Top Related Projects
A Material Design ViewPager easy to use library
A circular ImageView for Android
An Android Animation library which easily add itemanimator to RecyclerView items.
You can easily add awesome animated context menu to your app.
😍 A beautiful, fluid, and extensible dialogs API for Kotlin & Android.
The flexible, easy to use, all in one drawer library for your Android project. Now brand new with material 2 design.
Quick Overview
Timber is an Android library for easy logging in Android applications. It provides a more flexible and powerful logging solution compared to Android's default Log class, with features like custom log adapters, automatic tagging, and crash reporting integration.
Pros
- Easy to set up and use with minimal configuration
- Supports multiple logging trees for different environments (debug, release)
- Automatic tagging based on the calling class
- Integrates well with crash reporting tools
Cons
- Limited built-in formatting options for log messages
- Requires manual removal of debug logging trees in release builds
- May introduce a small performance overhead compared to native Android logging
- Limited support for structured logging
Code Examples
- Basic logging:
Timber.d("Debug message")
Timber.i("Info message")
Timber.e("Error message")
- Logging with formatted strings:
val name = "John"
val age = 30
Timber.d("User: %s, Age: %d", name, age)
- Logging exceptions:
try {
// Some code that might throw an exception
} catch (e: Exception) {
Timber.e(e, "An error occurred")
}
- Custom logging tree:
class ReleaseTree : Timber.Tree() {
override fun log(priority: Int, tag: String?, message: String, t: Throwable?) {
if (priority == Log.ERROR) {
// Send error logs to crash reporting service
FirebaseCrashlytics.getInstance().log(message)
}
}
}
Getting Started
- Add the dependency to your app's
build.gradle
:
dependencies {
implementation 'com.jakewharton.timber:timber:5.0.1'
}
- Initialize Timber in your Application class:
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
if (BuildConfig.DEBUG) {
Timber.plant(Timber.DebugTree())
} else {
Timber.plant(ReleaseTree())
}
}
}
- Start logging in your code:
Timber.d("Logging is now set up!")
Competitor Comparisons
A Material Design ViewPager easy to use library
Pros of MaterialViewPager
- Offers a more comprehensive Material Design implementation with ViewPager integration
- Provides smoother animations and transitions between pages
- Includes built-in header customization options
Cons of MaterialViewPager
- Less focused on audio playback functionality
- May require more setup and configuration for basic usage
- Potentially higher learning curve for developers new to Material Design
Code Comparison
MaterialViewPager:
mViewPager = (MaterialViewPager) findViewById(R.id.materialViewPager);
mViewPager.getViewPager().setAdapter(new FragmentStatePagerAdapter(getSupportFragmentManager()) {
@Override
public Fragment getItem(int position) {
return RecyclerViewFragment.newInstance();
}
// ... other methods
});
Timber:
public class MainActivity extends BaseActivity implements ATEActivityThemeCustomizer {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// ... other initialization code
}
}
MaterialViewPager focuses on creating a ViewPager with Material Design elements, while Timber is more oriented towards audio playback functionality. MaterialViewPager offers more advanced UI customization out of the box, but Timber provides a simpler setup for audio-centric applications. Developers should choose based on their specific project requirements and desired user interface complexity.
A circular ImageView for Android
Pros of CircleImageView
- Focused on a specific UI component (circular image view)
- Lightweight and easy to integrate into existing projects
- Provides additional features like border and shadow customization
Cons of CircleImageView
- Limited in scope compared to Timber's broader logging functionality
- May require additional libraries for more complex image processing tasks
- Less actively maintained (last update was over a year ago)
Code Comparison
CircleImageView usage:
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="96dp"
android:layout_height="96dp"
android:src="@drawable/profile"
app:civ_border_width="2dp"
app:civ_border_color="#FF000000"/>
Timber usage:
Timber.d("Debug message")
Timber.e(exception, "Error message")
Summary
CircleImageView is a specialized UI component for displaying circular images in Android applications, while Timber is a logging utility. CircleImageView offers easy integration and customization for a specific UI element, whereas Timber provides a more comprehensive logging solution for Android development. The choice between these libraries depends on the specific needs of your project: UI enhancements or improved logging capabilities.
An Android Animation library which easily add itemanimator to RecyclerView items.
Pros of recyclerview-animators
- Focuses specifically on RecyclerView animations, providing a wide range of pre-built animation options
- Offers easy-to-use methods for implementing complex animations with minimal code
- Regularly updated and maintained, with a large community of users and contributors
Cons of recyclerview-animators
- Limited to RecyclerView animations, lacking broader functionality for other UI elements
- May require additional setup and configuration for more complex use cases
- Potential performance impact when using multiple or complex animations on large datasets
Code Comparison
recyclerview-animators:
val animator = SlideInLeftAnimator()
recyclerView.itemAnimator = animator
Timber:
Timber.plant(Timber.DebugTree())
Timber.d("Debug message")
Summary
recyclerview-animators is a specialized library for RecyclerView animations, offering a wide range of pre-built options and easy implementation. However, it's limited to RecyclerView and may have performance considerations for complex animations.
Timber, on the other hand, is a logging library that provides enhanced logging capabilities for Android applications. It offers a broader utility for debugging and logging across various parts of an app, not just UI animations.
The choice between these libraries depends on the specific needs of your project: recyclerview-animators for enhancing RecyclerView animations, or Timber for improved logging and debugging capabilities.
You can easily add awesome animated context menu to your app.
Pros of Context-Menu.Android
- Provides a visually appealing and customizable circular context menu
- Offers smooth animations and transitions for menu items
- Includes a demo app for easy implementation and testing
Cons of Context-Menu.Android
- Limited to circular menu layout, less flexible than Timber's logging options
- Focused on UI elements, while Timber is a more versatile logging utility
- May require more setup and customization for specific use cases
Code Comparison
Context-Menu.Android:
MenuObject close = new MenuObject();
close.setResource(R.drawable.icn_close);
menuObjects.add(close);
Timber:
Timber.d("Debug message");
Timber.e(exception, "Error message");
Summary
Context-Menu.Android is a specialized UI library for creating circular context menus in Android applications. It offers attractive animations and customization options but is limited to this specific use case. Timber, on the other hand, is a versatile logging utility that provides a simple API for debugging and error logging across Android applications. While Context-Menu.Android enhances the visual aspect of an app, Timber focuses on improving the development and debugging process.
😍 A beautiful, fluid, and extensible dialogs API for Kotlin & Android.
Pros of Material Dialogs
- More comprehensive dialog solution with a wider range of customization options
- Actively maintained with frequent updates and bug fixes
- Extensive documentation and examples for easier implementation
Cons of Material Dialogs
- Larger library size, potentially increasing app size
- Steeper learning curve due to more complex API
- May include unnecessary features for simpler dialog requirements
Code Comparison
Material Dialogs:
MaterialDialog(this).show {
title(R.string.your_title)
message(R.string.your_message)
positiveButton(R.string.agree)
negativeButton(R.string.disagree)
}
Timber:
Timber.d("Debug message")
Timber.e(exception, "Error message")
Key Differences
- Purpose: Material Dialogs focuses on creating customizable dialogs, while Timber is a logging library
- Functionality: Material Dialogs offers UI components, whereas Timber provides logging utilities
- Integration: Material Dialogs requires more setup for UI elements, while Timber is simpler to integrate for logging purposes
Use Cases
- Material Dialogs: Best for apps requiring complex, customizable dialog interfaces
- Timber: Ideal for apps needing efficient and flexible logging capabilities
Community and Support
Both libraries have active communities, but Material Dialogs tends to have more frequent updates and contributions due to its broader scope and application in UI development.
The flexible, easy to use, all in one drawer library for your Android project. Now brand new with material 2 design.
Pros of MaterialDrawer
- More comprehensive drawer implementation with extensive customization options
- Actively maintained with frequent updates and bug fixes
- Larger community and better documentation
Cons of MaterialDrawer
- Steeper learning curve due to more complex API
- Potentially larger app size due to additional features
Code Comparison
MaterialDrawer:
drawer = DrawerBuilder()
.withActivity(this)
.withToolbar(toolbar)
.addDrawerItems(
PrimaryDrawerItem().withName("Home"),
SecondaryDrawerItem().withName("Settings")
)
.build()
Timber:
navigationView.setNavigationItemSelectedListener { menuItem ->
when (menuItem.itemId) {
R.id.nav_home -> // Handle home selection
R.id.nav_settings -> // Handle settings selection
}
true
}
Key Differences
- MaterialDrawer offers a more programmatic approach to drawer creation
- Timber uses Android's native NavigationView, which may be simpler for basic implementations
- MaterialDrawer provides more built-in item types and styling options
Use Cases
- MaterialDrawer: Complex drawer layouts with custom items and extensive theming
- Timber: Simpler drawer implementations using standard Android components
Community and Support
MaterialDrawer has a larger user base and more frequent updates, potentially offering better long-term support and feature additions compared to Timber.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Timber
Material Design Music Player
This project is no longer in active development. Please refer to TimberX instead
Screenshots
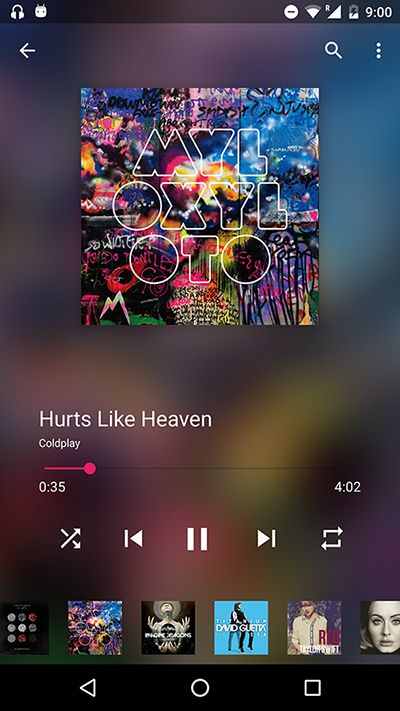
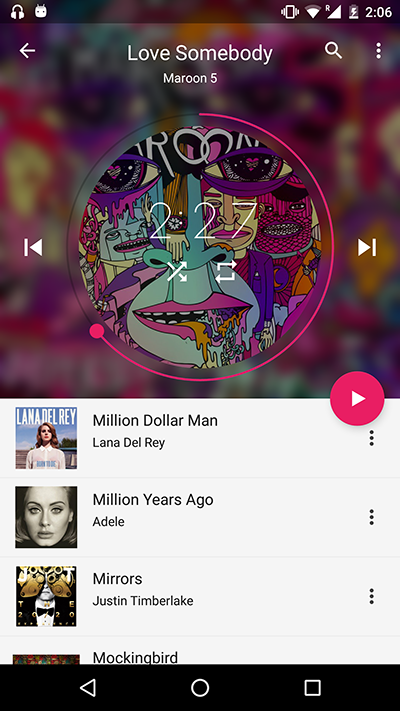
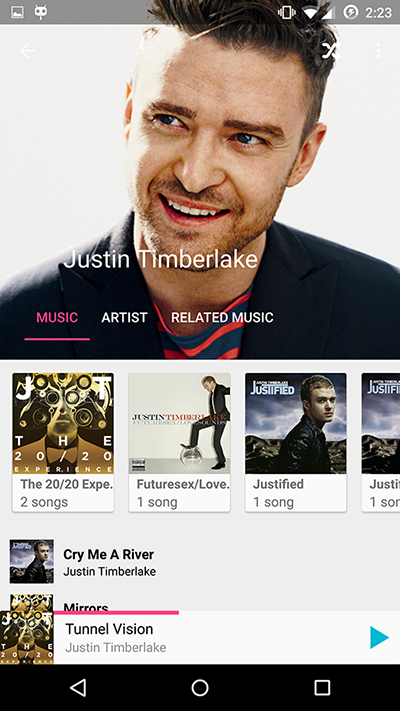
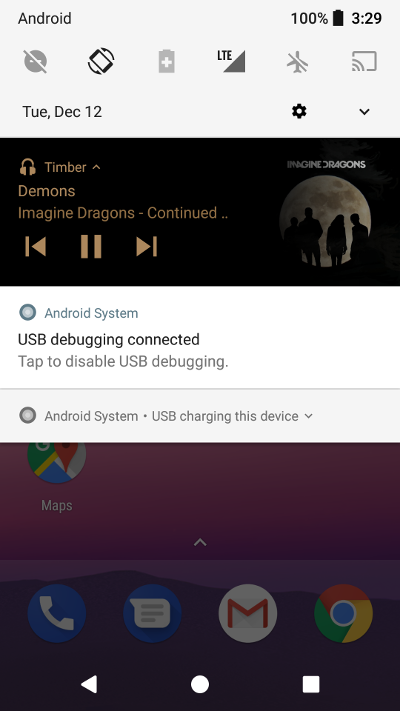
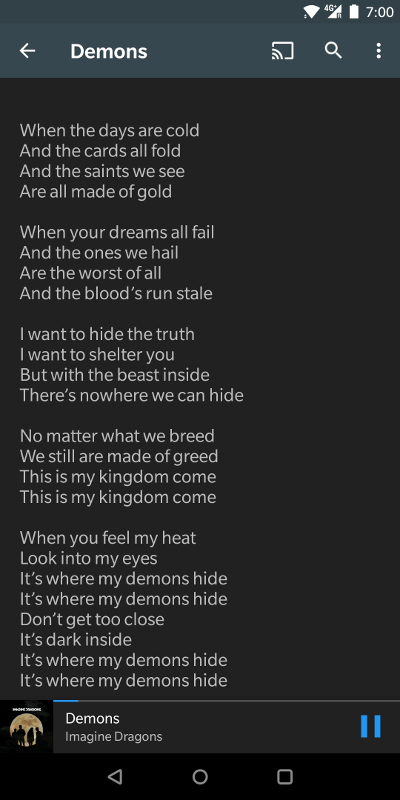
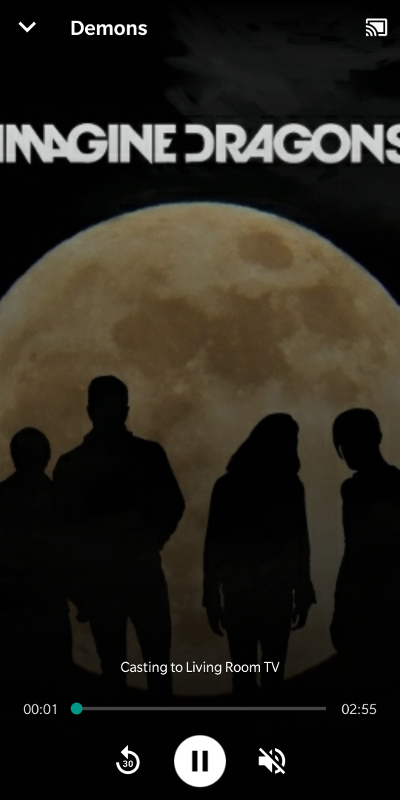
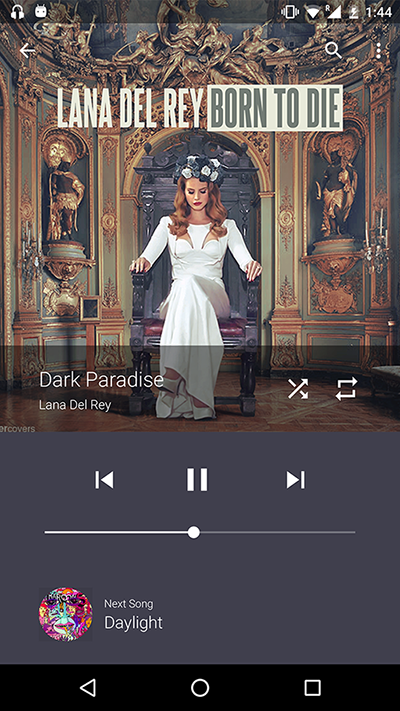
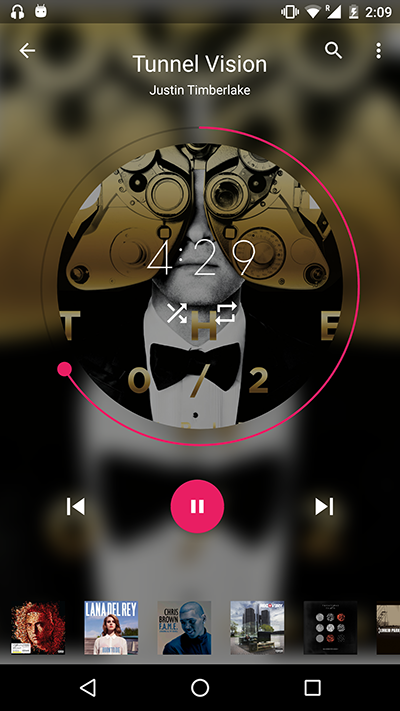
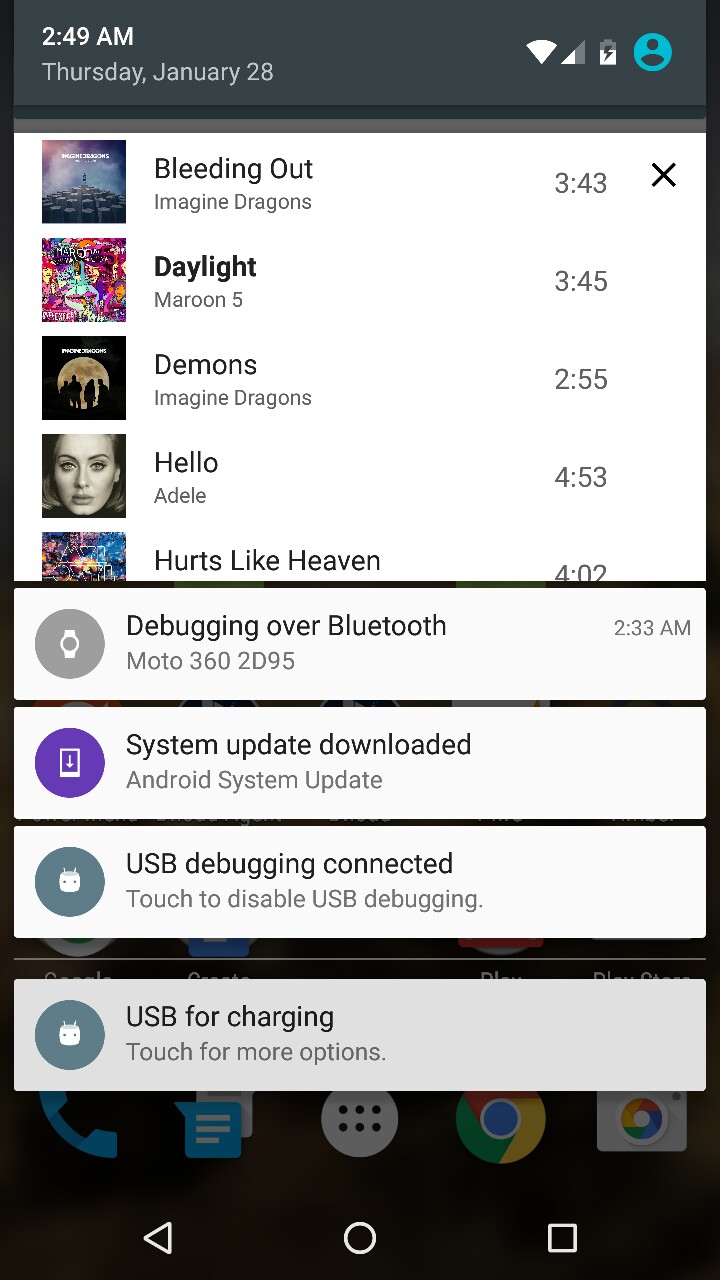
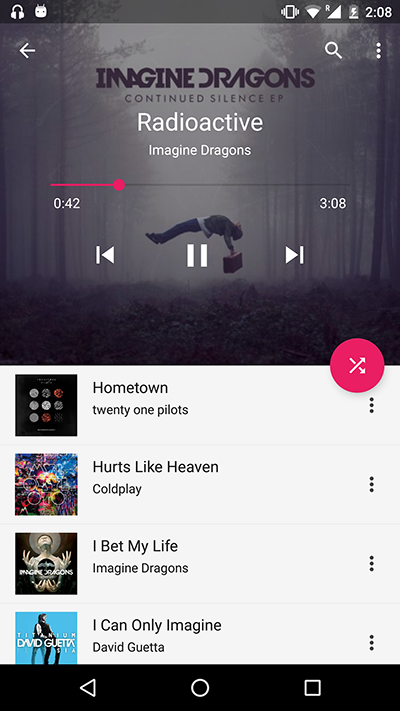
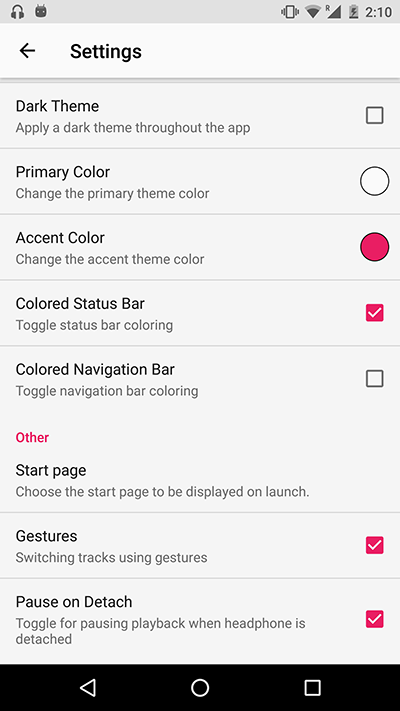
Features
- Material design
- Browse Songs, Albums, Artists
- Create and edit playlists
- 6 different now playing styles
- Homescreen widgets
- Browse device folders
- Dark theme and UI customisability
- Gestures for track switching
- LastFM scrobble
- Android Wear and Android Auto support
- Playing queue in notification (Xposed)
- Lyrics support
- Chromecast support
Changelog
Changelog is available here
Credits
- CyanogenMod's Eleven Music Player
- TimelyTextView
- MultiViewPager
- PlayPauseButton
- CircularSeekBar
- Nammu
Donate
Paypal donation email- namandwivedi14@gmail.com
License
(c) 2015 Naman Dwivedi
This is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version.
This software is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this app. If not, see https://www.gnu.org/licenses/.
Top Related Projects
A Material Design ViewPager easy to use library
A circular ImageView for Android
An Android Animation library which easily add itemanimator to RecyclerView items.
You can easily add awesome animated context menu to your app.
😍 A beautiful, fluid, and extensible dialogs API for Kotlin & Android.
The flexible, easy to use, all in one drawer library for your Android project. Now brand new with material 2 design.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot