Top Related Projects
Fast, unopinionated, minimalist web framework for node.
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
The API and real-time application framework
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Expressive middleware for node.js using ES2017 async functions
Quick Overview
Sails.js is a popular open-source Node.js web application framework that follows the Model-View-Controller (MVC) architectural pattern. It is designed to make it easier to build custom, enterprise-grade Node.js applications.
Pros
- Rapid Development: Sails.js provides a set of tools and conventions that allow developers to quickly build and deploy production-ready Node.js applications.
- Scalability: Sails.js is built on top of the Express.js web framework, which is known for its scalability and performance.
- Flexibility: Sails.js is highly customizable and allows developers to easily integrate with various databases, APIs, and other third-party services.
- Active Community: Sails.js has a large and active community of developers who contribute to the project, provide support, and create a wide range of plugins and extensions.
Cons
- Steep Learning Curve: Sails.js has a relatively steep learning curve, especially for developers who are new to Node.js and the MVC architectural pattern.
- Performance Overhead: Sails.js adds some performance overhead due to its abstraction layer and the additional features it provides on top of Express.js.
- Limited Documentation: While the Sails.js documentation is generally good, some areas may be lacking in depth or clarity, especially for more advanced use cases.
- Monolithic Architecture: Sails.js encourages a monolithic architecture, which may not be the best fit for all types of applications, especially as they grow in complexity.
Code Examples
Here are a few examples of how to use Sails.js:
- Creating a new Sails.js project:
npm install -g sails
sails new my-project
cd my-project
sails lift
- Defining a model:
// api/models/User.js
module.exports = {
attributes: {
name: { type: 'string', required: true },
email: { type: 'string', unique: true, required: true },
createdAt: { type: 'number', autoCreatedAt: true },
updatedAt: { type: 'number', autoUpdatedAt: true }
}
};
- Creating a controller:
// api/controllers/UserController.js
module.exports = {
create: function (req, res) {
User.create(req.body).exec(function (err, user) {
if (err) return res.negotiate(err);
return res.json(user);
});
},
// Other actions like find, update, and destroy
};
- Defining a route:
// config/routes.js
module.exports.routes = {
'POST /users': 'UserController.create',
// Other routes
};
Getting Started
To get started with Sails.js, follow these steps:
-
Install Node.js and npm (the Node.js package manager) on your system.
-
Install the Sails.js command-line interface (CLI) globally using npm:
npm install -g sails
-
Create a new Sails.js project:
sails new my-project
-
Change into the project directory:
cd my-project
-
Start the Sails.js development server:
sails lift
-
Open your web browser and navigate to
http://localhost:1337
to see the default Sails.js welcome page. -
Begin building your application by creating models, controllers, and routes as needed. Sails.js provides a set of generators to help you quickly scaffold new components:
sails generate api user
This will create a new model and controller for a
User
resource. -
Customize your application by modifying the generated files and adding additional functionality as needed.
Competitor Comparisons
Fast, unopinionated, minimalist web framework for node.
Pros of Express
- Lightweight and minimalist, offering more flexibility and control
- Larger ecosystem with extensive middleware and plugins
- Faster performance due to its simplicity
Cons of Express
- Requires more manual setup and configuration
- Less opinionated, which can lead to inconsistent project structures
- Lacks built-in features like ORM, making it less suitable for rapid development
Code Comparison
Express:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
Sails:
// api/controllers/HelloController.js
module.exports = {
index: function (req, res) {
return res.send('Hello World!');
}
};
Express provides a more direct approach to routing, while Sails uses a controller-based structure. Sails offers a higher level of abstraction, which can be beneficial for larger applications but may feel overly complex for simple projects.
Express is ideal for developers who want full control over their application structure and prefer to choose their own tools and libraries. Sails, on the other hand, is better suited for rapid development of data-driven applications, offering built-in ORM, WebSocket support, and a more opinionated project structure.
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
Pros of Nest
- Built on TypeScript, offering strong typing and better tooling support
- Modular architecture with dependency injection, promoting cleaner and more maintainable code
- Extensive ecosystem with built-in support for GraphQL, WebSockets, and microservices
Cons of Nest
- Steeper learning curve due to its complex architecture and TypeScript requirements
- Potentially slower development speed for simple applications compared to Sails
- Heavier framework with more boilerplate code
Code Comparison
Nest controller:
@Controller('cats')
export class CatsController {
@Get()
findAll(): string {
return 'This action returns all cats';
}
}
Sails controller:
module.exports = {
findAll: function(req, res) {
return res.send('This action returns all cats');
}
};
Nest offers a more structured approach with decorators and TypeScript, while Sails provides a simpler, more straightforward JavaScript implementation. Nest's approach may lead to better maintainability in larger projects, but Sails' simplicity can be advantageous for rapid development of smaller applications.
The API and real-time application framework
Pros of Feathers
- Lightweight and modular architecture, allowing for easier customization and scalability
- Strong support for real-time applications with built-in WebSocket integration
- Flexible database support, including SQL and NoSQL options
Cons of Feathers
- Steeper learning curve for developers new to its ecosystem
- Less opinionated structure compared to Sails, which may require more decision-making
Code Comparison
Feathers service creation:
const feathers = require('@feathersjs/feathers');
const app = feathers();
app.use('messages', {
async find() {
return [{ id: 1, text: 'Hello' }];
}
});
Sails model definition:
module.exports = {
attributes: {
text: { type: 'string', required: true },
user: { model: 'user' }
}
};
Both frameworks offer easy-to-use APIs for creating services or models, but Feathers focuses on a more functional approach, while Sails follows a more traditional ORM-like structure.
Feathers excels in real-time applications and provides more flexibility, while Sails offers a more structured, convention-over-configuration approach that may be easier for beginners to grasp. The choice between the two depends on project requirements and developer preferences.
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
Pros of Strapi
- More modern and actively maintained, with frequent updates and a larger community
- Provides a user-friendly admin panel out-of-the-box for content management
- Offers a plugin system for easy extensibility and customization
Cons of Strapi
- Steeper learning curve for developers new to headless CMS concepts
- Less flexible for building complex, custom applications compared to Sails
- Requires more server resources due to its feature-rich nature
Code Comparison
Strapi (Content-Type definition):
module.exports = {
attributes: {
title: {
type: 'string',
required: true
},
content: {
type: 'richtext'
}
}
};
Sails (Model definition):
module.exports = {
attributes: {
title: { type: 'string', required: true },
content: { type: 'string' }
}
};
Both frameworks use similar syntax for defining data models, but Strapi offers more built-in content types like 'richtext' for enhanced content management capabilities.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Pros of AdonisJS
- More modern and actively maintained, with frequent updates and a growing ecosystem
- Built-in TypeScript support, providing better type safety and developer experience
- Robust CLI tools for scaffolding and development, enhancing productivity
Cons of AdonisJS
- Smaller community and fewer third-party packages compared to Sails
- Steeper learning curve for developers new to TypeScript or opinionated frameworks
Code Comparison
AdonisJS:
import { HttpContextContract } from '@ioc:Adonis/Core/HttpContext'
export default class UsersController {
public async index({ response }: HttpContextContract) {
const users = await User.all()
return response.json(users)
}
}
Sails:
module.exports = {
async find(req, res) {
const users = await User.find();
return res.json(users);
}
};
Both frameworks offer MVC architecture and RESTful API development, but AdonisJS leverages TypeScript for enhanced type safety and developer experience. Sails, being older, has a larger community and more third-party integrations but may lack some modern features. AdonisJS provides a more structured approach with its dependency injection system, while Sails offers more flexibility in its configuration. Ultimately, the choice between the two depends on project requirements, team expertise, and preference for TypeScript or JavaScript.
Expressive middleware for node.js using ES2017 async functions
Pros of Koa
- Lightweight and minimalist, offering better performance and flexibility
- Supports modern JavaScript features like async/await out of the box
- Highly extensible with a rich ecosystem of middleware
Cons of Koa
- Requires more setup and configuration for complex applications
- Steeper learning curve for developers new to Node.js or Express-style frameworks
- Smaller community and fewer resources compared to more established frameworks
Code Comparison
Sails.js (Controller):
module.exports = {
hello: function(req, res) {
return res.send('Hello, World!');
}
};
Koa.js:
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = 'Hello, World!';
});
Sails provides a more structured, opinionated approach with built-in MVC architecture, while Koa offers a minimal foundation that developers can build upon. Sails is better suited for rapid development of full-stack applications, whereas Koa shines in scenarios where developers need fine-grained control over their application structure and middleware stack.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Website Β Get Started Β Docs Β News Β Submit Issue
Sails.js is a web framework that makes it easy to build custom, enterprise-grade Node.js apps. It is designed to resemble the MVC architecture from frameworks like Ruby on Rails, but with support for the more modern, data-oriented style of web app & API development. It's especially good for building realtime features like chat.
Since version 1.0, Sails supports await
out of the box. This replaces nested callbacks (and the commensurate error handling) with simple, familiar usage:
var orgs = await Organization.find();
Installation Β
# Get the latest stable release of Sails
$ npm install sails -g
Upgrading from an earlier version of Sails?
Upgrade guides for all major releases since 2013 are available on the Sails website under Upgrading.
Your First Sails Project
Create a new app:
# Create the app
sails new my-app
Lift sails:
# cd into the new folder
cd my-app
# fire up the server
sails lift
For the most up-to-date introduction to Sails, get started here.
Compatibility
Sails is built on Node.js, Express, and Socket.io.
Sails actions are compatible with Connect middleware, so in most cases, you can paste code into Sails from an existing Express project and everything will work-- plus you'll be able to use WebSockets to talk to your API, and vice versa.
The ORM, Waterline, has a well-defined adapter system for supporting all kinds of datastores. Officially supported databases include MySQL, PostgreSQL, MongoDB, Redis, and local disk / memory. Community adapters exist for CouchDB, neDB, SQLite, Oracle, MSSQL, DB2, ElasticSearch, Riak, neo4j, OrientDB, Amazon RDS, DynamoDB, Azure Tables, RethinkDB and Solr; for various 3rd-party REST APIs like Quickbooks, Yelp, and Twitter, including a configurable generic REST API adapter; plus some eclectic projects.
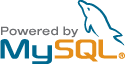



For the latest core adapters and notable community adapters, see Available Adapters.
Tutorial Course
- Sailscasts, taught by Kelvin Omereshone (English)
- Full-Stack JavaScript with Sails.js and Vue.js, taught by Mike McNeil (in English, with optional Spanish subtitles)
Books
- Sails.js in Action by Mike McNeil and Irl Nathan (Manning Publications).
- Sails.js Essentials by Shaikh Shahid (Packt)
- Pro Express.js: Part 3 by Azat Mardan (Apress).
Support
Need help or have a question?
Issue submission
Please read the submission guidelines and code of conduct before opening a new issue. Click here to search/post issues in this repository.
Contribute
There are many different ways you can contribute to Sails:
- answering questions on StackOverflow, Gitter, Facebook, or Twitter
- improving the documentation
- translating the documentation to your native language
- writing tests
- writing a tutorial, giving a talk, or supporting your local Sails meetup
- troubleshooting reported issues
- and submitting patches.
Please carefully read our contribution guide and check the build status for the relevant branch before submitting a pull request with code changes.
Links
Team
Sails is actively maintained with the help of many amazing contributors. Our core team consists of:
Mike McNeil | Kelvin Omereshone | Eric Shaw |
Our company designs/builds Node.js websites and apps for startups and enterprise customers. After building a few applications and taking them into production, we realized that the Node.js development landscape was very much still the Wild West. Over time, after trying lots of different methodologies, we decided to crystallize all of our best practices into this framework. Six years later, Sails is now one of the most widely-used web application frameworks in the world. I hope it saves you some time! :)
License
MIT License Copyright ΓΒ© 2012-present, Mike McNeil
Sails is built around so many great open-source technologies that it would never have crossed our minds to keep it proprietary. We owe huge gratitude and props to Ryan Dahl (@ry), TJ Holowaychuk (@tj), Doug Wilson (@dougwilson) and Guillermo Rauch (@rauchg) for the work they've done, as well as the stewards of all the other open-source modules we use. Sails could never have been developed without your tremendous contributions to the JavaScript community.
Top Related Projects
Fast, unopinionated, minimalist web framework for node.
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript π
The API and real-time application framework
π Strapi is the leading open-source headless CMS. Itβs 100% JavaScript/TypeScript, fully customizable, and developer-first.
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
Expressive middleware for node.js using ES2017 async functions
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot