Top Related Projects
Material Design Floating Action Button in liquid state
:octocat: 📃 FoldingCell is an expanding content cell with animation made by @Ramotion
:octocat: RAMAnimatedTabBarController is a Swift UI module library for adding animation to iOS tabbar items and icons. iOS library made by @Ramotion
Animated top menu for UITableView / UICollectionView / UIScrollView written in Swift
Animated side menu with customizable UI
Quick Overview
HMSegmentedControl is a customizable alternative to UISegmentedControl for iOS applications. It offers a drop-in replacement with additional features and styling options, allowing developers to create more visually appealing and interactive segmented controls.
Pros
- Highly customizable appearance and behavior
- Supports both text and images for segments
- Smooth animations for selection changes
- Compatible with iOS 7.0 and later
Cons
- Limited documentation and examples
- Not actively maintained (last update was in 2019)
- May require additional work to integrate with SwiftUI
- Some reported issues with Auto Layout constraints
Code Examples
Creating a basic segmented control:
let segmentedControl = HMSegmentedControl(sectionTitles: ["One", "Two", "Three"])
segmentedControl.frame = CGRect(x: 10, y: 10, width: 300, height: 40)
view.addSubview(segmentedControl)
Customizing appearance:
segmentedControl.selectedSegmentIndex = 1
segmentedControl.backgroundColor = .lightGray
segmentedControl.titleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.black]
segmentedControl.selectedTitleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.white]
segmentedControl.selectionIndicatorColor = .blue
Adding a selection callback:
segmentedControl.indexChangeBlock = { index in
print("Selected segment index: \(index)")
}
Getting Started
-
Add HMSegmentedControl to your project:
- CocoaPods: Add
pod 'HMSegmentedControl'
to your Podfile - Manual: Copy the
HMSegmentedControl.h
andHMSegmentedControl.m
files to your project
- CocoaPods: Add
-
Import the header in your view controller:
import HMSegmentedControl
-
Create and customize an instance of HMSegmentedControl:
let segmentedControl = HMSegmentedControl(sectionTitles: ["Option 1", "Option 2", "Option 3"]) segmentedControl.frame = CGRect(x: 10, y: 10, width: 300, height: 40) segmentedControl.selectedSegmentIndex = 0 segmentedControl.backgroundColor = .lightGray segmentedControl.titleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.black] segmentedControl.selectedTitleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.white] segmentedControl.selectionIndicatorColor = .blue view.addSubview(segmentedControl)
-
Implement the selection callback:
segmentedControl.indexChangeBlock = { index in // Handle segment selection }
Competitor Comparisons
Material Design Floating Action Button in liquid state
Pros of LiquidFloatingActionButton
- Offers a unique, visually appealing liquid animation effect
- Provides a more modern and eye-catching design for action buttons
- Allows for customizable cell animations and colors
Cons of LiquidFloatingActionButton
- Limited to floating action button functionality, less versatile than a segmented control
- May require more complex implementation for multiple options
- Potentially higher performance impact due to animations
Code Comparison
HMSegmentedControl:
let segmentedControl = HMSegmentedControl(sectionTitles: ["One", "Two", "Three"])
segmentedControl.frame = CGRect(x: 10, y: 10, width: 300, height: 40)
self.view.addSubview(segmentedControl)
LiquidFloatingActionButton:
let floatingActionButton = LiquidFloatingActionButton(frame: CGRect(x: 16, y: 16, width: 56, height: 56))
floatingActionButton.responsible = true
self.view.addSubview(floatingActionButton)
Both repositories offer UI components for iOS applications, but they serve different purposes. HMSegmentedControl provides a customizable segmented control for selecting between multiple options, while LiquidFloatingActionButton focuses on creating an animated floating action button with a unique liquid effect. The choice between the two depends on the specific UI requirements of the application and the desired user interaction style.
:octocat: 📃 FoldingCell is an expanding content cell with animation made by @Ramotion
Pros of folding-cell
- Offers a unique and visually appealing folding animation for cell expansion
- Provides a more interactive and engaging user experience
- Supports both Swift and Objective-C
Cons of folding-cell
- More complex implementation compared to HMSegmentedControl
- May not be suitable for all types of content or UI designs
- Requires more customization for different use cases
Code Comparison
HMSegmentedControl:
HMSegmentedControl *segmentedControl = [[HMSegmentedControl alloc] initWithSectionTitles:@[@"One", @"Two", @"Three"]];
segmentedControl.frame = CGRectMake(10, 10, 300, 60);
[self.view addSubview:segmentedControl];
folding-cell:
let cell = FoldingCell()
cell.itemCount = 2
cell.delegate = self
cell.foregroundView = foregroundView
cell.containerView = containerView
HMSegmentedControl is simpler to implement and focuses on creating customizable segmented controls. It's ideal for navigation and filtering options. folding-cell, on the other hand, offers a more dynamic and visually striking cell expansion animation, suitable for showcasing detailed content in a compact space. While folding-cell provides a unique user experience, it may require more effort to integrate and customize for specific use cases compared to the straightforward implementation of HMSegmentedControl.
:octocat: RAMAnimatedTabBarController is a Swift UI module library for adding animation to iOS tabbar items and icons. iOS library made by @Ramotion
Pros of animated-tab-bar
- Offers animated transitions between tab bar items, providing a more engaging user experience
- Supports custom animations and icon designs, allowing for greater customization
- Includes a variety of pre-built animations out of the box
Cons of animated-tab-bar
- More complex implementation due to animation features, potentially increasing development time
- May have a steeper learning curve for developers unfamiliar with custom animations
- Could potentially impact performance on older devices due to animations
Code Comparison
HMSegmentedControl:
let segmentedControl = HMSegmentedControl(sectionTitles: ["One", "Two", "Three"])
segmentedControl.frame = CGRect(x: 10, y: 10, width: 300, height: 60)
self.view.addSubview(segmentedControl)
animated-tab-bar:
let tabBar = RAMAnimatedTabBarController()
let item1 = RAMAnimatedTabBarItem(title: "One", image: UIImage(named: "icon1"), tag: 1)
item1.animation = RAMBounceAnimation()
tabBar.viewControllers = [UIViewController()]
tabBar.setViewControllers([UIViewController()], animated: true)
Both libraries offer solutions for customizable tab bars or segmented controls in iOS applications. HMSegmentedControl provides a simpler, more straightforward implementation for segmented controls, while animated-tab-bar focuses on animated tab bars with more advanced customization options. The choice between the two depends on the specific requirements of the project and the desired level of visual complexity.
Animated top menu for UITableView / UICollectionView / UIScrollView written in Swift
Pros of Persei
- Offers a more visually appealing and customizable UI with animated menu items
- Provides a scrollable content area beneath the menu, allowing for more versatile layouts
- Includes built-in support for custom animations and transitions
Cons of Persei
- More complex implementation compared to HMSegmentedControl's straightforward approach
- Potentially higher performance overhead due to additional UI elements and animations
- Less suitable for simple segmented control use cases where minimal customization is needed
Code Comparison
HMSegmentedControl:
let segmentedControl = HMSegmentedControl(sectionTitles: ["One", "Two", "Three"])
segmentedControl.frame = CGRect(x: 0, y: 0, width: 300, height: 40)
segmentedControl.addTarget(self, action: #selector(segmentedControlChangedValue(_:)), for: .valueChanged)
Persei:
let menu = MenuView()
menu.items = [MenuItem(title: "One"), MenuItem(title: "Two"), MenuItem(title: "Three")]
menu.delegate = self
view.addSubview(menu)
Both libraries offer segmented control functionality, but Persei provides a more feature-rich and customizable solution at the cost of increased complexity. HMSegmentedControl is better suited for simpler use cases, while Persei shines in scenarios requiring advanced UI and animations.
Animated side menu with customizable UI
Pros of Side-Menu.iOS
- Provides a complete side menu solution with customizable animations
- Offers a more visually appealing and modern UI design
- Includes gesture recognition for intuitive user interaction
Cons of Side-Menu.iOS
- More complex implementation compared to HMSegmentedControl
- Limited to side menu functionality, less versatile for other UI elements
- May require more resources due to animations and gesture handling
Code Comparison
Side-Menu.iOS:
let menuLeftNavigationController = UISideMenuNavigationController(rootViewController: YourViewController)
SideMenuManager.default.leftMenuNavigationController = menuLeftNavigationController
SideMenuManager.default.addPanGestureToPresent(toView: self.navigationController!.navigationBar)
HMSegmentedControl:
let segmentedControl = HMSegmentedControl(sectionTitles: ["One", "Two", "Three"])
segmentedControl.frame = CGRect(x: 0, y: 0, width: 300, height: 40)
segmentedControl.addTarget(self, action: #selector(segmentedControlChangedValue(_:)), for: .valueChanged)
Side-Menu.iOS focuses on creating a side menu with navigation, while HMSegmentedControl provides a customizable segmented control for switching between views or options. The code snippets demonstrate the different setup processes, with Side-Menu.iOS requiring more configuration for the menu and gestures, while HMSegmentedControl has a simpler initialization and target-action setup.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
HMSegmentedControl
A highly customizable drop-in replacement for UISegmentedControl, used by more than 22,000 apps, including TikTok, PayPal, Imgur and Bleacher Report.

Features
- ð¸ Supports both text and images
- âï¸ Multiple sizing and selection styles
- ð Horizontal scrolling for an infinite number of segments
- âï¸ Advanced title styling with text attributes for font, color, kerning, shadow, etc
- ð¥ Compatible with both Swift and Objective-C
- ð± Updated for Xcode 11, iOS 13 and Swift 5. Supports all the way back to iOS 7!
Installation
pod 'HMSegmentedControl'
Installation via Carthage is also supported..
Usage
The code below will create a segmented control with the default looks:
let segmentedControl = HMSegmentedControl(sectionTitles: [
"Trending",
"News",
"Library"
])
segmentedControl.frame = CGRect(x: 0, y: 0, width: 100, height: 40)
segmentedControl.addTarget(self, action: #selector(segmentedControlChangedValue(segmentedControl:)), for: .valueChanged)
view.addSubview(segmentedControl)
Included is a demo project showing how to fully customize HMSegmentedControl.
Possible Styles



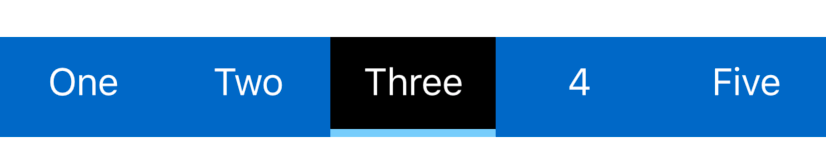
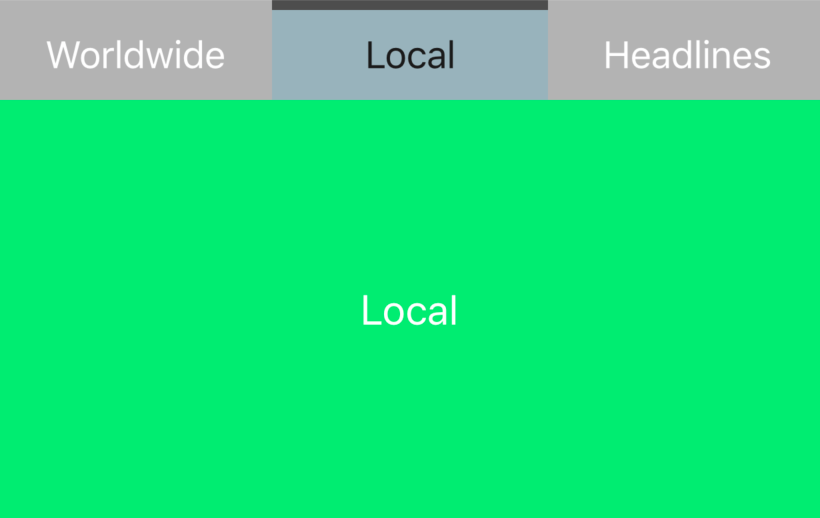
Apps Using HMSegmentedControl
If you are using HMSegmentedControl in your app or know of an app that uses it, please add it to this list.
Need Help?
If you need help with HMSegmentedControl, or with iOS/Swift development in general, check out swiftmentor.io
License
HMSegmentedControl is licensed under the terms of the MIT License. Please see the LICENSE file for full details.
If this code was helpful, I would love to hear from you.
Top Related Projects
Material Design Floating Action Button in liquid state
:octocat: 📃 FoldingCell is an expanding content cell with animation made by @Ramotion
:octocat: RAMAnimatedTabBarController is a Swift UI module library for adding animation to iOS tabbar items and icons. iOS library made by @Ramotion
Animated top menu for UITableView / UICollectionView / UIScrollView written in Swift
Animated side menu with customizable UI
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot