varlet
A Vue3 component library based on Material Design 2 and 3, supporting mobile and desktop.
Top Related Projects
🐉 Vue Component Framework
Quasar Framework - Build high-performance VueJS user interfaces in record time
🌈 An enterprise-class UI components based on Ant Design and Vue. 🐜
🎉 A Vue.js 3 UI Library made by Element team
A Vue.js 2.0 UI Toolkit for Web
Quick Overview
Varlet is a Material design mobile component library for Vue 3. It offers a rich set of UI components and utilities to build responsive and aesthetically pleasing mobile applications. The library is designed with a focus on customization and ease of use.
Pros
- Comprehensive set of Material Design components for mobile development
- Built specifically for Vue 3, leveraging its latest features and performance improvements
- Highly customizable with theming support and component-level customization options
- Well-documented with interactive examples and API references
Cons
- Primarily focused on mobile development, which may limit its use in desktop applications
- Relatively newer compared to some other UI libraries, potentially leading to fewer community resources
- Learning curve for developers not familiar with Material Design principles
Code Examples
- Basic Button Usage:
<template>
<var-button>Default Button</var-button>
<var-button type="primary">Primary Button</var-button>
<var-button type="warning" round>Warning Round Button</var-button>
</template>
- Form Input with Validation:
<template>
<var-form ref="form">
<var-input
v-model="username"
:rules="[(v) => !!v || 'Username is required']"
placeholder="Username"
/>
<var-button type="primary" @click="submit">Submit</var-button>
</var-form>
</template>
<script setup>
import { ref } from 'vue'
const form = ref(null)
const username = ref('')
const submit = () => {
form.value.validate().then(() => {
console.log('Form is valid')
})
}
</script>
- Dialog Component:
<template>
<var-button @click="show = true">Open Dialog</var-button>
<var-dialog v-model:show="show" title="Dialog Title">
<p>This is the dialog content.</p>
<template #footer>
<var-button type="primary" @click="show = false">Close</var-button>
</template>
</var-dialog>
</template>
<script setup>
import { ref } from 'vue'
const show = ref(false)
</script>
Getting Started
-
Install Varlet in your Vue 3 project:
npm i @varlet/ui -S
-
Import and use Varlet in your main.js:
import { createApp } from 'vue' import Varlet from '@varlet/ui' import '@varlet/ui/es/style.js' const app = createApp(App) app.use(Varlet) app.mount('#app')
-
Use Varlet components in your Vue components:
<template> <var-button type="primary">Hello Varlet!</var-button> </template>
Competitor Comparisons
🐉 Vue Component Framework
Pros of Vuetify
- Larger ecosystem and community support
- More comprehensive documentation and examples
- Wider range of pre-built components and layouts
Cons of Vuetify
- Heavier bundle size, which may impact performance
- Steeper learning curve due to its extensive feature set
- Less flexibility in customizing component styles
Code Comparison
Vuetify:
<template>
<v-app>
<v-btn color="primary">Click me</v-btn>
</v-app>
</template>
Varlet:
<template>
<var-button type="primary">Click me</var-button>
</template>
Vuetify uses a more opinionated structure with the v-app
wrapper, while Varlet offers a simpler component usage. Vuetify's components typically use the v-
prefix, whereas Varlet uses var-
.
Both libraries provide Material Design-inspired components, but Varlet focuses on mobile-first development and offers a lighter weight alternative. Vuetify is more suited for large-scale applications with complex UI requirements, while Varlet may be preferable for simpler projects or those prioritizing performance.
Varlet also supports Vue 3 out of the box, whereas Vuetify requires additional configuration for Vue 3 compatibility in its current stable version (Vuetify 3 is in beta as of this comparison).
Quasar Framework - Build high-performance VueJS user interfaces in record time
Pros of Quasar
- More comprehensive ecosystem with support for Vue 3, Capacitor, and Electron
- Larger community and more extensive documentation
- Built-in support for SSR and PWA out of the box
Cons of Quasar
- Steeper learning curve due to its extensive feature set
- Larger bundle size, which may impact initial load times
- Less flexibility for custom styling compared to Varlet
Code Comparison
Quasar component usage:
<template>
<q-btn color="primary" label="Click me" />
</template>
Varlet component usage:
<template>
<var-button type="primary">Click me</var-button>
</template>
Both frameworks offer a similar component-based approach, but Quasar's syntax is more concise. Varlet's components are prefixed with "var-" for easier identification.
Quasar provides a more opinionated structure and a wider range of built-in components, making it suitable for large-scale applications. Varlet, on the other hand, offers a lighter-weight solution with a focus on mobile UI components, making it ideal for simpler mobile-first projects.
While Quasar excels in its comprehensive tooling and cross-platform capabilities, Varlet shines in its simplicity and ease of use for Vue.js developers looking for a straightforward mobile UI framework.
🌈 An enterprise-class UI components based on Ant Design and Vue. 🐜
Pros of Ant Design Vue
- More comprehensive component library with a wider range of UI elements
- Stronger ecosystem and community support, with more frequent updates
- Better documentation and examples for complex use cases
Cons of Ant Design Vue
- Larger bundle size, which may impact performance for smaller projects
- Steeper learning curve due to the extensive API and configuration options
- Less flexibility in customizing the overall design system
Code Comparison
Ant Design Vue:
<template>
<a-button type="primary" @click="handleClick">
Click me
</a-button>
</template>
<script>
import { Button } from 'ant-design-vue';
export default {
components: {
AButton: Button,
},
methods: {
handleClick() {
console.log('Button clicked');
},
},
};
</script>
Varlet:
<template>
<var-button type="primary" @click="handleClick">
Click me
</var-button>
</template>
<script>
import { Button } from '@varlet/ui';
export default {
components: {
VarButton: Button,
},
methods: {
handleClick() {
console.log('Button clicked');
},
},
};
</script>
Both libraries offer similar component usage, but Ant Design Vue typically requires more configuration options for advanced customization, while Varlet aims for a simpler API with fewer props.
🎉 A Vue.js 3 UI Library made by Element team
Pros of Element Plus
- More comprehensive component library with a wider range of UI elements
- Stronger community support and more frequent updates
- Better documentation and examples for developers
Cons of Element Plus
- Larger bundle size, which may impact performance for smaller projects
- Steeper learning curve due to the extensive component library
- Less focus on mobile-first design compared to Varlet
Code Comparison
Element Plus (Vue 3):
<template>
<el-button type="primary">Primary Button</el-button>
</template>
<script>
import { ElButton } from 'element-plus'
export default {
components: { ElButton }
}
</script>
Varlet:
<template>
<var-button type="primary">Primary Button</var-button>
</template>
<script>
import { Button } from '@varlet/ui'
export default {
components: { 'var-button': Button }
}
</script>
Both Element Plus and Varlet are Vue-based UI component libraries, but they cater to different needs. Element Plus offers a more extensive set of components and is better suited for large-scale applications, while Varlet focuses on mobile-first design and lightweight implementation. The code comparison shows that both libraries have similar usage patterns, with slight differences in component naming conventions and import methods.
A Vue.js 2.0 UI Toolkit for Web
Pros of Element
- More mature and widely adopted UI component library for Vue.js
- Extensive documentation and community support
- Larger set of pre-built components and features
Cons of Element
- Primarily designed for Vue 2, with Vue 3 support in a separate package
- Heavier bundle size compared to Varlet
- Less focus on mobile-first design and touch interactions
Code Comparison
Element (Vue 2):
<el-button type="primary" @click="handleClick">Click me</el-button>
Varlet:
<var-button type="primary" @click="handleClick">Click me</var-button>
Key Differences
- Varlet is designed for Vue 3 and focuses on mobile-first development
- Element has a more extensive ecosystem and longer track record
- Varlet offers a lighter-weight solution with a modern component library
- Element provides more comprehensive theming and customization options
- Varlet emphasizes Material Design principles in its component designs
Use Cases
Element is well-suited for:
- Large-scale enterprise applications
- Desktop-first web applications
- Projects requiring extensive UI components and customization
Varlet is ideal for:
- Mobile-first and responsive web applications
- Modern Vue 3 projects
- Developers seeking a lightweight and performant UI library
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Intro
Varlet is a Vue3
component library based on Material Design 2 and 3, supporting mobile and desktop, developed and maintained by varletjs
organization.
Features
- ð Provide 60+ high quality general purpose components
- ð Components are very lightweight
- ðª Developed by Chinese, complete Chinese and English documentation and logistics support
- ð ï¸ Support on-demand introduction
- ð ï¸ Support theme customization
- ð Support internationalization
- ð¡ Support WebStorm syntax highlighting
- ðª Support the SSR
- ð¦ Support Nuxt Module
- ð¡ Support the Typescript
- ðª Make sure more than 90 percent unit test coverage, providing stability assurance
- ð¨ Supports both Material Design 2 and Material Design 3 design systems
- ð ï¸ Support dark mode
- ð§ Provide official VSCode extension
- â¨ï¸ Support Accessibility (still improving)
Install
CDN
varlet.js
contain all the styles and logic of the component library, and you can import them.
<div id="app"></div>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
<!-- Desktop Adaptation -->
<script src="https://cdn.jsdelivr.net/npm/@varlet/touch-emulator/iife.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@varlet/ui/umd/varlet.js"></script>
<script>
const app = Vue.createApp({
template: '<var-button>Button</var-button>'
})
app.use(Varlet).mount('#app')
</script>
Webpack / Vite
# Install with npm or yarn or pnpm
# npm
npm i @varlet/ui -S
# yarn
yarn add @varlet/ui
# pnpm
pnpm add @varlet/ui
import App from './App.vue'
import Varlet from '@varlet/ui'
import { createApp } from 'vue'
import '@varlet/ui/es/style'
createApp(App).use(Varlet).mount('#app')
Official Ecosystem
The following projects are maintained by the official team for a long time.
Name | Description |
---|---|
@varlet/cli | Vue3 component library rapid prototyping tool |
@varlet/touch-emulator | Desktop adapter, so that the mobile component library can run on the desktop |
@varlet/ui-playground | Varlet component library online editing tool |
@varlet/import-resolver | A resolver for unplugin-vue-components used to implement Varlet and import it on demand |
@varlet/preset-unocss | UnoCss presets for varlet |
@varlet/preset-tailwindcss | tailwindcss presets for varlet |
varlet-theme-builder | Theme generator for generating theme variables for varlet Material Design 3 |
varlet-vscode-extension | Varlet Component Library VSCode Plugin |
vscode-theme-varlet | Varlet VSCode Theme |
varlet-app-example | Varlet component library app template |
varlet-install-example | Case collection of Varlet component library and various ecosystem integration |
Community Ecosystem
The following projects are maintained by community personnel, welcome to add.
Name | Description |
---|---|
vue-h5-template | Vue-based mobile template scaffolding, providing mobile presets for Varlet component library |
create-vite-app | A desktop template scaffolding based on Vue3, providing desktop presets for Varlet component library |
vscode-common-intellisense | A VS Code extension that provides better intellisense to Varlet developers |
vue3-varlet-mobile | A mobile template based on Vue 3 and Varlet Component Library |
Playground
See Varlet UI Playground.
Contribution
See Contributing Guide.
GitCode Repo
See Here.
Community
We recommend that issue be used for problem feedback, or others:
- Wechat group
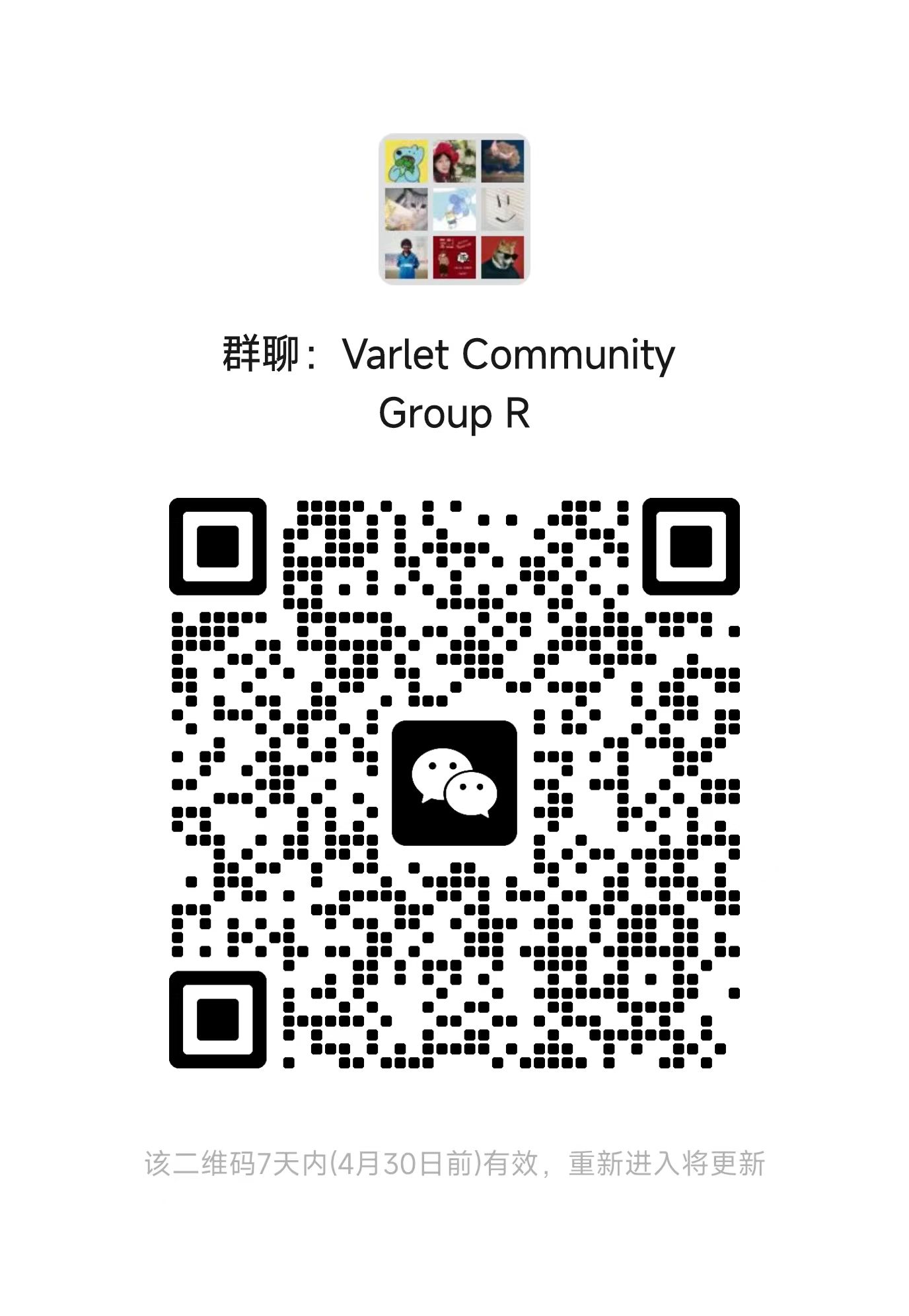
- Join the Discord
Thanks to contributors
Thanks to the following sponsors
Sponsor this project
Sponsor this project to support our better creation.
Wechat / Alipay
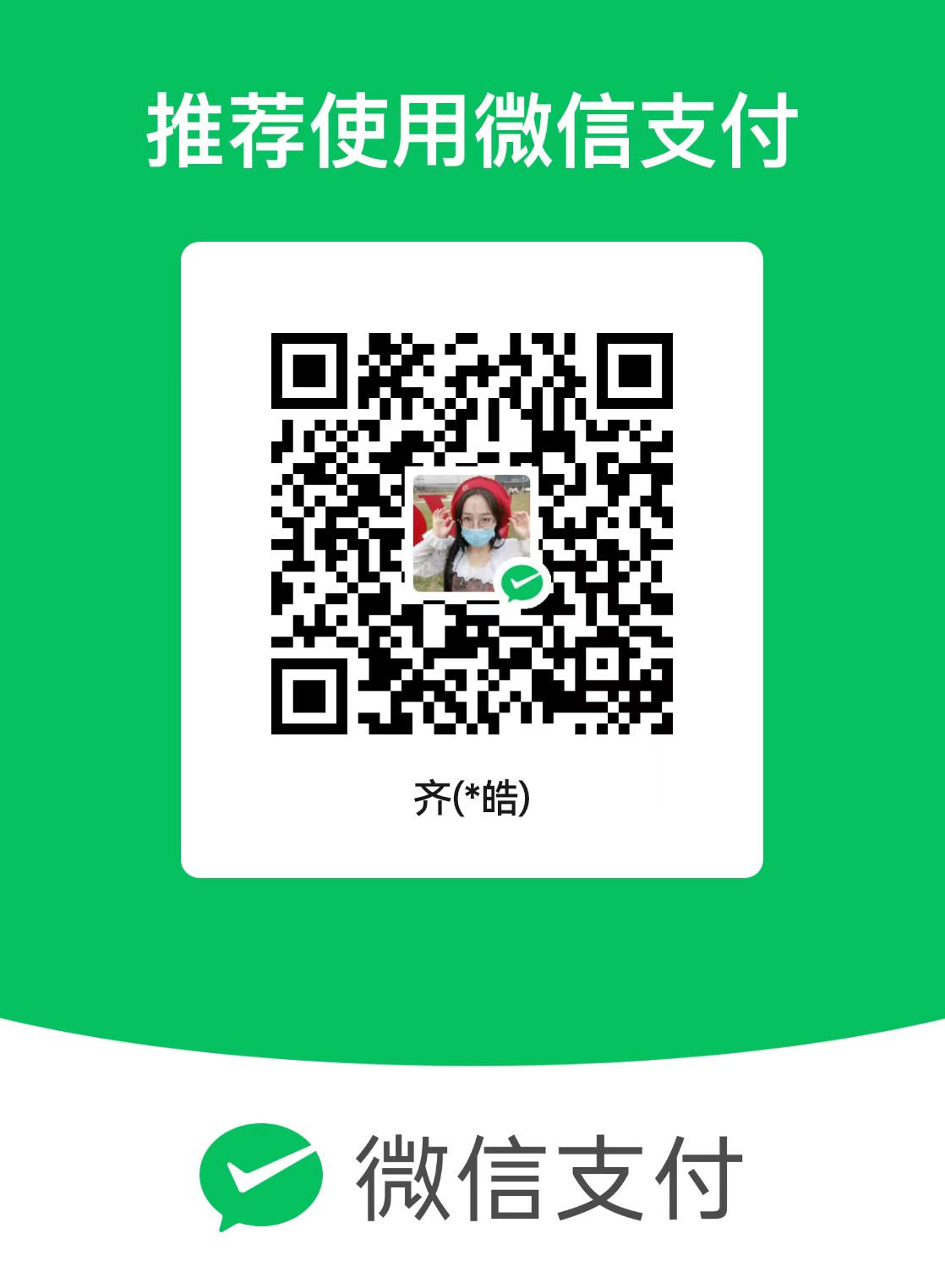
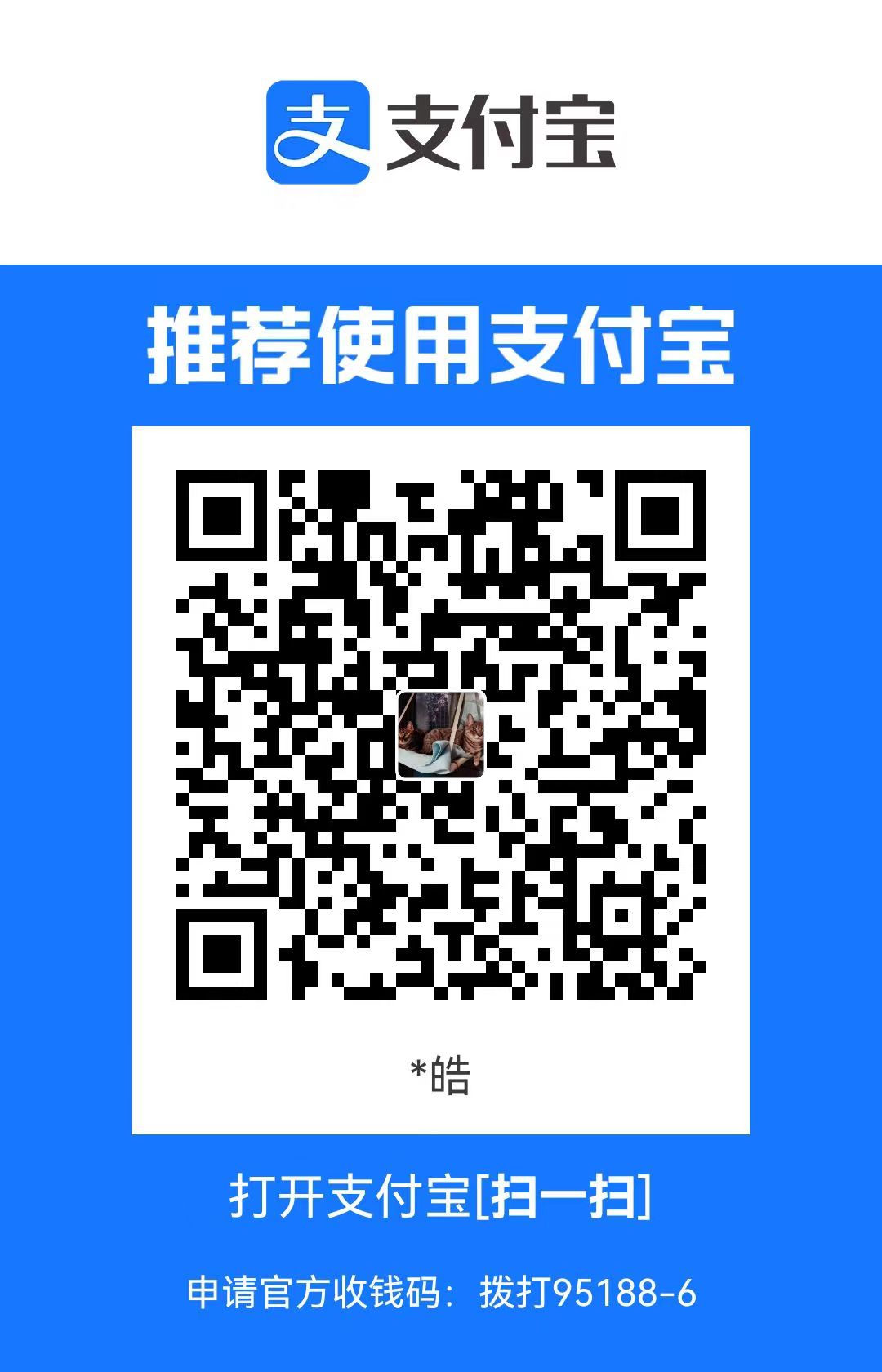
Top Related Projects
🐉 Vue Component Framework
Quasar Framework - Build high-performance VueJS user interfaces in record time
🌈 An enterprise-class UI components based on Ant Design and Vue. 🐜
🎉 A Vue.js 3 UI Library made by Element team
A Vue.js 2.0 UI Toolkit for Web
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot