glide-transformations
An Android transformation library providing a variety of image transformations for Glide.
Top Related Projects
An image loading and caching library for Android focused on smooth scrolling
Image loading for Android and Compose Multiplatform.
A powerful image downloading and caching library for Android
An Android library for managing images and the memory they use.
Powerful and flexible library for loading, caching and displaying images on Android.
Image Cropping Library for Android
Quick Overview
Glide Transformations is an Android image processing library that provides a collection of custom transformations for Glide, a popular image loading and caching library. It allows developers to easily apply various image effects and transformations, such as blurring, cropping, and color filtering, to images loaded with Glide.
Pros
- Extensive collection of ready-to-use image transformations
- Easy integration with Glide library
- Improves app performance by applying transformations efficiently
- Regularly maintained and updated
Cons
- Adds additional dependencies to the project
- May increase app size due to additional transformation code
- Some transformations might be computationally expensive for large images
- Limited customization options for certain transformations
Code Examples
- Applying a circular crop transformation:
Glide.with(context)
.load(imageUrl)
.transform(CircleCrop())
.into(imageView)
- Blurring an image:
Glide.with(context)
.load(imageUrl)
.transform(BlurTransformation(25))
.into(imageView)
- Applying multiple transformations:
Glide.with(context)
.load(imageUrl)
.transform(
CenterCrop(),
RoundedCornersTransformation(30, 0, RoundedCornersTransformation.CornerType.ALL)
)
.into(imageView)
Getting Started
- Add the following dependencies to your app's
build.gradle
file:
dependencies {
implementation 'com.github.bumptech.glide:glide:4.12.0'
implementation 'jp.wasabeef:glide-transformations:4.3.0'
}
- In your code, import the necessary classes:
import com.bumptech.glide.Glide
import jp.wasabeef.glide.transformations.*
- Use the transformations in your image loading code:
Glide.with(context)
.load(imageUrl)
.transform(GrayscaleTransformation())
.into(imageView)
Now you can start using Glide Transformations in your Android project to apply various image effects and transformations easily.
Competitor Comparisons
An image loading and caching library for Android focused on smooth scrolling
Pros of Glide
- Core image loading library with extensive features and optimizations
- Larger community and more frequent updates
- Supports a wider range of image sources and caching strategies
Cons of Glide
- Larger library size, potentially increasing app size
- Steeper learning curve for basic image loading tasks
- Requires more configuration for simple transformations
Code Comparison
Glide (basic image loading):
Glide.with(context)
.load(imageUrl)
.into(imageView);
Glide-transformations (with transformation):
Glide.with(context)
.load(imageUrl)
.apply(RequestOptions.bitmapTransform(new BlurTransformation(25)))
.into(imageView);
Key Differences
Glide is a full-featured image loading library, while Glide-transformations is a collection of image transformations built on top of Glide. Glide-transformations provides easy-to-use image manipulations like blur, crop, and color filters, which are not included in Glide's core functionality.
Glide-transformations is ideal for projects that require specific image transformations without the need for extensive customization. It offers a simpler API for applying transformations compared to implementing them manually in Glide.
Glide, on the other hand, provides more control over the entire image loading process, including memory management, caching, and request handling. It's better suited for projects with complex image loading requirements or those needing fine-grained control over the image pipeline.
Image loading for Android and Compose Multiplatform.
Pros of Coil
- Built with Kotlin coroutines, offering better performance and memory efficiency
- Supports image loading from various sources (network, resources, files) out of the box
- Lightweight library with minimal dependencies
Cons of Coil
- Newer library with potentially fewer community resources and third-party extensions
- Limited to Kotlin projects, not suitable for Java-only applications
Code Comparison
Glide Transformations:
Glide.with(context)
.load(url)
.transform(BlurTransformation(25), RoundedCornersTransformation(25, 0))
.into(imageView)
Coil:
imageView.load(url) {
transformations(BlurTransformation(25f), RoundedCornersTransformation(25f))
}
Summary
Coil is a modern, Kotlin-first image loading library that offers excellent performance and a concise API. It's well-suited for Kotlin projects and provides built-in support for various image sources. However, it may have fewer community resources compared to more established libraries like Glide Transformations.
Glide Transformations, on the other hand, is a mature library with a wide range of pre-built transformations and extensive community support. It works well with both Java and Kotlin projects but may require more boilerplate code compared to Coil.
Choose Coil for Kotlin projects prioritizing performance and simplicity, or Glide Transformations for broader language support and a larger ecosystem of transformations.
A powerful image downloading and caching library for Android
Pros of Picasso
- Simpler API and easier to use for basic image loading tasks
- Built-in memory and disk caching for improved performance
- Smaller library size, which can be beneficial for apps with limited resources
Cons of Picasso
- Limited built-in image transformation options
- Less flexible for complex image manipulation tasks
- Slower image loading compared to Glide in some scenarios
Code Comparison
Picasso:
Picasso.get()
.load(url)
.resize(250, 250)
.centerCrop()
.into(imageView);
Glide-transformations:
Glide.with(context)
.load(url)
.apply(RequestOptions.bitmapTransform(new RoundedCornersTransformation(25, 0)))
.into(imageView);
Summary
Picasso is a simpler, more lightweight image loading library that excels in basic image loading tasks with built-in caching. It's easier to use for beginners and has a smaller footprint. However, it lacks advanced transformation options and may be slower in some cases.
Glide-transformations, on the other hand, offers a wide range of image transformation options and is generally faster. It's more suitable for complex image manipulation tasks but may have a steeper learning curve and a larger library size.
The choice between the two depends on the specific needs of your project, such as required transformations, performance considerations, and ease of use.
An Android library for managing images and the memory they use.
Pros of Fresco
- More comprehensive image loading and caching solution
- Better memory management, especially for large images
- Supports progressive image loading and streaming
Cons of Fresco
- Larger library size and more complex integration
- Steeper learning curve compared to Glide transformations
- Limited to Android platform
Code Comparison
Fresco:
Uri uri = Uri.parse("https://example.com/image.jpg");
SimpleDraweeView draweeView = findViewById(R.id.my_image_view);
draweeView.setImageURI(uri);
Glide transformations:
Glide.with(context)
.load("https://example.com/image.jpg")
.transform(new CircleCrop())
.into(imageView);
Summary
Fresco is a more comprehensive image loading library with advanced features like progressive loading and better memory management. However, it comes with a larger footprint and steeper learning curve. Glide transformations, on the other hand, focuses specifically on image transformations and is easier to integrate, but lacks some of the advanced features of Fresco. The choice between the two depends on the specific requirements of your project and the level of complexity you're willing to manage.
Powerful and flexible library for loading, caching and displaying images on Android.
Pros of Android-Universal-Image-Loader
- More comprehensive image loading solution with built-in caching mechanisms
- Supports a wider range of image sources (network, file system, assets, etc.)
- Highly configurable with extensive options for customization
Cons of Android-Universal-Image-Loader
- Less actively maintained compared to Glide-transformations
- Heavier library size, which may impact app performance
- Steeper learning curve due to its extensive feature set
Code Comparison
Android-Universal-Image-Loader:
ImageLoader imageLoader = ImageLoader.getInstance();
imageLoader.displayImage(imageUri, imageView, options);
Glide-transformations:
Glide.with(context)
.load(imageUrl)
.transform(new CircleCrop())
.into(imageView);
Android-Universal-Image-Loader offers a more traditional approach with a singleton instance and separate options, while Glide-transformations uses a fluent API with chained methods for configuration and transformations.
Android-Universal-Image-Loader provides a complete image loading solution, whereas Glide-transformations focuses specifically on image transformations to be used with the Glide library. Glide-transformations offers a more modern and lightweight approach, making it easier to integrate into existing projects using Glide. However, Android-Universal-Image-Loader may be preferred for projects requiring extensive customization and support for various image sources.
Image Cropping Library for Android
Pros of uCrop
- Specialized for image cropping with a user-friendly interface
- Supports gesture-based interactions for intuitive cropping
- Offers advanced features like rotation and aspect ratio adjustments
Cons of uCrop
- Limited to cropping functionality, less versatile for other image transformations
- May require additional libraries for image loading and caching
- Potentially higher learning curve for integration compared to Glide transformations
Code Comparison
uCrop:
UCrop.of(sourceUri, destinationUri)
.withAspectRatio(16, 9)
.withMaxResultSize(maxWidth, maxHeight)
.start(activity);
Glide transformations:
Glide.with(context)
.load(url)
.transform(new CropTransformation(width, height))
.into(imageView);
Summary
uCrop excels in providing a comprehensive image cropping solution with a polished UI, while Glide transformations offers a wider range of image manipulations integrated seamlessly with Glide's image loading capabilities. uCrop is ideal for apps requiring detailed cropping functionality, whereas Glide transformations is more suitable for quick, programmatic image transformations within the Glide ecosystem.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Glide Transformations
An Android transformation library providing a variety of image transformations for Glide.
Please feel free to use this.
Are you using Picasso or Fresco?
Picasso Transformations
Fresco Processors
Demo
Original Image

Transformations

How do I use it?
Step 1
Gradle
repositories {
mavenCentral()
}
dependencies {
implementation 'jp.wasabeef:glide-transformations:4.3.0'
// If you want to use the GPU Filters
implementation 'jp.co.cyberagent.android:gpuimage:2.1.0'
}
Step 2
Set Glide Transform.
Glide.with(this).load(R.drawable.demo)
.apply(RequestOptions.bitmapTransform(BlurTransformation(25, 3)))
.into(imageView)
Advanced Step 3
You can set a multiple transformations.
val multi = MultiTransformation<Bitmap>(
BlurTransformation(25),
RoundedCornersTransformation(128, 0, CornerType.BOTTOM))))
Glide.with(this).load(R.drawable.demo)
.apply(RequestOptions.bitmapTransform(multi))
.into(imageView))
Transformations
Crop
CropTransformation
CropCircleTransformation
CropCircleWithBorderTransformation
CropSquareTransformation
RoundedCornersTransformation
Color
ColorFilterTransformation
GrayscaleTransformation
Blur
BlurTransformation
Mask
MaskTransformation
GPU Filter (use GPUImage)
Will require add dependencies for GPUImage.
ToonFilterTransformation
SepiaFilterTransformation
ContrastFilterTransformation
InvertFilterTransformation
PixelationFilterTransformation
SketchFilterTransformation
SwirlFilterTransformation
BrightnessFilterTransformation
KuwaharaFilterTransformation
VignetteFilterTransformation
Applications using Glide Transformations
Please ping me or send a pull request if you would like to be added here.
Icon | Application |
---|---|
Ameba Ownd | |
AbemaTV | |
TV Time | |
Christmas Radio |
Developed By
Daichi Furiya (Wasabeef) - dadadada.chop@gmail.com
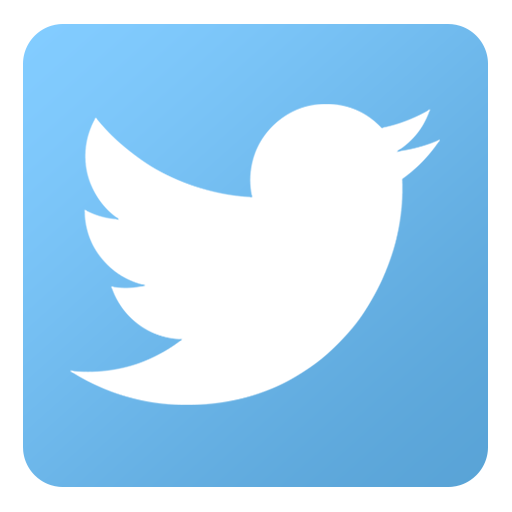
Contributions
Any contributions are welcome!
Contributors
Thanks
- Inspired by
Picasso Transformations
in TannerPerrien.
License
Copyright (C) 2020 Wasabeef
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Top Related Projects
An image loading and caching library for Android focused on smooth scrolling
Image loading for Android and Compose Multiplatform.
A powerful image downloading and caching library for Android
An Android library for managing images and the memory they use.
Powerful and flexible library for loading, caching and displaying images on Android.
Image Cropping Library for Android
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot