flet
Flet enables developers to easily build realtime web, mobile and desktop apps in Python. No frontend experience required.
Top Related Projects
Streamlit β A faster way to build and share data apps.
Data Apps & Dashboards for Python. No JavaScript Required.
Build and share delightful machine learning apps, all in Python. π Star to support our work!
πΈοΈ Web apps in pure Python π
Panel: The powerful data exploration & web app framework for Python
Write interactive web app in script way.
Quick Overview
Flet is a framework for building interactive multi-platform applications using Python. It allows developers to create user interfaces with Flutter's widgets while writing all the application logic in Python. Flet supports deploying applications as native apps, progressive web apps, or hosting them on Flet's servers.
Pros
- Enables Python developers to create rich, interactive UIs without learning new languages or frameworks
- Supports multiple platforms including desktop, web, and mobile
- Utilizes Flutter's extensive widget library for creating visually appealing interfaces
- Offers a simple and intuitive API for building responsive layouts
Cons
- Limited customization options compared to direct Flutter development
- Performance may be slower than native Flutter apps due to the Python layer
- Relatively new project, which may lead to potential instability or lack of community resources
- Dependency on both Python and Flutter ecosystems
Code Examples
- Creating a simple counter app:
import flet as ft
def main(page: ft.Page):
txt_number = ft.TextField(value="0", text_align="right", width=100)
def minus_click(e):
txt_number.value = str(int(txt_number.value) - 1)
page.update()
def plus_click(e):
txt_number.value = str(int(txt_number.value) + 1)
page.update()
page.add(
ft.Row(
[
ft.IconButton(ft.icons.REMOVE, on_click=minus_click),
txt_number,
ft.IconButton(ft.icons.ADD, on_click=plus_click),
],
alignment=ft.MainAxisAlignment.CENTER,
)
)
ft.app(target=main)
- Creating a simple todo list:
import flet as ft
def main(page: ft.Page):
def add_clicked(e):
page.add(ft.Checkbox(label=new_task.value))
new_task.value = ""
new_task.focus()
new_task.update()
new_task = ft.TextField(hint_text="Whats needs to be done?", width=300)
page.add(ft.Row([new_task, ft.ElevatedButton("Add", on_click=add_clicked)]))
ft.app(target=main)
- Creating a responsive grid layout:
import flet as ft
def main(page: ft.Page):
page.add(
ft.ResponsiveRow(
[
ft.Container(ft.Text("1"), padding=5, bgcolor=ft.colors.YELLOW, col={"sm": 6, "md": 4, "xl": 2}),
ft.Container(ft.Text("2"), padding=5, bgcolor=ft.colors.GREEN, col={"sm": 6, "md": 4, "xl": 2}),
ft.Container(ft.Text("3"), padding=5, bgcolor=ft.colors.BLUE, col={"sm": 6, "md": 4, "xl": 2}),
ft.Container(ft.Text("4"), padding=5, bgcolor=ft.colors.PINK, col={"sm": 6, "md": 4, "xl": 2}),
]
)
)
ft.app(target=main)
Getting Started
To get started with Flet, follow these steps:
-
Install Flet using pip:
pip install flet
-
Create a new Python file (e.g.,
main.py
) and import Flet:import flet as ft
-
Define your main function and create your UI:
def main(page: ft.Page): page.add(ft.Text("Hello, Flet!")) ft.app(target=main)
-
Run your application:
python main.py
This will launch a simple Flet application with a "Hello, Flet!" message.
Competitor Comparisons
Streamlit β A faster way to build and share data apps.
Pros of Streamlit
- More mature and established project with a larger community and ecosystem
- Extensive documentation and tutorials available
- Built-in support for data visualization and machine learning libraries
Cons of Streamlit
- Limited customization options for UI components
- Performance can be slower for complex applications
- Primarily designed for data science and ML applications, less flexible for general-purpose apps
Code Comparison
Streamlit:
import streamlit as st
st.title("Hello World")
name = st.text_input("Enter your name")
st.write(f"Hello, {name}!")
Flet:
import flet as ft
def main(page: ft.Page):
page.title = "Hello World"
name_input = ft.TextField(label="Enter your name")
greeting = ft.Text()
page.add(name_input, greeting)
ft.app(target=main)
Both frameworks aim to simplify GUI development in Python, but Flet offers a more flexible approach for creating general-purpose applications. Streamlit excels in data science and machine learning scenarios, while Flet provides a wider range of UI components and layout options. Streamlit's syntax is more concise, but Flet's object-oriented approach may be more familiar to developers with experience in other GUI frameworks.
Data Apps & Dashboards for Python. No JavaScript Required.
Pros of Dash
- Mature ecosystem with extensive documentation and community support
- Powerful interactive visualization capabilities through Plotly
- Seamless integration with data analysis libraries like Pandas and NumPy
Cons of Dash
- Steeper learning curve, especially for developers new to web technologies
- More complex setup and deployment process
- Heavier resource usage, which may impact performance on low-end devices
Code Comparison
Dash example:
import dash
import dash_core_components as dcc
import dash_html_components as html
app = dash.Dash(__name__)
app.layout = html.Div([
html.H1('Hello Dash'),
dcc.Graph(id='example-graph')
])
Flet example:
import flet as ft
def main(page: ft.Page):
page.add(ft.Text("Hello, Flet!"))
ft.app(target=main)
Flet offers a more straightforward approach for creating simple UIs, while Dash provides more advanced features for data visualization and complex web applications. Flet's syntax is more Pythonic and easier for beginners, whereas Dash requires knowledge of HTML and CSS concepts. Both frameworks have their strengths, with Dash excelling in data-driven applications and Flet focusing on rapid UI development across multiple platforms.
Build and share delightful machine learning apps, all in Python. π Star to support our work!
Pros of Gradio
- Specialized for machine learning model demos and interfaces
- Supports a wide range of input/output types (text, image, audio, video)
- Easier to create quick prototypes for AI/ML applications
Cons of Gradio
- Less flexible for general-purpose GUI applications
- Limited customization options for UI components
- Primarily focused on web-based interfaces
Code Comparison
Gradio example:
import gradio as gr
def greet(name):
return "Hello " + name + "!"
demo = gr.Interface(fn=greet, inputs="text", outputs="text")
demo.launch()
Flet example:
import flet as ft
def main(page: ft.Page):
def btn_click(e):
page.add(ft.Text(f"Hello, {txt_name.value}!"))
txt_name = ft.TextField(label="Your name")
page.add(txt_name, ft.ElevatedButton("Say hello!", on_click=btn_click))
ft.app(target=main)
Both Flet and Gradio aim to simplify GUI creation, but they cater to different use cases. Gradio excels in rapidly prototyping interfaces for machine learning models, while Flet offers more flexibility for general-purpose desktop and web applications. The code examples demonstrate the different approaches: Gradio focuses on defining inputs and outputs for a function, while Flet provides more control over UI components and layout.
πΈοΈ Web apps in pure Python π
Pros of Reflex
- Utilizes Python for both frontend and backend, allowing full-stack development in a single language
- Supports hot reloading, enabling faster development iterations
- Offers a more flexible and customizable UI framework
Cons of Reflex
- Steeper learning curve for developers new to reactive programming concepts
- Limited ecosystem compared to more established frameworks
- May have performance limitations for complex applications
Code Comparison
Reflex:
import reflex as rx
def index():
return rx.vstack(
rx.heading("Hello, Reflex!"),
rx.text("Welcome to my app.")
)
app = rx.App()
app.add_page(index)
Flet:
import flet as ft
def main(page: ft.Page):
page.add(
ft.Text("Hello, Flet!"),
ft.ElevatedButton("Welcome to my app")
)
ft.app(target=main)
Both frameworks aim to simplify Python-based UI development, but they differ in their approach. Reflex focuses on a reactive programming model, while Flet provides a more traditional UI toolkit. Reflex may be better suited for developers familiar with modern web frameworks, while Flet offers a simpler entry point for those new to GUI development in Python.
Panel: The powerful data exploration & web app framework for Python
Pros of Panel
- Extensive data visualization capabilities with support for various plotting libraries
- Seamless integration with Python data science ecosystem (NumPy, Pandas, etc.)
- More mature project with a larger community and extensive documentation
Cons of Panel
- Steeper learning curve, especially for those new to data visualization
- Primarily focused on data-driven applications, which may be overkill for simpler UIs
- Heavier dependency footprint compared to Flet
Code Comparison
Panel example:
import panel as pn
import numpy as np
pn.extension()
slider = pn.widgets.FloatSlider(start=0, end=10, step=0.1, value=5)
plot = pn.pane.Matplotlib(pn.bind(lambda x: plt.plot(np.sin(np.linspace(0, x))), slider))
pn.Column(slider, plot).servable()
Flet example:
import flet as ft
def main(page: ft.Page):
txt_number = ft.TextField(value="0", text_align="right", width=100)
page.add(txt_number)
ft.app(target=main)
Both frameworks offer Python-based UI development, but Panel excels in data visualization while Flet provides a more straightforward approach for general-purpose UI creation.
Write interactive web app in script way.
Pros of PyWebIO
- Simpler syntax for basic web applications
- Built-in support for async operations
- Easier to integrate with existing Python code
Cons of PyWebIO
- Less flexible for complex UI layouts
- Limited customization options for styling
- Smaller community and ecosystem compared to Flet
Code Comparison
PyWebIO example:
from pywebio.input import input
from pywebio.output import put_text
def main():
name = input("What's your name?")
put_text(f"Hello, {name}!")
if __name__ == '__main__':
main()
Flet example:
import flet as ft
def main(page: ft.Page):
def btn_click(e):
page.add(ft.Text(f"Hello, {txt.value}!"))
txt = ft.TextField(label="Your name")
page.add(txt, ft.ElevatedButton("Say hello!", on_click=btn_click))
ft.app(target=main)
Both frameworks aim to simplify web application development in Python, but they take different approaches. PyWebIO focuses on rapid prototyping and simple input/output operations, while Flet provides a more comprehensive UI toolkit with greater flexibility for complex layouts and interactions. PyWebIO's syntax is generally more concise for basic tasks, but Flet offers more control over the application's appearance and behavior.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Flet
Flet is a framework that enables you to easily build real-time web, mobile, and desktop apps in your favorite language and securely share them with your team. No frontend experience is required.
Γ’ΒΒ‘From idea to app in minutes
An internal tool or a dashboard for your team, weekend project, data entry form, kiosk app, or high-fidelity prototype - Flet is an ideal framework to quickly hack great-looking interactive apps to serve a group of users.
Γ°ΒΒΒ Simple architecture
No more complex architecture with JavaScript frontend, REST API backend, database, cache, etc. With Flet you just write a monolith stateful app in Python only and get multi-user, real-time Single-Page Application (SPA).
Γ°ΒΒΒBatteries included
To start developing with Flet, you just need your favorite IDE or text editor. No SDKs, no thousands of dependencies, no complex tooling - Flet has a built-in web server with assets hosting and desktop clients.
Β
Β Β Powered by Flutter
Flet UI is built with Flutter, so your app looks professional and could be delivered to any platform. Flet simplifies the Flutter model by combining smaller "widgets" to ready-to-use "controls" with an imperative programming model.
Γ°ΒΒΒ Speaks your language
Flet is language-agnostic, so anyone on your team could develop Flet apps in their favorite language. Python is already supported, Go, C# and others are coming next.
Γ°ΒΒΒ± Deliver to any device
Deploy Flet app as a web app and view it in a browser. Package it as a standalone desktop app for Windows, macOS, and Linux. Install it on mobile as PWA or view via Flet app for iOS and Android.
Flet app example
At the moment you can write Flet apps in Python and other languages will be added soon.
Here is a sample "Counter" app:
import flet
from flet import IconButton, Page, Row, TextField, icons
def main(page: Page):
page.title = "Flet counter example"
page.vertical_alignment = "center"
txt_number = TextField(value="0", text_align="right", width=100)
def minus_click(e):
txt_number.value = str(int(txt_number.value) - 1)
page.update()
def plus_click(e):
txt_number.value = str(int(txt_number.value) + 1)
page.update()
page.add(
Row(
[
IconButton(icons.REMOVE, on_click=minus_click),
txt_number,
IconButton(icons.ADD, on_click=plus_click),
],
alignment="center",
)
)
flet.app(target=main)
To run the app install flet
module:
pip install flet
and run the program:
python counter.py
The app will be started in a native OS window - what a nice alternative to Electron!
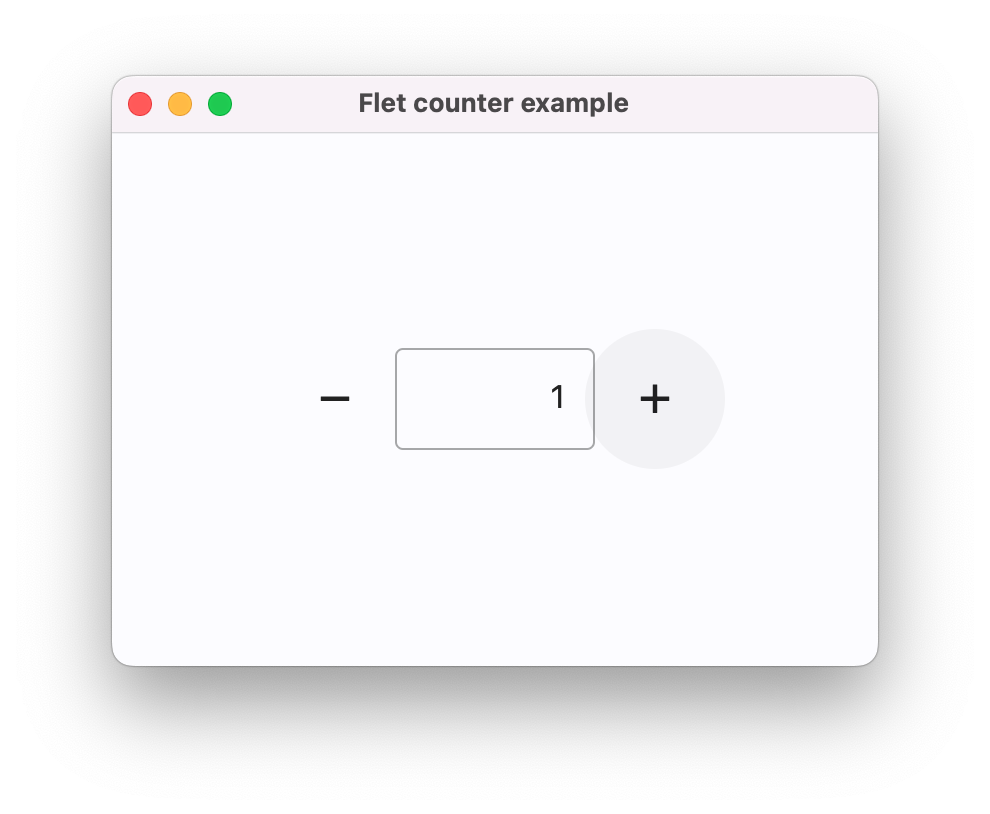
Now, if you want to run the app as a web app, just replace the last line with:
flet.app(target=main, view=flet.AppView.WEB_BROWSER)
run again and now you instantly get a web app:
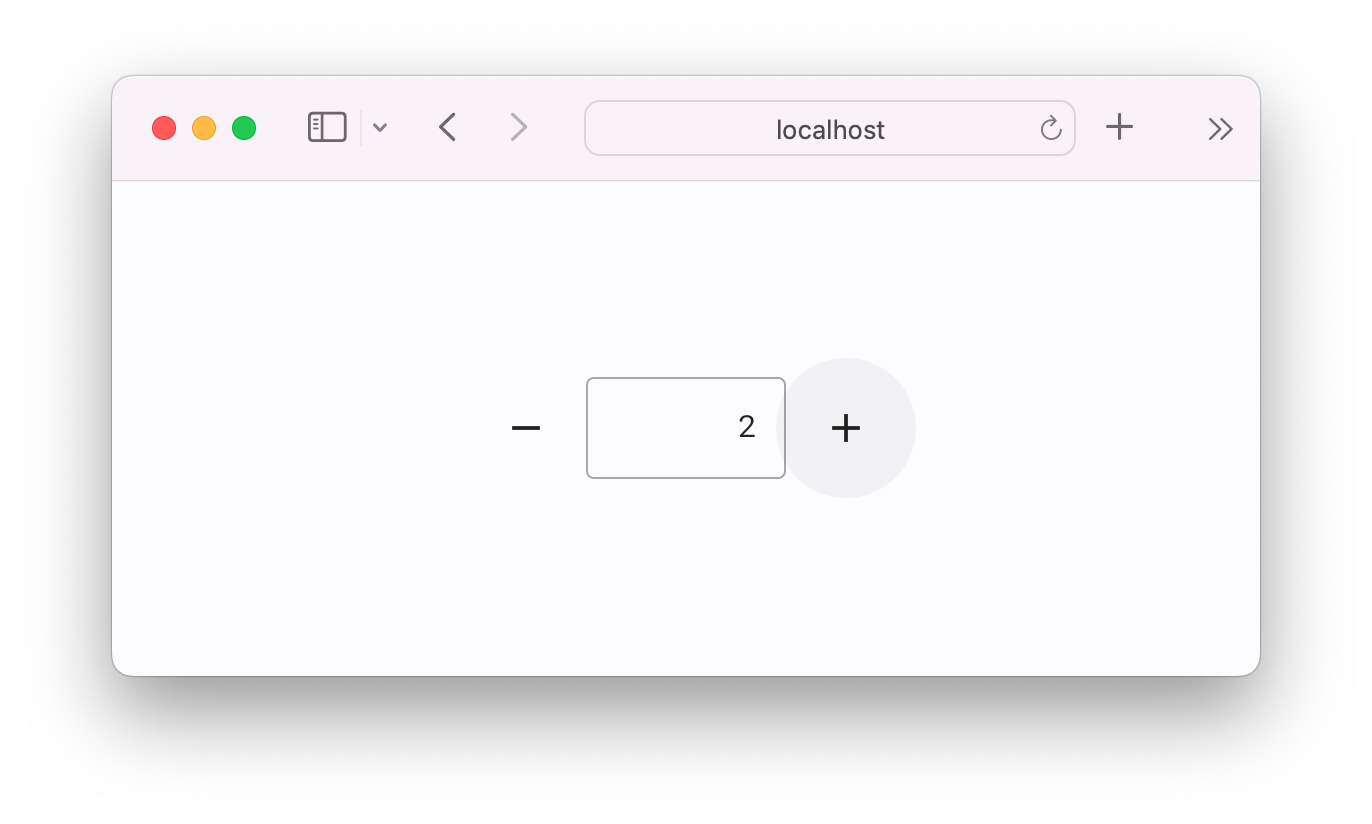
Getting started
Sample apps in Python
More demo applications can be found in the gallery.
Community
Contribute to this wonderful project
- Read the CONTRIBUTING.md file
Top Related Projects
Streamlit β A faster way to build and share data apps.
Data Apps & Dashboards for Python. No JavaScript Required.
Build and share delightful machine learning apps, all in Python. π Star to support our work!
πΈοΈ Web apps in pure Python π
Panel: The powerful data exploration & web app framework for Python
Write interactive web app in script way.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot