SpringCloud
基于SpringCloud2.1的微服务开发脚手架,整合了spring-security-oauth2、nacos、feign、sentinel、springcloud-gateway等。服务治理方面引入elasticsearch、skywalking、springboot-admin、zipkin等,让项目开发快速进入业务开发,而不需过多时间花费在架构搭建上。持续更新中
Top Related Projects
Spring Cloud Alibaba provides a one-stop solution for application development for the distributed solutions of Alibaba middleware.
Integration with Netflix OSS components
mall项目是一套电商系统,包括前台商城系统及后台管理系统,基于SpringBoot+MyBatis实现,采用Docker容器化部署。 前台商城系统包含首页门户、商品推荐、商品搜索、商品展示、购物车、订单流程、会员中心、客户服务、帮助中心等模块。 后台管理系统包含商品管理、订单管理、会员管理、促销管理、运营管理、内容管理、统计报表、财务管理、权限管理、设置等模块。
一个涵盖六个专栏:Spring Boot 2.X、Spring Cloud、Spring Cloud Alibaba、Dubbo、分布式消息队列、分布式事务的仓库。希望胖友小手一抖,右上角来个 Star,感恩 1024
《史上最简单的Spring Cloud教程源码》
Quick Overview
SpringCloud is a comprehensive microservices architecture project based on Spring Cloud. It provides a complete set of microservices solutions, including service discovery, configuration management, API gateway, circuit breaker, and more. The project aims to demonstrate best practices for building and deploying microservices using Spring Cloud.
Pros
- Offers a complete microservices ecosystem with various components integrated
- Provides detailed documentation and examples for each module
- Implements security features, including OAuth2 authentication and authorization
- Includes monitoring and logging solutions for better observability
Cons
- Some parts of the documentation are in Chinese, which may be challenging for non-Chinese speakers
- The project is quite complex, which might be overwhelming for beginners
- Some dependencies may be outdated and require updates
- Limited community support compared to more popular Spring Cloud examples
Code Examples
- Service Registration with Eureka
@SpringBootApplication
@EnableEurekaClient
public class ServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceApplication.class, args);
}
}
This code snippet shows how to enable Eureka client for service registration.
- API Gateway Configuration
spring:
cloud:
gateway:
routes:
- id: user-service
uri: lb://USER-SERVICE
predicates:
- Path=/user/**
This YAML configuration demonstrates how to set up a route in the API Gateway.
- Circuit Breaker with Hystrix
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String callService() {
// Service call logic
}
public String fallbackMethod() {
return "Fallback response";
}
This example shows how to use Hystrix for implementing a circuit breaker pattern.
Getting Started
-
Clone the repository:
git clone https://github.com/zhoutaoo/SpringCloud.git
-
Navigate to the project directory:
cd SpringCloud
-
Build the project using Maven:
mvn clean install
-
Start the required services (e.g., Eureka, Config Server, Gateway):
java -jar auth/authentication-server/target/authentication-server.jar java -jar gateway/gateway-web/target/gateway-web.jar java -jar center/eureka/target/eureka-server.jar
-
Access the Eureka dashboard at
http://localhost:8761
to verify service registration.
Competitor Comparisons
Spring Cloud Alibaba provides a one-stop solution for application development for the distributed solutions of Alibaba middleware.
Pros of spring-cloud-alibaba
- Extensive integration with Alibaba Cloud services
- Larger community and more frequent updates
- Comprehensive documentation and examples
Cons of spring-cloud-alibaba
- Steeper learning curve due to more complex features
- Potential vendor lock-in with Alibaba Cloud services
Code Comparison
SpringCloud:
@SpringBootApplication
@EnableDiscoveryClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
spring-cloud-alibaba:
@SpringBootApplication
@EnableDiscoveryClient
@EnableFeignClients
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Key Differences
- spring-cloud-alibaba offers more advanced features like Sentinel for circuit breaking and flow control
- SpringCloud focuses on a simpler, more straightforward implementation of microservices
- spring-cloud-alibaba provides better support for distributed configuration management with Nacos
Use Cases
- SpringCloud: Ideal for smaller projects or teams new to microservices architecture
- spring-cloud-alibaba: Better suited for large-scale applications, especially those leveraging Alibaba Cloud services
Community and Support
- spring-cloud-alibaba has a larger user base and more active development
- SpringCloud offers a simpler codebase, making it easier for contributors to understand and modify
Integration with Netflix OSS components
Pros of Spring Cloud Netflix
- Official Spring Cloud project with extensive documentation and community support
- Comprehensive set of tools for building microservices, including service discovery, load balancing, and circuit breakers
- Seamless integration with other Spring Cloud components
Cons of Spring Cloud Netflix
- Larger footprint and potentially higher resource consumption
- More complex configuration and setup process
- Some components (e.g., Hystrix) are in maintenance mode
Code Comparison
SpringCloud:
@SpringBootApplication
@EnableDiscoveryClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Spring Cloud Netflix:
@SpringBootApplication
@EnableEurekaClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The main difference in the code is the use of @EnableDiscoveryClient
in SpringCloud versus @EnableEurekaClient
in Spring Cloud Netflix. SpringCloud uses a more generic annotation, while Spring Cloud Netflix specifically enables Eureka for service discovery.
Both projects aim to simplify microservices development, but Spring Cloud Netflix offers a more comprehensive and widely adopted solution, while SpringCloud provides a lighter alternative with potentially easier setup and configuration.
mall项目是一套电商系统,包括前台商城系统及后台管理系统,基于SpringBoot+MyBatis实现,采用Docker容器化部署。 前台商城系统 包含首页门户、商品推荐、商品搜索、商品展示、购物车、订单流程、会员中心、客户服务、帮助中心等模块。 后台管理系统包含商品管理、订单管理、会员管理、促销管理、运营管理、内容管理、统计报表、财务管理、权限管理、设置等模块。
Pros of mall
- More comprehensive e-commerce functionality, including product management, order processing, and user systems
- Extensive documentation and deployment guides, making it easier for developers to understand and implement
- Active community with frequent updates and contributions
Cons of mall
- Larger codebase and more complex architecture, potentially harder to grasp for beginners
- Focused specifically on e-commerce, less flexible for other types of applications
- Heavier resource requirements due to its comprehensive nature
Code Comparison
mall:
@ApiOperation("添加商品")
@RequestMapping(value = "/create", method = RequestMethod.POST)
@ResponseBody
public CommonResult create(@RequestBody PmsProductParam productParam) {
int count = productService.create(productParam);
if (count > 0) {
return CommonResult.success(count);
} else {
return CommonResult.failed();
}
}
SpringCloud:
@PostMapping("/product")
public ResponseEntity<Product> createProduct(@RequestBody Product product) {
Product savedProduct = productService.save(product);
return ResponseEntity.ok(savedProduct);
}
The mall project provides more detailed API documentation and uses a custom result object, while SpringCloud uses standard Spring ResponseEntity. Mall's code is more tailored for e-commerce, whereas SpringCloud's is more generic.
一个涵盖六个专栏:Spring Boot 2.X、Spring Cloud、Spring Cloud Alibaba、Dubbo、分布式消息队列、分布式事务的仓库。希望胖友小手一抖,右上角来个 Star,感恩 1024
Pros of SpringBoot-Labs
- More comprehensive coverage of Spring Boot topics and features
- Regularly updated with new examples and technologies
- Better organized structure with separate modules for different concepts
Cons of SpringBoot-Labs
- Lacks focus on microservices architecture compared to SpringCloud
- May be overwhelming for beginners due to the large number of examples
- Less emphasis on production-ready configurations
Code Comparison
SpringBoot-Labs:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
SpringCloud:
@SpringCloudApplication
@EnableFeignClients
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The main difference in the code snippets is the use of @SpringCloudApplication
and @EnableFeignClients
annotations in SpringCloud, which are specific to microservices architecture and inter-service communication. SpringBoot-Labs uses the standard @SpringBootApplication
annotation, focusing on general Spring Boot applications.
SpringBoot-Labs provides a wider range of examples and topics related to Spring Boot, making it suitable for learning various aspects of the framework. On the other hand, SpringCloud is more focused on building microservices-based applications using Spring Cloud components.
《史上最简单的Spring Cloud教程源码》
Pros of SpringCloudLearning
- More beginner-friendly with step-by-step tutorials and explanations
- Covers a wider range of Spring Cloud components and features
- Regularly updated with newer Spring Cloud versions
Cons of SpringCloudLearning
- Less comprehensive in terms of a complete microservices architecture
- Lacks advanced security implementations and configurations
- Doesn't include as many real-world scenarios or production-ready examples
Code Comparison
SpringCloudLearning:
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
SpringCloud:
@SpringBootApplication
@EnableEurekaServer
@EnableResourceServer
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
The SpringCloud example includes additional security-related annotations, demonstrating a more production-ready approach to service discovery implementation.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
æ ¹æ®åæç使ç¨ååé¦ï¼ç®åå°èææ¶æ´ä½è¿è¡äºéæï¼åå¸äºæ°çæ¡æ¶ Opensabreï¼å½åä»åºä»£ç æåç»´æ¤ï¼è¯·ä½¿ç¨æ°ç
弿¾æºç ï¼ https://github.com/opensabre/opensabre-framework
å¨çº¿ææ¡£ï¼ å¨çº¿ææ¡£
è¿ç¾¤äº¤æµï¼ 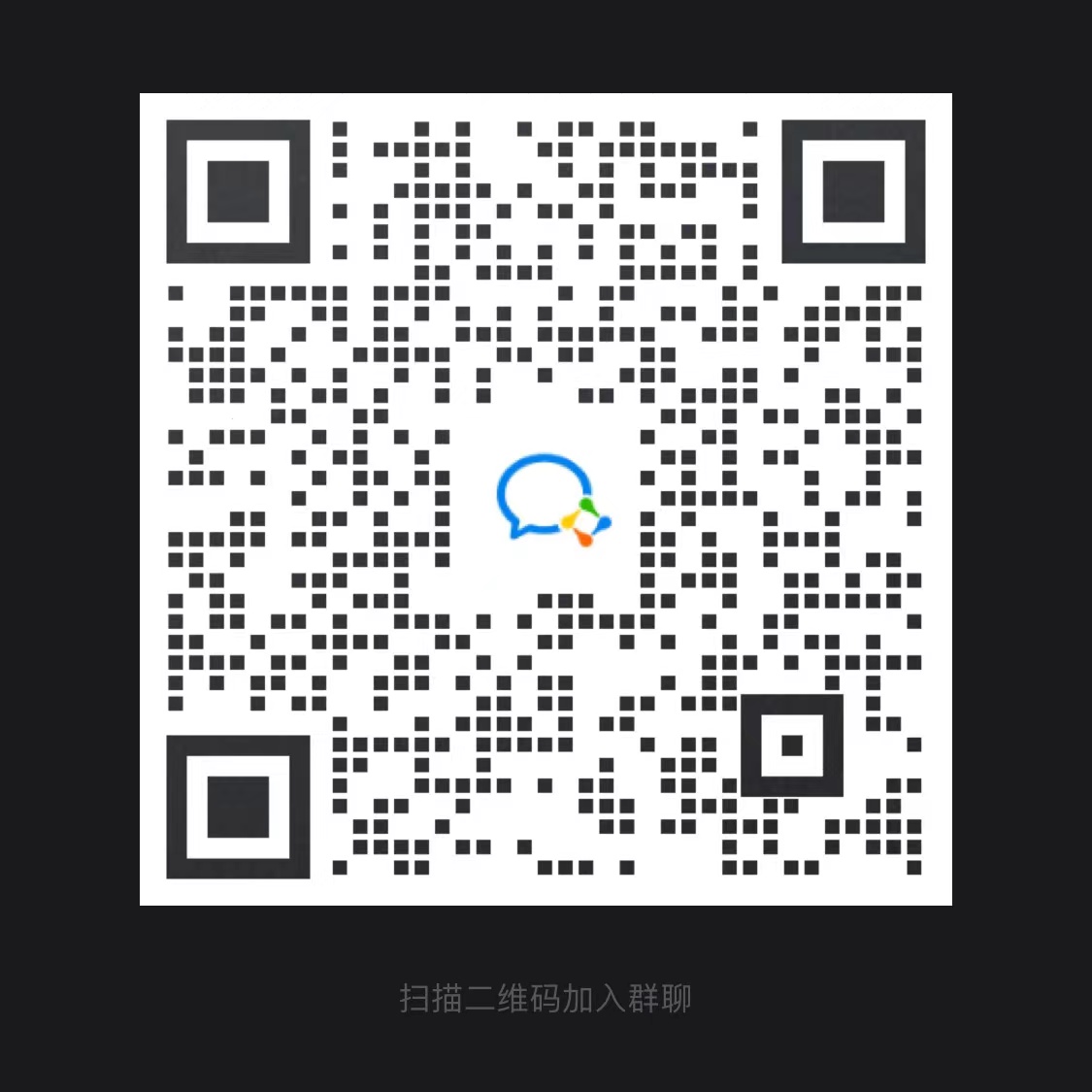
æ¶æç¹ç¹
1. ç»ä¸Restfulçååºæ¥æï¼controllè¿ååå§ç±»åå³å¯ï¼æ éæå¨å
è£
ï¼ç®å代ç ï¼å¯è¯»æ§æ´å¥½ã
2. ç»ä¸å¼å¸¸å¤çï¼å°è£
äºåºæ¬çå¼å¸¸çååºï¼å¦åæ°æ£éªãæä»¶ä¸ä¼ çãç®å代ç ï¼æ´æ¹ä¾¿æ©å±ã
3. é»è®¤éæSwagger 3.0 APIææ¡£ï¼æ¹ä¾¿æ¥å£ææ¡£çä¼ éãåä½ä¸è°è¯ã
4. æ ååWEBå¯¹è±¡ä¼ é/转æ¢/使ç¨ï¼æ¹ä¾¿ç»ä¸å¼å飿 ¼ï¼ç®åæä½ã
5. æ¡æ¶/ç¯å¢çå
æ°æ®èªå¨æ¶é注åè³propertiesåNacosï¼æ¹ä¾¿ç³»ç»è¿è¡æ¶ä½ä¸ºæ©å±å¤æï¼ä¿¡æ¯å¤çã
6. ç³»ç»å¯å¨æ¶èªå¨æ¶éææRestful url注åå°æéèµæºï¼æ¹ä¾¿è¿è¡é䏿é管çåææä½¿ç¨ã
7. 夿ºæ¿/åæ´»è·¯ç±è´è½½æ©å±æ¯æï¼èªå®ä¹è·¯ç±åè´è½½è§åï¼æ´çµæ´»ã坿§ã
8. é»è®¤å¼å
¥spring validationï¼å¹¶æ©å±æä¸¾ãææºå·çå¸¸ç¨æ ¡éªæ³¨è§£ã
9. é»è®¤å¼å
¥æ¥å¿traceãactuatorçç»ä»¶ï¼ç»ä¸æ¥å¿æå°æ ¼å¼ã
10. æ´ä½ç³»ç»å为ä¸å±ï¼frameworkæ¡æ¶ãframeworkç»ä»¶ãåºç¡åºç¨ï¼å±æ¬¡æ´æ¸
æ¥ï¼ç»ææ´åçã
11. é
ç½®ä¸å¿ï¼ååæ¡æ¶å
¨å±é
ç½®ä¸åºç¨é
ç½®ï¼çæé级ãç½å
³è·¯ç±ï¼ï¼è§åä¸ã
å¿«éå¼å§
å 峿¡ä»¶
é¦å æ¬æºå è¦å®è£ 以ä¸ç¯å¢ï¼å»ºè®®å å¦ä¹ äºè§£springbootåspringcloudåºç¡ç¥è¯ã
å¼åç¯å¢æå»º
linuxåmacä¸å¯å¨é¡¹ç®æ ¹ç®å½ä¸æ§è¡ ./install.sh
å¿«éæå»ºå¼åç¯å¢ãå¦è¦äºè§£å
·ä½çæ¥éª¤ï¼è¯·çå¦ä¸ææ¡£ã
å ·ä½æ¥éª¤å¦ä¸ï¼
-
å é代ç åºï¼
git clone https://github.com/zhoutaoo/SpringCloud.git
-
å®è£ å ¬å ±åºå°æ¬å°ä»åºï¼
mvn -pl ./common,./auth/authentication-client install
- çæideé
ç½®ï¼
mvn idea:idea
æmvn eclipse:eclipse
å¹¶å¯¼å ¥å¯¹åºçideè¿è¡å¼åï¼IDEå®è£ lombokæä»¶ï¼å¾éè¦ï¼å¦åIDE伿¾ç¤ºç¼è¯æ¥éï¼
ç¼è¯ & å¯å¨
- 1.å¯å¨åºç¡æå¡ï¼è¿å
¥docker-composeç®å½ï¼æ§è¡
docker-compose -f docker-compose.yml up
æå个å¯å¨docker-compose up æå¡å
, æå¡åå¦ä¸
å¨å¯å¨åºç¨ä¹åï¼éè¦å å¯å¨æ°æ®åºãç¼åãMQçä¸é´ä»¶ï¼å¯æ ¹æ®èªå·±éè¦å¯å¨çåºç¨éæ©å¯å¨æäºåºç¡ç»ä»¶ï¼ä¸è¬æ¥è¯´å¯å¨mysqlãredisãrabbitmqå³å¯ï¼å ¶å®ç»ä»¶è¥æéè¦ï¼æ ¹æ®å¦ä¸å½ä»¤å¯å¨å³å¯ã
该æ¥éª¤ä½¿ç¨äºdockerå¿«éæå»ºç¸åºçåºç¡ç¯å¢ï¼éè¦ä½ 对dockerãdocker-composeæä¸å®äºè§£å使ç¨ç»éªãä¹å¯ä»¥ä¸ä½¿ç¨dockerï¼èªè¡æå»ºä»¥ä¸æå¡å³å¯ã
æå¡ | æå¡å | ç«¯å£ | 夿³¨ |
---|---|---|---|
æ°æ®åº | mysql | 3306 | ç®åååºç¨å ±ç¨1个å®ä¾ï¼ååºç¨å¯å»ºä¸åçdatabase |
KVç¼å | redis | 6379 | ç®åå ±ç¨ï¼ååä¸ååºç¨åç¬å®ä¾ |
æ¶æ¯ä¸é´ä»¶ | rabbitmq | 5672 | å ±ç¨ |
注åä¸é ç½®ä¸å¿ | nacos | 8848 | å¯å¨åä½¿ç¨ææ¡£ |
æ¥å¿æ¶éä¸é´ä»¶ | zipkin-server | 9411 | å ±ç¨ |
æç´¢å¼æä¸é´ä»¶ | elasticsearch | 9200 | å ±ç¨ |
æ¥å¿åæå·¥å · | kibana | 5601 | å ±ç¨ |
æ°æ®å¯è§åå·¥å · | grafana | 3000 | å ±ç¨ |
- 2.åå»ºæ°æ®åºå表
åªæé¨ååºç¨ææ°æ®åºèæ¬ï¼è¥å¯å¨çåºç¨ææ°æ®åºçä¾èµï¼è¯·å使åè¡¨ç»æåæ°æ®ååå¯å¨åºç¨ã
dockeræ¹å¼èæ¬å使åï¼è¿å
¥docker-composeç®å½ï¼æ§è¡å½ä»¤ docker-compose up mysql-init
å项ç®èæ¬
è·¯å¾ä¸è¬ä¸ºï¼å项ç®/db
å¦ï¼auth/db
ä¸çèæ¬ï¼è¯·å
æ§è¡ddl建ç«è¡¨ç»æååæ§è¡dmlæ°æ®å使å
åºç¨èæ¬
è·¯å¾ä¸è¬ä¸ºï¼å项ç®/åºç¨å/src/main/db
å¦ï¼demos/producer/src/main/db ä¸çèæ¬
- 3.å¯å¨åºç¨
æ ¹æ®èªå·±éè¦ï¼å¯å¨ç¸åºæå¡è¿è¡æµè¯ï¼cd è¿å
¥ç¸å
³åºç¨ç®å½ï¼æ§è¡å½ä»¤ï¼ mvn spring-boot:run
以ä¸åºç¨é½ä¾èµäºrabbitmqãnacosï¼å¯å¨æå¡å请å å¯å¨mqåæ³¨åä¸å¿
æå¡åç±» | æå¡å | ä¾èµåºç¡ç»ä»¶ | ç®ä» | åºç¨å°å | ææ¡£ |
---|---|---|---|---|---|
center | bus-server | æ¶æ¯ä¸å¿ | http://localhost:8071 | æ¶æ¯ä¸å¿ææ¡£ | |
sysadmin | organization | mysqlãredis | ç¨æ·ç»ç»åºç¨ | http://localhost:8010 | å¾ å®å |
auth | authorization-server | mysqlãorganization | æææå¡ | http://localhost:8000 | æéæå¡ç®ä» ãææserverææ¡£ |
auth | authentication-server | mysqlãorganization | è®¤è¯æå¡ | http://localhost:8001 | 认è¯serverææ¡£ |
auth | authentication-client | æ | 认è¯å®¢æ·ç«¯ | jarå å¼å ¥ | |
gateway | gateway-web | redis | WEBç½å ³ | http://localhost:8443 | WEBç½å ³ç®ä» WEBç½å ³ææ¡£ |
gateway | gateway-admin | mysqlãredis | ç½å ³ç®¡ç | http://localhost:8445 | ç½å ³ç®¡çåå°ææ¡£ |
monitor | admin | æ»ä½çæ§ | http://localhost:8022 |
- 4.æ¡ä¾ç¤ºæå¾
以䏿¯ä¸ä¸ªç¨æ·è®¿é®çç示æå¾ï¼ç¨æ·è¯·æ±éè¿gateway-webåºç¨ç½å ³è®¿é®å端åºç¨ï¼éè¿authorization-serveråºç¨ç»éæææ¢åtokenï¼è¯·æ±éè¿authentication-serveråºç¨è¿è¡æéç¾å«å转åå°"æ¨çä¸å¡åºç¨"ä¸
authorization-server为ææåºç¨ï¼å¯å¨å请å使åå¥½æ°æ®åºï¼ææServerææ¡£ã
authentication-serverä¸ºç¾æåºç¨ï¼è¥ææ°å¢æ¥å£ï¼è¯·å使åç¸å ³æéæ°æ®å°resource表ä¸ã
gateway-adminå¯å¨æè°æ´gateway-webçè·¯ç±çç¥ï¼æµè¯å请å é ç½®ç½å ³ç转åçç¥ï¼è·¯ç±çç¥é ç½®ã
- 6.å端项ç®
确确ä¿gateway-adminãgateway-webãorganizationãauthorization-serverãauthentication-serveræå¡å¯å¨ï¼ç¶åå¯å¨
å端项ç®ï¼è¯¥é¡¹ç®ç®åè¿å¨å¼åä¸ï¼
大家å¯å¨å¦æé®é¢ï¼å¯ä»¥å å°è¿éççï¼ä¹å¯ä»¥å å ¥äº¤æµç¾¤
æµè¯
è¿è¡ mvn test
å¯å¨æµè¯.
æ¶æä¸å¼å
å¼åæå
åè½ä¸ç¹æ§
åè½é¢è§
ç¨æ·ç®¡ç
è§è²ç®¡ç
æå¡å®¹é
APIææ¡£
ç»ç»æ¶æç®¡ç
åºç¡æå¡
æå¡ | ä½¿ç¨ææ¯ | è¿åº¦ | 夿³¨ |
---|---|---|---|
注åä¸å¿ | Nacos | â | |
é ç½®ä¸å¿ | Nacos | â | |
æ¶æ¯æ»çº¿ | SpringCloud Bus+Rabbitmq | â | |
ç°åº¦åæµ | OpenResty + lua | ð | |
卿ç½å ³ | SpringCloud Gateway | â | å¤ç§ç»´åº¦çæµéæ§å¶ï¼æå¡ãIPãç¨æ·çï¼ï¼å端å¯é ç½®åð |
ææè®¤è¯ | Spring Security OAuth2 | â | Jwtæ¨¡å¼ |
æå¡å®¹é | SpringCloud Sentinel | â | |
æå¡è°ç¨ | SpringCloud OpenFeign | â | |
对象åå¨ | FastDFS/Minio | ð | |
ä»»å¡è°åº¦ | Elastic-Job | ð | |
ååºå表 | Mycat | ð | |
æ°æ®æé | ð | 使ç¨mybatiså¯¹åæ¥è¯¢åå¢å¼ºï¼ä¸å¡ä»£ç ä¸ç¨æ§å¶ï¼å³å¯å®ç°ã |
å¹³å°åè½
æå¡ | ä½¿ç¨ææ¯ | è¿åº¦ | 夿³¨ |
---|---|---|---|
ç¨æ·ç®¡ç | èªå¼å | â | ç¨æ·æ¯ç³»ç»æä½è ï¼è¯¥åè½ä¸»è¦å®æç³»ç»ç¨æ·é ç½®ã |
è§è²ç®¡ç | èªå¼å | â | è§è²èåæéåé ã设置è§è²ææºæè¿è¡æ°æ®èå´æéååã |
èå管ç | èªå¼å | ð | é 置系ç»èåï¼æä½æéï¼æé®æéæ è¯çã |
æºæç®¡ç | èªå¼å | ð | é 置系ç»ç»ç»æºæï¼æ ç»æå±ç°ï¼å¯éæè°æ´ä¸ä¸çº§ã |
ç½å ³å¨æè·¯ç± | èªå¼å | ð | ç½å ³å¨æè·¯ç±ç®¡ç |
å¼åè¿ç»´
æå¡ | ä½¿ç¨ææ¯ | è¿åº¦ | 夿³¨ |
---|---|---|---|
代ç çæ | ð | åå端代ç ççæï¼æ¯æVue | |
æµè¯ç®¡ç | ð | ||
ææ¡£ç®¡ç | Swagger2 | â | |
æå¡çæ§ | Spring Boot Admin | â | |
é¾è·¯è¿½è¸ª | SkyWalking | â | |
æä½å®¡è®¡ | ð | ç³»ç»å ³é®æä½æ¥å¿è®°å½åæ¥è¯¢ | |
æ¥å¿ç®¡ç | ES + KibanaãZipkin | â | |
çæ§åè¦ | Grafana | â |
æ´æ°æ¥å¿
2019-10-18ï¼
1.使ç¨nacosæ¿ä»£eureka为æå¡ç注åä¸å¿
2.使ç¨nacosæ¿ä»£apollo为æå¡çé ç½®ä¸å¿
3.å¼å ¥ä½¿ç¨sentinelæ¿æ¢æhystrixï¼å¼å ¥sentinel-dashboard
4.使ç¨jetcacheä½ä¸¤çº§ç¼åï¼ä¼åç¼åæ§è½
5.ç½å ³å¯å¨æ¶å è½½æ°æ®åºä¸çè·¯ç±å°redisç¼å
6.å ¶å®å·²ç¥bugä¿®å¤
è系交æµ
å å ¥è´¡ç®ä»£ç
è¯·å ¥ç¾¤ 请æ³è¿é å 群主微信ã
请ä½è å饮æ
å¦æä½ è§çæå¸®å©å°æ¨ï¼å¯ä»¥è¯·ä½è å饮æï¼è¿æ ·æ´æå¨åï¼è°¢è°¢ã
å¦ä¹ 交æµ
EMailï¼zhoutaoo@foxmail.com
群1ã2ã3ã4ã5ã6ã7ã8ã9已满ï¼è¯·å 群10ï¼å 群请æ³è¿é
æ¤äºç¾¤ä» ä¸ºææ¯äº¤æµç¾¤ï¼è¯·å¤§å®¶ä¸è¦è®¨è®ºæ¿æ²»ãå广åçä¸ææ¯æ å ³çä¸è¥¿ã大家å¦è¥æé®é¢å¯ä»¥å¨ç¾¤éç´æ¥åé®ï¼æä¼æ½ç©ºçå¤ã
请大家é®é®é¢æ¶å°½éæè¿°æ¸ æ¥èæ¯ä¸é®é¢å ³é®ä¿¡æ¯ï¼æè¿°çè¶æ¸ æ¥è¶å®¹æå¾å°çæ¡ã乿´å®¹æå¤æé®é¢å¯è½ç¹ï¼èçå¤æçæ¶é´ï¼èä¸ç¨æ¥åé®çã
åæ¶ä¹é¼å±ç¾¤å们积æåå¤å·²ç¥çé®é¢ï¼å¤§å®¶ç¸äºå¸®å©å ±åæé¿ã妿bugææ°éæ±ä¹å¯ä»¥ç´æ¥æäº¤issueå°githubï¼æä¼é æ å¤çã
å¦æä½ åç°ä½ çé®é¢å¾ä¹ 齿²¡æäººçå¤ï¼é£å¾æå¯è½å°±æ¯é®é¢æè¿°çä¸å¤æ¸ æ¥ï¼å«äººæ æ³åå¤ã
**é®é®é¢çä¸è¦ç´ **
-
说æèæ¯ï¼ä½¿ç¨äºåªä¸ªæ¨¡åï¼è¦åä»ä¹ï¼
-
æä¹è¾å ¥ææä½çå¾å°äºä»ä¹ç»æï¼ æªå¾ï¼æ¥å¿
-
åªéä¸æç½ææä»ä¹çé® ï¼
Stargazers over time
Top Related Projects
Spring Cloud Alibaba provides a one-stop solution for application development for the distributed solutions of Alibaba middleware.
Integration with Netflix OSS components
mall项目是一套电商系统,包括前台商城系统及后台管理系统,基于SpringBoot+MyBatis实现,采用Docker容器化部署。 前台商城系统包含首页门户、商品推荐、商品搜索、商品展示、购物车、订单流程、会员中心、客户服务、帮助中心等模块。 后台管理系统包含商品管理、订单管理、会员管理、促销管理、运营管理、内容管理、统计报表、财务管理、权限管理、设置等模块。
一个涵盖六个专栏:Spring Boot 2.X、Spring Cloud、Spring Cloud Alibaba、Dubbo、分布式消息队列、分布式事务的仓库。希望胖友小手一抖,右上角来个 Star,感恩 1024
《史上最简单的Spring Cloud教程源码》
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot