ReactiveUI
An advanced, composable, functional reactive model-view-viewmodel framework for all .NET platforms that is inspired by functional reactive programming. ReactiveUI allows you to abstract mutable state away from your user interfaces, express the idea around a feature in one readable place and improve the testability of your application.
Top Related Projects
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
The Reactive Extensions for .NET
Reactive Programming in Swift
RxJava – Reactive Extensions for the JVM – a library for composing asynchronous and event-based programs using observable sequences for the Java VM.
A reactive programming library for JavaScript
The Reactive Extensions for .NET
Quick Overview
ReactiveUI is a composable, cross-platform model-view-viewmodel framework for reactive programming in .NET. It integrates with the Reactive Extensions (Rx) to create elegant, testable User Interfaces that run on any mobile or desktop platform.
Pros
- Promotes a reactive and functional programming style, leading to more maintainable code
- Cross-platform support for various .NET platforms (WPF, Xamarin, UWP, etc.)
- Strong integration with Reactive Extensions (Rx) for powerful asynchronous and event-based programming
- Extensive documentation and active community support
Cons
- Steep learning curve for developers new to reactive programming concepts
- Can be overkill for simple applications or small projects
- Requires a shift in thinking from traditional imperative programming paradigms
- Performance overhead in some scenarios due to the reactive nature of the framework
Code Examples
- Creating a reactive property:
public class MyViewModel : ReactiveObject
{
private string _myProperty;
public string MyProperty
{
get => _myProperty;
set => this.RaiseAndSetIfChanged(ref _myProperty, value);
}
}
- Binding a command to a view:
public class MyViewModel : ReactiveObject
{
public ReactiveCommand<Unit, Unit> MyCommand { get; }
public MyViewModel()
{
MyCommand = ReactiveCommand.Create(() => Console.WriteLine("Command executed!"));
}
}
- Using WhenAnyValue for property changes:
public class MyViewModel : ReactiveObject
{
private string _firstName;
public string FirstName
{
get => _firstName;
set => this.RaiseAndSetIfChanged(ref _firstName, value);
}
public MyViewModel()
{
this.WhenAnyValue(x => x.FirstName)
.Subscribe(name => Console.WriteLine($"First name changed to: {name}"));
}
}
Getting Started
To get started with ReactiveUI, follow these steps:
-
Install the ReactiveUI NuGet package in your project:
dotnet add package ReactiveUI
-
Create a ViewModel that inherits from
ReactiveObject
:public class MainViewModel : ReactiveObject { private string _greeting; public string Greeting { get => _greeting; set => this.RaiseAndSetIfChanged(ref _greeting, value); } public ReactiveCommand<Unit, Unit> UpdateGreetingCommand { get; } public MainViewModel() { UpdateGreetingCommand = ReactiveCommand.Create(() => Greeting = "Hello, ReactiveUI!"); } }
-
Bind your ViewModel to your View using the appropriate platform-specific bindings.
Competitor Comparisons
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
Pros of ReactiveCocoa
- Native support for Swift and Objective-C, optimized for iOS and macOS development
- Extensive documentation and a large, active community
- Seamless integration with Apple's frameworks and UIKit
Cons of ReactiveCocoa
- Limited cross-platform support compared to ReactiveUI
- Steeper learning curve for developers new to reactive programming
- Less flexibility in terms of supported programming languages
Code Comparison
ReactiveUI example:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.ObserveOn(RxApp.MainThreadScheduler)
.InvokeCommand(SearchCommand);
ReactiveCocoa example:
searchTextField.reactive.continuousTextValues
.throttle(0.8, on: QueueScheduler.main)
.flatMap(.latest) { text in
return self.performSearch(text)
}
.observe(on: UIScheduler())
.observe(next: { results in
self.updateSearchResults(results)
})
Both frameworks provide similar functionality for reactive programming, but ReactiveUI offers broader cross-platform support and language flexibility, while ReactiveCocoa is more focused on Apple's ecosystem with native Swift and Objective-C support. ReactiveUI may be preferred for cross-platform projects, while ReactiveCocoa is often the choice for iOS-specific development.
The Reactive Extensions for .NET
Pros of Reactive
- More focused on core Rx functionality, providing a lightweight and flexible foundation
- Broader platform support, including .NET Framework, .NET Core, and .NET Standard
- Maintained directly by Microsoft, ensuring long-term support and alignment with .NET ecosystem
Cons of Reactive
- Less opinionated, requiring more setup and configuration for specific use cases
- Fewer high-level abstractions and helper methods compared to ReactiveUI
- Steeper learning curve for developers new to reactive programming concepts
Code Comparison
ReactiveUI:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.SelectMany(term => SearchService.Search(term))
.ObserveOn(RxApp.MainThreadScheduler)
.ToProperty(this, x => x.SearchResults, out _searchResults);
Reactive:
Observable.FromEventPattern<TextChangedEventArgs>(searchBox, "TextChanged")
.Select(e => ((TextBox)e.Sender).Text)
.Throttle(TimeSpan.FromSeconds(0.8))
.SelectMany(term => Observable.FromAsync(() => SearchService.Search(term)))
.ObserveOn(SynchronizationContext.Current)
.Subscribe(results => SearchResults = results);
Reactive Programming in Swift
Pros of RxSwift
- Specifically designed for Swift, offering better integration with iOS and macOS development
- More extensive documentation and community resources for Swift developers
- Provides a more idiomatic Swift API, making it easier for Swift developers to adopt
Cons of RxSwift
- Limited to Swift and Apple platforms, whereas ReactiveUI supports multiple .NET languages and platforms
- Steeper learning curve for developers new to reactive programming concepts
- Smaller ecosystem compared to ReactiveUI's integration with the broader .NET community
Code Comparison
ReactiveUI example:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.ObserveOn(RxApp.MainThreadScheduler)
.InvokeCommand(SearchCommand);
RxSwift example:
searchBar.rx.text
.throttle(.milliseconds(800), scheduler: MainScheduler.instance)
.distinctUntilChanged()
.flatMapLatest { query in
self.performSearch(query)
}
.subscribe(onNext: { results in
self.updateUI(with: results)
})
.disposed(by: disposeBag)
Both examples demonstrate reactive programming concepts, but RxSwift's syntax is more tailored to Swift's language features and conventions, while ReactiveUI leverages .NET's language-agnostic approach.
RxJava – Reactive Extensions for the JVM – a library for composing asynchronous and event-based programs using observable sequences for the Java VM.
Pros of RxJava
- Broader language support: RxJava can be used with Java, Kotlin, and Android, offering more flexibility
- More extensive ecosystem: Larger community and more third-party libraries available
- Better performance: Generally faster and more efficient for large-scale applications
Cons of RxJava
- Steeper learning curve: More complex API and concepts compared to ReactiveUI
- Less integrated with UI frameworks: Requires additional setup for UI binding in Android
Code Comparison
ReactiveUI:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.ObserveOn(RxApp.MainThreadScheduler)
.InvokeCommand(SearchCommand);
RxJava:
Observable.fromCallable(() -> searchTerm)
.debounce(800, TimeUnit.MILLISECONDS)
.observeOn(AndroidSchedulers.mainThread())
.subscribe(term -> performSearch(term));
Both libraries provide similar functionality for reactive programming, but ReactiveUI is more focused on .NET and WPF applications, while RxJava offers broader language support and is more commonly used in Android development. ReactiveUI provides tighter integration with UI frameworks, making it easier to bind observables to UI elements. RxJava, on the other hand, offers better performance and a more extensive ecosystem, but with a steeper learning curve.
A reactive programming library for JavaScript
Pros of RxJS
- Widely adopted in the JavaScript ecosystem
- Extensive documentation and community support
- Supports a broader range of reactive programming concepts
Cons of RxJS
- Steeper learning curve for developers new to reactive programming
- Can lead to complex and hard-to-debug code if not used carefully
Code Comparison
ReactiveUI:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.Where(x => !string.IsNullOrWhiteSpace(x))
.SelectMany(x => this.SearchService.Search(x))
.ObserveOn(RxApp.MainThreadScheduler)
.ToProperty(this, x => x.SearchResults, out _searchResults);
RxJS:
this.searchTerm$.pipe(
debounceTime(800),
filter(term => term.length > 0),
switchMap(term => this.searchService.search(term)),
)
.subscribe(results => this.searchResults = results);
Both libraries provide similar functionality for reactive programming, but ReactiveUI is specifically designed for .NET applications, while RxJS is more versatile and can be used in various JavaScript environments. ReactiveUI offers tighter integration with .NET frameworks, while RxJS provides a more flexible approach for web and Node.js applications.
The Reactive Extensions for .NET
Pros of Reactive
- More focused on core Rx functionality, providing a lightweight and flexible foundation
- Broader platform support, including .NET Framework, .NET Core, and .NET Standard
- Maintained directly by Microsoft, ensuring long-term support and alignment with .NET ecosystem
Cons of Reactive
- Less opinionated, requiring more setup and configuration for specific use cases
- Fewer high-level abstractions and helper methods compared to ReactiveUI
- Steeper learning curve for developers new to reactive programming concepts
Code Comparison
ReactiveUI:
this.WhenAnyValue(x => x.SearchTerm)
.Throttle(TimeSpan.FromSeconds(0.8))
.SelectMany(term => SearchService.Search(term))
.ObserveOn(RxApp.MainThreadScheduler)
.ToProperty(this, x => x.SearchResults, out _searchResults);
Reactive:
Observable.FromEventPattern<TextChangedEventArgs>(searchBox, "TextChanged")
.Select(e => ((TextBox)e.Sender).Text)
.Throttle(TimeSpan.FromSeconds(0.8))
.SelectMany(term => Observable.FromAsync(() => SearchService.Search(term)))
.ObserveOn(SynchronizationContext.Current)
.Subscribe(results => SearchResults = results);
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
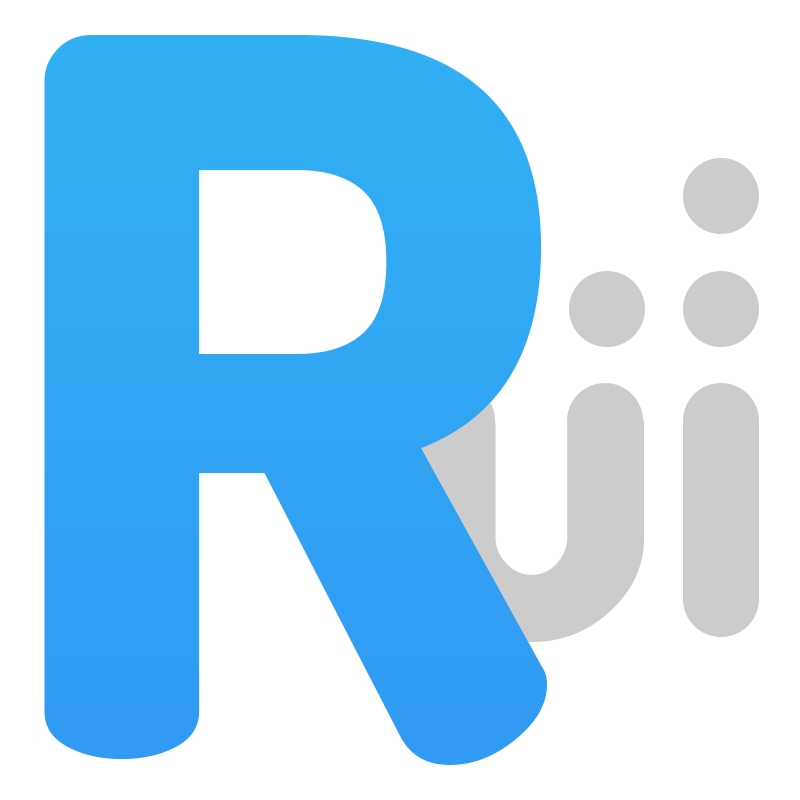
What is ReactiveUI?
ReactiveUI is a composable, cross-platform model-view-viewmodel framework for all .NET platforms that is inspired by functional reactive programming, which is a paradigm that allows you to abstract mutable state away from your user interfaces and express the idea around a feature in one readable place and improve the testability of your application.
ð¨ Get Started ð Install Packages ð Watch Videos ð View Samples ð¤ Discuss ReactiveUI
Book
There has been an excellent book written by our Alumni maintainer Kent Boogart.
NuGet Packages
Install the following packages to start building your own ReactiveUI app. Note: some of the platform-specific packages are required. This means your app won't perform as expected until you install the packages properly. See the Installation docs page for more info.
Platform | ReactiveUI Package | NuGet |
---|---|---|
.NET Standard | ReactiveUI | |
ReactiveUI.Fody | ||
Unit Testing | ReactiveUI.Testing | |
WPF | ReactiveUI.WPF | |
UWP | ReactiveUI.Uwp | |
WinUI | ReactiveUI.WinUI | |
MAUI | ReactiveUI.Maui | |
Windows Forms | ReactiveUI.WinForms | |
Xamarin.Forms | ReactiveUI.XamForms | |
Xamarin.Essentials | ReactiveUI | |
AndroidX (Xamarin) | ReactiveUI.AndroidX | |
Xamarin.Android | ReactiveUI.AndroidSupport | |
Xamarin.iOS | ReactiveUI | |
Xamarin.Mac | ReactiveUI | |
Tizen | ReactiveUI | |
Blazor | ReactiveUI.Blazor | |
Platform Uno | ReactiveUI.Uno | |
Platform Uno | ReactiveUI.Uno.WinUI | |
Avalonia | Avalonia.ReactiveUI | |
Any | ReactiveUI.Validation |
Sponsorship
The core team members, ReactiveUI contributors and contributors in the ecosystem do this open-source work in their free time. If you use ReactiveUI, a serious task, and you'd like us to invest more time on it, please donate. This project increases your income/productivity too. It makes development and applications faster and it reduces the required bandwidth.
This is how we use the donations:
- Allow the core team to work on ReactiveUI
- Thank contributors if they invested a large amount of time in contributing
- Support projects in the ecosystem
Support
If you have a question, please see if any discussions in our GitHub issues or Stack Overflow have already answered it.
If you want to discuss something or just need help, here is our Slack room, where there are always individuals looking to help out!
Please do not open GitHub issues for support requests.
Contribute
ReactiveUI is developed under an OSI-approved open source license, making it freely usable and distributable, even for commercial use.
If you want to submit pull requests please first open a GitHub issue to discuss. We are first time PR contributors friendly.
See Contribution Guidelines for further information how to contribute changes.
Core Team
![]() Glenn Watson Melbourne, Australia |
![]() Chris Pulman United Kingdom |
![]() Rodney Littles II Texas, USA |
![]() Colt Bauman South Korea |
Alumni Core Team
The following have been core team members in the past.
![]() Geoffrey Huntley Sydney, Australia |
![]() Kent Boogaart Brisbane, Australia |
![]() Olly Levett London, United Kingdom |
![]() Anaïs Betts San Francisco, USA |
![]() Brendan Forster Melbourne, Australia |
![]() Claire Novotny New York, USA |
![]() Artyom Gorchakov Moscow, Russia |
.NET Foundation
ReactiveUI is part of the .NET Foundation. Other projects that are associated with the foundation include the Microsoft .NET Compiler Platform ("Roslyn") as well as the Microsoft ASP.NET family of projects, Microsoft .NET Core & Xamarin Forms.
Top Related Projects
Cocoa framework and Obj-C dynamism bindings for ReactiveSwift.
The Reactive Extensions for .NET
Reactive Programming in Swift
RxJava – Reactive Extensions for the JVM – a library for composing asynchronous and event-based programs using observable sequences for the Java VM.
A reactive programming library for JavaScript
The Reactive Extensions for .NET
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot