stitches
[Not Actively Maintained] CSS-in-JS with near-zero runtime, SSR, multi-variant support, and a best-in-class developer experience.
Top Related Projects
👩🎤 CSS-in-JS library designed for high performance style composition
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
Documentation about css-modules
Zero-runtime Stylesheets-in-TypeScript
Zero-runtime CSS in JS library
Quick Overview
Stitches is a lightweight, high-performance CSS-in-JS library with a focus on component-based styling. It offers near-zero runtime, SSR support, and a fully-typed API, making it an excellent choice for building scalable and maintainable user interfaces in React applications.
Pros
- Near-zero runtime overhead, resulting in improved performance
- Fully-typed API, providing better developer experience and type safety
- Server-side rendering (SSR) support out of the box
- Atomic CSS generation for optimized stylesheet size
Cons
- Steeper learning curve compared to traditional CSS or some other CSS-in-JS solutions
- Limited ecosystem and community support compared to more established libraries
- May require additional setup for certain build tools or frameworks
- Not as feature-rich as some other CSS-in-JS libraries
Code Examples
Creating a styled component:
import { styled } from '@stitches/react';
const Button = styled('button', {
backgroundColor: 'gainsboro',
borderRadius: '9999px',
fontSize: '13px',
padding: '10px 15px',
'&:hover': {
backgroundColor: 'lightgray',
},
});
Using variants:
const Button = styled('button', {
// Base styles
padding: '10px 15px',
// Variants
variants: {
color: {
violet: { backgroundColor: 'blueviolet' },
gray: { backgroundColor: 'gainsboro' },
},
size: {
small: { fontSize: '13px' },
large: { fontSize: '15px' },
},
},
});
// Usage
<Button color="violet" size="large">Click me</Button>
Responsive styles:
import { styled, css } from '@stitches/react';
const responsiveStyles = css({
'@bp1': {
fontSize: '14px',
},
'@bp2': {
fontSize: '16px',
},
});
const Text = styled('p', responsiveStyles);
Getting Started
-
Install Stitches:
npm install @stitches/react
-
Create a configuration file (e.g.,
stitches.config.js
):import { createStitches } from '@stitches/react'; export const { styled, css, globalCss, keyframes, getCssText, theme, createTheme, config } = createStitches({ theme: { colors: { gray500: 'hsl(206,10%,76%)', blue500: 'hsl(206,100%,50%)', }, fontSizes: { 1: '13px', 2: '15px', }, }, media: { bp1: '(min-width: 640px)', bp2: '(min-width: 768px)', }, });
-
Use Stitches in your components:
import { styled } from './stitches.config'; const Button = styled('button', { backgroundColor: '$blue500', color: 'white', padding: '10px 15px', '&:hover': { backgroundColor: '$gray500', }, }); export default Button;
Competitor Comparisons
👩🎤 CSS-in-JS library designed for high performance style composition
Pros of Emotion
- More mature and widely adopted, with a larger ecosystem and community support
- Offers a broader range of styling options, including object styles and string styles
- Provides better support for server-side rendering out of the box
Cons of Emotion
- Slightly larger bundle size compared to Stitches
- Runtime performance can be slower, especially with heavy use of dynamic styles
- Less type-safe compared to Stitches' static extraction
Code Comparison
Emotion:
import { css } from '@emotion/react'
const style = css`
color: hotpink;
`
const SomeComponent = ({ children }) => (
<div css={style}>{children}</div>
)
Stitches:
import { styled } from '@stitches/react'
const StyledDiv = styled('div', {
color: 'hotpink',
})
const SomeComponent = ({ children }) => (
<StyledDiv>{children}</StyledDiv>
)
Both libraries offer similar functionality, but Stitches provides a more type-safe approach with its static extraction, while Emotion offers more flexibility in styling options. Stitches focuses on performance and smaller bundle sizes, whereas Emotion has a larger ecosystem and better out-of-the-box server-side rendering support.
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
Pros of styled-components
- More mature ecosystem with extensive community support and resources
- Dynamic theming capabilities with ThemeProvider
- Better support for server-side rendering out of the box
Cons of styled-components
- Larger bundle size due to runtime CSS generation
- Slower performance compared to Stitches, especially for complex applications
- Requires additional setup for static CSS extraction
Code Comparison
styled-components:
const Button = styled.button`
background-color: ${props => props.primary ? 'blue' : 'white'};
color: ${props => props.primary ? 'white' : 'blue'};
padding: 10px 20px;
`;
Stitches:
const Button = styled('button', {
variants: {
primary: {
true: {
backgroundColor: 'blue',
color: 'white',
},
false: {
backgroundColor: 'white',
color: 'blue',
},
},
},
padding: '10px 20px',
});
Both libraries offer component-based styling solutions, but Stitches focuses on performance and type safety. styled-components provides a more familiar CSS-in-JS syntax and better dynamic theming, while Stitches offers improved performance and a smaller bundle size. The choice between them depends on project requirements, team preferences, and performance considerations.
Documentation about css-modules
Pros of CSS Modules
- Simpler learning curve, as it builds on existing CSS knowledge
- Works with any CSS preprocessor (SASS, LESS, etc.)
- Easier integration with existing projects and codebases
Cons of CSS Modules
- Less powerful runtime capabilities compared to Stitches
- Requires additional tooling and build process setup
- Limited theming and variant support out of the box
Code Comparison
CSS Modules:
.button {
background-color: blue;
color: white;
}
import styles from './Button.module.css';
const Button = () => <button className={styles.button}>Click me</button>;
Stitches:
import { styled } from '@stitches/react';
const Button = styled('button', {
backgroundColor: 'blue',
color: 'white',
});
const MyComponent = () => <Button>Click me</Button>;
CSS Modules offers a familiar CSS syntax with scoped class names, while Stitches provides a more JavaScript-centric approach with enhanced runtime capabilities and built-in theming support. CSS Modules is easier to adopt in existing projects, whereas Stitches offers more powerful features for building design systems and component libraries.
Zero-runtime Stylesheets-in-TypeScript
Pros of vanilla-extract
- Zero-runtime CSS-in-JS, resulting in better performance
- Type-safe styles with TypeScript integration
- Supports static extraction of CSS, enabling server-side rendering
Cons of vanilla-extract
- Requires a build step, which may increase complexity
- Less dynamic than runtime CSS-in-JS solutions
- Limited runtime theming capabilities compared to Stitches
Code Comparison
vanilla-extract:
import { style } from '@vanilla-extract/css';
export const button = style({
backgroundColor: 'blue',
color: 'white',
padding: '10px 20px',
});
Stitches:
import { styled } from '@stitches/react';
const Button = styled('button', {
backgroundColor: 'blue',
color: 'white',
padding: '10px 20px',
});
Both vanilla-extract and Stitches offer CSS-in-JS solutions, but with different approaches. vanilla-extract focuses on zero-runtime, type-safe CSS generation, while Stitches provides a more dynamic runtime solution with powerful theming capabilities. The choice between them depends on project requirements, performance needs, and developer preferences.
Zero-runtime CSS in JS library
Pros of Linaria
- Zero runtime CSS-in-JS solution, resulting in better performance
- Supports static extraction of CSS, enabling server-side rendering without additional setup
- Works with any framework or vanilla JavaScript, offering greater flexibility
Cons of Linaria
- Requires additional build step and configuration
- Limited dynamic styling capabilities compared to runtime CSS-in-JS solutions
- Steeper learning curve for developers used to traditional CSS or other CSS-in-JS libraries
Code Comparison
Linaria:
import { css } from '@linaria/core';
const button = css`
background-color: blue;
color: white;
padding: 10px 20px;
`;
Stitches:
import { styled } from '@stitches/react';
const Button = styled('button', {
backgroundColor: 'blue',
color: 'white',
padding: '10px 20px',
});
Both libraries offer a way to create styled components, but Linaria uses template literals while Stitches uses an object-based syntax. Linaria's approach results in static CSS extraction, while Stitches generates styles at runtime. This difference impacts performance and flexibility in dynamic styling scenarios.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Update June 19, 2023: Stitches is no longer actively maintained due to changes in the React ecosystem and maintainer availability. You can read more here.
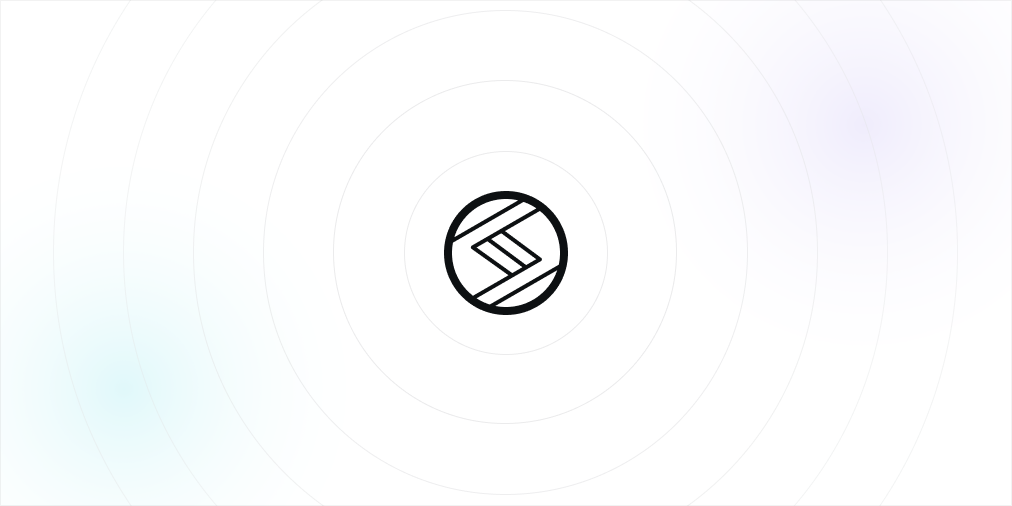
Stitches
Style your components with confidence
CSS-in-JS with near-zero runtime, SSR, multi-variant support, and a best-in-class developer experience.
Stitches Core
Framework-agnostic implementation.
npm install @stitches/core
Stitches React
React wrapper including the styled
API.
npm install @stitches/react
Documentation
For full documentation, visit stitches.dev.
Contributing
Please follow our contributing guidelines.
Community
You can join the Stitches Discord to chat with other members of the community.
Here's a list of community-built projects:
- babel-plugin-transform-stitches-display-name
- stitches-normalize-css
- stitches-crochet
- stitches-native
Authors
License
Licensed under the MIT License, Copyright © 2022-present WorkOS.
See LICENSE for more information.
Top Related Projects
👩🎤 CSS-in-JS library designed for high performance style composition
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress 💅
Documentation about css-modules
Zero-runtime Stylesheets-in-TypeScript
Zero-runtime CSS in JS library
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot