Top Related Projects
:tada: A magical vue admin https://panjiachen.github.io/vue-element-admin
🎉 vue admin,vue3 admin,vue3.0 admin,vue后台管理,vue-admin,vue3.0-admin,admin,vue-admin,vue-element-admin,ant-design,vab admin pro,vab admin plus,vue admin plus,vue admin pro
A modern vue admin panel built with Vue3, Shadcn UI, Vite, TypeScript, and Monorepo. It's fast!
Naive Ui Admin 是一款基于 Vue3、Vite3 和 TypeScript 的先进中后台解决方案,集成了前沿的前端技术栈和典型业务模型。它拥有二次封装组件、动态菜单、权限校验、粒子化权限控制等核心功能,旨在帮助企业快速构建高质量的中后台项目。无论在新技术运用或业务实践层面,都能为您提供有力支持,并将持续更新,以满足您不断变化的需求
A clean, elegant, beautiful and powerful admin template, based on Vue3, Vite5, TypeScript, Pinia, NaiveUI and UnoCSS. 一个清新优雅、高颜值且功能强大的后台管理模板,基于最新的前端技术栈,包括 Vue3, Vite5, TypeScript, Pinia, NaiveUI 和 UnoCSS。
Vue3、Element Plus、typescript后台管理系统
Quick Overview
Vue Pure Admin is a free and open-source admin template based on Vue 3, Vite, and TypeScript. It offers a clean, modern interface with a variety of pre-built components and features, making it easier for developers to create robust admin panels and dashboards.
Pros
- Built with modern technologies (Vue 3, Vite, TypeScript)
- Comprehensive set of pre-built components and layouts
- Supports both light and dark themes
- Responsive design for various screen sizes
Cons
- Learning curve for developers new to Vue 3 or TypeScript
- May require customization for specific business needs
- Documentation is primarily in Chinese, which could be a barrier for non-Chinese speakers
Code Examples
- Creating a basic route:
const route: RouteRecordRaw = {
path: "/dashboard",
component: () => import("@/layout/index.vue"),
redirect: "/dashboard/analysis",
meta: {
icon: "homeFilled",
title: "Dashboard",
rank: 0
},
children: [
{
path: "analysis",
name: "Analysis",
component: () => import("@/views/dashboard/analysis/index.vue"),
meta: {
title: "Analysis"
}
}
]
};
- Using a pre-built component:
<template>
<pure-table
:data="tableData"
:columns="columns"
:pagination="pagination"
@page-change="handlePageChange"
/>
</template>
<script setup lang="ts">
import { ref } from "vue";
import { PureTable } from "@/components/Table";
const tableData = ref([/* ... */]);
const columns = [/* ... */];
const pagination = ref({ pageSize: 10, total: 100 });
const handlePageChange = (page: number) => {
// Handle page change
};
</script>
- Implementing dark mode toggle:
<template>
<el-switch
v-model="isDark"
inline-prompt
:active-icon="Sunny"
:inactive-icon="Moon"
@change="changeDarken"
/>
</template>
<script setup lang="ts">
import { ref } from "vue";
import { useLayout } from "@/layout/hooks/useLayout";
import { Sunny, Moon } from "@element-plus/icons-vue";
const { isDark, changeDarken } = useLayout();
</script>
Getting Started
-
Clone the repository:
git clone https://github.com/pure-admin/vue-pure-admin.git
-
Install dependencies:
cd vue-pure-admin pnpm install
-
Start the development server:
pnpm dev
-
Build for production:
pnpm build
Visit http://localhost:8848
to see your app running.
Competitor Comparisons
:tada: A magical vue admin https://panjiachen.github.io/vue-element-admin
Pros of vue-element-admin
- More comprehensive documentation and examples
- Larger community and ecosystem
- Extensive internationalization support
Cons of vue-element-admin
- Heavier and more complex, potentially overkill for smaller projects
- Less frequent updates compared to vue-pure-admin
- Steeper learning curve for beginners
Code Comparison
vue-element-admin:
import { mapGetters } from 'vuex'
export default {
computed: {
...mapGetters([
'sidebar',
'device',
'visitedViews',
'cachedViews'
])
}
}
vue-pure-admin:
import { useAppStore } from '@/store/modules/app'
export default {
setup() {
const appStore = useAppStore()
return {
sidebar: computed(() => appStore.sidebar),
device: computed(() => appStore.device)
}
}
}
The code comparison shows that vue-element-admin uses Vuex with mapGetters for state management, while vue-pure-admin employs the Composition API with Pinia for a more modern approach. vue-pure-admin's code is generally more concise and easier to read, especially for developers familiar with the Composition API.
🎉 vue admin,vue3 admin,vue3.0 admin,vue后台管理,vue-admin,vue3.0-admin,admin,vue-admin,vue-element-admin,ant-design,vab admin pro,vab admin plus,vue admin plus,vue admin pro
Pros of vue-admin-better
- More comprehensive documentation and examples
- Larger community and more frequent updates
- Better internationalization support
Cons of vue-admin-better
- Steeper learning curve due to more complex architecture
- Heavier bundle size, which may impact performance
- Less flexibility for customization compared to vue-pure-admin
Code Comparison
vue-admin-better:
<template>
<el-config-provider :locale="locale">
<router-view />
</el-config-provider>
</template>
vue-pure-admin:
<template>
<router-view v-slot="{ Component, route }">
<transition :name="getTransitionName(route)" mode="out-in">
<keep-alive v-if="keepAliveComponents" :include="keepAliveComponents">
<component :is="Component" :key="route.fullPath" />
</keep-alive>
<component v-else :is="Component" :key="route.fullPath" />
</transition>
</router-view>
</template>
The code comparison shows that vue-admin-better uses a simpler approach with el-config-provider for internationalization, while vue-pure-admin implements more advanced routing features like transitions and keep-alive components. This reflects the trade-off between ease of use and flexibility in the two projects.
A modern vue admin panel built with Vue3, Shadcn UI, Vite, TypeScript, and Monorepo. It's fast!
Pros of vue-vben-admin
- More comprehensive documentation and examples
- Larger community and more frequent updates
- Better internationalization support
Cons of vue-vben-admin
- Steeper learning curve due to complexity
- Heavier bundle size
- More opinionated structure, potentially less flexible
Code Comparison
vue-vben-admin:
import { defineComponent } from 'vue';
import { BasicForm, useForm } from '/@/components/Form';
import { formSchema } from './data';
export default defineComponent({
setup() {
const [registerForm, { setFieldsValue }] = useForm({
schemas: formSchema,
});
// ... more code
},
});
vue-pure-admin:
import { defineComponent, reactive } from 'vue';
import { ElForm } from 'element-plus';
export default defineComponent({
setup() {
const form = reactive({
name: '',
email: '',
});
// ... more code
},
});
The code comparison shows that vue-vben-admin uses a more abstracted approach with custom hooks and schemas, while vue-pure-admin opts for a simpler, more direct implementation using Vue 3's Composition API and Element Plus components.
Naive Ui Admin 是一款基于 Vue3、Vite3 和 TypeScript 的先进中后台解决方案,集成了前沿的前端技术栈和典型业务模型。它拥 有二次封装组件、动态菜单、权限校验、粒子化权限控制等核心功能,旨在帮助企业快速构建高质量的中后台项目。无论在新技术运用或业务实践层面,都能为您提供有力支持,并将持续更新,以满足您不断变化的需求
Pros of naive-ui-admin
- Built on Naive UI, offering a rich set of customizable components
- Includes a comprehensive permission management system
- Provides a more modern and sleek user interface design
Cons of naive-ui-admin
- Less extensive documentation compared to vue-pure-admin
- Smaller community and fewer contributors
- May have a steeper learning curve for developers new to Naive UI
Code Comparison
naive-ui-admin:
<template>
<n-config-provider :theme="theme">
<n-layout>
<!-- Layout content -->
</n-layout>
</n-config-provider>
</template>
vue-pure-admin:
<template>
<el-config-provider :locale="locale">
<router-view />
</el-config-provider>
</template>
The code snippets show that naive-ui-admin uses Naive UI components (n-config-provider, n-layout) while vue-pure-admin uses Element Plus components (el-config-provider). This reflects the different UI libraries each project is built upon.
Both projects offer robust admin template solutions for Vue.js applications, with vue-pure-admin having a larger community and more extensive documentation, while naive-ui-admin provides a modern interface and comprehensive permission management system. The choice between the two would depend on specific project requirements and developer preferences.
A clean, elegant, beautiful and powerful admin template, based on Vue3, Vite5, TypeScript, Pinia, NaiveUI and UnoCSS. 一个清新优雅、高颜值 且功能强大的后台管理模板,基于最新的前端技术栈,包括 Vue3, Vite5, TypeScript, Pinia, NaiveUI 和 UnoCSS。
Pros of soybean-admin
- More comprehensive documentation, including detailed setup instructions and component usage guides
- Stronger focus on TypeScript integration, providing better type safety and developer experience
- Includes built-in internationalization support, making it easier to create multilingual applications
Cons of soybean-admin
- Slightly steeper learning curve due to its more complex architecture and feature set
- May have more overhead for smaller projects that don't require all the included features
- Less frequent updates compared to vue-pure-admin
Code Comparison
soybean-admin:
import { defineComponent } from 'vue';
import { NLayout, NLayoutSider, NLayoutContent } from 'naive-ui';
export default defineComponent({
setup() {
// Component logic
}
});
vue-pure-admin:
<template>
<el-container>
<el-aside width="200px"><!-- Sidebar content --></el-aside>
<el-main><!-- Main content --></el-main>
</el-container>
</template>
<script>
export default {
// Component logic
}
</script>
The code comparison shows that soybean-admin uses Naive UI components and TypeScript, while vue-pure-admin uses Element Plus components and Vue's Options API. This reflects soybean-admin's focus on TypeScript integration and a different UI library choice.
Vue3、Element Plus、typescript后台管理系统
Pros of vue-manage-system
- Simpler and more lightweight, making it easier to understand and customize
- Includes a variety of pre-built components and layouts for quick development
- Well-documented with clear examples and usage instructions
Cons of vue-manage-system
- Less comprehensive feature set compared to vue-pure-admin
- May require more manual configuration for advanced functionalities
- Limited built-in themes and styling options
Code Comparison
vue-manage-system:
<template>
<div class="sidebar">
<el-menu class="sidebar-el-menu" :default-active="onRoutes" :collapse="collapse" background-color="#324157"
text-color="#bfcbd9" active-text-color="#20a0ff" unique-opened router>
<template v-for="item in items">
<template v-if="item.subs">
vue-pure-admin:
<template>
<el-menu
:default-active="activeMenu"
:collapse="getCollapse"
:unique-opened="getUniqueOpened"
:collapse-transition="false"
:background-color="menuBgColor"
:text-color="menuTextColor"
:active-text-color="menuActiveTextColor"
>
<sidebar-item
Both repositories offer Vue-based admin templates, but vue-pure-admin provides a more feature-rich and customizable solution, while vue-manage-system focuses on simplicity and ease of use. The code comparison shows differences in menu implementation, with vue-pure-admin offering more configuration options.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
vue-pure-admin
䏿 | English
ç®ä»
vue-pure-admin
æ¯ä¸æ¬¾å¼æºå
è´¹ä¸å¼ç®±å³ç¨çä¸åå°ç®¡çç³»ç»æ¨¡çãå®å
¨éç¨ ECMAScript
模åï¼ESM
ï¼è§èæ¥ç¼ååç»ç»ä»£ç ï¼ä½¿ç¨äºææ°ç Vue3
ã
Vite
ãElement-Plus
ãTypeScript
ãPinia
ãTailwindcss
çä¸»æµææ¯å¼å
ç åç念
稳å®ä¸æ±åæ°ï¼ææ¯ä¸è§æªæ¥
ç²¾ç®çæ¬ï¼å®é
项ç®å¼å请ç¨ç²¾ç®çæ¬ï¼æä¾ éå½é
å
ãå½é
å
ä¸¤ä¸ªçæ¬éæ©ï¼
ç²¾ç®çæ¬æ¯åºäº vue-pure-admin æç¼åºçæ¶åï¼å
å«ä¸»ä½åè½ï¼æ´éåå®é
项ç®å¼åï¼æå
åç大å°å¨å
¨å±å¼å
¥ element-plus çæ
åµä¸ä»ç¶ä½äº 2.3MB
ï¼å¹¶ä¸ä¼æ°¸ä¹
忥宿´çç代ç ãå¼å¯ brotli
å缩å cdn
æ¿æ¢æ¬å°åºæ¨¡å¼åï¼æå
大å°ä½äº 350kb
ç¹ææ¥çéå½é
åç²¾ç®çæ¬
ç¹ææ¥çå½é
åç²¾ç®çæ¬
é å¥è§é¢
ç¹ææ¥ç UI 设计
ç¹ææ¥çå¿«éå¼åæç¨
é å¥ä¿å§çº§ææ¡£
ç¹ææ¥ç vue-pure-admin ææ¡£
ç¹ææ¥ç @pureadmin/utils ææ¡£
ä¼è´¨æå¡ã软件å¤å ãèµå©æ¯æ
js
çæ¬
max
çæ¬
Tauri
çæ¬
Electron
çæ¬
é¢è§
PC
端
æè²é£æ ¼
ç§»å¨ç«¯
ä½¿ç¨ Gitpod
å¨ Gitpod
ï¼éç¨äº GitHub
çå
è´¹å¨çº¿å¼åç¯å¢ï¼ä¸æå¼é¡¹ç®ï¼å¹¶ç«å³å¼å§ç¼ç .
å®è£ 使ç¨
æå代ç
æ¨èä½¿ç¨ @pureadmin/cli
èææ¶
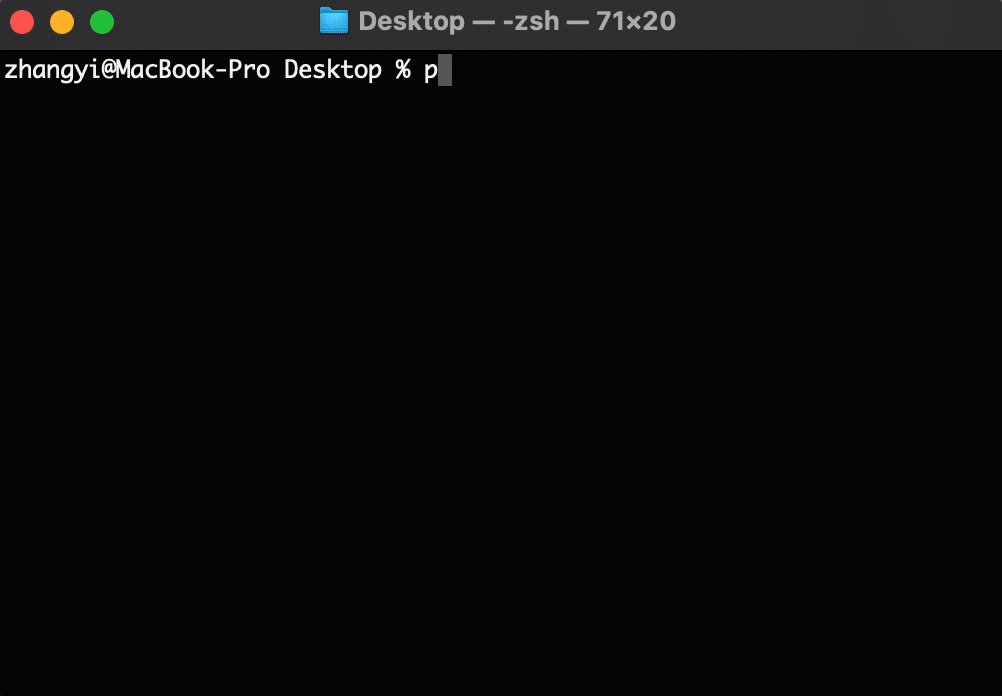
- å ¨å±å®è£
npm install -g @pureadmin/cli
- 交äºå¼éæ©æ¨¡æ¿å¹¶å建项ç®
pure create
ç¹ææ¥ç @pureadmin/cli èææ¶è¯¦ç»ç¨æ³
ä» GitHub
䏿å
git clone https://github.com/pure-admin/vue-pure-admin.git
ä» Gitee
䏿å
git clone https://gitee.com/yiming_chang/vue-pure-admin.git
å®è£ ä¾èµ
cd vue-pure-admin
pnpm install
å¯å¨å¹³å°
pnpm dev
é¡¹ç®æå
pnpm build
Docker æ¯æ
- èªå®ä¹éåå为
vue-pure-admin
çéåï¼è¯·æ³¨æä¸é¢å½ä»¤æ«å°¾æä¸ä¸ªç¹.
表示使ç¨å½åè·¯å¾ä¸çDockerfile
æä»¶ï¼å¯æ ¹æ®å®é æ 嵿å®è·¯å¾ï¼
docker build -t vue-pure-admin .
- ç«¯å£æ å°å¹¶å¯å¨
docker
容å¨ï¼8080:80
ï¼è¡¨ç¤ºå¨å®¹å¨ä¸ä½¿ç¨80
端å£ï¼å¹¶å°è¯¥ç«¯å£è½¬åå°ä¸»æºç8080
端å£ï¼pure-admin
ï¼è¡¨ç¤ºèªå®ä¹å®¹å¨åï¼vue-pure-admin
ï¼è¡¨ç¤ºèªå®ä¹éååï¼
docker run -dp 8080:80 --name pure-admin vue-pure-admin
æä½å®ä¸é¢ä¸¤ä¸ªå½ä»¤åï¼å¨æµè§å¨æå¼ http://localhost:8080
å³å¯é¢è§
å½ç¶ä¹å¯ä»¥éè¿ Docker Desktop å¯è§åçé¢å»æä½ docker
项ç®ï¼å¦ä¸å¾
æ´æ°æ¥å¿
å¦ä½è´¡ç®
éå¸¸æ¬¢è¿æ¨çå å
¥ï¼æä¸ä¸ª Issue æè
æäº¤ä¸ä¸ª Pull Request
Pull Request:
- Fork 代ç !
- å建èªå·±ç忝:
git checkout -b feat/xxxx
- æäº¤æ¨çä¿®æ¹:
git commit -am 'feat(function): add xxxxx'
- æ¨éæ¨ç忝:
git push origin feat/xxxx
- æäº¤
pull request
ç¹å«ä»£ç è´¡ç®
é常æè°¢ä½ ä»¬è½æ·±å
¥äºè§£æºç 并对 pure-admin
ç»ç»ä½åºä¼ç§è´¡ç® â¤ï¸
è´¡ç®äºº | å ·ä½ä»£ç |
---|---|
hb0730 | 代ç |
o-cc | 代ç |
yj-liuzepeng | 代ç |
skyline523 | 代ç |
shark-lajiao | 代ç |
WitMiao | 代ç |
QFifteen | 代ç |
edgexie | 代ç |
way-jm | 代ç |
simple-hui | 代ç |
Git
è´¡ç®æäº¤è§è
feat
å¢å æ°åè½fix
ä¿®å¤é®é¢/BUGstyle
代ç 飿 ¼ç¸å ³æ å½±åè¿è¡ç»æçperf
ä¼å/æ§è½æårefactor
éærevert
æ¤éä¿®æ¹test
æµè¯ç¸å ³docs
ææ¡£/注échore
ä¾èµæ´æ°/èææ¶é 置修æ¹çworkflow
工使µæ¹è¿ci
æç»éætypes
ç±»åå®ä¹æä»¶æ´æ¹wip
å¼åä¸
æµè§å¨æ¯æ
æ¬å°å¼åæ¨èä½¿ç¨ Chrome
ãEdge
ãFirefox
æµè§å¨ï¼ä½è
常ç¨çæ¯ææ°ç Chrome
æµè§å¨
å®é
使ç¨ä¸æè§ Firefox
å¨å¨ç»ä¸è¦æ¯å«çæµè§å¨æ´å 䏿»ï¼åªæ¯ä½è
ç¨ Chrome
å·²ç»ä¹ æ¯äºï¼ç个人ç±å¥½éæ©å§
æ´è¯¦ç»çæµè§å¨å
¼å®¹æ§æ¯æè¯·ç Vue æ¯æåªäºæµè§å¨ï¼ å Vite æµè§å¨å
¼å®¹æ§
![]() IE | ![]() Edge | ![]() Firefox | ![]() Chrome | ![]() Safari |
---|---|---|---|---|
䏿¯æ | æåä¸¤ä¸ªçæ¬ | æåä¸¤ä¸ªçæ¬ | æåä¸¤ä¸ªçæ¬ | æåä¸¤ä¸ªçæ¬ |
ç»´æ¤è
许å¯è¯
å®å ¨å è´¹å¼æº
MIT © 2020-present, pure-admin
Star
é常æè°¢ç䏿æç好å¿äººï¼æè°¢æ¨çæ¯æ :heart:
Fork
ç§ï¼é£äº å°å¥å¥
ãå°å§å§
认ç å¦ä¹
çæ ·åçæ»´æ¯ åå¦ä¸éå¦
:heart:
Top Related Projects
:tada: A magical vue admin https://panjiachen.github.io/vue-element-admin
🎉 vue admin,vue3 admin,vue3.0 admin,vue后台管理,vue-admin,vue3.0-admin,admin,vue-admin,vue-element-admin,ant-design,vab admin pro,vab admin plus,vue admin plus,vue admin pro
A modern vue admin panel built with Vue3, Shadcn UI, Vite, TypeScript, and Monorepo. It's fast!
Naive Ui Admin 是一款基于 Vue3、Vite3 和 TypeScript 的先进中后台解决方案,集成了前沿的前端技术栈和典型业务模型。它拥有二次封装组件、动态菜单、权限校验、粒子化权限控制等核心功能,旨在帮助企业快速构建高质量的中后台项目。无论在新技术运用或业务实践层面,都能为您提供有力支持,并将持续更新,以满足您不断变化的需求
A clean, elegant, beautiful and powerful admin template, based on Vue3, Vite5, TypeScript, Pinia, NaiveUI and UnoCSS. 一个清新优雅、高颜值且功能强大的后台管理模板,基于最新的前端技术栈,包括 Vue3, Vite5, TypeScript, Pinia, NaiveUI 和 UnoCSS。
Vue3、Element Plus、typescript后台管理系统
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot