 ],
[ 1551132000000, 13.7, 30, 6.6, 30, 206 ],
[ 1551135600000, 29.9, 33, 21.3, 21.8, 74 ],
[ 1551139200000, 21.7, 25.9, 18, 24, 140 ],
[ 1551142800000, 24.1, 24.1, 24, 24.1, 29 ],
]
}
}
}
</script>
Core philosophy
The core philosophy is Data -> Screen Mapping (DSM). The library provides you with functions that map your data (it could be anything) to screen coordinates. The lib does all the dirty work behind the scenes: scrolling, scaling, reactivity, etc.
layout.t2screen(t) // time -> x
layout.$2screen($) // price -> y
layout.t_magnet(t) // time -> nearest candle x
layout.screen2$(y) // y -> price
layout.screen2t(x) // x -> time
Using these functions and the standard js canvas API, you can do magic.
Data structure
PRO TIP: chart is mandatory if you want to see something other than a white screen
IMPORTANT: All data must be sorted by time (in ascending order). The main OHLCV must not contain duplicate timestamps.
{
"chart": { // Mandatory
"type": "<Chart Type, e.g. Candles>",
"data": [
[timestamp, open, high, low, close, volume],
...
],
"settings": { } // Settings (depending on Chart Type)
},
"onchart": [ // Displayed ON the chart
{
"name": "<Indicator name>",
"type": "<e.g. EMA, SMA>",
"data": [
[timestamp, ... ], // Arbitrary length
...
],
"settings": { } // Arbitrary settings format
},
...
],
"offchart": [ // Displayed BELOW the chart
{
"name": "<Indicator name>",
"type": "<e.g. RSI, Stoch>",
"data": [
[timestamp, ... ], // Arbitrary length
...
],
"settings": { } // Arbitrary settings format
},
...
]
}
The process of adding a new indicator is simple: first you define your own data format (should be timestamped though) and display settings. For example, EMA data might look like this:
{
"name": "EMA, 25",
"type": "EMA",
"data": [
[ 1551128400000, 3091 ],
[ 1551132000000, 3112 ],
[ 1551135600000, 3105 ]
],
"settings": {
"color": "#42b28a"
}
},
Example of a simple overlay class
And then you make a new overlay class to display that data on the grid:
import { Overlay } from 'trading-vue-js'
export default {
name: 'EMA',
mixins: [Overlay],
methods: {
draw(ctx) {
const layout = this.$props.layout
ctx.strokeStyle = this.color
ctx.beginPath()
for (var p of this.$props.data) {
// t2screen & $2screen - special functions that
// map your data coordinates to grid coordinates
let x = layout.t2screen(p[0])
let y = layout.$2screen(p[1])
ctx.lineTo(x, y)
}
ctx.stroke()
},
use_for() { return ['EMA'] },
data_colors() { return [this.color] }
},
computed: {
color() {
return this.$props.settings.color
}
}
}
That's why the title doesn't lie: you can draw ANYTHING.
Grin

Code | click your ð
Roadmap
DocsTestsSolve known issues (marked as 'TODO: IMPORTANT')[PARTIALLY]Performance improvementsData-manipulation helpersAdd more built-in overlaysAdd toolbar (drawing tools)Custom loayout / layout persistence[POST-RELEASE]Fix and improve mobile version- Version 1.0.0 here
Progress in details: https://github.com/tvjsx/trading-vue-js/projects/1
Changelog
See CHANGELOG.md
Development & Building
Install devDependencies
npm install
Run development enviroment (hot)
npm run dev
Server is running on http://localhost:8080
Run in a CDN-like mode
npm run cdn
Server is running on http://localhost:8080
Build the bundle
npm run build
Visual testing
npm run test
Server is running on http://localhost:8080
Automatic testing
npm run auto-test
Contributing
- Fork ( https://github.com/tvjsx/trading-vue-js/fork )
- Create your feature branch (
git checkout -b cool-new-feature
) - Commit your changes (
git commit -am 'Let's rock smth'
) - Push to the branch (
git push origin cool-new-feature
) - Create a new Pull Request
Please read the guidelines in CONTRIBUTING.md
Top Related Projects
📊 Interactive JavaScript Charts built on SVG
Performant financial charts built with HTML5 canvas
Highcharts JS, the JavaScript charting framework
Open-source JavaScript charting library behind Plotly and Dash
Simple HTML5 Charts using the <canvas> tag
Bring data to life with SVG, Canvas and HTML. :bar_chart::chart_with_upwards_trend::tada:
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot