Top Related Projects
A framework for building native Windows apps with React.
Material Design for React Native (Android & iOS)
Cross-platform React UI packages
Cross-Platform React Native UI Toolkit
UI Components Library for React Native
Mobile-first, accessible components for React Native & Web to build consistent UI across Android, iOS and Web.
Quick Overview
React-sketchapp is a library that allows developers to render React components to Sketch. It enables the creation of design systems and UI components directly in Sketch using React and JavaScript, bridging the gap between design and development workflows.
Pros
- Seamless integration of React components into Sketch designs
- Enables developers to create and maintain design systems programmatically
- Facilitates better collaboration between designers and developers
- Supports hot reloading for faster iteration and development
Cons
- Limited to Sketch, not compatible with other design tools
- Requires knowledge of React and JavaScript, which may be challenging for designers
- Some complex React components may not translate perfectly to Sketch
- Performance can be slow for large or complex designs
Code Examples
- Rendering a simple React component to Sketch:
import React from 'react';
import { render, Text, View } from 'react-sketchapp';
const MyComponent = () => (
<View>
<Text>Hello, Sketch!</Text>
</View>
);
export default () => {
render(<MyComponent />, context.document.currentPage());
};
- Creating a styled button component:
import React from 'react';
import { render, Text, View, StyleSheet } from 'react-sketchapp';
const styles = StyleSheet.create({
button: {
backgroundColor: '#007AFF',
borderRadius: 8,
padding: 16,
},
buttonText: {
color: '#FFFFFF',
fontSize: 16,
fontWeight: 'bold',
},
});
const Button = ({ label }) => (
<View style={styles.button}>
<Text style={styles.buttonText}>{label}</Text>
</View>
);
export default () => {
render(<Button label="Click me" />, context.document.currentPage());
};
- Rendering a list of items:
import React from 'react';
import { render, Text, View, StyleSheet } from 'react-sketchapp';
const styles = StyleSheet.create({
list: {
padding: 16,
},
item: {
marginBottom: 8,
},
});
const List = ({ items }) => (
<View style={styles.list}>
{items.map((item, index) => (
<Text key={index} style={styles.item}>{item}</Text>
))}
</View>
);
export default () => {
const items = ['Apple', 'Banana', 'Cherry', 'Date'];
render(<List items={items} />, context.document.currentPage());
};
Getting Started
-
Install react-sketchapp:
npm install react-sketchapp
-
Create a new Sketch plugin:
npx skpm create my-plugin cd my-plugin
-
Replace the contents of
src/my-command.js
with your React component:import React from 'react'; import { render, Text } from 'react-sketchapp'; export default () => { render(<Text>Hello, Sketch!</Text>, context.document.currentPage()); };
-
Build and run the plugin:
npm run build npm run watch
-
Open Sketch and run your plugin from the Plugins menu.
Competitor Comparisons
A framework for building native Windows apps with React.
Pros of react-native-windows
- Enables development of native Windows applications using React Native
- Provides access to Windows-specific APIs and features
- Supports both UWP and Win32 app development
Cons of react-native-windows
- Limited to Windows platform, reducing cross-platform compatibility
- Steeper learning curve for developers unfamiliar with Windows development
- May require additional Windows-specific code and configurations
Code Comparison
react-native-windows:
import { NativeModules } from 'react-native';
const { WindowsModule } = NativeModules;
WindowsModule.showNativeDialog('Hello from Windows!');
react-sketchapp:
import { Text, View } from 'react-sketchapp';
<View>
<Text>Hello from Sketch!</Text>
</View>
Summary
react-native-windows focuses on building native Windows applications using React Native, offering access to Windows-specific features and APIs. It supports both UWP and Win32 app development but is limited to the Windows platform.
react-sketchapp, on the other hand, is designed for creating Sketch documents using React components. It allows designers and developers to collaborate more effectively by using a shared language (React) for both design and development.
While react-native-windows provides a way to build native Windows apps, react-sketchapp bridges the gap between design and development workflows within the Sketch environment.
Material Design for React Native (Android & iOS)
Pros of react-native-paper
- Comprehensive set of pre-built UI components for React Native
- Follows Material Design guidelines, ensuring a consistent and modern look
- Regular updates and active community support
Cons of react-native-paper
- Limited to React Native development, not suitable for web or desktop applications
- May require additional customization to match specific design requirements
- Larger bundle size due to the extensive component library
Code Comparison
react-native-paper:
import { Button } from 'react-native-paper';
const MyComponent = () => (
<Button mode="contained" onPress={() => console.log('Pressed')}>
Press me
</Button>
);
react-sketchapp:
import { View, Text } from 'react-sketchapp';
const MyComponent = () => (
<View>
<Text>Hello, Sketch!</Text>
</View>
);
Key Differences
- react-native-paper focuses on providing ready-to-use UI components for React Native applications
- react-sketchapp is designed for rendering React components to Sketch for design purposes
- react-native-paper follows Material Design principles, while react-sketchapp is more flexible in terms of design output
- react-sketchapp allows designers to create and maintain design systems using React, bridging the gap between design and development
Cross-platform React UI packages
Pros of react-native-web
- Broader application: Can be used for web, iOS, and Android development
- More active community and frequent updates
- Better performance for complex web applications
Cons of react-native-web
- Steeper learning curve for developers new to React Native
- Some React Native components may not have exact web equivalents
- Potential inconsistencies in behavior across platforms
Code Comparison
react-native-web:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => (
<View style={styles.container}>
<Text>Hello, Web and Mobile!</Text>
</View>
);
react-sketchapp:
import React from 'react';
import { View, Text, StyleSheet } from 'react-sketchapp';
const App = () => (
<View style={styles.container}>
<Text>Hello, Sketch!</Text>
</View>
);
The code structure is similar, but react-native-web uses React Native components that work across platforms, while react-sketchapp is specifically designed for creating Sketch designs using React components.
react-native-web offers more flexibility for cross-platform development, while react-sketchapp excels in design-to-code workflows within Sketch. The choice between the two depends on the specific project requirements and target platforms.
Cross-Platform React Native UI Toolkit
Pros of react-native-elements
- Comprehensive UI toolkit with a wide range of pre-built components
- Active community and frequent updates
- Customizable and themeable components
Cons of react-native-elements
- Limited design-to-code functionality
- Not specifically tailored for Sketch integration
- May require additional setup for complex design workflows
Code Comparison
react-native-elements:
import { Button } from 'react-native-elements';
<Button
title="Click me!"
buttonStyle={{ backgroundColor: 'blue' }}
onPress={() => console.log('Button pressed')}
/>
react-sketchapp:
import { View, Text, StyleSheet } from 'react-sketchapp';
<View style={styles.container}>
<Text style={styles.text}>Hello, Sketch!</Text>
</View>
Summary
react-native-elements is a comprehensive UI toolkit for React Native applications, offering a wide range of customizable components. It's ideal for rapid development of mobile apps with consistent UI elements. However, it lacks specific design-to-code functionality and Sketch integration.
react-sketchapp, on the other hand, is tailored for bridging the gap between design and development, allowing React components to be rendered directly in Sketch. It's more focused on the design-to-code workflow but may have a steeper learning curve for developers not familiar with Sketch.
Choose react-native-elements for building React Native apps with pre-built UI components, or react-sketchapp for a more design-centric approach with Sketch integration.
UI Components Library for React Native
Pros of react-native-ui-lib
- Specifically designed for React Native, offering a more tailored experience for mobile app development
- Provides a comprehensive set of pre-built UI components, reducing development time
- Offers a theming system for easy customization and consistent design across the app
Cons of react-native-ui-lib
- Limited to React Native development, not suitable for web or other platforms
- May have a steeper learning curve due to its extensive component library
- Less focus on design-to-code workflow compared to react-sketchapp
Code Comparison
react-native-ui-lib:
import { View, Text, Button } from 'react-native-ui-lib';
const MyComponent = () => (
<View>
<Text>Hello, World!</Text>
<Button label="Click me" onPress={() => {}} />
</View>
);
react-sketchapp:
import { View, Text, StyleSheet } from 'react-sketchapp';
const MyComponent = () => (
<View style={styles.container}>
<Text>Hello, World!</Text>
</View>
);
const styles = StyleSheet.create({
container: { /* styles */ },
});
The main difference in the code examples is that react-native-ui-lib provides ready-to-use components like Button, while react-sketchapp focuses more on rendering basic components and styles in Sketch. react-sketchapp is primarily used for creating design systems and prototypes in Sketch, while react-native-ui-lib is geared towards building fully functional React Native applications.
Mobile-first, accessible components for React Native & Web to build consistent UI across Android, iOS and Web.
Pros of NativeBase
- Extensive set of pre-built UI components for React Native
- Cross-platform compatibility (iOS, Android, and Web)
- Customizable theme system for consistent styling
Cons of NativeBase
- Steeper learning curve due to its comprehensive nature
- Larger bundle size compared to more lightweight alternatives
- May require additional configuration for optimal performance
Code Comparison
NativeBase:
import { Box, Text, Button } from 'native-base';
const MyComponent = () => (
<Box>
<Text>Hello, NativeBase!</Text>
<Button>Click me</Button>
</Box>
);
react-sketchapp:
import { View, Text, StyleSheet } from 'react-sketchapp';
const MyComponent = () => (
<View style={styles.container}>
<Text>Hello, react-sketchapp!</Text>
</View>
);
While NativeBase provides a rich set of pre-built components and a theming system, react-sketchapp focuses on rendering React components to Sketch. NativeBase is better suited for building full-fledged mobile applications, while react-sketchapp is primarily used for design-to-code workflows and creating Sketch assets programmatically.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
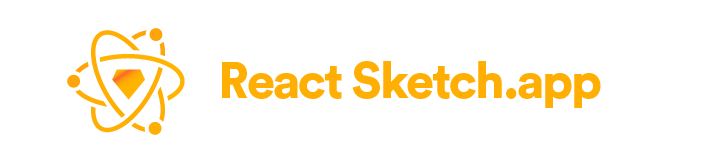
Quick-start ðâ
First, make sure you have installed Sketch version 50+, & a recent npm.
Open a new Sketch file, then in a terminal:
git clone https://github.com/airbnb/react-sketchapp.git
cd react-sketchapp/examples/basic-setup && npm install
npm run render
Next, check out some more examples!
Why?!
Managing the assets of design systems in Sketch is complex, error-prone and time consuming. Sketch is scriptable, but the API often changes. React provides the perfect wrapper to build reusable documents in a way already familiar to JavaScript developers.
What does the code look like?
import * as React from 'react';
import { render, Text, Artboard } from 'react-sketchapp';
const App = props => (
<Artboard>
<Text style={{ fontFamily: 'Comic Sans MS', color: 'hotPink' }}>{props.message}</Text>
</Artboard>
);
export default context => {
render(<App message="Hello world!" />, context.document.currentPage());
};
What can I do with it?
- Manage design systemsâ
react-sketchapp
was built for Airbnbâs design system; this is the easiest way to manage Sketch assets in a large design system - Use real components for designsâ Implement your designs in code as React components and render them into Sketch
- Design with real dataâ Designing with data is important but challenging;
react-sketchapp
makes it simple to fetch and incorporate real data into your Sketch files - Build new tools on top of Sketchâ the easiest way to use Sketch as a canvas for custom design tooling
Found a novel use? We'd love to hear about it!
Read more about why we built it
Documentation
Top Related Projects
A framework for building native Windows apps with React.
Material Design for React Native (Android & iOS)
Cross-platform React UI packages
Cross-Platform React Native UI Toolkit
UI Components Library for React Native
Mobile-first, accessible components for React Native & Web to build consistent UI across Android, iOS and Web.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot