android-advancedrecyclerview
RecyclerView extension library which provides advanced features. (ex. Google's Inbox app like swiping, Play Music app like drag and drop sorting)
Top Related Projects
BRVAH:Powerful and flexible RecyclerAdapter
An Android Animation library which easily add itemanimator to RecyclerView items.
The bullet proof, fast and easy to use adapter library, which minimizes developing time to a fraction...
"Favor composition over inheritance" for RecyclerView Adapters
Flexible multiple types for Android RecyclerView.
Epoxy is an Android library for building complex screens in a RecyclerView
Quick Overview
Android-AdvancedRecyclerView is a library that extends the functionality of Android's RecyclerView widget. It provides advanced features such as swipe-to-dismiss, drag-and-drop, expandable items, and section headers, making it easier for developers to create complex and interactive list layouts in Android applications.
Pros
- Offers a wide range of advanced features not available in the standard RecyclerView
- Highly customizable and flexible for various use cases
- Well-documented with comprehensive examples and demos
- Actively maintained with regular updates and bug fixes
Cons
- Steeper learning curve compared to the standard RecyclerView
- May introduce additional complexity to simpler projects
- Some features might require more setup and configuration
- Performance impact for very large lists with multiple advanced features enabled
Code Examples
- Setting up a basic draggable list:
val draggableManager = RecyclerViewDragDropManager()
val wrappedAdapter = draggableManager.createWrappedAdapter(myAdapter)
recyclerView.adapter = wrappedAdapter
draggableManager.attachRecyclerView(recyclerView)
- Implementing swipe-to-dismiss:
val swipeToDismissManager = RecyclerViewSwipeManager()
val wrappedAdapter = swipeToDismissManager.createWrappedAdapter(myAdapter)
recyclerView.adapter = wrappedAdapter
swipeToDismissManager.attachRecyclerView(recyclerView)
- Creating an expandable list:
val expandableManager = RecyclerViewExpandableItemManager(null)
val wrappedAdapter = expandableManager.createWrappedAdapter(myExpandableAdapter)
recyclerView.adapter = wrappedAdapter
expandableManager.attachRecyclerView(recyclerView)
Getting Started
- Add the dependency to your app's
build.gradle
:
dependencies {
implementation 'com.h6ah4i.android.widget.advrecyclerview:advrecyclerview:1.0.0'
}
- Create a custom adapter extending
AbstractDraggableItemAdapter
:
class MyAdapter : AbstractDraggableItemAdapter<MyViewHolder>() {
// Implement required methods
}
- Set up the RecyclerView with the advanced features:
val dragDropManager = RecyclerViewDragDropManager()
val wrappedAdapter = dragDropManager.createWrappedAdapter(myAdapter)
recyclerView.adapter = wrappedAdapter
dragDropManager.attachRecyclerView(recyclerView)
Competitor Comparisons
BRVAH:Powerful and flexible RecyclerAdapter
Pros of BaseRecyclerViewAdapterHelper
- Simpler API and easier to use for basic RecyclerView implementations
- More comprehensive documentation and examples
- Larger community and more frequent updates
Cons of BaseRecyclerViewAdapterHelper
- Less flexible for complex custom layouts and animations
- Fewer advanced features for drag-and-drop and swipe actions
- May introduce unnecessary overhead for simple use cases
Code Comparison
BaseRecyclerViewAdapterHelper:
class MyAdapter : BaseQuickAdapter<String, BaseViewHolder>(R.layout.item_layout) {
override fun convert(holder: BaseViewHolder, item: String) {
holder.setText(R.id.textView, item)
}
}
android-advancedrecyclerview:
class MyAdapter : AbstractDraggableItemAdapter<MyAdapter.ViewHolder>() {
override fun onCreateItemViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val v = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(v)
}
}
BaseRecyclerViewAdapterHelper offers a more concise implementation for simple use cases, while android-advancedrecyclerview provides more control over item interactions and animations at the cost of additional complexity.
Both libraries have their strengths, and the choice between them depends on the specific requirements of your project. BaseRecyclerViewAdapterHelper is better suited for simpler implementations with basic functionality, while android-advancedrecyclerview excels in scenarios requiring advanced features and customization.
An Android Animation library which easily add itemanimator to RecyclerView items.
Pros of recyclerview-animators
- Focuses specifically on animations, providing a wide range of pre-built animation options
- Lightweight and easy to implement for basic animation needs
- Offers simple customization of animation duration and interpolators
Cons of recyclerview-animators
- Limited functionality beyond animations compared to android-advancedrecyclerview
- Lacks advanced features like drag-and-drop, swipe-to-dismiss, or expandable items
- May require additional libraries or custom code for more complex RecyclerView implementations
Code Comparison
recyclerview-animators:
val animator = SlideInLeftAnimator()
animator.addDuration = 300
recyclerView.itemAnimator = animator
android-advancedrecyclerview:
val dragDropManager = RecyclerViewDragDropManager()
val wrappedAdapter = dragDropManager.createWrappedAdapter(myAdapter)
recyclerView.adapter = wrappedAdapter
dragDropManager.attachRecyclerView(recyclerView)
The code snippets demonstrate the primary focus of each library. recyclerview-animators provides a simple way to add animations, while android-advancedrecyclerview offers more complex functionality like drag-and-drop support.
recyclerview-animators is ideal for projects that require quick and easy implementation of RecyclerView animations. android-advancedrecyclerview is better suited for applications needing a wider range of advanced RecyclerView features beyond just animations.
The bullet proof, fast and easy to use adapter library, which minimizes developing time to a fraction...
Pros of FastAdapter
- More active development and frequent updates
- Extensive documentation and samples
- Built-in support for various item types and animations
Cons of FastAdapter
- Steeper learning curve due to more complex API
- Larger library size, which may impact app size
Code Comparison
FastAdapter:
val fastAdapter = FastAdapter.with(itemAdapter)
recyclerView.adapter = fastAdapter
itemAdapter.add(SimpleItem("Item 1"))
itemAdapter.add(SimpleItem("Item 2"))
AdvancedRecyclerView:
val adapter = MyAdapter()
recyclerView.adapter = adapter
adapter.add(SimpleItem("Item 1"))
adapter.add(SimpleItem("Item 2"))
FastAdapter offers a more flexible approach with its FastAdapter.with()
method, allowing easy integration of multiple adapters. AdvancedRecyclerView uses a more traditional adapter pattern.
FastAdapter provides built-in support for different item types and animations, making it easier to create complex lists. AdvancedRecyclerView requires more manual implementation for advanced features.
Both libraries offer drag-and-drop and swipe-to-dismiss functionality, but FastAdapter's implementation is generally more straightforward to use.
AdvancedRecyclerView excels in providing a lightweight solution with a focus on expandable items and section headers, while FastAdapter offers a more comprehensive set of features for various RecyclerView use cases.
"Favor composition over inheritance" for RecyclerView Adapters
Pros of AdapterDelegates
- Simpler and more lightweight implementation
- Focuses specifically on delegation pattern for RecyclerView adapters
- Easier to understand and implement for developers new to advanced RecyclerView techniques
Cons of AdapterDelegates
- Less feature-rich compared to AdvancedRecyclerView
- Lacks built-in support for advanced features like expandable items or dragging and dropping
Code Comparison
AdapterDelegates:
class CatAdapterDelegate : AdapterDelegate<List<Animal>>() {
override fun isForViewType(items: List<Animal>, position: Int): Boolean {
return items[position] is Cat
}
override fun onCreateViewHolder(parent: ViewGroup): RecyclerView.ViewHolder {
return CatViewHolder(parent.inflate(R.layout.item_cat))
}
}
AdvancedRecyclerView:
class MyExpandableItem : AbstractExpandableItem<MyChildItem>() {
override fun getInitiallyExpandedState(): Boolean = false
override fun getSwipeReactionType(): Int = SwipeableItemConstants.REACTION_CAN_SWIPE_BOTH
override fun isDraggable(): Boolean = true
}
The code snippets demonstrate the different approaches:
- AdapterDelegates focuses on delegating view types to separate classes
- AdvancedRecyclerView provides a more comprehensive set of features, including expandable items and swipe/drag functionality
Both libraries aim to simplify RecyclerView implementation, but AdvancedRecyclerView offers more built-in features at the cost of increased complexity.
Flexible multiple types for Android RecyclerView.
Pros of MultiType
- Simpler API and easier to use for basic multi-type RecyclerView implementations
- Lightweight library with minimal dependencies
- Better documentation and more beginner-friendly
Cons of MultiType
- Fewer advanced features compared to AdvancedRecyclerView
- Limited built-in support for drag-and-drop or swipe-to-dismiss functionality
- Less flexibility for complex RecyclerView layouts and interactions
Code Comparison
MultiType:
class TextItemViewBinder : ItemViewBinder<TextItem, TextItemViewBinder.ViewHolder>() {
override fun onCreateViewHolder(inflater: LayoutInflater, parent: ViewGroup): ViewHolder {
return ViewHolder(inflater.inflate(R.layout.item_text, parent, false))
}
override fun onBindViewHolder(holder: ViewHolder, item: TextItem) {
holder.textView.text = item.text
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val textView: TextView = itemView.findViewById(R.id.text)
}
}
AdvancedRecyclerView:
class TextItemViewHolder(itemView: View) : AbstractDraggableSwipeableItemViewHolder(itemView) {
val textView: TextView = itemView.findViewById(R.id.text)
override fun getSwipeableContainerView(): View = itemView
}
class TextItemAdapter : AbstractDraggableSwipeableItemAdapter<TextItemViewHolder, TextItem>() {
override fun onCreateItemViewHolder(parent: ViewGroup, viewType: Int): TextItemViewHolder {
return TextItemViewHolder(LayoutInflater.from(parent.context).inflate(R.layout.item_text, parent, false))
}
override fun onBindItemViewHolder(holder: TextItemViewHolder, position: Int) {
holder.textView.text = getItem(position).text
}
}
Epoxy is an Android library for building complex screens in a RecyclerView
Pros of Epoxy
- More comprehensive and feature-rich, offering a complete solution for building complex RecyclerViews
- Better support for data binding and view binding
- Stronger type safety with generated models
Cons of Epoxy
- Steeper learning curve due to its more complex architecture
- Requires annotation processing, which can increase build times
- May be overkill for simpler RecyclerView implementations
Code Comparison
Epoxy:
@EpoxyModelClass
abstract class HeaderModel : EpoxyModelWithHolder<HeaderHolder>() {
@EpoxyAttribute lateinit var title: String
override fun bind(holder: HeaderHolder) {
holder.titleView.text = title
}
}
AdvancedRecyclerView:
class HeaderViewHolder(view: View) : RecyclerView.ViewHolder(view) {
val titleView: TextView = view.findViewById(R.id.title)
}
override fun onBindViewHolder(holder: HeaderViewHolder, position: Int) {
holder.titleView.text = items[position].title
}
Epoxy provides a more declarative approach with generated models, while AdvancedRecyclerView follows a more traditional ViewHolder pattern. Epoxy's approach can lead to cleaner, more maintainable code for complex layouts, but may require more setup for simpler use cases.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Advanced RecyclerView
This RecyclerView extension library provides Google's Inbox app like swiping, Play Music app like drag-and-drop sorting and expandable item features. Works on API level 14 or later.
Documentation site: https://advancedrecyclerview.h6ah4i.com/
Download the example app

Demonstration video on YouTube
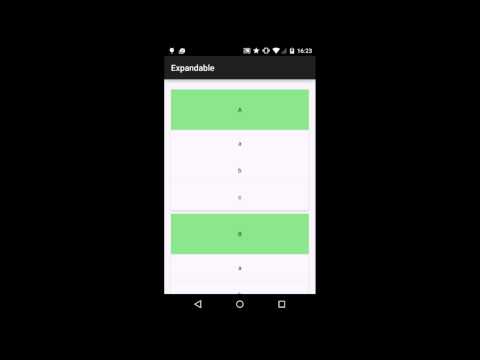
Target platforms
- API level 14 or later
Latest version
- Version 1.0.0 (December 16, 2018) (RELEASE NOTES)
Recent Breaking Change Info
- v1.0.0:
- Migrated to AndroidX (Use v0.11.0 instead if your project uses support libraries)
BaseWrapperAdapter
- Removed some deprecated features
SwipeableItemConstants.REACTION_CAN_SWIPE_BOTH
SwipeableItemConstants.REACTION_CAN_NOT_SWIPE_BOTH
SwipeableItemConstants.REACTION_CAN_NOT_SWIPE_BOTH_WITH_RUBBER_BAND_EFFECT
- New
DraggableItemState getDragState()
method added to theDraggableItemViewHolder
interface - New
SwipeableItemState getSwipeState()
method added to theSwipeableItemViewHolder
interface - New
ExpandableItemState getExpandState()
method added to theExpandableItemViewHolder
interface
:point_right: Migration guide from v0.11.0 to v1.0.0
Getting started
This library is published on Maven Central. Just add these lines to build.gradle
.
repositories {
+ mavenCentral()
}
dependencies {
+ implementation 'com.h6ah4i.android.widget.advrecyclerview:advrecyclerview:1.0.0'
}
Please check the Getting Started section on the official documentation site for more details.
Examples
Please check the implementation of the simple examples.
Drag & Drop related examples
- Basic
- Minimal
- Draggable grid
- Draggable staggered grid
- Draggable with section
- Drag on Long press
- Uses onCheckCanDrop()
Expandable item related examples
Swipeable related examples
Headers and Footers examples
WrapperAdapter examples
Hybrid examples
Other examples
iOS Mail app like swipe action

License
This library is licensed under the Apache Software License, Version 2.0.
See LICENSE
for full of the license text.
Copyright (C) 2015 Haruki Hasegawa
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Top Related Projects
BRVAH:Powerful and flexible RecyclerAdapter
An Android Animation library which easily add itemanimator to RecyclerView items.
The bullet proof, fast and easy to use adapter library, which minimizes developing time to a fraction...
"Favor composition over inheritance" for RecyclerView Adapters
Flexible multiple types for Android RecyclerView.
Epoxy is an Android library for building complex screens in a RecyclerView
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot