Top Related Projects
React Native date & time picker component for iOS, Android and Windows
React Native Calendar Components 🗓️ 📆
React Native Date Picker is datetime picker for Android and iOS. It includes date, time and datetime picker modes. The datepicker is customizable and is supporting different languages. It's written with native code to achieve the best possible look, feel and performance.
react native datePicker component for both Android and IOS, useing DatePikcerAndroid, TimePickerAndroid and DatePickerIOS
A React-Native datetime-picker for Android and iOS
Minimalistic graph card for Home Assistant Lovelace UI
Quick Overview
React Native Calendars is a customizable calendar component library for React Native applications. It offers a range of calendar views, including day, week, month, and agenda, with support for various styling options and event management features.
Pros
- Highly customizable with extensive styling options
- Supports multiple calendar views (day, week, month, agenda)
- Includes features like marking specific dates and handling events
- Well-documented with regular updates and active community support
Cons
- Can be complex to set up for advanced use cases
- Some users report performance issues with large datasets
- Limited built-in internationalization support
- Occasional bugs and inconsistencies across different React Native versions
Code Examples
- Basic Calendar:
import {Calendar} from 'react-native-calendars';
<Calendar
onDayPress={(day) => {console.log('selected day', day)}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'}
}}
/>
- Agenda View:
import {Agenda} from 'react-native-calendars';
<Agenda
items={{
'2023-05-22': [{name: 'Meeting', time: '10:00 AM'}],
'2023-05-23': [{name: 'Lunch', time: '12:30 PM'}]
}}
renderItem={(item, firstItemInDay) => {
return (
<View>
<Text>{item.name}</Text>
<Text>{item.time}</Text>
</View>
);
}}
/>
- Customized Calendar:
<Calendar
theme={{
backgroundColor: '#ffffff',
calendarBackground: '#ffffff',
textSectionTitleColor: '#b6c1cd',
selectedDayBackgroundColor: '#00adf5',
selectedDayTextColor: '#ffffff',
todayTextColor: '#00adf5',
dayTextColor: '#2d4150',
textDisabledColor: '#d9e1e8',
dotColor: '#00adf5',
selectedDotColor: '#ffffff',
arrowColor: 'orange',
monthTextColor: 'blue',
indicatorColor: 'blue',
textDayFontFamily: 'monospace',
textMonthFontFamily: 'monospace',
textDayHeaderFontFamily: 'monospace',
textDayFontWeight: '300',
textMonthFontWeight: 'bold',
textDayHeaderFontWeight: '300',
textDayFontSize: 16,
textMonthFontSize: 16,
textDayHeaderFontSize: 16
}}
/>
Getting Started
-
Install the package:
npm install react-native-calendars
-
Import and use the component:
import {Calendar} from 'react-native-calendars'; function MyComponent() { return ( <Calendar onDayPress={(day) => {console.log('selected day', day)}} markedDates={{ '2023-05-16': {selected: true, marked: true, selectedColor: 'blue'} }} /> ); }
-
Customize as needed using the available props and theme options.
Competitor Comparisons
React Native date & time picker component for iOS, Android and Windows
Pros of datetimepicker
- Native date and time picker components for both iOS and Android
- Lightweight and focused on date/time selection functionality
- Easy integration with React Native core components
Cons of datetimepicker
- Limited to date and time selection, lacks calendar view and advanced features
- Less customizable in terms of appearance and behavior
- Requires separate handling for iOS and Android due to platform differences
Code Comparison
datetimepicker:
import DateTimePicker from '@react-native-community/datetimepicker';
<DateTimePicker
value={date}
mode="date"
display="default"
onChange={onChange}
/>
react-native-calendars:
import { Calendar } from 'react-native-calendars';
<Calendar
onDayPress={(day) => {console.log('selected day', day)}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'}
}}
/>
The datetimepicker provides a native date picker experience, while react-native-calendars offers a more customizable calendar view with additional features like marking specific dates. The choice between the two depends on the specific requirements of your application, such as the need for a full calendar view versus a simple date/time selector.
React Native Calendar Components 🗓️ 📆
Pros of react-native-calendars
- Comprehensive calendar component with various customization options
- Supports both iOS and Android platforms
- Well-documented API with examples and usage instructions
Cons of react-native-calendars
- May have a steeper learning curve due to its extensive features
- Larger package size compared to simpler calendar implementations
- Some users report occasional performance issues with complex configurations
Code Comparison
Both repositories contain the same codebase, as they are the same project. Here's a sample of how to use the calendar component:
import {Calendar} from 'react-native-calendars';
<Calendar
onDayPress={(day) => {console.log('selected day', day)}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'},
'2023-05-17': {marked: true},
'2023-05-18': {marked: true, dotColor: 'red', activeOpacity: 0}
}}
/>
This code snippet demonstrates how to create a basic calendar with marked dates and a day press handler. The component offers extensive customization options for styling and functionality.
React Native Date Picker is datetime picker for Android and iOS. It includes date, time and datetime picker modes. The datepicker is customizable and is supporting different languages. It's written with native code to achieve the best possible look, feel and performance.
Pros of react-native-date-picker
- Simpler implementation for basic date and time picking functionality
- Native look and feel on both iOS and Android platforms
- Smaller package size, potentially leading to better performance
Cons of react-native-date-picker
- Less customizable compared to react-native-calendars
- Focused solely on date/time picking, lacking calendar view and range selection
- Limited theming options
Code Comparison
react-native-date-picker:
import DatePicker from 'react-native-date-picker'
<DatePicker
date={new Date()}
onDateChange={setDate}
mode="date"
/>
react-native-calendars:
import {Calendar} from 'react-native-calendars'
<Calendar
onDayPress={day => {
console.log('selected day', day)
}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'}
}}
/>
react-native-date-picker is more straightforward for simple date selection, while react-native-calendars offers a full calendar view with more customization options. The choice between them depends on the specific requirements of your project, such as the need for a calendar view, range selection, or extensive customization.
react native datePicker component for both Android and IOS, useing DatePikcerAndroid, TimePickerAndroid and DatePickerIOS
Pros of react-native-datepicker
- Simpler implementation for basic date picking functionality
- Smaller package size, potentially leading to faster load times
- Easier to customize for specific date-only use cases
Cons of react-native-datepicker
- Less feature-rich compared to react-native-calendars
- Limited calendar view options
- Less active maintenance and community support
Code Comparison
react-native-datepicker:
<DatePicker
style={{width: 200}}
date={this.state.date}
mode="date"
placeholder="Select date"
format="YYYY-MM-DD"
onDateChange={(date) => {this.setState({date: date})}}
/>
react-native-calendars:
<Calendar
onDayPress={(day) => {console.log('selected day', day)}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'},
'2023-05-17': {marked: true},
'2023-05-18': {marked: true, dotColor: 'red', activeOpacity: 0}
}}
/>
The code comparison shows that react-native-datepicker offers a more straightforward implementation for basic date picking, while react-native-calendars provides more advanced features like marking specific dates and customizing their appearance. react-native-calendars is better suited for applications requiring a full calendar view with additional functionality, whereas react-native-datepicker is more appropriate for simple date selection tasks.
A React-Native datetime-picker for Android and iOS
Pros of react-native-modal-datetime-picker
- Focused on date and time picking functionality with a modal interface
- Simpler implementation for basic date/time selection needs
- Lightweight and easy to integrate into existing projects
Cons of react-native-modal-datetime-picker
- Limited to date and time picking, lacks calendar view and range selection
- Less customizable compared to react-native-calendars
- May require additional components for more complex date-related features
Code Comparison
react-native-modal-datetime-picker:
<DateTimePickerModal
isVisible={isDatePickerVisible}
mode="date"
onConfirm={handleConfirm}
onCancel={hideDatePicker}
/>
react-native-calendars:
<Calendar
onDayPress={(day) => {console.log('selected day', day)}}
markedDates={{
'2023-05-16': {selected: true, marked: true, selectedColor: 'blue'}
}}
/>
react-native-modal-datetime-picker is more suitable for simple date/time selection tasks with a modal interface, while react-native-calendars offers a full calendar view with more advanced features like range selection and customization options. The choice between the two depends on the specific requirements of your project and the level of calendar functionality needed.
Minimalistic graph card for Home Assistant Lovelace UI
Pros of mini-graph-card
- Specifically designed for Home Assistant, offering seamless integration
- Lightweight and customizable, allowing for easy embedding in dashboards
- Supports various graph types and styles, including line, bar, and area charts
Cons of mini-graph-card
- Limited to Home Assistant ecosystem, not as versatile for other platforms
- Fewer calendar-specific features compared to react-native-calendars
- May require more manual configuration for complex data visualization
Code Comparison
mini-graph-card:
type: custom:mini-graph-card
entities:
- sensor.temperature
- sensor.humidity
hours_to_show: 24
points_per_hour: 4
line_color: var(--primary-color)
react-native-calendars:
import {Calendar} from 'react-native-calendars';
<Calendar
markedDates={{
'2023-05-16': {selected: true, marked: true},
'2023-05-17': {marked: true},
'2023-05-18': {disabled: true}
}}
/>
The code snippets demonstrate the different focus areas of each library. mini-graph-card is configured using YAML and is tailored for displaying sensor data in graph form, while react-native-calendars uses JSX and is designed for rendering and interacting with calendar views in React Native applications.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
React Native Calendars ðï¸ ð
A declarative cross-platform React Native calendar component for iOS and Android.
This module includes information on how to use this customizable React Native calendar component.
The package is compatible with both Android and iOS
Official documentation
This README provides basic examples of how to get started with
react-native-calendars
. For detailed information, refer to the official documentation site.
Features â¨
- Pure JS. No Native code required
- Date marking - dot, multi-dot, period, multi-period and custom marking
- Customization of style, content (days, months, etc) and dates
- Detailed documentation and examples
- Swipeable calendar with flexible custom rendering
- Scrolling to today, selecting dates, and more
- Allowing or blocking certain dates
- Accessibility support
- Automatic date formatting for different locales
Try it out â¡
You can run a sample module using these steps:
$ git clone git@github.com:wix/react-native-calendars.git
$ cd react-native-calendars
$ yarn install
$ cd ios && pod install && cd ..
$ react-native run-ios
You can check example screens source code in example module screens
This project is compatible with Expo/CRNA (without ejecting), and the examples have been published on Expo
Getting Started ð§
Here's how to get started with react-native-calendars in your React Native project:
Install the package:
$ yarn add react-native-calendars
RN Calendars is implemented in JavaScript, so no native module linking is required.
Usage ð
Basic usage examples of the library
For detailed information on using this component, see the official documentation site
Importing the Calendar
component
import {Calendar, CalendarList, Agenda} from 'react-native-calendars';
Use the Calendar
component in your app:
<Calendar
onDayPress={day => {
console.log('selected day', day);
}}
/>
Some Code Examples
Here are a few code snippets that demonstrate how to use some of the key features of react-native-calendars:
Creating a basic calendar with default settings:
import React, {useState} from 'react';
import {Calendar, LocaleConfig} from 'react-native-calendars';
const App = () => {
const [selected, setSelected] = useState('');
return (
<Calendar
onDayPress={day => {
setSelected(day.dateString);
}}
markedDates={{
[selected]: {selected: true, disableTouchEvent: true, selectedDotColor: 'orange'}
}}
/>
);
};
export default App;
Customize the appearance of the calendar:
<Calendar
// Customize the appearance of the calendar
style={{
borderWidth: 1,
borderColor: 'gray',
height: 350
}}
// Specify the current date
current={'2012-03-01'}
// Callback that gets called when the user selects a day
onDayPress={day => {
console.log('selected day', day);
}}
// Mark specific dates as marked
markedDates={{
'2012-03-01': {selected: true, marked: true, selectedColor: 'blue'},
'2012-03-02': {marked: true},
'2012-03-03': {selected: true, marked: true, selectedColor: 'blue'}
}}
/>
Configuring the locale:
import {LocaleConfig} from 'react-native-calendars';
import React, {useState} from 'react';
import {Calendar, LocaleConfig} from 'react-native-calendars';
LocaleConfig.locales['fr'] = {
monthNames: [
'Janvier',
'Février',
'Mars',
'Avril',
'Mai',
'Juin',
'Juillet',
'Août',
'Septembre',
'Octobre',
'Novembre',
'Décembre'
],
monthNamesShort: ['Janv.', 'Févr.', 'Mars', 'Avril', 'Mai', 'Juin', 'Juil.', 'Août', 'Sept.', 'Oct.', 'Nov.', 'Déc.'],
dayNames: ['Dimanche', 'Lundi', 'Mardi', 'Mercredi', 'Jeudi', 'Vendredi', 'Samedi'],
dayNamesShort: ['Dim.', 'Lun.', 'Mar.', 'Mer.', 'Jeu.', 'Ven.', 'Sam.'],
today: "Aujourd'hui"
};
LocaleConfig.defaultLocale = 'fr';
const App = () => {
const [selected, setSelected] = useState('');
return (
<Calendar
onDayPress={day => {
setSelected(day.dateString);
}}
markedDates={{
[selected]: {selected: true, disableTouchEvent: true, selectedDotColor: 'orange'}
}}
/>
);
};
export default App;
Adding a global theme to the calendar:
<Calendar
style={{
borderWidth: 1,
borderColor: 'gray',
height: 350,
}}
theme={{
backgroundColor: '#ffffff',
calendarBackground: '#ffffff',
textSectionTitleColor: '#b6c1cd',
selectedDayBackgroundColor: '#00adf5',
selectedDayTextColor: '#ffffff',
todayTextColor: '#00adf5',
dayTextColor: '#2d4150',
textDisabledColor: '#dd99ee'
}}
</Calendar>
Customized Calendar Examples
Calendar

Dot marking

Multi-Dot marking
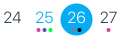
Period Marking


Multi-Period marking

Custom marking

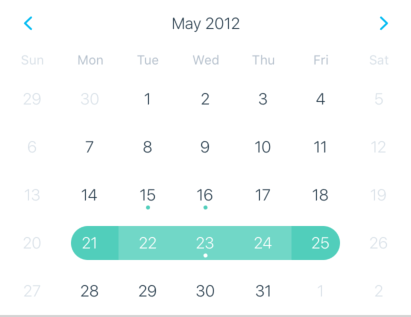
Date loading indicator

Scrollable semi-infinite calendar

Horizontal calendar

Agenda

Authors
- Tautvilas Mecinskas - Initial code - @tautvilas
- Katrin Zotchev - Initial design - @katrin_zot
See also the list of contributors who participated in this project.
Contributing
We welcome contributions to react-native-calendars.
If you have an idea for a new feature or have discovered a bug, please open an issue.
Please yarn test
and yarn lint
before pushing changes.
Don't forget to add a title and a description explaining the issue you're trying to solve and your proposed solution.
Screenshots and Gifs are VERY helpful to add to the PR for reviews.
Please DO NOT format the files - we're trying to keep a unified syntax and keep the reviewing process fast and simple.
License
React Native Calendars is MIT licensed
Top Related Projects
React Native date & time picker component for iOS, Android and Windows
React Native Calendar Components 🗓️ 📆
React Native Date Picker is datetime picker for Android and iOS. It includes date, time and datetime picker modes. The datepicker is customizable and is supporting different languages. It's written with native code to achieve the best possible look, feel and performance.
react native datePicker component for both Android and IOS, useing DatePikcerAndroid, TimePickerAndroid and DatePickerIOS
A React-Native datetime-picker for Android and iOS
Minimalistic graph card for Home Assistant Lovelace UI
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot