BottomNavigation
This Library helps users to use Bottom Navigation Bar (A new pattern from google) with ease and allows ton of customizations
Top Related Projects
(Deprecated) A custom view component that mimics the new Material Design Bottom Navigation pattern.
A library to reproduce the behavior of the Bottom Navigation guidelines from Material Design.
An android lib for enhancing BottomNavigationView. 一个增强BottomNavigationView的安卓库。
An bottom navigation bar for Android
Bottom Navigation widget component inspired by the Google Material Design Guidelines at https://www.google.com/design/spec/components/bottom-navigation.html
Yet another material bottom bar library for Android
Quick Overview
BottomNavigation is an Android library that provides an implementation of the Bottom Navigation component from Material Design. It offers a customizable and easy-to-use solution for adding a bottom navigation bar to Android applications, allowing users to quickly switch between top-level views.
Pros
- Easy integration with minimal setup required
- Highly customizable appearance and behavior
- Supports both fixed and shifting modes
- Compatible with older Android versions (API 14+)
Cons
- Limited to bottom navigation use cases
- May require additional customization for complex layouts
- Not officially supported by Google (third-party library)
- Potential for conflicts with other navigation libraries
Code Examples
- Basic setup of BottomNavigation:
val bottomNavigation: BottomNavigationView = findViewById(R.id.bottom_navigation)
bottomNavigation.setOnNavigationItemSelectedListener { item ->
when(item.itemId) {
R.id.action_home -> {
// Handle home action
true
}
R.id.action_search -> {
// Handle search action
true
}
R.id.action_profile -> {
// Handle profile action
true
}
else -> false
}
}
- Customizing the appearance:
val bottomNavigation: BottomNavigationView = findViewById(R.id.bottom_navigation)
bottomNavigation.setBackgroundColor(ContextCompat.getColor(this, R.color.colorPrimary))
bottomNavigation.itemIconTintList = null
bottomNavigation.itemTextColor = ContextCompat.getColorStateList(this, R.color.bottom_nav_colors)
- Adding badges to menu items:
val bottomNavigation: BottomNavigationView = findViewById(R.id.bottom_navigation)
val badge = bottomNavigation.getOrCreateBadge(R.id.action_notifications)
badge.isVisible = true
badge.number = 99
Getting Started
- Add the dependency to your app's
build.gradle
file:
dependencies {
implementation 'com.ashokvarma.android:bottom-navigation-bar:2.2.0'
}
- Add the BottomNavigationView to your layout XML:
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_navigation"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:menu="@menu/bottom_navigation_menu" />
- Create a menu resource file (
bottom_navigation_menu.xml
) with your navigation items:
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/action_home"
android:icon="@drawable/ic_home"
android:title="Home" />
<item
android:id="@+id/action_search"
android:icon="@drawable/ic_search"
android:title="Search" />
<item
android:id="@+id/action_profile"
android:icon="@drawable/ic_profile"
android:title="Profile" />
</menu>
- Initialize and set up the BottomNavigationView in your activity or fragment as shown in the code examples above.
Competitor Comparisons
(Deprecated) A custom view component that mimics the new Material Design Bottom Navigation pattern.
Pros of BottomBar
- More customizable appearance with extensive theming options
- Supports badges and notifications out of the box
- Smoother animations and transitions between tabs
Cons of BottomBar
- Larger library size compared to BottomNavigation
- Less frequently updated and maintained
- May require more setup and configuration for basic usage
Code Comparison
BottomNavigation:
bottomNavigation.add(BottomNavigationItem(R.drawable.ic_home, "Home"))
bottomNavigation.add(BottomNavigationItem(R.drawable.ic_search, "Search"))
bottomNavigation.add(BottomNavigationItem(R.drawable.ic_profile, "Profile"))
bottomNavigation.setOnTabSelectedListener { position, wasSelected ->
// Handle tab selection
}
BottomBar:
bottomBar.setItems(
BottomBarTab(R.drawable.ic_home, "Home"),
BottomBarTab(R.drawable.ic_search, "Search"),
BottomBarTab(R.drawable.ic_profile, "Profile")
)
bottomBar.setOnTabSelectListener { tabId ->
// Handle tab selection
}
Both libraries offer similar functionality for creating bottom navigation bars in Android applications. BottomNavigation provides a more straightforward API with less configuration required, while BottomBar offers more customization options and built-in features like badges. The choice between the two depends on the specific needs of your project and the level of customization desired.
A library to reproduce the behavior of the Bottom Navigation guidelines from Material Design.
Pros of ahbottomnavigation
- More customization options for the bottom navigation bar appearance
- Supports landscape mode and tablet layouts
- Includes a notification badge feature out of the box
Cons of ahbottomnavigation
- Less frequently updated compared to BottomNavigation
- Slightly more complex implementation due to additional features
- May have a steeper learning curve for beginners
Code Comparison
BottomNavigation:
val bottomNavigation = findViewById<BottomNavigationView>(R.id.bottom_navigation)
bottomNavigation.setOnNavigationItemSelectedListener { item ->
when(item.itemId) {
R.id.action_home -> // Handle home action
R.id.action_profile -> // Handle profile action
}
true
}
ahbottomnavigation:
val bottomNavigation = findViewById<AHBottomNavigation>(R.id.bottom_navigation)
bottomNavigation.setOnTabSelectedListener { position, wasSelected ->
when(position) {
0 -> // Handle home action
1 -> // Handle profile action
}
true
}
Both libraries offer similar basic functionality for implementing bottom navigation in Android apps. BottomNavigation provides a simpler, more straightforward implementation, while ahbottomnavigation offers more advanced features and customization options at the cost of slightly increased complexity.
An android lib for enhancing BottomNavigationView. 一个增强BottomNavigationView的安卓库。
Pros of BottomNavigationViewEx
- Built on top of the official BottomNavigationView, providing extended functionality
- Supports dynamic badge updates and customization
- Offers more flexibility in styling and behavior customization
Cons of BottomNavigationViewEx
- May require more setup and configuration compared to BottomNavigation
- Less actively maintained (last update was in 2020)
- Potentially larger library size due to additional features
Code Comparison
BottomNavigation:
BottomNavigationBar bottomNavigationBar = (BottomNavigationBar) findViewById(R.id.bottom_navigation_bar);
bottomNavigationBar
.addItem(new BottomNavigationItem(R.drawable.ic_home, "Home"))
.addItem(new BottomNavigationItem(R.drawable.ic_search, "Search"))
.initialise();
BottomNavigationViewEx:
BottomNavigationViewEx bnve = (BottomNavigationViewEx) findViewById(R.id.bnve);
bnve.enableAnimation(false);
bnve.enableShiftingMode(false);
bnve.enableItemShiftingMode(false);
bnve.setTextVisibility(false);
Both libraries provide easy-to-use APIs for implementing bottom navigation in Android apps. BottomNavigation offers a simpler setup process, while BottomNavigationViewEx provides more advanced customization options. The choice between the two depends on the specific requirements of your project and the level of customization needed.
An bottom navigation bar for Android
Pros of PagerBottomTabStrip
- More customization options for tab appearance and behavior
- Built-in support for ViewPager integration
- Smaller library size, potentially reducing app bloat
Cons of PagerBottomTabStrip
- Less active maintenance and updates compared to BottomNavigation
- Fewer stars and forks on GitHub, indicating potentially lower community adoption
- Documentation is primarily in Chinese, which may be challenging for non-Chinese speakers
Code Comparison
BottomNavigation:
BottomNavigationBar bottomNavigationBar = (BottomNavigationBar) findViewById(R.id.bottom_navigation_bar);
bottomNavigationBar
.addItem(new BottomNavigationItem(R.drawable.ic_home, "Home"))
.addItem(new BottomNavigationItem(R.drawable.ic_search, "Search"))
.initialise();
PagerBottomTabStrip:
BottomTabLayout bottomTabLayout = (BottomTabLayout) findViewById(R.id.bottomTabLayout);
bottomTabLayout.setTabItems(
new TabItem(this, R.drawable.ic_home, "Home", Color.BLUE),
new TabItem(this, R.drawable.ic_search, "Search", Color.RED)
);
Both libraries offer similar functionality for creating bottom navigation bars in Android applications. BottomNavigation provides a more straightforward API with built-in Material Design compliance, while PagerBottomTabStrip offers more flexibility in customization and ViewPager integration. The choice between the two depends on specific project requirements and developer preferences.
Bottom Navigation widget component inspired by the Google Material Design Guidelines at https://www.google.com/design/spec/components/bottom-navigation.html
Pros of Material-BottomNavigation
- More customizable appearance with support for custom behaviors and animations
- Better adherence to Material Design guidelines
- Includes a CoordinatorLayout Behavior for scrolling content
Cons of Material-BottomNavigation
- Less actively maintained (last update was 3 years ago)
- More complex implementation, potentially steeper learning curve
- Fewer stars and forks on GitHub, indicating less community adoption
Code Comparison
BottomNavigation:
bottomNavigation.addItem(BottomNavigationItem(R.drawable.ic_home, "Home"))
bottomNavigation.addItem(BottomNavigationItem(R.drawable.ic_search, "Search"))
bottomNavigation.setFirstSelectedPosition(0)
bottomNavigation.initialise()
Material-BottomNavigation:
bottomNavigation.setDefaultSelectedIndex(0)
bottomNavigation.setOnMenuItemClickListener { item ->
when (item.id) {
R.id.home -> // Handle home click
R.id.search -> // Handle search click
}
}
Both libraries offer similar functionality for creating bottom navigation bars in Android applications. BottomNavigation provides a simpler API and is more actively maintained, making it a good choice for developers who prioritize ease of use and ongoing support. Material-BottomNavigation offers more customization options and closer adherence to Material Design guidelines, which may be preferable for projects requiring a high degree of visual polish or specific design requirements.
Yet another material bottom bar library for Android
Pros of ReadableBottomBar
- More customizable animation options for item transitions
- Supports text labels alongside icons for better accessibility
- Lighter weight library with fewer dependencies
Cons of ReadableBottomBar
- Less mature project with fewer contributors and updates
- Limited documentation and usage examples compared to BottomNavigation
- Fewer built-in styling options out of the box
Code Comparison
ReadableBottomBar:
val bottomBar = findViewById<ReadableBottomBar>(R.id.bottomBar)
bottomBar.setOnItemSelectListener { index ->
when (index) {
0 -> // Handle first item selection
1 -> // Handle second item selection
}
}
BottomNavigation:
val bottomNavigation = findViewById<AHBottomNavigation>(R.id.bottom_navigation)
bottomNavigation.setOnTabSelectedListener { position, wasSelected ->
when (position) {
0 -> // Handle first tab selection
1 -> // Handle second tab selection
}
true
}
Both libraries offer similar functionality for implementing bottom navigation in Android apps. ReadableBottomBar provides more animation customization and text label support, while BottomNavigation has a more established ecosystem and extensive documentation. The code usage is relatively similar, with minor differences in method names and listener implementations.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
BottomNavigation
get sample apk from Google Play Store
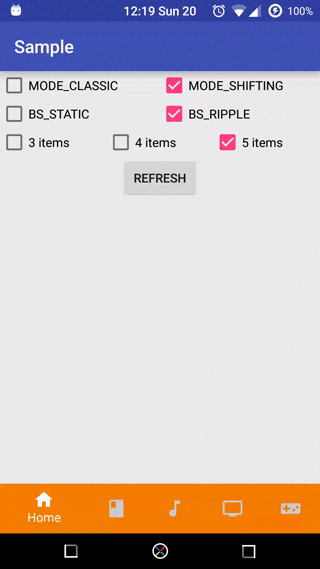
What is this component about?
This component that mimics the new Material Design Bottom Navigation pattern.
(currently under active development, expect to see new releases almost daily)
Features
- This library offers ton of customisations that you can do to Bottom Navigation Bar.
- Follows google bottom navigation bar guidelines
- Choose your background style and tab mode.
- each tab has it's own colors
- supports badges with complete customization
Download
Based on your IDE you can import library in one of the following ways
Download the latest JAR or grab via Maven:
<dependency>
<groupId>com.ashokvarma.android</groupId>
<artifactId>bottom-navigation-bar</artifactId>
<version>2.2.0</version>
<type>pom</type>
</dependency>
or Gradle:
implementation 'com.ashokvarma.android:bottom-navigation-bar:2.2.0'
or Ivy:
<dependency org='com.ashokvarma.android' name='bottom-navigation-bar' rev='2.2.0'>
<artifact name='$AID' ext='pom'/>
</dependency>
For Usage Docs Visit Wiki
Migration from V1 to V2
- BadgeItem has been changed to TextBadgeItem
- New ShapeBadgeItem implementation changed. check this page for new api usage
- hideText replaced with new modes. To use those mode should be set to MODE_FIXED_NO_TITLE / MODE_SHIFTING_NO_TITLE
License
BottomNavigation library for Android
Copyright (c) 2016 Ashok Varma (http://ashokvarma.me/).
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Other Open Source Libraries
- Gander : Gander is a simple in-app HTTP inspector for Android OkHttp clients. Gander intercepts and persists all HTTP requests and responses inside your application, and provides a UI for inspecting their content.
- SqliteManager : Sqlite Manager is a Dev Debug tool that helps to manage(Edit, Add, Clear) your android Sqlite Databases.
- SharedPrefManager : SharedPref Manager is a Dev Debug tool that helps to manage(Edit, Add, Clear) your android Shared Preferences.
Top Related Projects
(Deprecated) A custom view component that mimics the new Material Design Bottom Navigation pattern.
A library to reproduce the behavior of the Bottom Navigation guidelines from Material Design.
An android lib for enhancing BottomNavigationView. 一个增强BottomNavigationView的安卓库。
An bottom navigation bar for Android
Bottom Navigation widget component inspired by the Google Material Design Guidelines at https://www.google.com/design/spec/components/bottom-navigation.html
Yet another material bottom bar library for Android
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot