go-zero
A cloud-native Go microservices framework with cli tool for productivity.
Top Related Projects
A standard library for microservices.
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin.
High performance, minimalist Go web framework
⚡️ Express inspired web framework written in Go
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
beego is an open-source, high-performance web framework for the Go programming language.
Quick Overview
go-zero is a web and RPC framework with integrated tools to boost productivity in Go development. It's designed for building microservices, focusing on simplicity, performance, and reliability. The framework provides a comprehensive set of components for rapid development of distributed systems.
Pros
- Comprehensive toolkit for microservices development
- High performance and scalability
- Built-in service governance features (e.g., rate limiting, circuit breaking)
- Strong emphasis on code generation to reduce boilerplate
Cons
- Steeper learning curve for developers new to microservices architecture
- Opinionated structure may not fit all project requirements
- Limited documentation in English (primarily in Chinese)
- Relatively young project compared to some alternatives
Code Examples
- Defining an API using go-zero's API file:
type (
LoginReq {
Username string `json:"username"`
Password string `json:"password"`
}
LoginResp {
AccessToken string `json:"accessToken"`
ExpiresIn int64 `json:"expiresIn"`
}
)
service user-api {
@handler login
post /user/login (LoginReq) returns (LoginResp)
}
- Implementing a simple HTTP handler:
func (l *LoginLogic) Login(req *types.LoginReq) (resp *types.LoginResp, err error) {
// authentication logic here
return &types.LoginResp{
AccessToken: "generated_token",
ExpiresIn: 3600,
}, nil
}
- Using go-zero's built-in middleware:
server := rest.MustNewServer(c.RestConf, rest.WithMiddlewares(
[]rest.Middleware{
middleware.Recover(),
middleware.Metrics(),
}...,
))
Getting Started
-
Install go-zero:
go get -u github.com/zeromicro/go-zero
-
Create an API file (e.g.,
user.api
):type ( RegisterReq { Username string `json:"username"` Password string `json:"password"` } RegisterResp { Message string `json:"message"` } ) service user-api { @handler register post /user/register (RegisterReq) returns (RegisterResp) }
-
Generate code:
goctl api go -api user.api -dir .
-
Implement the logic and run the service:
func main() { server := rest.MustNewServer(c.RestConf) defer server.Stop() server.Start() }
Competitor Comparisons
A standard library for microservices.
Pros of go-kit
- More mature and established project with a larger community
- Highly modular and flexible architecture
- Extensive documentation and examples
Cons of go-kit
- Steeper learning curve due to its flexibility
- Requires more boilerplate code
- Less opinionated, which may lead to inconsistencies across projects
Code Comparison
go-kit example:
type Service interface {
Hello(name string) (string, error)
}
func makeHelloEndpoint(svc Service) endpoint.Endpoint {
return func(ctx context.Context, request interface{}) (interface{}, error) {
req := request.(HelloRequest)
v, err := svc.Hello(req.Name)
return HelloResponse{v}, err
}
}
go-zero example:
type (
HelloReq struct {
Name string `json:"name"`
}
HelloResp struct {
Message string `json:"message"`
}
)
@server(
handler: HelloHandler
)
service hello-api {
@handler Hello
post /hello (HelloReq) returns (HelloResp)
}
go-kit offers more flexibility but requires more code, while go-zero provides a more streamlined approach with less boilerplate. go-kit is better suited for complex microservices architectures, whereas go-zero excels in rapid development and simplicity.
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin.
Pros of gin
- Lightweight and minimalistic, offering high performance
- Easy to learn and use, with a simple API
- Extensive middleware ecosystem
Cons of gin
- Limited built-in features for complex microservices
- Lacks integrated tools for API documentation and testing
- Requires additional libraries for advanced functionalities
Code Comparison
gin:
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{"message": "pong"})
})
r.Run()
go-zero:
type Config struct {
rest.RestConf
}
type Service struct {
c Config
}
func NewService(c Config) *Service {
return &Service{c: c}
}
go-zero is a more comprehensive microservices framework, offering built-in features for distributed systems, while gin is a lightweight web framework focused on simplicity and performance. go-zero provides tools for service discovery, load balancing, and API documentation out of the box, whereas gin requires additional libraries for these functionalities. gin's simplicity makes it easier to learn and use for smaller projects, while go-zero's extensive feature set is better suited for complex microservices architectures.
High performance, minimalist Go web framework
Pros of Echo
- Lightweight and minimalist framework, offering simplicity and ease of use
- Excellent performance with low overhead
- Extensive middleware support and easy customization
Cons of Echo
- Less opinionated, requiring more manual setup for larger projects
- Fewer built-in features compared to go-zero's comprehensive toolkit
- Limited code generation capabilities
Code Comparison
Echo:
e := echo.New()
e.GET("/", func(c echo.Context) error {
return c.String(http.StatusOK, "Hello, World!")
})
e.Logger.Fatal(e.Start(":1323"))
go-zero:
type Config struct {
rest.RestConf
}
func main() {
var c Config
conf.MustLoad("config.yaml", &c)
server := rest.MustNewServer(c.RestConf)
defer server.Stop()
server.Start()
}
Echo provides a more straightforward setup for simple applications, while go-zero offers a more structured approach with built-in configuration management. Echo's routing is more concise, but go-zero provides additional features like automatic API documentation and microservices support out of the box.
Both frameworks have their strengths, with Echo excelling in simplicity and performance for smaller projects, and go-zero offering a more comprehensive toolkit for building complex, scalable microservices.
⚡️ Express inspired web framework written in Go
Pros of Fiber
- Extremely fast performance due to its lightweight design and use of the fasthttp library
- Simple and expressive API, making it easy for developers to quickly build web applications
- Extensive middleware ecosystem and built-in features like WebSocket support
Cons of Fiber
- Less comprehensive than go-zero, focusing primarily on HTTP routing and middleware
- Smaller community and ecosystem compared to more established frameworks
- Limited built-in support for microservices architecture and related patterns
Code Comparison
Fiber:
app := fiber.New()
app.Get("/", func(c *fiber.Ctx) error {
return c.SendString("Hello, World!")
})
app.Listen(":3000")
go-zero:
type Config struct {
rest.RestConf
}
func main() {
var c Config
conf.MustLoad("config.yaml", &c)
server := rest.MustNewServer(c.RestConf)
defer server.Stop()
server.Start()
}
Fiber offers a more concise and straightforward setup for basic HTTP servers, while go-zero provides a more structured approach with built-in configuration management and microservices support. go-zero is better suited for complex, large-scale applications, while Fiber excels in simplicity and raw performance for smaller projects or microservices.
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
Pros of mux
- Lightweight and focused on HTTP routing
- Simple and easy to learn for beginners
- Widely adopted and battle-tested in production environments
Cons of mux
- Limited built-in features compared to go-zero's comprehensive toolkit
- Requires additional libraries for more advanced functionality
- Less suitable for large-scale microservices architectures
Code Comparison
mux:
r := mux.NewRouter()
r.HandleFunc("/api/{key}", handler).Methods("GET")
http.ListenAndServe(":8080", r)
go-zero:
type Config struct {
rest.RestConf
}
s := rest.MustNewServer(c.RestConf)
s.HandleFunc("/api/{key}", handler)
s.Start()
Key Differences
- go-zero provides a more comprehensive toolkit for microservices development
- mux focuses primarily on HTTP routing and is more lightweight
- go-zero includes built-in support for service discovery, load balancing, and distributed tracing
- mux requires additional libraries for advanced features like middleware and validation
- go-zero offers code generation tools for rapid development, while mux relies on manual implementation
Both libraries have their strengths and are suitable for different use cases. mux is ideal for simpler web applications, while go-zero is better suited for complex microservices architectures.
beego is an open-source, high-performance web framework for the Go programming language.
Pros of Beego
- More mature and established framework with a larger community
- Comprehensive documentation and extensive learning resources
- Built-in ORM for database operations
Cons of Beego
- Heavier and more opinionated framework, which may lead to less flexibility
- Steeper learning curve for beginners due to its full-stack nature
- Less focus on microservices architecture compared to Go-Zero
Code Comparison
Beego routing example:
beego.Router("/", &controllers.MainController{})
beego.Router("/api/list", &controllers.APIController{}, "get:List")
beego.Run()
Go-Zero routing example:
server := rest.MustNewServer(c.RestConf)
handler.RegisterHandlers(server, ctx)
server.Start()
Both frameworks offer easy-to-use routing mechanisms, but Go-Zero's approach is more concise and focused on microservices. Beego provides a more traditional MVC-style routing, while Go-Zero emphasizes simplicity and performance for distributed systems.
Go-Zero is designed specifically for microservices, offering features like built-in service discovery and load balancing. Beego, on the other hand, is a full-stack web framework that can be used for various types of applications, including monolithic ones.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
go-zero
go-zero is a web and rpc framework with lots of builtin engineering practices. Itâs born to ensure the stability of the busy services with resilience design and has been serving sites with tens of millions of users for years.
ð¤·â What is go-zero?
English | ç®ä½ä¸æ
go-zero (listed in CNCF Landscape: https://landscape.cncf.io/?selected=go-zero) is a web and rpc framework with lots of builtin engineering practices. Itâs born to ensure the stability of the busy services with resilience design and has been serving sites with tens of millions of users for years.
go-zero contains simple API description syntax and code generation tool called goctl
. You can generate Go, iOS, Android, Kotlin, Dart, TypeScript, JavaScript from .api files with goctl
.
Advantages of go-zero:
- Improves the stability of the services with tens of millions of daily active users
- Builtin chained timeout control, concurrency control, rate limit, adaptive circuit breaker, adaptive load shedding, even no configuration needed
- Builtin middlewares also can be integrated into your frameworks
- Simple API syntax, one command to generate a couple of different languages
- Auto validate the request parameters from clients
- Plenty of builtin microservice management and concurrent toolkits
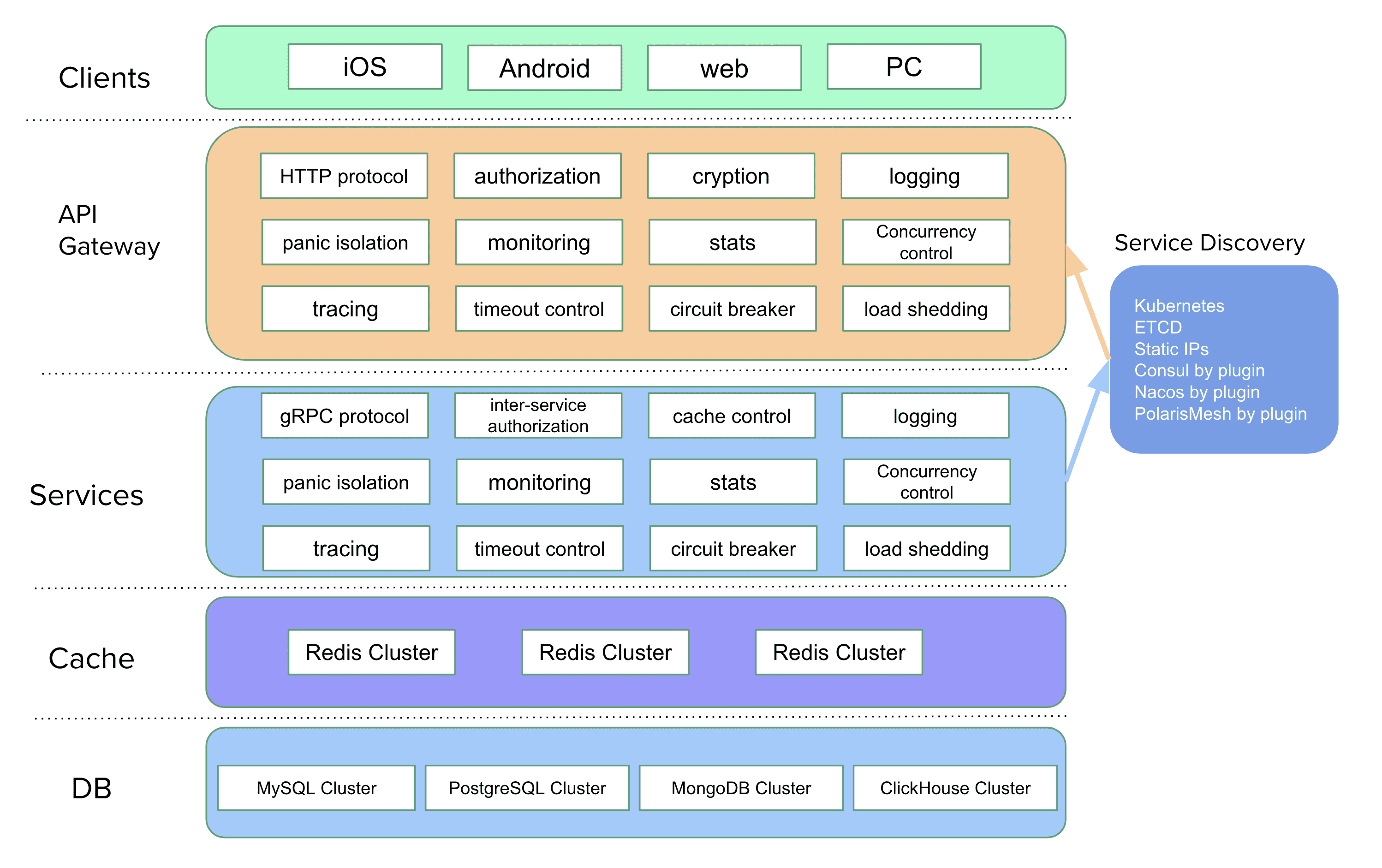
Backgrounds of go-zero
In early 2018, we embarked on a transformative journey to redesign our system, transitioning from a monolithic architecture built with Java and MongoDB to a microservices architecture. After careful research and comparison, we made a deliberate choice to:
-
Go Beyond with Golang
- Great performance
- Simple syntax
- Proven engineering efficiency
- Extreme deployment experience
- Less server resource consumption
-
Self-Design Our Microservice Architecture
- Microservice architecture facilitates the creation of scalable, flexible, and maintainable software systems with independent, reusable components.
- Easy to locate the problems within microservices.
- Easy to extend the features by adding or modifying specific microservices without impacting the entire system.
Design considerations on go-zero
By designing the microservice architecture, we expected to ensure stability, as well as productivity. And from just the beginning, we have the following design principles:
- Keep it simple
- High availability
- Stable on high concurrency
- Easy to extend
- Resilience design, failure-oriented programming
- Try best to be friendly to the business logic development, encapsulate the complexity
- One thing, one way
After almost half a year, we finished the transfer from a monolithic system to microservice system and deployed on August 2018. The new system guaranteed business growth and system stability.
The implementation and features of go-zero
go-zero is a web and rpc framework that integrates lots of engineering practices. The features are mainly listed below:
- Powerful tool included, less code to write
- Simple interfaces
- Fully compatible with net/http
- Middlewares are supported, easy to extend
- High performance
- Failure-oriented programming, resilience design
- Builtin service discovery, load balancing
- Builtin concurrency control, adaptive circuit breaker, adaptive load shedding, auto-trigger, auto recover
- Auto validation of API request parameters
- Chained timeout control
- Auto management of data caching
- Call tracing, metrics, and monitoring
- High concurrency protected
As below, go-zero protects the system with a couple of layers and mechanisms:
The simplified architecture that we use with go-zero
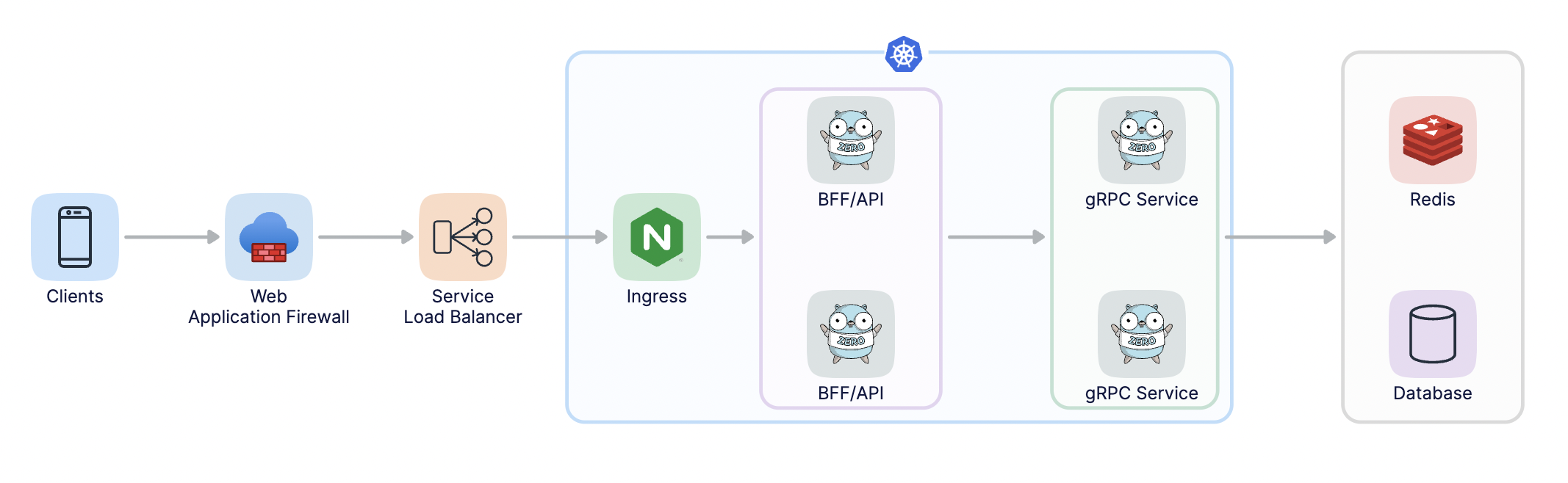
Installation
Run the following command under your project:
go get -u github.com/zeromicro/go-zero
Quick Start
-
Full examples can be checked out from below:
-
Install goctl
goctl
can be read asgo control
.goctl
means not to be controlled by code, instead, we control it. The insidego
is notgolang
. At the very beginning, I was expecting it to help us improve productivity, and make our lives easier.# for Go go install github.com/zeromicro/go-zero/tools/goctl@latest # For Mac brew install goctl # docker for all platforms docker pull kevinwan/goctl # run goctl docker run --rm -it -v `pwd`:/app kevinwan/goctl --help
make sure goctl is executable and in your $PATH.
-
Create the API file, like greet.api, you can install the plugin of goctl in vs code, api syntax is supported.
type ( Request { Name string `path:"name,options=[you,me]"` // parameters are auto validated } Response { Message string `json:"message"` } ) service greet-api { @handler GreetHandler get /greet/from/:name(Request) returns (Response) }
the .api files also can be generated by goctl, like below:
goctl api -o greet.api
-
Generate the go server-side code
goctl api go -api greet.api -dir greet
the generated files look like:
âââ greet â  âââ etc â  â  âââ greet-api.yaml // configuration file â  âââ greet.go // main file â  âââ internal â  âââ config â  â  âââ config.go // configuration definition â  âââ handler â  â  âââ greethandler.go // get/put/post/delete routes are defined here â  â  âââ routes.go // routes list â  âââ logic â  â  âââ greetlogic.go // request logic can be written here â  âââ svc â  â  âââ servicecontext.go // service context, mysql/redis can be passed in here â  âââ types â  âââ types.go // request/response defined here âââ greet.api // api description file
the generated code can be run directly:
cd greet go mod tidy go run greet.go -f etc/greet-api.yaml
by default, itâs listening on port 8888, while it can be changed in the configuration file.
you can check it by curl:
curl -i http://localhost:8888/greet/from/you
the response looks like below:
HTTP/1.1 200 OK Date: Sun, 30 Aug 2020 15:32:35 GMT Content-Length: 0
-
Write the business logic code
- the dependencies can be passed into the logic within servicecontext.go, like mysql, redis, etc.
- add the logic code in a logic package according to .api file
-
Generate code like Java, TypeScript, Dart, JavaScript, etc. just from the api file
goctl api java -api greet.api -dir greet goctl api dart -api greet.api -dir greet ...
Benchmark
Documents
- Documents
- Rapid development of microservice systems
- Rapid development of microservice systems - multiple RPCs
- Examples
Chat group
Join the chat via https://discord.gg/4JQvC5A4Fe
Cloud Native Landscape
go-zero enlisted in the CNCF Cloud Native Landscape.
Give a Star! â
If you like this project or are using it to learn or start your own solution, give it a star to get updates on new releases. Your support matters!
Buy me a coffee
Top Related Projects
A standard library for microservices.
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin.
High performance, minimalist Go web framework
⚡️ Express inspired web framework written in Go
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
beego is an open-source, high-performance web framework for the Go programming language.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot