gin
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin.
Top Related Projects
High performance, minimalist Go web framework
⚡️ Express inspired web framework written in Go
A high performance HTTP request router that scales well
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
lightweight, idiomatic and composable router for building Go HTTP services
Fast HTTP package for Go. Tuned for high performance. Zero memory allocations in hot paths. Up to 10x faster than net/http
Quick Overview
Gin is a high-performance HTTP web framework written in Go (Golang). It features a martini-like API with much better performance, up to 40 times faster. Gin is designed to be simple, fast, and flexible, making it ideal for building web applications and microservices.
Pros
- Extremely fast and efficient, with minimal memory footprint
- Easy to learn and use, with a simple and intuitive API
- Extensive middleware support for customization and extensibility
- Built-in support for JSON validation and rendering
Cons
- Less mature compared to some other Go web frameworks
- Limited built-in templating options
- Smaller community and ecosystem compared to more established frameworks
- May be overkill for very simple applications
Code Examples
- Basic HTTP server:
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
- Handling URL parameters:
func main() {
router := gin.Default()
router.GET("/user/:name", func(c *gin.Context) {
name := c.Param("name")
c.String(http.StatusOK, "Hello %s", name)
})
router.Run(":8080")
}
- Using middleware:
func main() {
r := gin.New()
r.Use(gin.Logger())
r.Use(gin.Recovery())
r.GET("/", func(c *gin.Context) {
c.String(200, "Hello World!")
})
r.Run(":8080")
}
Getting Started
To start using Gin, follow these steps:
- Install Gin:
go get -u github.com/gin-gonic/gin
- Create a new Go file (e.g.,
main.go
) and add the following code:
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.String(200, "Hello, Gin!")
})
r.Run() // listen and serve on 0.0.0.0:8080
}
- Run your application:
go run main.go
- Open your browser and visit
http://localhost:8080
to see your Gin application in action.
Competitor Comparisons
High performance, minimalist Go web framework
Pros of Echo
- More feature-rich with built-in middleware for JWT, CORS, and rate limiting
- Better documentation and more comprehensive examples
- Slightly faster performance in some benchmarks
Cons of Echo
- Larger codebase and slightly steeper learning curve
- Less community adoption and fewer third-party packages
- More opinionated structure, which may limit flexibility in some cases
Code Comparison
Echo:
e := echo.New()
e.GET("/", func(c echo.Context) error {
return c.String(http.StatusOK, "Hello, World!")
})
e.Logger.Fatal(e.Start(":1323"))
Gin:
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.String(http.StatusOK, "Hello, World!")
})
r.Run(":8080")
Both Echo and Gin are popular Go web frameworks with similar syntax and functionality. Echo offers more built-in features and middleware, while Gin is known for its simplicity and performance. The choice between the two often comes down to personal preference and specific project requirements. Echo's more comprehensive feature set may be beneficial for larger projects, while Gin's simplicity and performance make it attractive for smaller applications or microservices.
⚡️ Express inspired web framework written in Go
Pros of Fiber
- Higher performance and lower memory usage
- Built-in WebSocket support
- More extensive middleware ecosystem
Cons of Fiber
- Less mature and stable compared to Gin
- Smaller community and fewer third-party integrations
- Steeper learning curve for developers familiar with net/http
Code Comparison
Gin example:
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{"message": "pong"})
})
r.Run()
Fiber example:
app := fiber.New()
app.Get("/ping", func(c *fiber.Ctx) error {
return c.JSON(fiber.Map{"message": "pong"})
})
app.Listen(":3000")
Both frameworks offer similar syntax for routing and handling requests. Fiber uses a more modern approach with error handling and a custom context type. Gin follows a pattern closer to the standard net/http library, which may be more familiar to Go developers.
While Fiber boasts higher performance, Gin's maturity and extensive community support make it a reliable choice for many projects. The decision between the two often depends on specific project requirements and developer preferences.
A high performance HTTP request router that scales well
Pros of httprouter
- Extremely lightweight and fast, with minimal overhead
- Simple and straightforward API, easy to learn and use
- Excellent performance for basic routing needs
Cons of httprouter
- Limited middleware support compared to Gin
- Fewer built-in features and utilities
- Less active community and ecosystem
Code Comparison
httprouter:
router := httprouter.New()
router.GET("/", Index)
router.GET("/hello/:name", Hello)
log.Fatal(http.ListenAndServe(":8080", router))
Gin:
router := gin.Default()
router.GET("/", Index)
router.GET("/hello/:name", Hello)
router.Run(":8080")
Summary
httprouter is a minimalist, high-performance HTTP router for Go, focusing on speed and simplicity. It's ideal for projects that require basic routing without additional features. Gin, built on top of httprouter, offers a more comprehensive framework with middleware support, input validation, and a larger ecosystem. While httprouter excels in raw performance, Gin provides a richer set of tools and abstractions for building web applications. The choice between the two depends on the specific needs of your project, balancing between simplicity and feature set.
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
Pros of Mux
- More flexible routing with support for complex URL patterns and variables
- Better integration with standard Go HTTP library
- Lightweight and minimalistic, allowing for more control over the application structure
Cons of Mux
- Less built-in functionality compared to Gin
- Slower performance in high-load scenarios
- Requires more manual setup for common web application features
Code Comparison
Mux:
r := mux.NewRouter()
r.HandleFunc("/users/{id:[0-9]+}", GetUser).Methods("GET")
http.ListenAndServe(":8080", r)
Gin:
r := gin.Default()
r.GET("/users/:id", GetUser)
r.Run(":8080")
Both Gin and Mux are popular Go web frameworks, but they have different focuses. Gin is designed for high performance and comes with more built-in features, making it easier to quickly develop web applications. Mux, on the other hand, offers more flexibility in routing and integrates seamlessly with the standard Go HTTP library, giving developers more control over their application structure.
Gin provides better performance out of the box, especially in high-load scenarios, and includes features like middleware support and JSON validation. Mux is more lightweight and allows for more complex routing patterns, which can be beneficial for applications with intricate URL structures.
The choice between Gin and Mux often depends on the specific requirements of the project, such as performance needs, routing complexity, and desired level of control over the application structure.
lightweight, idiomatic and composable router for building Go HTTP services
Pros of Chi
- Lightweight and minimalist design, offering more flexibility
- Follows standard Go HTTP conventions, making it easier for Go developers
- Built-in support for middleware chaining and context-based request handling
Cons of Chi
- Less feature-rich out of the box compared to Gin
- Smaller community and ecosystem
- Requires more manual setup for certain functionalities
Code Comparison
Chi:
r := chi.NewRouter()
r.Get("/", func(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Welcome"))
})
Gin:
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.String(http.StatusOK, "Welcome")
})
Both Chi and Gin are popular Go web frameworks, but they have different philosophies. Chi focuses on simplicity and adhering to Go's standard library patterns, while Gin offers more built-in features and performance optimizations. Chi's approach may appeal to developers who prefer a minimalist framework that closely follows Go idioms, whereas Gin might be more suitable for those seeking a feature-rich solution with extensive middleware support out of the box.
Fast HTTP package for Go. Tuned for high performance. Zero memory allocations in hot paths. Up to 10x faster than net/http
Pros of fasthttp
- Significantly higher performance and lower memory usage
- Optimized for high-concurrency scenarios
- Provides low-level control over HTTP operations
Cons of fasthttp
- Steeper learning curve due to its low-level nature
- Less extensive middleware ecosystem compared to Gin
- Not fully compatible with net/http, requiring some code adjustments
Code Comparison
fasthttp example:
func handleFastHTTP(ctx *fasthttp.RequestCtx) {
fmt.Fprintf(ctx, "Hello, %s!", ctx.UserValue("name"))
}
fasthttp.ListenAndServe(":8080", handleFastHTTP)
Gin example:
r := gin.Default()
r.GET("/hello/:name", func(c *gin.Context) {
name := c.Param("name")
c.String(http.StatusOK, "Hello, %s!", name)
})
r.Run(":8080")
fasthttp focuses on raw performance and low-level control, while Gin provides a more user-friendly API with built-in features like routing and middleware support. fasthttp excels in high-concurrency scenarios but requires more manual configuration. Gin offers a gentler learning curve and better compatibility with the standard library, making it suitable for a wider range of applications.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Gin Web Framework
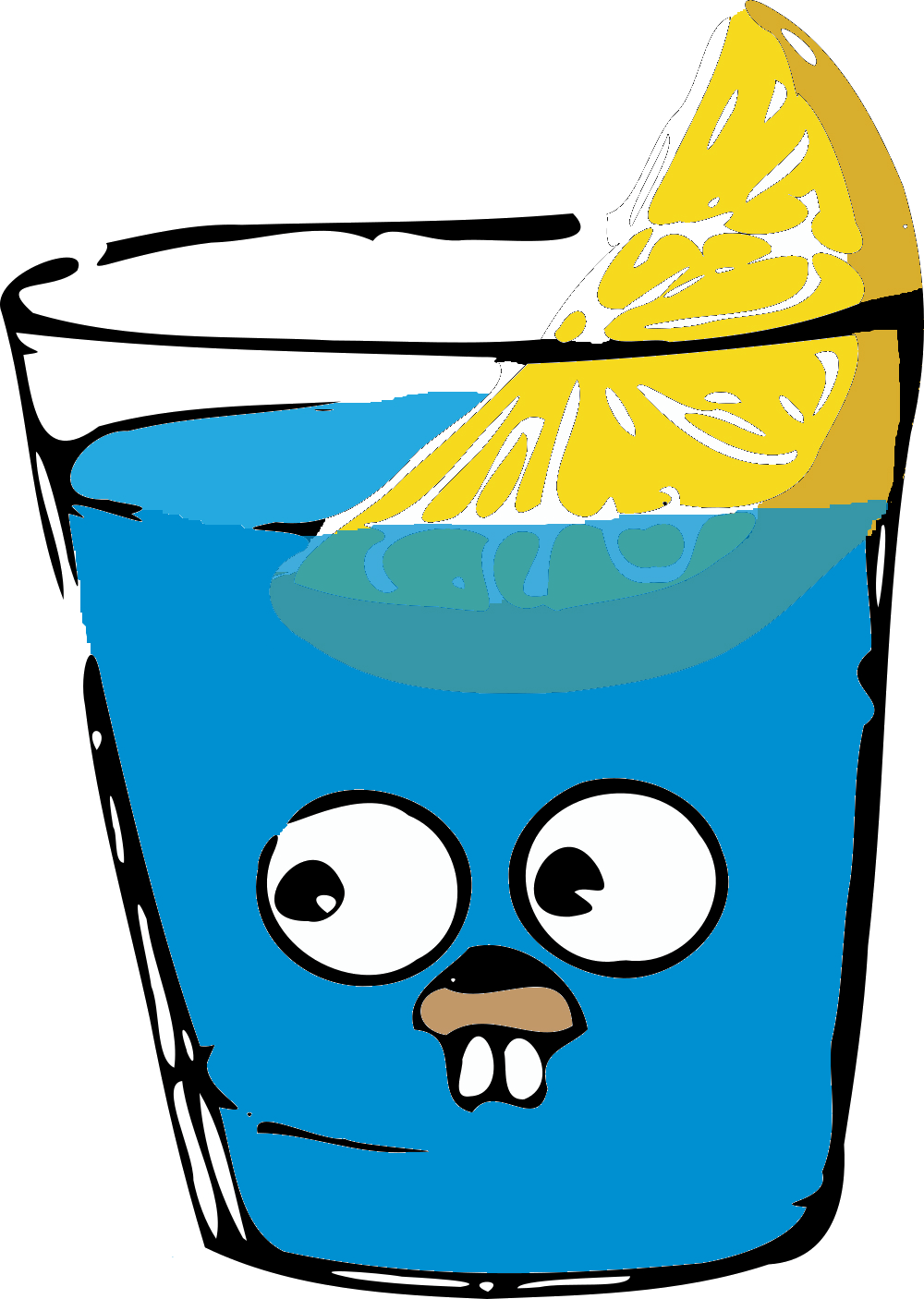
Gin is a web framework written in Go. It features a martini-like API with performance that is up to 40 times faster thanks to httprouter. If you need performance and good productivity, you will love Gin.
Gin's key features are:
- Zero allocation router
- Speed
- Middleware support
- Crash-free
- JSON validation
- Route grouping
- Error management
- Built-in rendering
- Extensible
Getting started
Prerequisites
Gin requires Go version 1.23 or above.
Getting Gin
With Go's module support, go [build|run|test]
automatically fetches the necessary dependencies when you add the import in your code:
import "github.com/gin-gonic/gin"
Alternatively, use go get
:
go get -u github.com/gin-gonic/gin
Running Gin
A basic example:
package main
import (
"net/http"
"github.com/gin-gonic/gin"
)
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080 (for windows "localhost:8080")
}
To run the code, use the go run
command, like:
go run example.go
Then visit 0.0.0.0:8080/ping
in your browser to see the response!
See more examples
Quick Start
Learn and practice with the Gin Quick Start, which includes API examples and builds tag.
Examples
A number of ready-to-run examples demonstrating various use cases of Gin are available in the Gin examples repository.
Documentation
See the API documentation on go.dev.
The documentation is also available on gin-gonic.com in several languages:
Articles
Benchmarks
Gin uses a custom version of HttpRouter, see all benchmarks.
Benchmark name | (1) | (2) | (3) | (4) |
---|---|---|---|---|
BenchmarkGin_GithubAll | 43550 | 27364 ns/op | 0 B/op | 0 allocs/op |
BenchmarkAce_GithubAll | 40543 | 29670 ns/op | 0 B/op | 0 allocs/op |
BenchmarkAero_GithubAll | 57632 | 20648 ns/op | 0 B/op | 0 allocs/op |
BenchmarkBear_GithubAll | 9234 | 216179 ns/op | 86448 B/op | 943 allocs/op |
BenchmarkBeego_GithubAll | 7407 | 243496 ns/op | 71456 B/op | 609 allocs/op |
BenchmarkBone_GithubAll | 420 | 2922835 ns/op | 720160 B/op | 8620 allocs/op |
BenchmarkChi_GithubAll | 7620 | 238331 ns/op | 87696 B/op | 609 allocs/op |
BenchmarkDenco_GithubAll | 18355 | 64494 ns/op | 20224 B/op | 167 allocs/op |
BenchmarkEcho_GithubAll | 31251 | 38479 ns/op | 0 B/op | 0 allocs/op |
BenchmarkGocraftWeb_GithubAll | 4117 | 300062 ns/op | 131656 B/op | 1686 allocs/op |
BenchmarkGoji_GithubAll | 3274 | 416158 ns/op | 56112 B/op | 334 allocs/op |
BenchmarkGojiv2_GithubAll | 1402 | 870518 ns/op | 352720 B/op | 4321 allocs/op |
BenchmarkGoJsonRest_GithubAll | 2976 | 401507 ns/op | 134371 B/op | 2737 allocs/op |
BenchmarkGoRestful_GithubAll | 410 | 2913158 ns/op | 910144 B/op | 2938 allocs/op |
BenchmarkGorillaMux_GithubAll | 346 | 3384987 ns/op | 251650 B/op | 1994 allocs/op |
BenchmarkGowwwRouter_GithubAll | 10000 | 143025 ns/op | 72144 B/op | 501 allocs/op |
BenchmarkHttpRouter_GithubAll | 55938 | 21360 ns/op | 0 B/op | 0 allocs/op |
BenchmarkHttpTreeMux_GithubAll | 10000 | 153944 ns/op | 65856 B/op | 671 allocs/op |
BenchmarkKocha_GithubAll | 10000 | 106315 ns/op | 23304 B/op | 843 allocs/op |
BenchmarkLARS_GithubAll | 47779 | 25084 ns/op | 0 B/op | 0 allocs/op |
BenchmarkMacaron_GithubAll | 3266 | 371907 ns/op | 149409 B/op | 1624 allocs/op |
BenchmarkMartini_GithubAll | 331 | 3444706 ns/op | 226551 B/op | 2325 allocs/op |
BenchmarkPat_GithubAll | 273 | 4381818 ns/op | 1483152 B/op | 26963 allocs/op |
BenchmarkPossum_GithubAll | 10000 | 164367 ns/op | 84448 B/op | 609 allocs/op |
BenchmarkR2router_GithubAll | 10000 | 160220 ns/op | 77328 B/op | 979 allocs/op |
BenchmarkRivet_GithubAll | 14625 | 82453 ns/op | 16272 B/op | 167 allocs/op |
BenchmarkTango_GithubAll | 6255 | 279611 ns/op | 63826 B/op | 1618 allocs/op |
BenchmarkTigerTonic_GithubAll | 2008 | 687874 ns/op | 193856 B/op | 4474 allocs/op |
BenchmarkTraffic_GithubAll | 355 | 3478508 ns/op | 820744 B/op | 14114 allocs/op |
BenchmarkVulcan_GithubAll | 6885 | 193333 ns/op | 19894 B/op | 609 allocs/op |
- (1): Total Repetitions achieved in constant time, higher means more confident result
- (2): Single Repetition Duration (ns/op), lower is better
- (3): Heap Memory (B/op), lower is better
- (4): Average Allocations per Repetition (allocs/op), lower is better
Middleware
You can find many useful Gin middlewares at gin-contrib.
Uses
Here are some awesome projects that are using the Gin web framework.
- gorush: A push notification server.
- fnproject: A container native, cloud agnostic serverless platform.
- photoprism: Personal photo management powered by Google TensorFlow.
- lura: Ultra performant API Gateway with middleware.
- picfit: An image resizing server.
- dkron: Distributed, fault tolerant job scheduling system.
Contributing
Gin is the work of hundreds of contributors. We appreciate your help!
Please see CONTRIBUTING.md for details on submitting patches and the contribution workflow.
Top Related Projects
High performance, minimalist Go web framework
⚡️ Express inspired web framework written in Go
A high performance HTTP request router that scales well
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
lightweight, idiomatic and composable router for building Go HTTP services
Fast HTTP package for Go. Tuned for high performance. Zero memory allocations in hot paths. Up to 10x faster than net/http
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot