Top Related Projects
ZXing ("Zebra Crossing") barcode scanning library for Java, Android
Barcode scanner library for Android, based on the ZXing decoder
Code scanner library for Android, based on ZXing
Modification of ZXING Barcode Scanner project for easy Android QR-Code detection and AR purposes
A cross platform HTML5 QR code reader. See end to end implementation at: https://scanapp.org
Quick Overview
The dm77/barcodescanner repository is an Android barcode scanning library based on ZXing and ZBar. It provides a simple way to integrate barcode scanning functionality into Android applications, offering both portrait and landscape scanning modes.
Pros
- Easy integration with minimal setup required
- Supports both ZXing and ZBar scanning engines
- Customizable UI elements and scan overlay
- Handles multiple barcode formats
Cons
- Limited to Android platform only
- May have performance issues on older devices
- Depends on external libraries (ZXing and ZBar)
- Not actively maintained (last update was in 2019)
Code Examples
- Basic barcode scanning:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
integrator.setPrompt("Scan a barcode");
integrator.setCameraId(0); // Use a specific camera of the device
integrator.setBeepEnabled(false);
integrator.setBarcodeImageEnabled(true);
integrator.initiateScan();
- Customizing scanner appearance:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setOrientationLocked(false);
integrator.setCaptureActivity(CustomScannerActivity.class);
integrator.initiateScan();
- Handling scan results:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
IntentResult result = IntentIntegrator.parseActivityResult(requestCode, resultCode, data);
if(result != null) {
if(result.getContents() == null) {
Toast.makeText(this, "Cancelled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Scanned: " + result.getContents(), Toast.LENGTH_LONG).show();
}
} else {
super.onActivityResult(requestCode, resultCode, data);
}
}
Getting Started
- Add the following to your
build.gradle
file:
dependencies {
implementation 'me.dm7.barcodescanner:zxing:1.9.13'
// For ZBar scanner, use:
// implementation 'me.dm7.barcodescanner:zbar:1.9.13'
}
- Add camera permission to your
AndroidManifest.xml
:
<uses-permission android:name="android.permission.CAMERA" />
- Implement barcode scanning in your activity:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button scanButton = findViewById(R.id.scan_button);
scanButton.setOnClickListener(v -> {
IntentIntegrator integrator = new IntentIntegrator(this);
integrator.initiateScan();
});
}
// Don't forget to implement onActivityResult as shown in the code examples
}
Competitor Comparisons
ZXing ("Zebra Crossing") barcode scanning library for Java, Android
Pros of ZXing
- More comprehensive barcode support, including 1D and 2D formats
- Actively maintained with regular updates and bug fixes
- Extensive documentation and community support
Cons of ZXing
- Larger library size, which may impact app size
- Steeper learning curve due to more complex API
Code Comparison
ZXing:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
integrator.setPrompt("Scan a barcode");
integrator.setCameraId(0);
integrator.initiateScan();
Barcodescanner:
scanner.setResultHandler(this);
scanner.startCamera();
scanner.setFlash(true);
scanner.setAutoFocus(true);
ZXing offers more configuration options but requires more setup code, while Barcodescanner provides a simpler API for basic scanning functionality. ZXing's extensive format support and active maintenance make it suitable for complex projects, whereas Barcodescanner's lightweight nature and ease of use are advantageous for simpler applications with basic barcode scanning needs.
Barcode scanner library for Android, based on the ZXing decoder
Pros of zxing-android-embedded
- More actively maintained with frequent updates
- Better integration with modern Android development practices
- Improved performance and scanning capabilities
Cons of zxing-android-embedded
- Larger library size due to additional features
- Steeper learning curve for beginners
- May require more configuration for basic use cases
Code Comparison
barcodescanner:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
integrator.setPrompt("Scan a barcode");
integrator.initiateScan();
zxing-android-embedded:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ONE_D_CODE_TYPES);
integrator.setPrompt("Scan a barcode");
integrator.setCameraId(0);
integrator.setBeepEnabled(false);
integrator.setBarcodeImageEnabled(true);
integrator.initiateScan();
The code comparison shows that zxing-android-embedded offers more customization options out of the box, such as camera selection and additional scanning features. However, this also means it requires more configuration for basic use cases compared to barcodescanner's simpler implementation.
Code scanner library for Android, based on ZXing
Pros of code-scanner
- More active development with recent updates and bug fixes
- Better support for modern Android features and API levels
- Improved performance and scanning accuracy
Cons of code-scanner
- Larger library size compared to barcodescanner
- May require more setup and configuration for basic use cases
Code Comparison
code-scanner:
val scannerView = findViewById<CodeScannerView>(R.id.scanner_view)
val codeScanner = CodeScanner(this, scannerView)
codeScanner.decodeCallback = DecodeCallback {
runOnUiThread {
Toast.makeText(this, "Scan result: ${it.text}", Toast.LENGTH_LONG).show()
}
}
scannerView.setOnClickListener {
codeScanner.startPreview()
}
barcodescanner:
ZXingScannerView mScannerView = new ZXingScannerView(this);
setContentView(mScannerView);
mScannerView.setResultHandler(this);
mScannerView.startCamera();
@Override
public void handleResult(Result rawResult) {
Toast.makeText(this, "Scan result: " + rawResult.getText(), Toast.LENGTH_SHORT).show();
mScannerView.resumeCameraPreview(this);
}
Both libraries offer similar functionality, but code-scanner provides a more modern, Kotlin-friendly API with improved performance and ongoing development. However, barcodescanner may be simpler to implement for basic use cases and has a smaller footprint.
Modification of ZXING Barcode Scanner project for easy Android QR-Code detection and AR purposes
Pros of QRCodeReaderView
- More focused on QR code scanning, potentially offering better performance for this specific use case
- Simpler API and easier integration for basic QR code scanning functionality
- More recent updates and active maintenance
Cons of QRCodeReaderView
- Limited to QR codes only, lacking support for other barcode formats
- Fewer customization options compared to barcodescanner's extensive features
- Smaller community and fewer third-party resources available
Code Comparison
QRCodeReaderView implementation:
qrCodeReaderView.setOnQRCodeReadListener(new QRCodeReaderView.OnQRCodeReadListener() {
@Override
public void onQRCodeRead(String text, PointF[] points) {
// Handle QR code result
}
});
barcodescanner implementation:
barcodeView.decodeSingle(new BarcodeCallback() {
@Override
public void barcodeResult(BarcodeResult result) {
// Handle barcode result
}
});
Both libraries offer straightforward ways to implement barcode scanning, with QRCodeReaderView focusing specifically on QR codes and barcodescanner providing a more versatile solution for various barcode formats. The choice between the two depends on the specific requirements of the project, such as the need for multiple barcode format support or a more lightweight QR code-only solution.
A cross platform HTML5 QR code reader. See end to end implementation at: https://scanapp.org
Pros of html5-qrcode
- Pure JavaScript implementation, no native dependencies required
- Works directly in web browsers without additional plugins
- Supports both front and back cameras on mobile devices
Cons of html5-qrcode
- Limited to QR codes and some 1D barcodes
- May have lower performance compared to native solutions
- Requires a secure (HTTPS) connection for camera access
Code Comparison
html5-qrcode:
const html5QrCode = new Html5Qrcode("reader");
html5QrCode.start(
{ facingMode: "environment" },
{ fps: 10, qrbox: 250 },
qrCodeSuccessCallback,
qrCodeErrorCallback
);
barcodescanner:
IntentIntegrator integrator = new IntentIntegrator(activity);
integrator.setDesiredBarcodeFormats(IntentIntegrator.ALL_CODE_TYPES);
integrator.setPrompt("Scan a barcode");
integrator.setCameraId(0);
integrator.initiateScan();
The html5-qrcode project offers a JavaScript-based solution for QR code scanning directly in web browsers, making it ideal for web applications. It's easy to implement and doesn't require native app development. However, it may have limitations in terms of supported barcode types and performance compared to native solutions like barcodescanner.
barcodescanner, being a native Android library, provides broader barcode format support and potentially better performance. It integrates seamlessly with Android apps but requires native development and is not suitable for web-based applications.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Project Archived
July 1 2020
This project is no longer maintained. When I first started this project in late 2013 there were very few libraries to help with barcode scanning on Android. But the situation today is much different. We have lots of great libraries based on ZXing and there is also barcode scanning API in Google's MLKit (https://github.com/googlesamples/mlkit). So given the options I have decided to stop working on this project.
Introduction
Android library projects that provides easy to use and extensible Barcode Scanner views based on ZXing and ZBar.
Screenshots
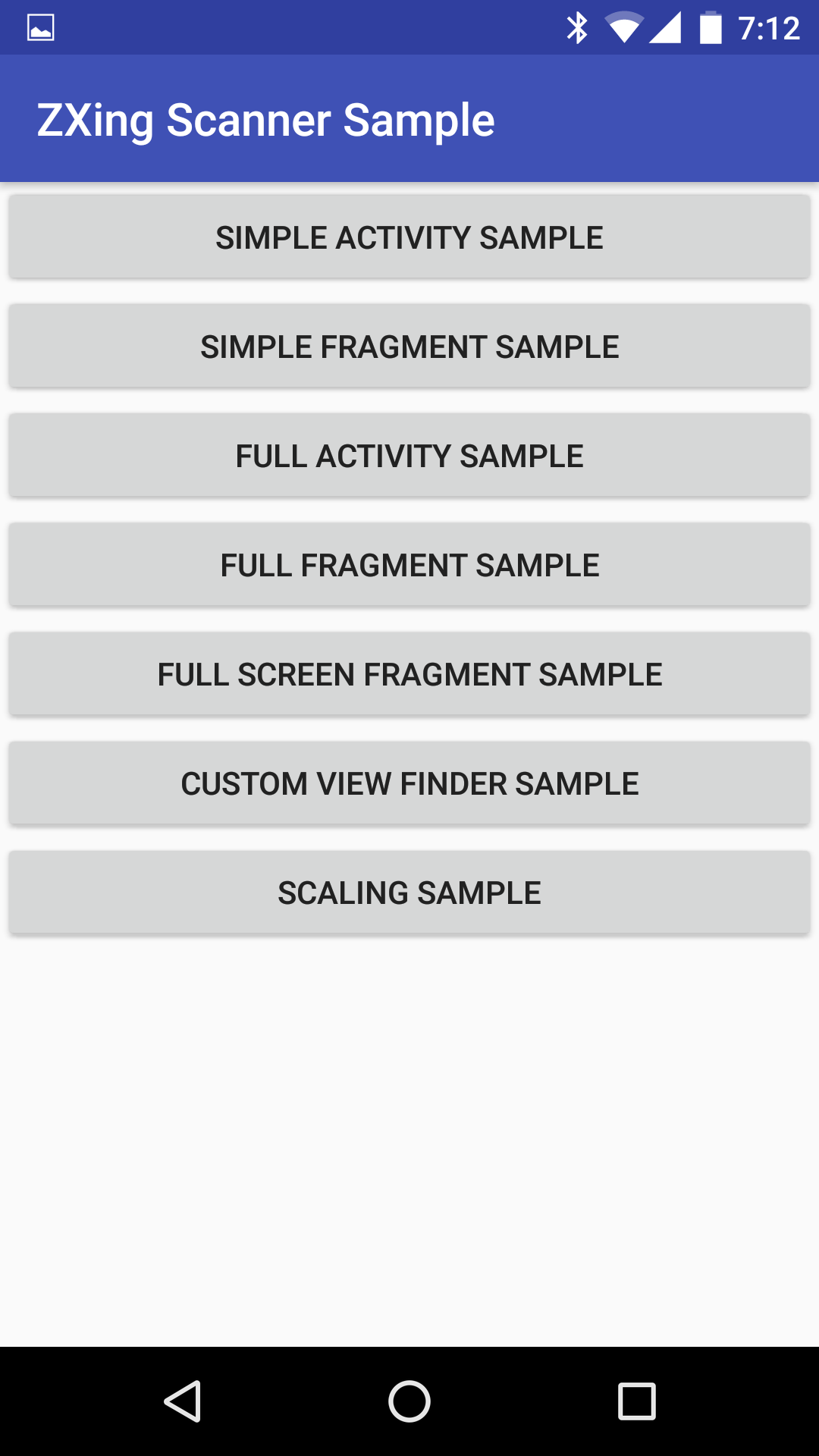
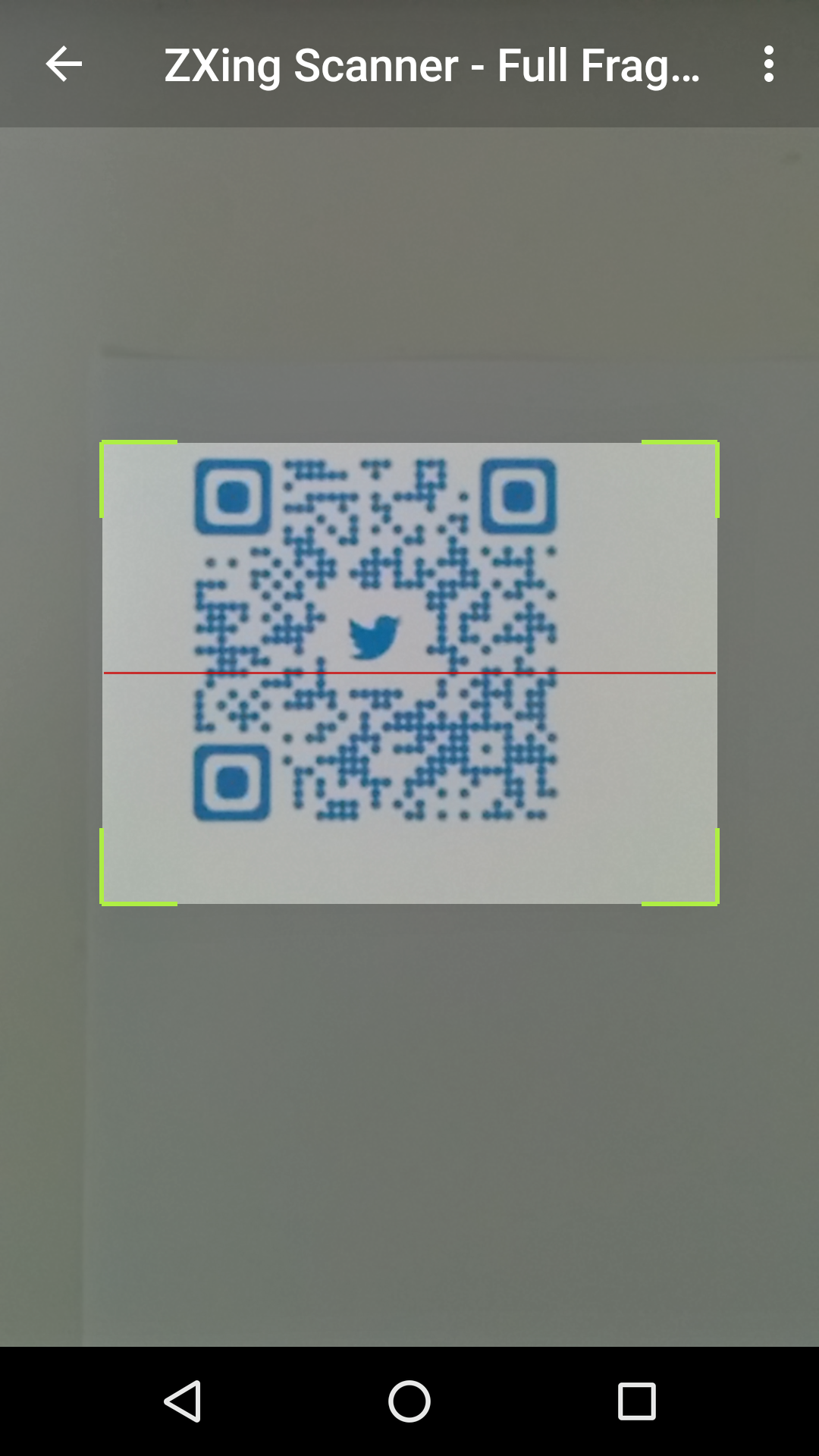
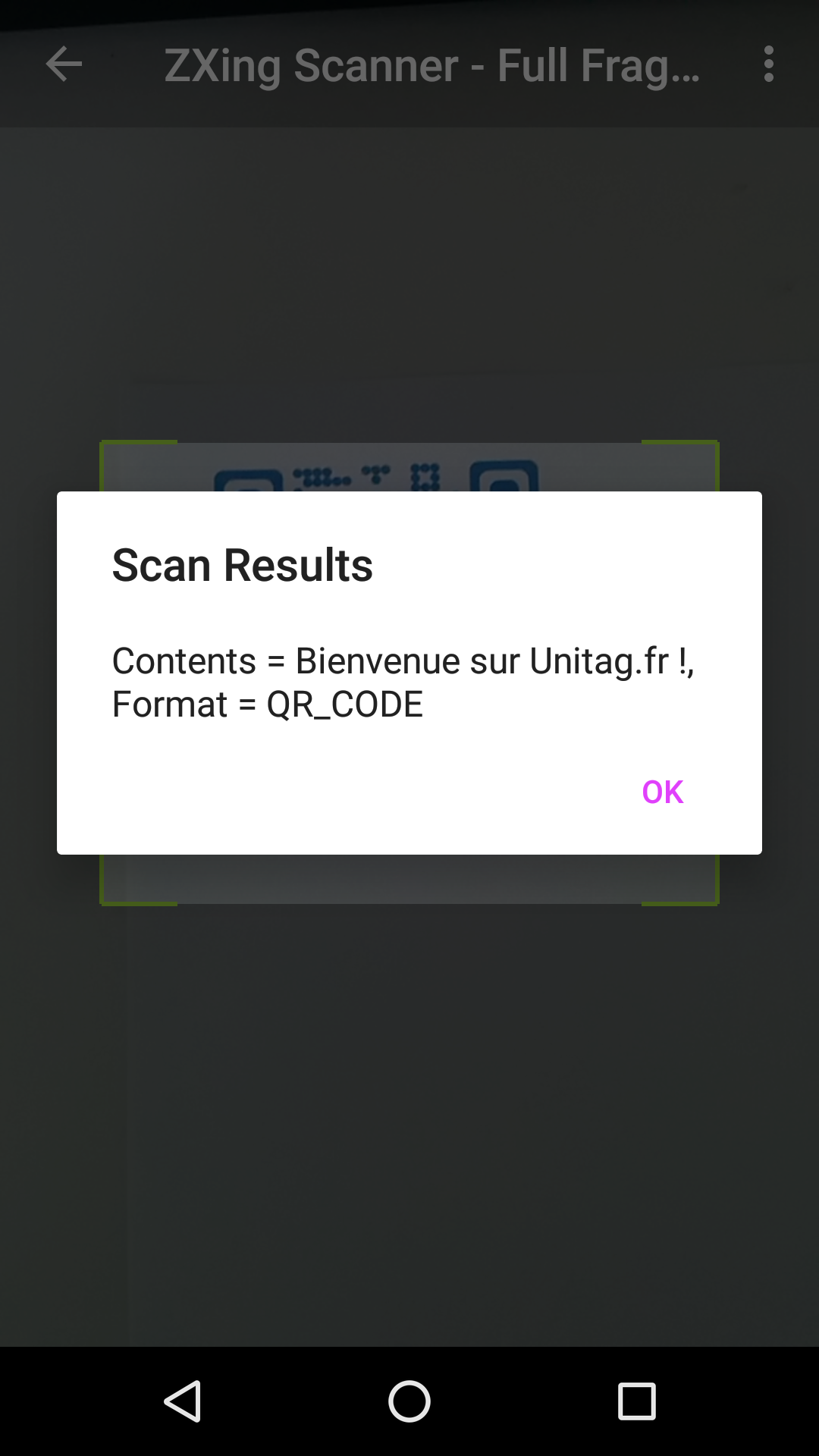
Minor BREAKING CHANGE in 1.8.4
Version 1.8.4 introduces a couple of new changes:
- Open Camera and handle preview frames in a separate HandlerThread (#1, #99): Though this has worked fine in my testing on 3 devices, I would advise you to test on your own devices before blindly releasing apps with this version. If you run into any issues please file a bug report.
- Do not automatically stopCamera after a result is found #115: This means that upon a successful scan only the cameraPreview is stopped but the camera is not released. So previously if your code was calling mScannerView.startCamera() in the handleResult() method, please replace that with a call to mScannerView.resumeCameraPreview(this);
ZXing
Installation
Add the following dependency to your build.gradle file.
repositories {
jcenter()
}
implementation 'me.dm7.barcodescanner:zxing:1.9.13'
Simple Usage
1.) Add camera permission to your AndroidManifest.xml file:
<uses-permission android:name="android.permission.CAMERA" />
2.) A very basic activity would look like this:
public class SimpleScannerActivity extends Activity implements ZXingScannerView.ResultHandler {
private ZXingScannerView mScannerView;
@Override
public void onCreate(Bundle state) {
super.onCreate(state);
mScannerView = new ZXingScannerView(this); // Programmatically initialize the scanner view
setContentView(mScannerView); // Set the scanner view as the content view
}
@Override
public void onResume() {
super.onResume();
mScannerView.setResultHandler(this); // Register ourselves as a handler for scan results.
mScannerView.startCamera(); // Start camera on resume
}
@Override
public void onPause() {
super.onPause();
mScannerView.stopCamera(); // Stop camera on pause
}
@Override
public void handleResult(Result rawResult) {
// Do something with the result here
Log.v(TAG, rawResult.getText()); // Prints scan results
Log.v(TAG, rawResult.getBarcodeFormat().toString()); // Prints the scan format (qrcode, pdf417 etc.)
// If you would like to resume scanning, call this method below:
mScannerView.resumeCameraPreview(this);
}
}
Please take a look at the zxing-sample project for a full working example.
Advanced Usage
Take a look at the FullScannerActivity.java or FullScannerFragment.java classes to get an idea on advanced usage.
Interesting methods on the ZXingScannerView include:
// Toggle flash:
void setFlash(boolean);
// Toogle autofocus:
void setAutoFocus(boolean);
// Specify interested barcode formats:
void setFormats(List<BarcodeFormat> formats);
// Specify the cameraId to start with:
void startCamera(int cameraId);
Specify front-facing or rear-facing cameras by using the void startCamera(int cameraId);
method.
For HUAWEI mobile phone like P9, P10, when scanning using the default settings, it won't work due to the "preview size", please adjust the parameter as below:
mScannerView = (ZXingScannerView) findViewById(R.id.zx_view);
// this paramter will make your HUAWEI phone works great!
mScannerView.setAspectTolerance(0.5f);
Supported Formats:
BarcodeFormat.UPC_A
BarcodeFormat.UPC_E
BarcodeFormat.EAN_13
BarcodeFormat.EAN_8
BarcodeFormat.RSS_14
BarcodeFormat.CODE_39
BarcodeFormat.CODE_93
BarcodeFormat.CODE_128
BarcodeFormat.ITF
BarcodeFormat.CODABAR
BarcodeFormat.QR_CODE
BarcodeFormat.DATA_MATRIX
BarcodeFormat.PDF_417
ZBar
Installation
Add the following dependency to your build.gradle file.
repositories {
jcenter()
}
implementation 'me.dm7.barcodescanner:zbar:1.9.13'
Simple Usage
1.) Add camera permission to your AndroidManifest.xml file:
<uses-permission android:name="android.permission.CAMERA" />
2.) A very basic activity would look like this:
public class SimpleScannerActivity extends Activity implements ZBarScannerView.ResultHandler {
private ZBarScannerView mScannerView;
@Override
public void onCreate(Bundle state) {
super.onCreate(state);
mScannerView = new ZBarScannerView(this); // Programmatically initialize the scanner view
setContentView(mScannerView); // Set the scanner view as the content view
}
@Override
public void onResume() {
super.onResume();
mScannerView.setResultHandler(this); // Register ourselves as a handler for scan results.
mScannerView.startCamera(); // Start camera on resume
}
@Override
public void onPause() {
super.onPause();
mScannerView.stopCamera(); // Stop camera on pause
}
@Override
public void handleResult(Result rawResult) {
// Do something with the result here
Log.v(TAG, rawResult.getContents()); // Prints scan results
Log.v(TAG, rawResult.getBarcodeFormat().getName()); // Prints the scan format (qrcode, pdf417 etc.)
// If you would like to resume scanning, call this method below:
mScannerView.resumeCameraPreview(this);
}
}
Please take a look at the zbar-sample project for a full working example.
Advanced Usage
Take a look at the FullScannerActivity.java or FullScannerFragment.java classes to get an idea on advanced usage.
Interesting methods on the ZBarScannerView include:
// Toggle flash:
void setFlash(boolean);
// Toogle autofocus:
void setAutoFocus(boolean);
// Specify interested barcode formats:
void setFormats(List<BarcodeFormat> formats);
Specify front-facing or rear-facing cameras by using the void startCamera(int cameraId);
method.
Supported Formats:
BarcodeFormat.PARTIAL
BarcodeFormat.EAN8
BarcodeFormat.UPCE
BarcodeFormat.ISBN10
BarcodeFormat.UPCA
BarcodeFormat.EAN13
BarcodeFormat.ISBN13
BarcodeFormat.I25
BarcodeFormat.DATABAR
BarcodeFormat.DATABAR_EXP
BarcodeFormat.CODABAR
BarcodeFormat.CODE39
BarcodeFormat.PDF417
BarcodeFormat.QR_CODE
BarcodeFormat.CODE93
BarcodeFormat.CODE128
Rebuilding ZBar Libraries
mkdir some_work_dir
cd work_dir
wget http://ftp.gnu.org/pub/gnu/libiconv/libiconv-1.14.tar.gz
tar zxvf libiconv-1.14.tar.gz
Patch the localcharset.c file: vim libiconv-1.14/libcharset/lib/localcharset.c
On line 48, add the following line of code:
#undef HAVE_LANGINFO_CODESET
Save the file and continue with steps below:
cd libiconv-1.14
./configure
cd ..
hg clone http://hg.code.sf.net/p/zbar/code zbar-code
cd zbar-code/android
android update project -p . -t 'android-19'
Open jni/Android.mk file and add fPIC flag to LOCAL_C_FLAGS. Open jni/Application.mk file and specify APP_ABI targets as needed.
ant -Dndk.dir=$NDK_HOME -Diconv.src=some_work_dir/libiconv-1.14 zbar-clean zbar-all
Upon completion you can grab the .so and .jar files from the libs folder.
Credits
Almost all of the code for these library projects is based on:
- CameraPreview app from Android SDK APIDemos
- The ZXing project: https://github.com/zxing/zxing
- The ZBar Android SDK: https://github.com/ZBar/ZBar/tree/master/android (Previously: http://sourceforge.net/projects/zbar/files/AndroidSDK/)
Contributors
https://github.com/dm77/barcodescanner/graphs/contributors
License
License for code written in this project is: Apache License, Version 2.0
License for zxing and zbar projects is here:
Top Related Projects
ZXing ("Zebra Crossing") barcode scanning library for Java, Android
Barcode scanner library for Android, based on the ZXing decoder
Code scanner library for Android, based on ZXing
Modification of ZXING Barcode Scanner project for easy Android QR-Code detection and AR purposes
A cross platform HTML5 QR code reader. See end to end implementation at: https://scanapp.org
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot