Top Related Projects
The ChatGPT Retrieval Plugin lets you easily find personal or work documents by asking questions in natural language.
🦜🔗 Build context-aware reasoning applications
A guidance language for controlling large language models.
JARVIS, a system to connect LLMs with ML community. Paper: https://arxiv.org/pdf/2303.17580.pdf
AI orchestration framework to build customizable, production-ready LLM applications. Connect components (models, vector DBs, file converters) to pipelines or agents that can interact with your data. With advanced retrieval methods, it's best suited for building RAG, question answering, semantic search or conversational agent chatbots.
A Gradio web UI for Large Language Models with support for multiple inference backends.
Quick Overview
Chart-GPT is an AI-powered data visualization tool that generates charts and graphs based on natural language prompts. It leverages OpenAI's GPT models to interpret user requests and create appropriate visualizations using the Chart.js library.
Pros
- Intuitive natural language interface for creating charts
- Supports a wide range of chart types and customization options
- Easy integration with existing web applications
- Open-source project with potential for community contributions
Cons
- Requires an OpenAI API key, which may have associated costs
- Limited to the capabilities of Chart.js for visualization options
- May produce inconsistent results depending on the complexity of the prompt
- Potential privacy concerns when sending data to external AI services
Code Examples
- Creating a basic bar chart:
const chartGPT = new ChartGPT(apiKey);
const prompt = "Create a bar chart showing sales data for Q1, Q2, Q3, and Q4";
const chartData = await chartGPT.generateChart(prompt);
new Chart(ctx, chartData);
- Generating a line chart with custom colors:
const prompt = "Generate a line chart of monthly temperatures with red line";
const options = { colors: ['red'] };
const chartData = await chartGPT.generateChart(prompt, options);
new Chart(ctx, chartData);
- Creating a pie chart with specific data:
const prompt = "Create a pie chart of market share: Google 40%, Apple 30%, Microsoft 20%, Others 10%";
const chartData = await chartGPT.generateChart(prompt);
new Chart(ctx, chartData);
Getting Started
To use Chart-GPT in your project, follow these steps:
-
Install the package:
npm install chart-gpt
-
Import and initialize Chart-GPT:
import ChartGPT from 'chart-gpt'; const apiKey = 'your-openai-api-key'; const chartGPT = new ChartGPT(apiKey);
-
Generate a chart:
const prompt = "Create a scatter plot of height vs weight"; const chartData = await chartGPT.generateChart(prompt); new Chart(document.getElementById('myChart'), chartData);
Remember to replace 'your-openai-api-key' with your actual OpenAI API key.
Competitor Comparisons
The ChatGPT Retrieval Plugin lets you easily find personal or work documents by asking questions in natural language.
Pros of chatgpt-retrieval-plugin
- More comprehensive and feature-rich, designed for enterprise-level applications
- Supports multiple vector database options, including Pinecone, Weaviate, and Zilliz
- Offers advanced security features and authentication mechanisms
Cons of chatgpt-retrieval-plugin
- More complex setup and configuration process
- Requires more resources and technical expertise to implement and maintain
- May be overkill for simple chart generation tasks
Code Comparison
chatgpt-retrieval-plugin:
from datastore.providers import DatastoreProvider
from models.models import (
DocumentMetadata,
QueryResult,
DocumentChunkMetadata,
DocumentChunkWithScore,
)
chart-gpt:
import React, { useState } from 'react';
import { Bar } from 'react-chartjs-2';
import { Chart, registerables } from 'chart.js';
Chart.register(...registerables);
The code snippets highlight the different focus areas of the two projects. chatgpt-retrieval-plugin deals with data storage and retrieval, while chart-gpt is centered around chart generation and visualization using React and Chart.js.
chatgpt-retrieval-plugin is better suited for large-scale applications requiring advanced data retrieval and processing capabilities. chart-gpt, on the other hand, is more appropriate for projects that primarily need chart generation functionality with a simpler setup process.
🦜🔗 Build context-aware reasoning applications
Pros of langchain
- More comprehensive framework for building LLM-powered applications
- Larger community and ecosystem with extensive documentation
- Supports multiple LLM providers and integration with various tools
Cons of langchain
- Steeper learning curve due to its extensive features and abstractions
- May be overkill for simple chart generation tasks
- Requires more setup and configuration compared to chart-gpt
Code comparison
chart-gpt:
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": user_input}
]
)
langchain:
from langchain.llms import OpenAI
from langchain.prompts import PromptTemplate
llm = OpenAI(temperature=0.9)
prompt = PromptTemplate(
input_variables=["product"],
template="What is a good name for a company that makes {product}?",
)
chart-gpt is focused specifically on generating charts from natural language input, while langchain provides a more versatile framework for building various LLM-powered applications. chart-gpt offers a simpler, more straightforward approach for chart generation, whereas langchain provides greater flexibility and extensibility at the cost of increased complexity.
A guidance language for controlling large language models.
Pros of guidance
- More comprehensive and flexible language model control framework
- Supports multiple AI models and providers (OpenAI, Anthropic, etc.)
- Offers advanced features like constrained generation and structured outputs
Cons of guidance
- Steeper learning curve due to more complex API and features
- Requires more setup and configuration compared to chart-gpt
- May be overkill for simple chart generation tasks
Code comparison
chart-gpt:
const response = await axios.post('https://api.openai.com/v1/chat/completions', {
model: 'gpt-3.5-turbo',
messages: [{ role: 'user', content: prompt }],
max_tokens: 500,
});
guidance:
import guidance
gpt = guidance.llms.OpenAI("gpt-3.5-turbo")
program = guidance('''
{{#system~}}
You are a helpful assistant.
{{~/system}}
{{#user~}}
{{prompt}}
{{~/user}}
{{#assistant~}}
{{gen 'response' max_tokens=500}}
{{~/assistant}}
''')
executed = program(prompt=user_prompt)
The chart-gpt repository focuses specifically on generating charts using natural language prompts, while guidance provides a more general-purpose framework for controlling language models. guidance offers greater flexibility and advanced features but requires more setup and coding knowledge. chart-gpt is simpler to use for its specific purpose of chart generation but lacks the broader capabilities of guidance.
JARVIS, a system to connect LLMs with ML community. Paper: https://arxiv.org/pdf/2303.17580.pdf
Pros of JARVIS
- More comprehensive AI system with broader capabilities
- Supports multiple modalities including vision and speech
- Backed by Microsoft with extensive resources and research
Cons of JARVIS
- More complex to set up and use
- Requires more computational resources
- Less focused on specific chart generation tasks
Code Comparison
JARVIS (Python):
from jarvis import JARVIS
jarvis = JARVIS()
response = jarvis.run("Analyze this image and describe its contents.")
print(response)
Chart-GPT (JavaScript):
import ChartGPT from 'chart-gpt';
const chartGPT = new ChartGPT();
const chart = await chartGPT.generateChart('Bar chart of sales by quarter');
chart.render();
Summary
JARVIS is a more comprehensive AI system with broader capabilities, while Chart-GPT is focused specifically on chart generation. JARVIS supports multiple modalities and is backed by Microsoft's resources, but it's more complex to use and requires more computational power. Chart-GPT offers a simpler, more targeted solution for creating charts and visualizations using natural language prompts.
AI orchestration framework to build customizable, production-ready LLM applications. Connect components (models, vector DBs, file converters) to pipelines or agents that can interact with your data. With advanced retrieval methods, it's best suited for building RAG, question answering, semantic search or conversational agent chatbots.
Pros of Haystack
- More comprehensive NLP framework with broader capabilities
- Larger community and more active development
- Better documentation and extensive examples
Cons of Haystack
- Steeper learning curve due to its complexity
- Requires more setup and configuration
- May be overkill for simple chart generation tasks
Code Comparison
Haystack (Pipeline creation):
from haystack import Pipeline
p = Pipeline()
p.add_node(component=retriever, name="Retriever", inputs=["Query"])
p.add_node(component=reader, name="Reader", inputs=["Retriever"])
Chart-GPT (Chart generation):
from chart_gpt import ChartGPT
chart_gpt = ChartGPT()
chart = chart_gpt.generate_chart(prompt="Create a bar chart of sales data")
Summary
Haystack is a more robust and versatile NLP framework, offering a wide range of capabilities beyond chart generation. It's better suited for complex NLP tasks and has a larger community. However, it comes with a steeper learning curve and more setup requirements.
Chart-GPT, on the other hand, is focused specifically on chart generation using AI. It's simpler to use for this specific task but lacks the broader functionality of Haystack. Choose Chart-GPT for quick chart creation, and Haystack for more comprehensive NLP projects.
A Gradio web UI for Large Language Models with support for multiple inference backends.
Pros of text-generation-webui
- More comprehensive and feature-rich, supporting multiple models and architectures
- Offers a web-based interface for easier interaction and management
- Provides advanced features like model fine-tuning and parameter customization
Cons of text-generation-webui
- More complex setup and configuration process
- Requires more computational resources due to its extensive features
- Steeper learning curve for users new to language models
Code Comparison
text-generation-webui:
def generate_reply(
question, state, stopping_strings=None, is_chat=False, for_ui=False
):
# Complex generation logic
# ...
chart-gpt:
def generate_chart(prompt):
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=1000,
)
return response.choices[0].text.strip()
text-generation-webui offers more advanced generation options and customization, while chart-gpt focuses specifically on chart generation using OpenAI's API. The code snippets highlight the difference in complexity and scope between the two projects.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Chart-GPT - text to beautiful charts within seconds
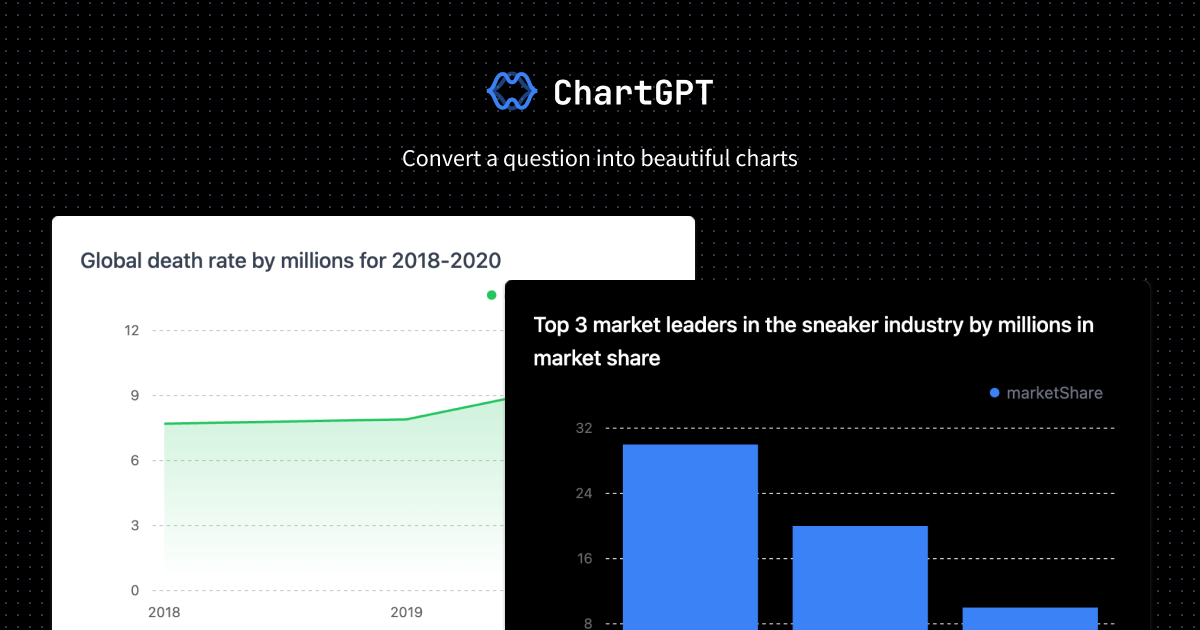

Getting Started
To get started, first clone this repository:
git clone https://github.com/whoiskatrin/chart-gpt.git
cd chart-gpt
Then duplicate the .env.example
template with cp .env.example .env
and add your PaLM API key:
BARD_KEY="your-api-key"
Then install the dependencies and start the development server:
npm install
npm run dev
# or
yarn
yarn dev
This will start the development server at http://localhost:3000.
To use the full functionality of the credit system as well, you'll need to setup Supabase, Stripe, and NextAuth with Google â and their respective environment variables found in the .env.example
file.
Contributing
If you would like to contribute to this project, please follow these steps:
- Fork this repository.
- Clone your forked repository:
- For your changes:
- Make your changes, commit them, and push them to your forked repository:
- Create a pull request on this repository.
Top Related Projects
The ChatGPT Retrieval Plugin lets you easily find personal or work documents by asking questions in natural language.
🦜🔗 Build context-aware reasoning applications
A guidance language for controlling large language models.
JARVIS, a system to connect LLMs with ML community. Paper: https://arxiv.org/pdf/2303.17580.pdf
AI orchestration framework to build customizable, production-ready LLM applications. Connect components (models, vector DBs, file converters) to pipelines or agents that can interact with your data. With advanced retrieval methods, it's best suited for building RAG, question answering, semantic search or conversational agent chatbots.
A Gradio web UI for Large Language Models with support for multiple inference backends.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot