Top Related Projects
Fast, unopinionated, minimalist web framework for node.
Expressive middleware for node.js using ES2017 async functions
Fast and low overhead web framework, for Node.js
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
The API and real-time application framework
Quick Overview
Hapi (HTTP API) is a powerful and feature-rich Node.js web framework for building scalable and secure applications. It provides a robust plugin system, built-in caching, input validation, and authentication, making it an excellent choice for large-scale enterprise applications.
Pros
- Extensive plugin ecosystem for easy extensibility
- Built-in support for input validation and authentication
- Excellent performance and scalability
- Comprehensive documentation and active community support
Cons
- Steeper learning curve compared to simpler frameworks like Express
- Can be overkill for small, simple applications
- Less flexibility in routing compared to some other frameworks
- Slightly more verbose configuration compared to minimalist frameworks
Code Examples
- Creating a basic Hapi server:
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: 'localhost'
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
init();
- Adding a route with input validation:
server.route({
method: 'GET',
path: '/hello/{name}',
handler: (request, h) => {
return `Hello ${request.params.name}!`;
},
options: {
validate: {
params: Joi.object({
name: Joi.string().min(3).max(30).required()
})
}
}
});
- Using a plugin:
const plugin = {
name: 'myPlugin',
register: async function (server, options) {
server.route({
method: 'GET',
path: '/plugin',
handler: (request, h) => {
return 'Plugin route!';
}
});
}
};
await server.register(plugin);
Getting Started
To get started with Hapi, follow these steps:
-
Install Hapi:
npm install @hapi/hapi
-
Create a new file (e.g.,
server.js
) and add the following code:const Hapi = require('@hapi/hapi'); const init = async () => { const server = Hapi.server({ port: 3000, host: 'localhost' }); server.route({ method: 'GET', path: '/', handler: (request, h) => { return 'Hello World!'; } }); await server.start(); console.log('Server running on %s', server.info.uri); }; init();
-
Run the server:
node server.js
-
Open your browser and navigate to
http://localhost:3000
to see the "Hello World!" message.
Competitor Comparisons
Fast, unopinionated, minimalist web framework for node.
Pros of Express
- Lightweight and minimalist, allowing for greater flexibility and customization
- Extensive ecosystem with a wide range of middleware and plugins
- Simpler learning curve, making it easier for beginners to get started
Cons of Express
- Less opinionated, requiring more setup and configuration for complex applications
- Lacks built-in features for input validation and authentication, often requiring additional libraries
Code Comparison
Express:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => console.log('Server running on port 3000'));
Hapi:
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({ port: 3000 });
server.route({
method: 'GET',
path: '/',
handler: (request, h) => 'Hello World!'
});
await server.start();
console.log('Server running on port 3000');
};
init();
Key Differences
- Express uses middleware for request processing, while Hapi uses plugins
- Hapi provides built-in input validation and authentication features
- Express offers more flexibility in routing, while Hapi has a more structured approach
- Hapi focuses on configuration over code, whereas Express is more code-centric
Both frameworks are popular choices for Node.js applications, with Express being more widely adopted due to its simplicity and flexibility, while Hapi offers more built-in features and a more opinionated structure.
Expressive middleware for node.js using ES2017 async functions
Pros of Koa
- Lightweight and minimalist, allowing for greater flexibility and customization
- Uses modern JavaScript features like async/await for cleaner, more readable code
- Smaller learning curve due to its simplicity and straightforward API
Cons of Koa
- Requires additional middleware for many common functionalities that Hapi includes out-of-the-box
- Less opinionated, which can lead to inconsistencies in large projects or teams
- Smaller ecosystem and community compared to Hapi
Code Comparison
Koa:
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = 'Hello World';
});
app.listen(3000);
Hapi:
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({ port: 3000 });
server.route({
method: 'GET',
path: '/',
handler: (request, h) => 'Hello World'
});
await server.start();
};
init();
Both Koa and Hapi are popular Node.js frameworks, but they cater to different needs. Koa is more suitable for developers who prefer a minimalist approach and want to build their stack from the ground up. Hapi, on the other hand, provides a more comprehensive solution out-of-the-box, making it ideal for larger projects that require a structured and opinionated framework.
Fast and low overhead web framework, for Node.js
Pros of Fastify
- Significantly faster performance, especially for JSON serialization
- Lightweight and minimal core with a plugin system for extensibility
- Built-in support for schema validation and serialization
Cons of Fastify
- Smaller ecosystem and community compared to Hapi
- Less mature and battle-tested in large-scale production environments
- Steeper learning curve for developers coming from Express-like frameworks
Code Comparison
Hapi:
const Hapi = require('@hapi/hapi');
const server = Hapi.server({ port: 3000 });
server.route({
method: 'GET',
path: '/',
handler: (request, h) => 'Hello World!'
});
await server.start();
Fastify:
const fastify = require('fastify')({ logger: true });
fastify.get('/', async (request, reply) => {
return 'Hello World!'
});
await fastify.listen(3000);
Both Hapi and Fastify are popular Node.js web frameworks, but they have different design philosophies and strengths. Hapi focuses on configuration-driven development and robustness, while Fastify prioritizes performance and developer experience. The choice between them depends on project requirements, team expertise, and specific use cases.
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
Pros of Nest
- Built-in support for TypeScript, offering strong typing and better tooling
- Modular architecture with dependency injection, promoting cleaner and more maintainable code
- Extensive ecosystem with built-in support for various features like GraphQL, WebSockets, and microservices
Cons of Nest
- Steeper learning curve due to its opinionated structure and TypeScript focus
- Potentially higher overhead for simple applications compared to Hapi's lightweight approach
- Less flexibility in terms of customization compared to Hapi's plugin-based architecture
Code Comparison
Nest:
@Controller('cats')
export class CatsController {
@Get()
findAll(): string {
return 'This action returns all cats';
}
}
Hapi:
server.route({
method: 'GET',
path: '/cats',
handler: (request, h) => {
return 'This action returns all cats';
}
});
The code comparison shows Nest's decorator-based approach for defining routes and controllers, while Hapi uses a more traditional route configuration object. Nest's approach is more concise and leverages TypeScript features, while Hapi's method is more explicit and JavaScript-friendly.
The API and real-time application framework
Pros of Feathers
- Real-time capabilities out of the box with WebSocket support
- Modular architecture with a plugin system for easy extensibility
- Built-in support for multiple databases and ORMs
Cons of Feathers
- Steeper learning curve for developers new to its concepts
- Less mature ecosystem compared to Hapi
- Smaller community and fewer third-party plugins
Code Comparison
Feathers:
const feathers = require('@feathersjs/feathers');
const app = feathers();
app.use('/users', {
async find() {
return [{ id: 1, name: 'John' }];
}
});
app.service('users').find().then(console.log);
Hapi:
const Hapi = require('@hapi/hapi');
const server = Hapi.server({ port: 3000 });
server.route({
method: 'GET',
path: '/users',
handler: () => [{ id: 1, name: 'John' }]
});
server.start();
Both frameworks offer different approaches to building APIs. Feathers focuses on real-time capabilities and database integration, while Hapi provides a more traditional HTTP server setup with a robust plugin system. The choice between them depends on project requirements and developer preferences.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
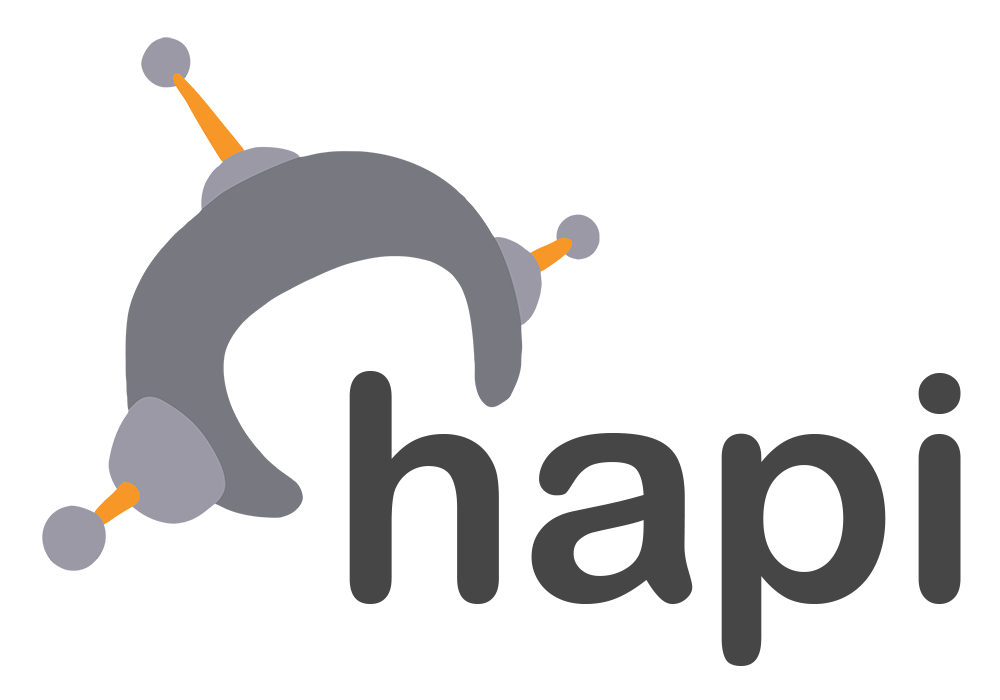
@hapi/hapi
The Simple, Secure Framework Developers Trust
Build powerful, scalable applications, with minimal overhead and full out-of-the-box functionality - your code, your way.
Visit the hapi.dev Developer Portal for tutorials, documentation, and support
Useful resources
- Documentation and API
- Version status (builds, dependencies, node versions, licenses, eol)
- Changelog
- Project policies
- Support
Technical Steering Committee (TSC) Members
- Devin Ivy (@devinivy)
- Lloyd Benson (@lloydbenson)
- Nathan LaFreniere (@nlf)
- Wyatt Lyon Preul (@geek)
- Nicolas Morel (@marsup)
- Jonathan Samines (@jonathansamines)
Top Related Projects
Fast, unopinionated, minimalist web framework for node.
Expressive middleware for node.js using ES2017 async functions
Fast and low overhead web framework, for Node.js
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
The API and real-time application framework
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot