SwiftUI
Examples projects using SwiftUI released by WWDC2019. Include Layout, UI, Animations, Gestures, Draw and Data.
Top Related Projects
An iOS library to natively render After Effects vector animations
Elegant HTTP Networking in Swift
Beautiful charts for iOS/tvOS/OSX! The Apple side of the crossplatform MPAndroidChart.
Reactive Programming in Swift
A Swift Autolayout DSL for iOS & OS X
The better way to deal with JSON data in Swift.
Quick Overview
SwiftUI is a comprehensive collection of SwiftUI components and extensions, designed to enhance and simplify iOS app development. It provides a wide range of ready-to-use UI elements, custom views, and utility functions that complement Apple's SwiftUI framework, allowing developers to create rich and interactive user interfaces more efficiently.
Pros
- Extensive library of pre-built components and extensions
- Regularly updated to support the latest SwiftUI features and iOS versions
- Well-documented with clear examples and usage instructions
- Customizable components that can be easily integrated into existing projects
Cons
- May increase app size due to the inclusion of additional resources
- Some components might not perfectly match specific design requirements
- Learning curve for developers new to the library's structure and conventions
- Potential dependency on third-party code for core UI functionality
Code Examples
- Creating a custom button with a gradient background:
import SwiftUI
import SPAlert
struct ContentView: View {
var body: some View {
Button(action: {
SPAlert.present(title: "Hello!", message: "This is a custom alert.", preset: .done)
}) {
Text("Show Alert")
.padding()
.background(LinearGradient(gradient: Gradient(colors: [.blue, .purple]), startPoint: .leading, endPoint: .trailing))
.foregroundColor(.white)
.cornerRadius(10)
}
}
}
- Implementing a custom progress bar:
import SwiftUI
import SPIndicator
struct ContentView: View {
@State private var progress: CGFloat = 0.0
var body: some View {
VStack {
SPIndicator(title: "Downloading...", message: "Please wait", preset: .custom(UIImage(systemName: "arrow.down.circle")!))
ProgressView(value: progress)
.padding()
Button("Increment Progress") {
withAnimation {
progress += 0.1
if progress >= 1.0 {
SPIndicator.present(title: "Download Complete!", preset: .done)
}
}
}
}
}
}
- Creating a custom tab bar:
import SwiftUI
import SPPerspective
struct ContentView: View {
var body: some View {
TabView {
Text("Home")
.tabItem {
Image(systemName: "house")
Text("Home")
}
Text("Search")
.tabItem {
Image(systemName: "magnifyingglass")
Text("Search")
}
}
.modifier(SPPerspectiveModifier())
}
}
Getting Started
To use SwiftUI in your project, follow these steps:
-
Add the SwiftUI package to your Xcode project:
- File > Swift Packages > Add Package Dependency
- Enter the repository URL: https://github.com/ivanvorobei/SwiftUI.git
-
Import the required modules in your Swift file:
import SwiftUI
import SPAlert
import SPIndicator
import SPPerspective
- Start using the components in your SwiftUI views:
struct ContentView: View {
var body: some View {
Button("Show Alert") {
SPAlert.present(title: "Hello!", preset: .done)
}
}
}
Competitor Comparisons
An iOS library to natively render After Effects vector animations
Pros of Lottie-iOS
- Specialized for animations: Lottie-iOS is designed specifically for rendering After Effects animations, providing a powerful and efficient solution for complex animations
- Cross-platform compatibility: Supports iOS, Android, and web platforms, allowing for consistent animations across different environments
- Large community and extensive documentation: Being an Airbnb project, it has a robust community and comprehensive resources for developers
Cons of Lottie-iOS
- Limited to animation: Unlike SwiftUI, which is a general-purpose UI framework, Lottie-iOS focuses solely on animations
- Learning curve: Requires knowledge of After Effects for creating animations, which may be challenging for developers without design experience
- File size: Lottie animations can increase app size, especially for complex animations
Code Comparison
SwiftUI (Creating a simple animation):
Circle()
.fill(Color.blue)
.frame(width: 100, height: 100)
.scaleEffect(isAnimating ? 1.5 : 1.0)
.animation(.easeInOut(duration: 1.0))
Lottie-iOS (Loading and playing an animation):
let animationView = AnimationView(name: "animation")
animationView.frame = view.bounds
animationView.contentMode = .scaleAspectFit
view.addSubview(animationView)
animationView.play()
Elegant HTTP Networking in Swift
Pros of Alamofire
- More mature and widely adopted networking library for iOS
- Extensive documentation and community support
- Offers advanced features like request/response serialization and authentication
Cons of Alamofire
- Focused solely on networking, while SwiftUI is a comprehensive UI framework
- Steeper learning curve for beginners compared to SwiftUI's declarative syntax
- May require additional setup and configuration for basic networking tasks
Code Comparison
Alamofire (HTTP request):
AF.request("https://api.example.com/data").responseJSON { response in
switch response.result {
case .success(let value):
print("Success: \(value)")
case .failure(let error):
print("Error: \(error)")
}
}
SwiftUI (UI component):
struct ContentView: View {
var body: some View {
Text("Hello, World!")
.padding()
.background(Color.blue)
.foregroundColor(.white)
}
}
While both repositories serve different purposes, Alamofire excels in networking tasks, offering robust features and extensive community support. SwiftUI, on the other hand, provides a modern, declarative approach to building user interfaces for Apple platforms. The choice between the two depends on the specific needs of your project, whether it's focused on networking or UI development.
Beautiful charts for iOS/tvOS/OSX! The Apple side of the crossplatform MPAndroidChart.
Pros of Charts
- Specialized for creating various types of charts and graphs
- Extensive documentation and examples for different chart types
- Highly customizable with a wide range of styling options
Cons of Charts
- Limited to chart-specific functionality, not a general-purpose UI framework
- Steeper learning curve for complex chart customizations
- May require additional setup for integration with SwiftUI
Code Comparison
SwiftUI example:
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, SwiftUI!")
Button("Tap me") {
print("Button tapped")
}
}
}
}
Charts example:
let chart = LineChartView()
let dataSet = LineChartDataSet(entries: [
ChartDataEntry(x: 1, y: 10),
ChartDataEntry(x: 2, y: 15),
ChartDataEntry(x: 3, y: 12)
])
chart.data = LineChartData(dataSet: dataSet)
SwiftUI focuses on general UI components and layout, while Charts specializes in creating data visualizations. SwiftUI offers a more declarative syntax for building user interfaces, whereas Charts provides specific methods and classes for constructing various chart types. The choice between the two depends on the project's requirements and whether the primary focus is on general UI development or data visualization.
Reactive Programming in Swift
Pros of RxSwift
- More mature and established framework with a larger community
- Provides powerful tools for handling asynchronous programming and event-based systems
- Offers a wide range of operators for complex data transformations
Cons of RxSwift
- Steeper learning curve, especially for developers new to reactive programming
- Can lead to increased code complexity if not used judiciously
- Requires additional setup and integration compared to SwiftUI's built-in features
Code Comparison
RxSwift:
Observable.from([1, 2, 3, 4, 5])
.filter { $0 % 2 == 0 }
.map { $0 * 2 }
.subscribe(onNext: { print($0) })
SwiftUI:
List([1, 2, 3, 4, 5]) { number in
if number % 2 == 0 {
Text("\(number * 2)")
}
}
While RxSwift excels in handling complex asynchronous operations and data streams, SwiftUI provides a more straightforward approach for building user interfaces and managing simple state changes. RxSwift offers greater flexibility for advanced scenarios, but SwiftUI's declarative syntax makes it easier to create and maintain UI components. The choice between the two depends on the specific requirements of your project and your team's expertise.
A Swift Autolayout DSL for iOS & OS X
Pros of SnapKit
- Mature and well-established library with a large community and extensive documentation
- Supports both UIKit and AppKit, making it versatile for iOS and macOS development
- Offers a concise and readable syntax for creating complex layouts programmatically
Cons of SnapKit
- Limited to Auto Layout constraints, while SwiftUI offers a more declarative approach
- Requires more code to achieve the same layouts compared to SwiftUI's concise syntax
- Steeper learning curve for developers new to programmatic UI development
Code Comparison
SnapKit:
view.addSubview(label)
label.snp.makeConstraints { make in
make.center.equalToSuperview()
make.width.equalTo(200)
}
SwiftUI:
VStack {
Text("Hello, World!")
.frame(width: 200)
}
.frame(maxWidth: .infinity, maxHeight: .infinity)
SnapKit focuses on creating constraints programmatically, while SwiftUI uses a more declarative approach to define layouts. SwiftUI generally requires less code and offers a more intuitive syntax for UI development, especially for those familiar with modern declarative UI frameworks. However, SnapKit provides greater flexibility and control over complex layouts, particularly when working with existing UIKit or AppKit projects.
The better way to deal with JSON data in Swift.
Pros of SwiftyJSON
- Focused specifically on JSON parsing and manipulation
- Lightweight and easy to integrate into existing projects
- Extensive documentation and community support
Cons of SwiftyJSON
- Limited to JSON-related functionality
- May require additional libraries for UI components
- Less frequent updates compared to SwiftUI
Code Comparison
SwiftyJSON:
let json = JSON(data: dataFromNetworking)
if let name = json["name"].string {
// Do something with name
}
SwiftUI:
struct ContentView: View {
var body: some View {
Text("Hello, World!")
}
}
Summary
SwiftyJSON is a specialized library for JSON handling in Swift, offering a simple and intuitive way to work with JSON data. It's ideal for projects that require extensive JSON manipulation.
SwiftUI, on the other hand, is a comprehensive framework for building user interfaces across Apple platforms. It provides a declarative way to design and implement UIs, making it more suitable for full-scale app development.
While SwiftyJSON excels in JSON parsing, SwiftUI offers a broader range of features for creating modern, responsive user interfaces. The choice between the two depends on the specific needs of your project – JSON handling vs. UI development.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
SwiftUI
Examples

About
Examples projects using SwiftUI
& Combine
.
Include Layout, UI, Animations, Gestures, Draw and Data.
See projects files in Files
& Other Projects
folders. If you have project, make a pull request or create issue with link to repo.
Interested in UI and animations in UIKit
?
See awesome-ios-ui pack with UI elements.
If you like the project, don't forget to put star â
and follow me on GitHub:
Community
Navigate
Other projects
- Transition And Blur
- 2048 Game
- SFSymbols
- Calculator
- Creating And Combining Views
- Building Lists And Navigation
- Handling User Input
- WWDCPlayer
- Composing Complex Interfaces
- Working With UIControls
- Example To-Do App
- iPadOS Scenes
- Combine using GitHub API
- Async image loading
- Interfacing With UIKit
- GitHub Search
- Time Travel
- Drawing Paths And Shapes
- Animating Views And Transitions
- Jike
- Flux
- PureGenius
- SwiftUI Download Progress View
- SwiftUI SideMenu
- SwiftUI Currency App
- SwiftUI Weather App
- DesignCode SwiftUI App
- SwiftUI SlideToOpen
- Currency Converter & Calculator
- FlipClock-SwiftUI
- Countdown Film Clutter
- SpotlightSearch
- Growing text view in SwiftUI
- Calculator Clone for iPadOS
- MGFlipView
Also include:
- Movie
- InstaFake
- TempusRomanumII
- SwiftUI + Redux
- React Meets SwiftUI
- Webview
- UINote
- SplitView
- Card Animation
Animatable Cards

3D
For add 3D rotation for back cards use code:
.rotation3DEffect(
Angle(degrees: dragState.isActive ? 0 : 60), axis: (x: 10.0, y: 10.0, z: 10.0)
)
For medium card use 30 angles.
Animation
In preview I am use Spring
animation for all cards:
.animation(.spring())
Area to Card

SFSymbols
For button using SFSymbols
pack with ready-use icons. Also support customisable weight:
Image(systemName: show ? "slash.circle.fill" : "slash.circle")
.font(Font.title.weight(.semibold))
Button
For change state using @State
as property:
@State var show = false
Transition And Blur

2048 Game

SFSymbols

Calculator

Creating And Combining Views

Building Lists And Navigation

WWDCPlayer

Handling User Input

Composing Complex Interfaces

Working With UIControls


Example To-Do App

iPadOS Scenes

Combine using GitHub API

Async image loading

Interfacing With UIKit

GitHub Search

Time Travel

Drawing Paths And Shapes

Animating Views And Transitions

Jike

Flux

PureGenius

SwiftUIDownloadView

SwiftUI SideMenu

SwiftUI Currency

SwiftUI Weather

DesignCode SwiftUI
MTSlideToOpen-SwiftUI
Currency Converter & Calculator
FlipClock-SwiftUI
Light | Dark |
---|---|
![]() | ![]() |
CountdownFilmClutter-SwiftUI

SpotlightSearch
Light | Dark |
---|---|
![]() | ![]() |
SwiftUI Weather App with MVVM and CoreML
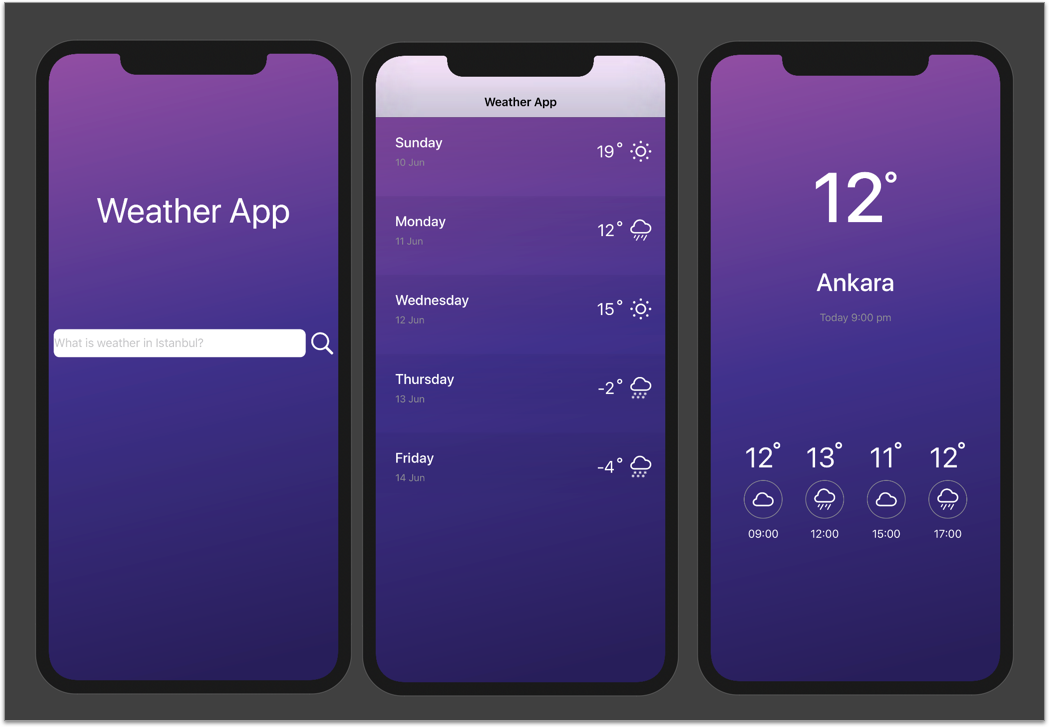
Growing text view in SwiftUI

MGFlipView

Authors
Thanks for Jinxiansen, ra1028, timdonnelly, TwoLivesLeft, devxoul, cmtrounce, unixzii, ra1028 for examples project.
Other Projects
I love being helpful. Here I have provided a list of libraries that I keep up to date. For see video previews
of libraries without install open opensource.ivanvorobei.by website.
I have libraries with native interface and managing permissions. Also available pack of useful extensions for boost your development process.
Russian Community
ÐодпиÑÑвайÑÑ Ð² ÑелегÑамм-канал, еÑли Ñ
оÑеÑÑ Ð¿Ð¾Ð»ÑÑаÑÑ ÑÐ²ÐµÐ´Ð¾Ð¼Ð»ÐµÐ½Ð¸Ñ Ð¾ новÑÑ
ÑÑÑоÑиалаÑ
.
Со ÑложнÑми и непонÑÑнÑми задаÑами помогÑÑ Ð² ÑаÑе.
Ðидео-ÑÑÑоÑÐ¸Ð°Ð»Ñ Ð²ÑклÑдÑÐ²Ð°Ñ Ð½Ð° YouTube:
Top Related Projects
An iOS library to natively render After Effects vector animations
Elegant HTTP Networking in Swift
Beautiful charts for iOS/tvOS/OSX! The Apple side of the crossplatform MPAndroidChart.
Reactive Programming in Swift
A Swift Autolayout DSL for iOS & OS X
The better way to deal with JSON data in Swift.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot